Graph Applications
Please help me debug this code, expected output is attached below
#include
#include
#include
#include
#include
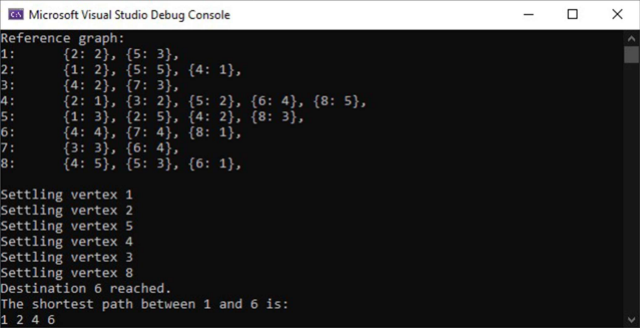
Extracted text: A Microsoft Visual Studio Debug Console Reference graph: O X {2: 2}, {5: 3}, {1: 2}, {5: 5}, {4: 1}, {4: 2}, {7: 3}, {2: 1}, {3: 2}, {5: 2}, {6: 4}, {8: 5}, {1: 3}, {2: 5}, {4: 2}, {8: 3}, {4: 4}, {7: 4}, {8: 1}, {3: 3}, {6: 4}, {4: 5}, {5: 3}, {6: 1}, 1: 2: 3: 4: 5: 6: 7: 8: Settling vertex 1 Settling vertex 2 Settling vertex 5 Settling vertex 4 Settling vertex 3 Settling vertex 8 Destination 6 reached. The shortest path between 1 and 6 is: 1 2 4 6