Implementing Breadth First Search
Please help me debug this code, the expected output is attached below.
#include
#include
#include
#include
#include
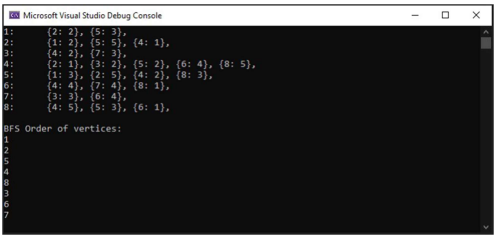
Extracted text: 3: 4: 5: 6: 7: 8: A Microsoft Visual Studio Debug Console (2: 2}, (5: 3), {1: 2}, (5: 5), (4: 1}, {4: 2}, (7: 3}, {2: 1}, {3: 2}, {5: 2}, {6: 4}, {8: 5}, {1: 3}, (2: 5}, (4: 2}, (8: 3}, (4: 4), (7: 4), (8: 1), {3: 3), (6: 4}, {4: 5}, {5: 3}, {6: 1}, BFS Order of vertices: