I can make the application but seem to always have issues with the Class UML Diagram. Below is the program I made and I attached the UML diagram. I was not completely sure about the
Guest guest1 = new Guest (" ", " ")
objects in the UML diagram. Any Advice?
//-------------------First class:
public class Guest {
// Attributes below
private String firstName;
private String lastName;
//constructor below
public Guest(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
// Overloaded constructor below
public Guest() {
this("No First Name Given", "No Last Name Given");
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
@Override
public String toString() {
return firstName + " " + lastName;
}
}
//------Second class
import java.util.ArrayList;
public class GuestList {
public static void main(String[]args) {
ArrayList nameList = new ArrayList ();
//Guest objects with names
Guest guest1 = new Guest("John", "Doe");
Guest guest2 = new Guest("Mary", "Smith");
Guest guest3 = new Guest("Jean", "Peterson");
Guest guest4 = new Guest("Sean", "Bott");
Guest guest5 = new Guest("Anna", "Hall");
//adds Guest Objects to the nameList array
nameList.add(guest1);
nameList.add(guest2);
nameList.add(guest3);
nameList.add(guest4);
nameList.add(guest5);
// Call the displayGuests().
System.out.println("Here is the list of guests.");
displayGuests(nameList);
System.out.println(); // for readability purposes
// Update the list.
// Add 2 more guests.
System.out.println("Adding Adriana Lima to the guest list.");
nameList.add(new Guest("Adriana","Lima"));
System.out.println(); // for readability purposes
System.out.println("Adding Robert Shabazz to the guest list.");
nameList.add(new Guest("Robert","Shabazz"));
System.out.println(); // for readability purposes
// Delete the guest who has registered first.
System.out.println("Deleting the first guest from the guest list...");
nameList.remove(0); // John Doe will be removed
System.out.println(); // for readability purposes
// Change the first name of guest 'Mary Smith' to 'Jane'.
System.out.println("Changing the first name of guest Mary Smith to Jane.");
for (Guest g : nameList) {
if (g.getFirstName().equalsIgnoreCase("Mary") &&
g.getLastName().equalsIgnoreCase("Smith")) {
g.setFirstName("Jane");
}
}
System.out.println(); // for readability purposes
// Call the displayGuests().
System.out.println("Here is the modified list of guests.");
displayGuests(nameList);
} // end main()
public static void displayGuests(ArrayList guestList) {
for (Guest guest : guestList) {
System.out.println(guest.toString());
}
} // end displayGuests()
}
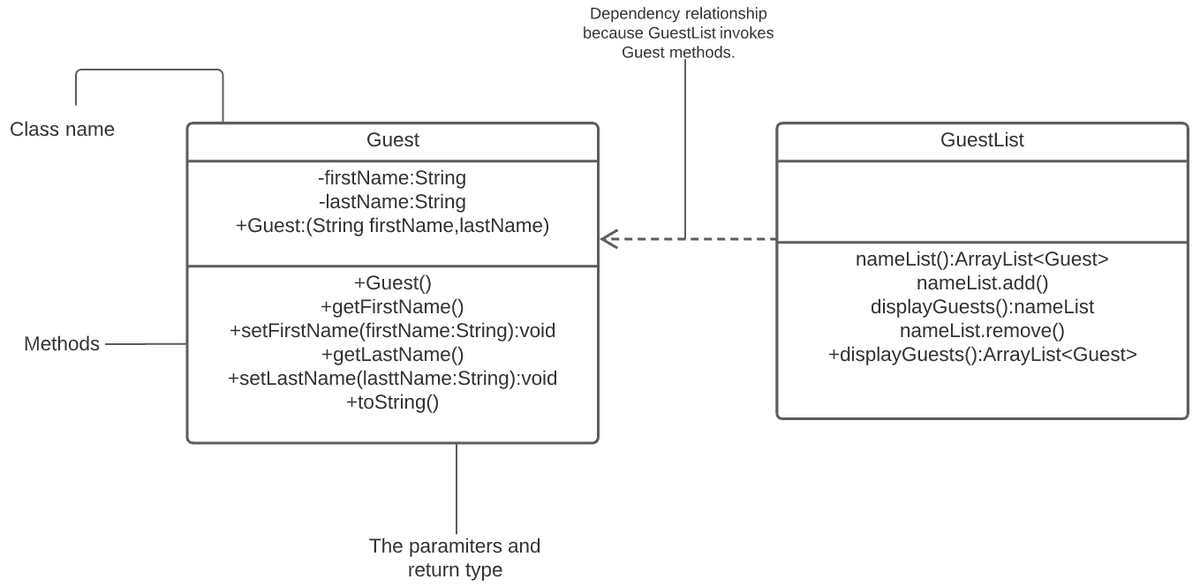
Extracted text: Dependency relationship because GuestList invokes Guest methods. Class name Guest GuestList -firstName:String -lastName:String +Guest:(String firstName, lastName) +Guest() +getFirstName() +setFirstName(firstName:String):void +getLastName() +setLastName(lasttName:String):void +toString() nameList():ArrayList nameList.add() displayGuests(:nameList nameList.remove() +displayGuests():ArrayList Methods The paramiters and return type