Please use java. In this assignment, you will implement a simple class called CustomString. This class represents a more customizable version of a String, with additional attributes and methods. For example, the CustomString class has a “reverse” method which returns a new string version of the current string where the capitalization is reversed (i.e., lowercase to uppercase and uppercase to lowercase) for the alphabetical characters specified in a given argument. For CustomString “abc, XYZ; 123.”, calling reverse("bcdxyz@3210.") will return "aBC, xyz; 123.". The CustomString class also has a “remove” method which returns a new string version of the current string where the alphabetical characters specified in a given argument, are removed. For CustomString "my lucky numbers are 6, 8, and 19.", calling remove("ra6") will return "my lucky numbers e 6, 8, nd 19.". There are 5 methods that need to be implemented in the CustomString class: ● getString() - Returns the current string. ● setString(String string) - Sets the value of the current string. ● remove(String arg) - Returns a new string version of the current string where the alphabetical characters specified in the given arg, are removed. ● reverse(String arg) - Returns a new string version of the current string where the capitalization is reversed (i.e., lowercase to uppercase, and uppercase to lowercase) for the alphabetical characters specified in the given arg. ● filterLetters(char n, boolean more) - Returns a new string version of the current string where all the letters either >= or operators. For example: char myChar1 = ‘s’; char myChar2 = ‘t’; //compared is true boolean compared = myChar1 <>
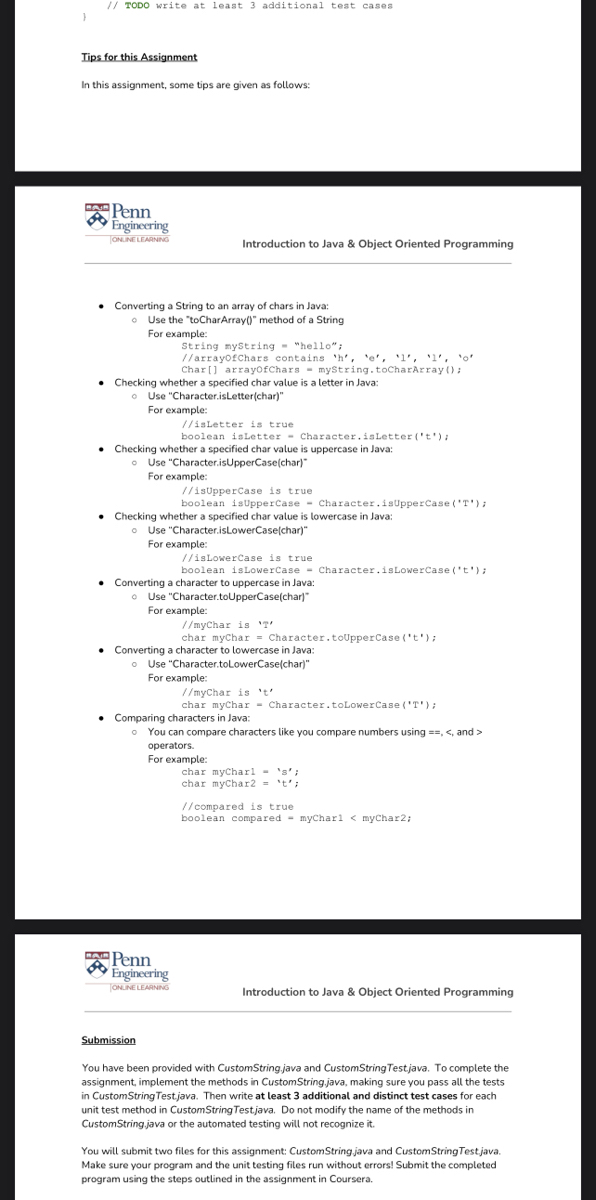
operators. For example: char mycharl - 's'; char mychar2 = 't'; // compared is true boolean compared - mycharl < mychar2;="" penn="" engineering="" online="" learning="" introduction="" to="" java="" &="" object="" oriented="" programming="" submission="" you="" have="" been="" provided="" with="" customstring="" java="" and="" customstring="" testjava.="" to="" complete="" the="" assignment,="" implement="" the="" methods="" in="" customstring.java,="" making="" sure="" you="" pass="" all="" the="" tests="" in="" customstringtestjava.="" then="" write="" at="" least="" 3="" additional="" and="" distinct="" test="" cases="" for="" each="" unit="" test="" method="" in="" customstring="" testjava.="" do="" not="" modify="" the="" name="" of="" the="" methods="" in="" customstring="" java="" or="" the="" automated="" testing="" will="" not="" recognize="" it.="" you="" will="" submit="" two="" files="" for="" this="" assignment:="" customstring="" java="" and="" customstringtest.java.="" make="" sure="" your="" program="" and="" the="" unit="" testing="" files="" run="" without="" errors!="" submit="" the="" completed="" program="" using="" the="" steps="" outlined="" in="" the="" assignment="" in="" coursera.="" "/="">
Extracted text: // TODO write at least 3 additional test cases Tips for this Assignment In this assignment, some tips are given as follows: APenn Engineering TONUINE LEARNING Introduction to Java & Object Oriented Programming • Converting a String to an array of chars in Java: • Use the "toCharArray()" method of a String For example: String mystring - "hello"; //arrayofChars contains 'h', 'e', 'l', 'l', 'o' Char[] arrayofChars - mystring.toCharArray (): Checking whether a specified char value is a letter in Java: • Use "Character.isLetter(char)" For example: //isLetter is true boolean isLetter - Character.isLetter ('t'): Checking whether a specified char value is uppercase in Java: • Use "Character.isUpperCase(char)" For example: //isUpperCase is true boolean isUpperCase - character.isUpperCase ('T'); Checking whether a specified char value is lowercase in Java: o Use "Character.isLowerCase(char)" For example: //isLowerCase is true boolean isLowerCase - Character.isLowerCase ('t'); • Converting a character to uppercase