Overview and Requirements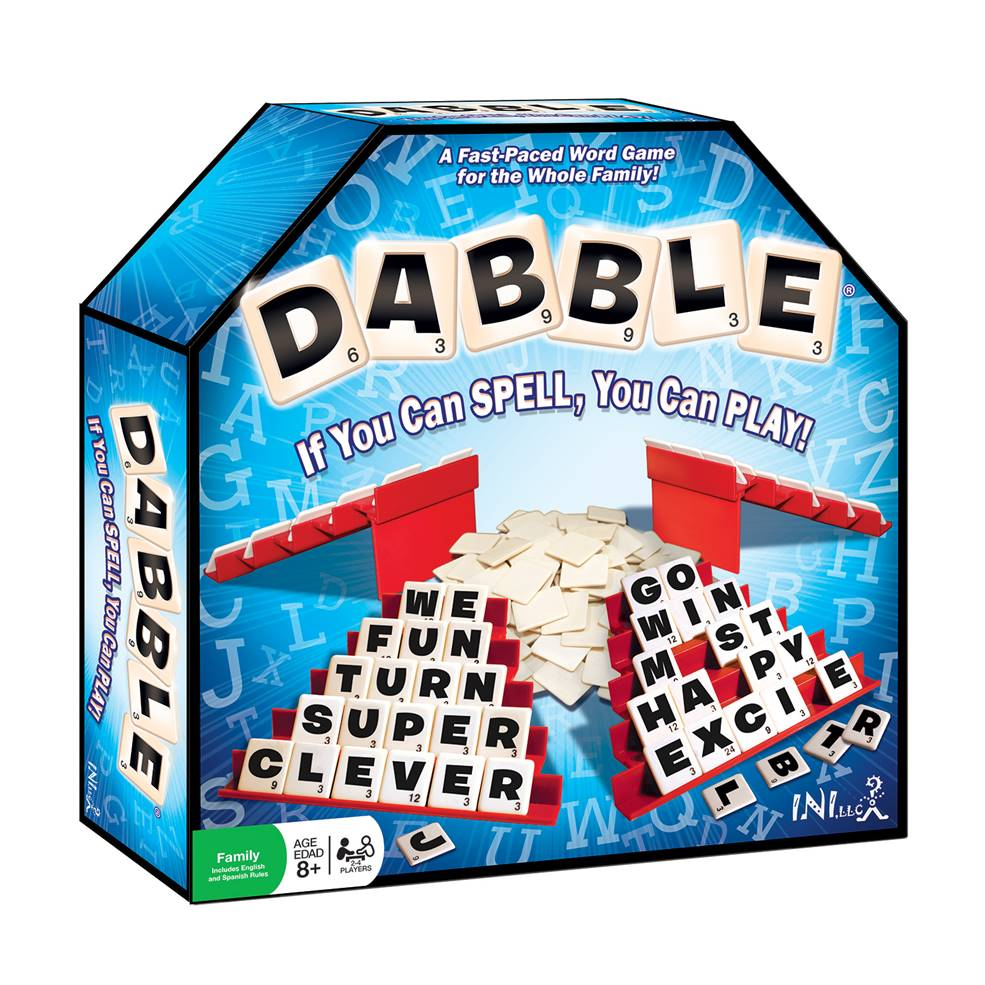
Write a command line program to play the game of
Dabble: “Dabble is a great learning tool for kids - and fun for the entire family! At the core of the game lies a fast-paced word game that will help children develop many useful skills - including vocabulary, spelling, and quick thinking.” If you are not familiar with the game, you can read about it
here. For our program, the game will be adapted as an simpler, untimed, 1 player game that is played as follows:
Program selects 20 random letter tiles from a “tile bag” for the user to play with
One at a time, the program prompts the user for a 2, 3, 4, 5, and 6 letter word made from all 20 tiles
Program reports the score of the user’s words and asks if they want to play again
Program Details
Show the user instructions on how to play Dabble.
Prepare the “tile bag” of 138 letter tiles using the following letter frequencies. The point values will be used later for scoring. Note: I found these values on
BoardGameGeek.
Select 20 random tiles from the tile bag for the user to play with.
Load words from
words_alpha.txt
(original file source
here) into an array or list. These will be used for validating user-entered words as real or not. Here is a function to do this that you may use (words_alpha.txt must be placed in your top level project directory):
The main game loop:
Display information regarding the status of the game (20 tiles and the number of letters in the word the user is trying to create).
Prompt the user to enter their word and validate it. If the user tries to guess a word that is the wrong number of letters, uses letters they do not have, or is not a real word (using words_alpha.txt), inform the user and re-prompt.
Once a valid word is entered, score it using the above letter point values. Inform the user of their word’s score.
Move on to the next word size. Note that we are allowing users to use all 20 letters for each word size (it is much easier this way!)
Exit the main game loop if the user comes up with all 5 words (thus winning the game) or if they enter “0” to give up/quit.
At the end of the game, inform the user their total score (sum of 5 words’ points) and prompt the user to see if they want to play again. If they do, select 20 new random tiles.
Note: You can assume the user will always enter a letter when a letter is needed. You do not need to worry about cases where the user may enter malformed input. We will cover this when we discuss exceptions and try/catch blocks.
Functions to Define
You are free to decide which functions to define to solve this problem. The first step is to draw a structure chart to help you understand the decomposition of functions for this program. Remember to start with the overall problem and break it down into inputs, computations, and outputs. Build your solution accordingly. You will be graded on your approach. Ask the instructor for help with this!
Note: code written for this warm-up Java assignment does not need to be object-oriented, all of your functions may be static. You are encouraged to apply OOP design if you’d like!
Grading Guidelines
This assignment is worth 50 points + 3 points bonus. Your assignment will be evaluated based on a successful compilation and adherence to the program requirements. We will grade according to the following criteria:
5 pts for correctly filling the tile bag
5 pts for dealing 20 random letters and displaying game state information to the user
5 pts for validating the user’s guess is the right length and uses the right tiles
5 pts for validating the user’s guess is a real word from words_alpha.txt
5 pts for correctly scoring the game
15 pts for correct game loop logic, including determining a winner
5 pts for function design
5 pts for adherence to
proper programming style and comments established for the class