The correct version of the code we are testing is in a module namedpiggy. This module simulates a ceramic piggy bank. It works like this: initially it starts off containing no coins and having no value. As you add coins, thenumber of coins in the bank increases, and also the totalvalue of the coins increases. Of course, it is limited in size, so there is a maximum number of coins it can hold (we are assuming that all coins take up the same space regardless of their value). Once it reaches that maximum, you can't add any more. Finally, it can besmashed to get the money out. But after it is smashed, it contains no coins and you can't add any more, until you re-initialize the module by calling start again.
The module has the following functions:
start(max) - initializes the piggy bank so it can hold the given maximum number of coins. Initially it contains no coins and has zero value and is not smashed.
add_coin(coin_value) - adds a coin with the given value to the piggy bank, unless it is full or smashed. The coin value can be anything
get_value() - returns the total value of all the coins in the piggy bank
get_num_coins() - returns the total number of coins in the piggy bank
smash() - smashes the piggy bank, after which it has no coins and no coins can be added
For example, we should be able to write code like this:
import piggy as p # initialize the piggy bank to have a capacity of 5 coins p.start(5) print(p.get_num_coins()) # expected 0 print(p.get_value()) # expected 0 # add a couple of coins p.add_coin(10) p.add_coin(25) # check what we've got print(p.get_num_coins()) # expected 2 print(p.get_value()) # expected 35
Bad piggies
There are also six implementations of the piggy bank specification that have errors in the code, namedpiggy1 throughpiggy6. Your task is to write a set of test cases, similar to the ones above, so that the originalpiggy modulepasses all your tests, but each ofpiggy1 throughpiggy6fails at least one of your test cases.
To simplify the whole process of testing and checking, we are going to do two things
- Notice the form of the import statement above. Instead of
import piggy
we write
import piggy as p
Then, we can refer to thepiggy module simply asp throughout our code. The advantage of this is that we can test a different module just by changing the import.
- Use a helper function to check whether we're getting correct values. Instead of simply printing the output as above, and then having to carefully read it to see if it's correct, we'll just pass the value we expect into the helper function to be checked, along with a description of what we're testing.