in Java: • Use "Character.toUpperCase(char)" For example: //myChar is 'T' char mychar = Character.toUpperCase ('t'); Converting a character to lowercase in Java: o Use "Character.toLowerCase(char)" For example: //myChar is 't' char mychar - Character.toLowerCase ('T'); Comparing characters in Java: o You can compare characters like you compare numbers using ==, <, and=""> operators. For example: char mycharl - 's'; char mychar2 = 't'; // compared is true boolean compared - mycharl < mychar2;="" penn="" engineering="" online="" learning="" introduction="" to="" java="" &="" object="" oriented="" programming="" submission="" you="" have="" been="" provided="" with="" customstring="" java="" and="" customstring="" testjava.="" to="" complete="" the="" assignment,="" implement="" the="" methods="" in="" customstring.java,="" making="" sure="" you="" pass="" all="" the="" tests="" in="" customstringtestjava.="" then="" write="" at="" least="" 3="" additional="" and="" distinct="" test="" cases="" for="" each="" unit="" test="" method="" in="" customstring="" testjava.="" do="" not="" modify="" the="" name="" of="" the="" methods="" in="" customstring="" java="" or="" the="" automated="" testing="" will="" not="" recognize="" it.="" you="" will="" submit="" two="" files="" for="" this="" assignment:="" customstring="" java="" and="" customstringtest.java.="" make="" sure="" your="" program="" and="" the="" unit="" testing="" files="" run="" without="" errors!="" submit="" the="" completed="" program="" using="" the="" steps="" outlined="" in="" the="" assignment="" in="">
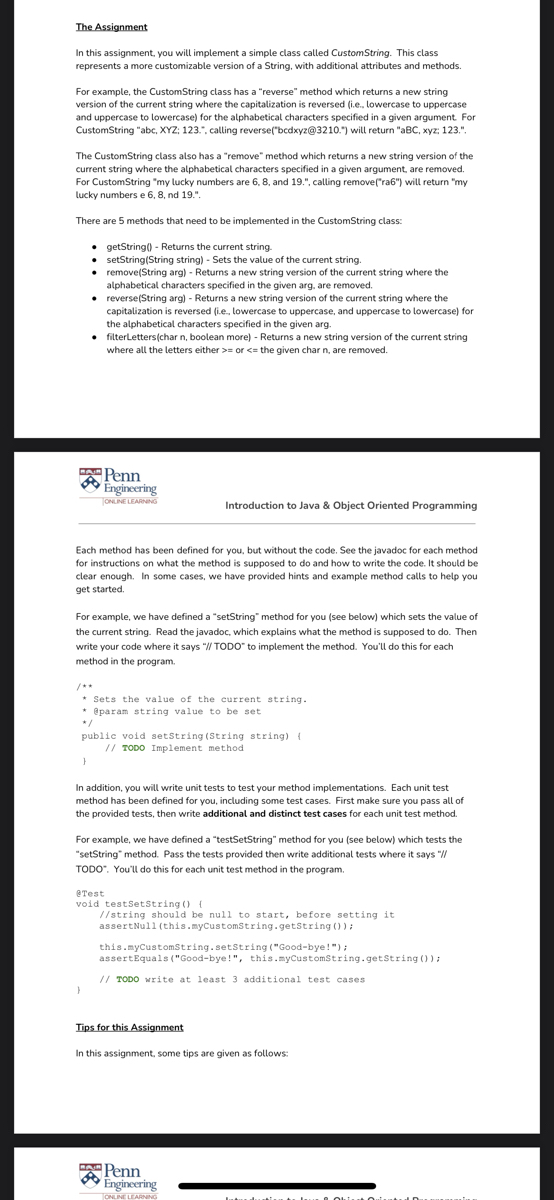
= or <= the="" given="" char="" n,="" are="" removed.="" .="" a="" penn="" engineering="" tonline="" learning="" introduction="" to="" java="" &="" object="" oriented="" programming="" each="" method="" has="" been="" defined="" for="" you,="" but="" without="" the="" code.="" see="" the="" javadoc="" for="" each="" method="" for="" instructions="" on="" what="" the="" method="" is="" supposed="" to="" do="" and="" how="" to="" write="" the="" code.="" it="" should="" be="" clear="" enough.="" in="" some="" cases,="" we="" have="" provided="" hints="" and="" example="" method="" calls="" to="" help="" you="" get="" started.="" for="" example,="" we="" have="" defined="" a="" "setstring"="" method="" for="" you="" (see="" below)="" which="" sets="" the="" value="" of="" the="" current="" string.="" read="" the="" javadoc,="" which="" explains="" what="" the="" method="" is="" supposed="" to="" do.="" then="" write="" your="" code="" where="" it="" says="" "ii="" todo"="" to="" implement="" the="" method.="" you'll="" do="" this="" for="" each="" method="" in="" the="" program.="" *="" sets="" the="" value="" of="" the="" current="" string.="" *="" @param="" string="" value="" to="" be="" set="" */="" public="" void="" setstring="" (string="" string)="" {="" todo="" implement="" method="" in="" addition,="" you="" will="" write="" unit="" tests="" to="" test="" your="" method="" implementations.="" each="" unit="" test="" method="" has="" been="" defined="" for="" you,="" including="" some="" test="" cases.="" first="" make="" sure="" you="" pass="" all="" of="" the="" provided="" tests,="" then="" write="" additional="" and="" distinct="" test="" cases="" for="" each="" unit="" test="" method.="" for="" example,="" we="" have="" defined="" a="" "testsetstring"="" method="" for="" you="" (see="" below)="" which="" tests="" the="" "setstring"="" method.="" pass="" the="" tests="" provided="" then="" write="" additional="" tests="" where="" it="" says="" "//="" todo",="" you'lu="" do="" this="" for="" each="" unit="" test="" method="" in="" the="" program.="" etest="" void="" testsetstring="" ()="" {="" string="" should="" be="" null="" to="" start,="" before="" setting="" it="" assertnull="" (this.mycustomstring.getstring="" 0):="" this.mycustomstring.setstring="" ("good-bye!"):="" assertequals="" ("good-bye!",="" this.mycustomstring.getstring="" ()):="" todo="" write="" at="" least="" 3="" additional="" test="" cases="" tips="" for="" this="" assignment="" in="" this="" assignment,="" some="" tips="" are="" given="" as="" follows:="" penn="" engineering="" tonline="" learning="" "/="">
