Part 2: Programming - 75%
A program to compute the last digit of a barcode
If you look on the back of the box/label of any commercial product, you will find a barcode which represents its product code. A product code is used to uniquely identify it (e.g. when you're at the grocery store, the cashier scans the barcode with his/her barcode reader and instantly knows which product you are buying and its price).
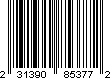
Product codes vary in length from 8 to 18 digits. You may have heard of these product codes referred to as UPCs (Universal Product Codes), but UPCs are only one of the many flavours of product codes found on items we buy every day:
Different Product codes and their lengths
Produce Code Name |
Length |
---|
EAN-8 |
8 |
UPC-A |
12 |
EAN-13 |
13 |
EAN-14 |
14 |
SSCC |
18 |
The last digit of every flavour of product code is a check digit used as a validity check. This lets the scanner check for errors in the scanning: if the check digit is right, it probably scanned correctly. The check digit is calculated by performing a calculation on the firstn-1 digits of an-digit product code.
Your programming task for this assignment will be to create a program which calculates the check digit for any of the different product codes listed above, given the firstn-1 digits of a product code. Your program should be created in a file calledupc.py
.
The algorithm to implement check digit calculation for any length product code is the same. Let's assume the first 11 digits of a UPC-A code are “23139085377” and we would like to derive the check digit:
- From the right to the left, starting with position 1 every other position is odd and starting with 2, every other position is even:
Position |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
---|
Even/Odd |
odd |
even |
odd |
even |
odd |
even |
odd |
even |
odd |
even |
odd |
---|
Digit |
2 |
3 |
1 |
3 |
9 |
0 |
8 |
5 |
3 |
7 |
7 |
---|
- Add together all the digits in the odd positions and multiply the result by 3:
(2 + 1 + 9 + 8 + 3 + 7) * 3 = 90
- Add together all the digits in the even positions:
3 + 3 + 0 + 5 + 7 = 18
- Add together these two numbers:
90 + 18 = 108
- Take the remainder of dividingthe negativeof this number by 10. (In Python the
%
operator gives the remainder.)
-108 % 10 = 2
So, in the example, the check digit is 2. The whole UPC code for this product is "231390853772".
Using the above described algorithm, create a program that:
- Asks the user which type of product code he/she would like to find the check digit for.
- Based on the user's choice of product code, asks the user for the firstn-1 digits of the product code. [Get the input as a string; it's easier to work with the string, so don't convert to an integer.]
- Using the user's input of the firstn-1 digits, finds the check digit.
- Displays the resulting product code.
Your program should interact with the user as follows:
Check Digit Calculator
--------------------
1. EAN-8
2. UPC-A
3. EAN-13
4. EAN-14
5. SSCC
--------------------
Choose your product code type:
2Enter first 11 digits of your product code:
23139085377--------------------
The check digit is 2.
The product code is 231390853772.
Remember, your program should ask for the appropriate number of digits based on the type of product code for which a check digit is being calculated. For this, you'll need to useif
/else
conditional statements and boolean expressions (Section 3.1 of the Study Guide). Your program should also catch the following two error conditions by printing out the appropriate message, and exiting the program:
- The user enters a number that is not on the menu:
Check Digit Calculator
--------------------
1. EAN-8
2. UPC-A
3. EAN-13
4. EAN-14
5. SSCC
--------------------
Choose your product code type:18
Error: 18 is an invalid menu choice.
- The user enters a number of digits not expected by the program based on the type of product code selected:
Check Digit Calculator
--------------------
1. EAN-8
2. UPC-A
3. EAN-13
4. EAN-14
5. SSCC
--------------------
Choose your product code type:1
Enter first 7 digits of your product code:12
Error: You should have entered 7 digits, but you entered 2 digits.
Before you submit the program, check it with some products you have around. Determine the type of barcode from the number of digits; enter all but the last digit and make sure the check digit matches. (But see the last “hint” below.)
Hints
When you're done, create a ZIP file containing all of the files you created for this assignment and submit it.
To create some test cases, use:
https://www.axicon.com/checkdigitcalculator.html