Take a look at the template in the code editor below, and try running it (in "Develop" mode). Then, modify the import statement:
import piggy1 as p
and run it again. Notice it is obvious that piggy1 has a bug, since the actual value returned by getValue doesn't match the expected. value 35.
Value after add_coin(25): expected 35, found 25
Please solve in python :)
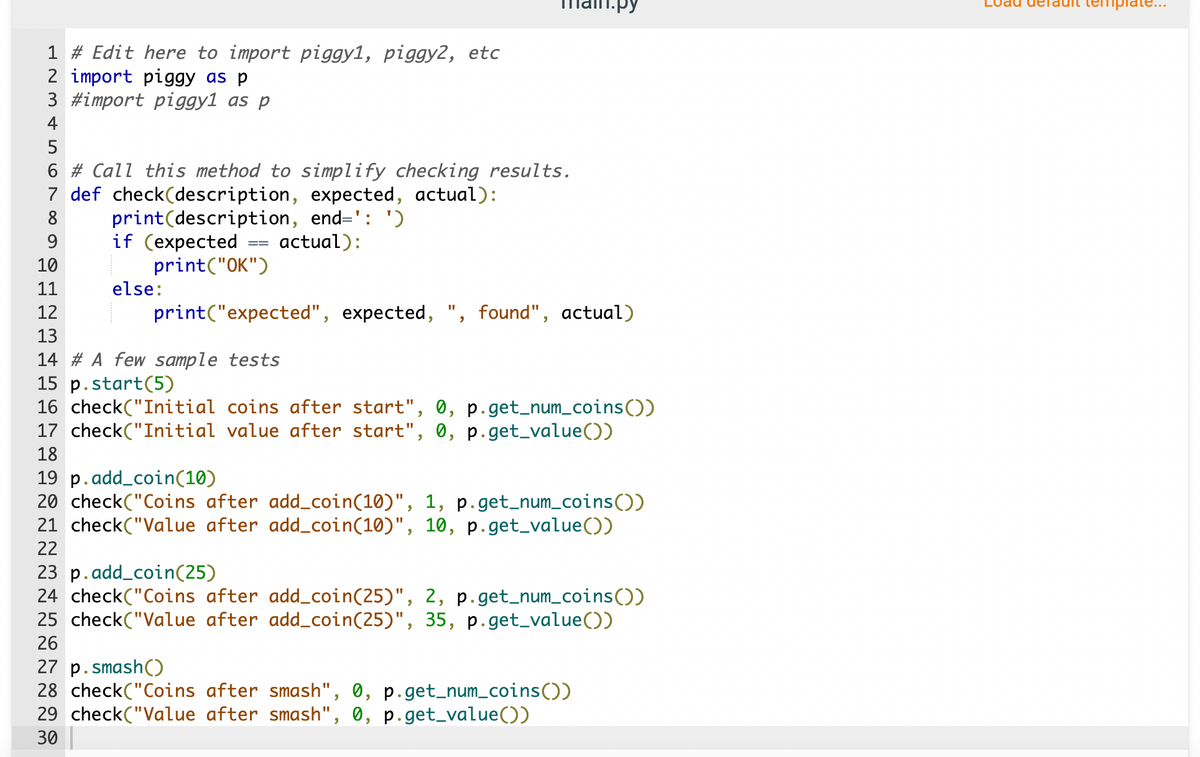
Extracted text: 11.py iplate... 1 # Edit here to import piggy1, piggy2, etc 2 import piggy as p 3 #import piggy1 as p 4 5 6 # Call this method to simplify checking results. 7 def check(description, expected, actual): print(description, end=': ') if (expected print("OK") else: 8 9. actual): 10 11 12 print("expected", expected, ", found", actual) 13 14 # A few sample tests 15 p.start(5) 16 check("Initial coins after start", 0, p.get_num_coins()) 17 check("Initial value after start", 0, p.get_value()) 18 19 p.add_coin(10) 20 check("Coins after add_coin(10)", 1, p.get_num_coins()) 21 check("Value after add_coin(10)", 10, p.get_value()) 22 23 p.add_coin(25) 24 check("Coins after add_coin(25)", 2, p.get_num_coins(O) 25 check("Value after add_coin(25)", 35, p.get_value()) 26 27 p.smash() 28 check("Coins after smash", 0, 29 check("Value after smash", 0, p.get_value()) %3D p.get_num_coins()) 30
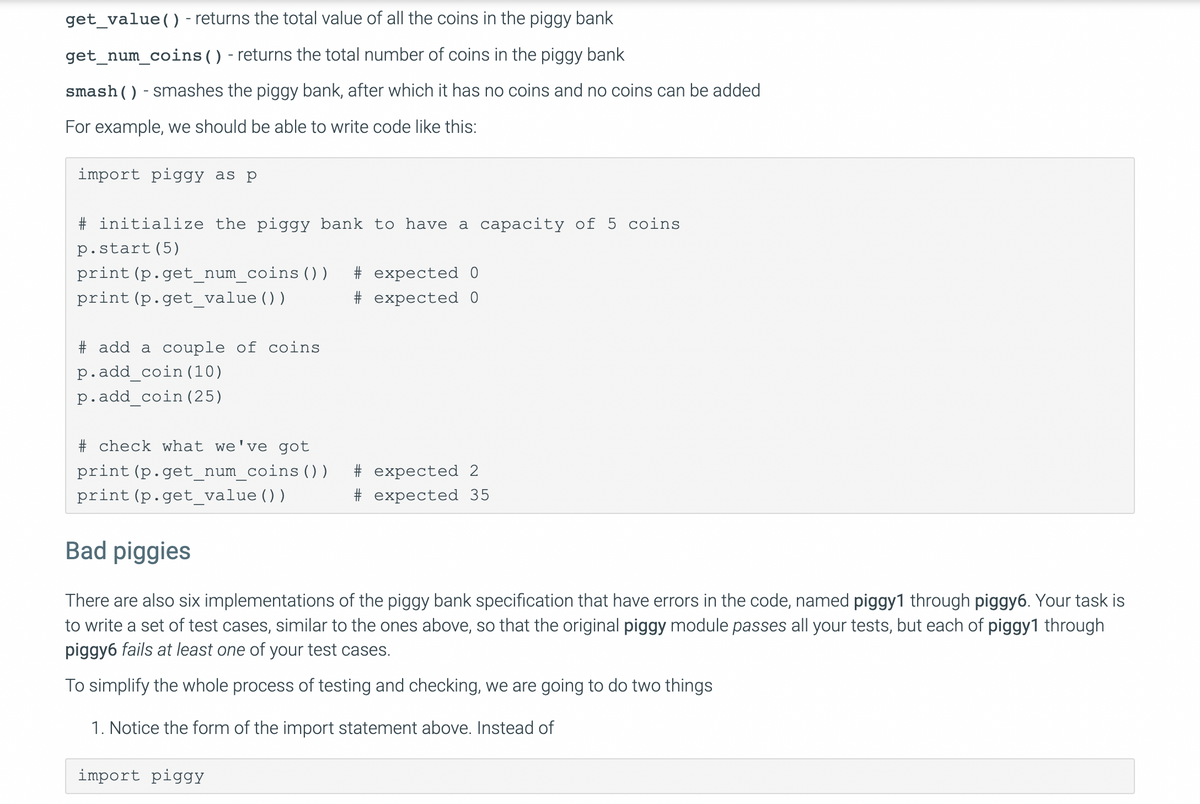
Extracted text: get_value() - returns the total value of all the coins in the piggy bank get_num_coins () - returns the total number of coins in the piggy bank smash()- smashes the piggy bank, after which it has no coins and no coins can be added For example, we should be able to write code like this: import piggy as p # initialize the piggy bank to have a capacity of 5 coins p.start(5) print (p.get_num_coins () ) # expected 0 print (p.get_value ()) # expected 0 # add a couple of coins p.add_coin(10) p.add_coin (25) # check what we've got print (p.get_num_coins () ) # expected 2 print (p.get_value ()) # expected 35 Bad piggies There are also six implementations of the piggy bank specification that have errors in the code, named piggy1 through piggy6. Your task is to write a set of test cases, similar to the ones above, so that the original piggy module passes all your tests, but each of piggy1 through piggy6 fails at least one of your test cases. To simplify the whole process of testing and checking, we are going to do two things 1. Notice the form of the import statement above. Instead of import piggY