Extracted text: The Assignment In this assignment, you will implement a simple class called CustomString. This class represents a more customizable version of a String, with additional attributes and methods. For example, the CustomString class has a "reverse" method which returns a new string version of the current string where the capitalization is reversed (i.e., lowercase to uppercase and uppercase to lowercase) for the alphabetical characters specified in a given argument. For CustomString "abe, XYZ; 123.", calling reverse("bcdxyz@3210.") will return "aBC, xyz; 123.". The CustomString class also has a "remove" method which returns a new string version of the current string where the alphabetical characters specified in a given argument, are removed. For CustomString "my lucky numbers are 6, 8, and 19.", calling remove("ra6") will return "my lucky numbers e 6, 8, nd 19.". There are 5 methods that need to be implemented in the CustomString class: • getString) - Returns the current string • setString(String string) - Sets the value of the current string. • remove(String arg) - Returns a new string version of the current string where the alphabetical characters specified in the given arg, are removed. • reverse(String arg) - Returns a new string version of the current string where the capitalization is reversed (i.e., lowercase to uppercase, and uppercase to lowercase) for the alphabetical characters specified in the given arg. filterLetters(char n, boolean more) - Returns a new string version of the current string where all the letters either >= or <= the given char n, are removed. . a penn engineering tonline learning introduction to java & object oriented programming each method has been defined for you, but without the code. see the javadoc for each method for instructions on what the method is supposed to do and how to write the code. it should be clear enough. in some cases, we have provided hints and example method calls to help you get started. for example, we have defined a "setstring" method for you (see below) which sets the value of the current string. read the javadoc, which explains what the method is supposed to do. then write your code where it says "ii todo" to implement the method. you'll do this for each method in the program. * sets the value of the current string. * @param string value to be set */ public void setstring (string string) { // todo implement method in addition, you will write unit tests to test your method implementations. each unit test method has been defined for you, including some test cases. first make sure you pass all of the provided tests, then write additional and distinct test cases for each unit test method. for example, we have defined a "testsetstring" method for you (see below) which tests the "setstring" method. pass the tests provided then write additional tests where it says "// todo", you'lu do this for each unit test method in the program. etest void testsetstring () { //string should be null to start, before setting it assertnull (this.mycustomstring.getstring 0): this.mycustomstring.setstring ("good-bye!"): assertequals ("good-bye!", this.mycustomstring.getstring ()): // todo write at least 3 additional test cases tips for this assignment in this assignment, some tips are given as follows: penn engineering tonline learning the="" given="" char="" n,="" are="" removed.="" .="" a="" penn="" engineering="" tonline="" learning="" introduction="" to="" java="" &="" object="" oriented="" programming="" each="" method="" has="" been="" defined="" for="" you,="" but="" without="" the="" code.="" see="" the="" javadoc="" for="" each="" method="" for="" instructions="" on="" what="" the="" method="" is="" supposed="" to="" do="" and="" how="" to="" write="" the="" code.="" it="" should="" be="" clear="" enough.="" in="" some="" cases,="" we="" have="" provided="" hints="" and="" example="" method="" calls="" to="" help="" you="" get="" started.="" for="" example,="" we="" have="" defined="" a="" "setstring"="" method="" for="" you="" (see="" below)="" which="" sets="" the="" value="" of="" the="" current="" string.="" read="" the="" javadoc,="" which="" explains="" what="" the="" method="" is="" supposed="" to="" do.="" then="" write="" your="" code="" where="" it="" says="" "ii="" todo"="" to="" implement="" the="" method.="" you'll="" do="" this="" for="" each="" method="" in="" the="" program.="" *="" sets="" the="" value="" of="" the="" current="" string.="" *="" @param="" string="" value="" to="" be="" set="" */="" public="" void="" setstring="" (string="" string)="" {="" todo="" implement="" method="" in="" addition,="" you="" will="" write="" unit="" tests="" to="" test="" your="" method="" implementations.="" each="" unit="" test="" method="" has="" been="" defined="" for="" you,="" including="" some="" test="" cases.="" first="" make="" sure="" you="" pass="" all="" of="" the="" provided="" tests,="" then="" write="" additional="" and="" distinct="" test="" cases="" for="" each="" unit="" test="" method.="" for="" example,="" we="" have="" defined="" a="" "testsetstring"="" method="" for="" you="" (see="" below)="" which="" tests="" the="" "setstring"="" method.="" pass="" the="" tests="" provided="" then="" write="" additional="" tests="" where="" it="" says="" "//="" todo",="" you'lu="" do="" this="" for="" each="" unit="" test="" method="" in="" the="" program.="" etest="" void="" testsetstring="" ()="" {="" string="" should="" be="" null="" to="" start,="" before="" setting="" it="" assertnull="" (this.mycustomstring.getstring="" 0):="" this.mycustomstring.setstring="" ("good-bye!"):="" assertequals="" ("good-bye!",="" this.mycustomstring.getstring="" ()):="" todo="" write="" at="" least="" 3="" additional="" test="" cases="" tips="" for="" this="" assignment="" in="" this="" assignment,="" some="" tips="" are="" given="" as="" follows:="" penn="" engineering="" tonline="">= the given char n, are removed. . a penn engineering tonline learning introduction to java & object oriented programming each method has been defined for you, but without the code. see the javadoc for each method for instructions on what the method is supposed to do and how to write the code. it should be clear enough. in some cases, we have provided hints and example method calls to help you get started. for example, we have defined a "setstring" method for you (see below) which sets the value of the current string. read the javadoc, which explains what the method is supposed to do. then write your code where it says "ii todo" to implement the method. you'll do this for each method in the program. * sets the value of the current string. * @param string value to be set */ public void setstring (string string) { // todo implement method in addition, you will write unit tests to test your method implementations. each unit test method has been defined for you, including some test cases. first make sure you pass all of the provided tests, then write additional and distinct test cases for each unit test method. for example, we have defined a "testsetstring" method for you (see below) which tests the "setstring" method. pass the tests provided then write additional tests where it says "// todo", you'lu do this for each unit test method in the program. etest void testsetstring () { //string should be null to start, before setting it assertnull (this.mycustomstring.getstring 0): this.mycustomstring.setstring ("good-bye!"): assertequals ("good-bye!", this.mycustomstring.getstring ()): // todo write at least 3 additional test cases tips for this assignment in this assignment, some tips are given as follows: penn engineering tonline learning>=>,>