neverwrote-master@df4dcf72582 (2).zip
neverwrote-master@df4dcf72582/.gitignore
build/
node_modules/
dist/
*.swp
neverwrote-master@df4dcf72582/api/.eslintrc
{
"extends": "eslint-config-airbnb",
"env" : {
"browser": true,
"node": true,
"jasmine": true
},
"plugins": [
"react"
],
"rules": {
"react/no-multi-comp": 0,
"react/prop-types": 0,
"react/sort-comp": 0,
"react/no-did-mount-set-state": 0,
"react/prefer-es6-class": 0,
"comma-dangle": 0,
"indent": [2, 2, {"SwitchCase": 1}],
"no-console": 0,
"no-alert": 0,
"new-cap": 0,
"func-names": 0,
"keyword-spacing": 0,
"space-before-function-paren": [2, "never"],
"object-shorthand": [2, "properties"],
"no-param-reassign": 0
}
}
neverwrote-master@df4dcf72582/api/.sequelizerc
const path = require('path');
const dbConfig = require('./src/config/database');
// Build the connection URL string
const connectionUrl = 'mysql://' +
dbConfig.user + ':' + dbConfig.password + '@' +
dbConfig.host + ':' + dbConfig.port + '/' + dbConfig.database;
// Export settings for the Sequelize command line tool
module.exports = {
'url': connectionUrl,
'migrations-path': path.resolve('src', 'migrations'),
'models-path': path.resolve('src', 'models'),
'seeders-path': path.resolve('src', 'seeders')
};
neverwrote-master@df4dcf72582/api/Dockerfile
# ## Dockerfile ##
#
# This file describes the complete environment in which our server will run,
# including the operating system and all software dependencies. It is used
# by Docker Compose to build a Docker image for our application.
# Base this image on an official Node.js long term support image.
FROM node:8.1.2-alpine
# Install some additional packages that we need.
RUN apk add --no-cache tini curl bash sudo
# Use Tini as the init process. Tini will take care of important system stuff
# for us, like forwarding signals and reaping zombie processes.
ENTRYPOINT ["/sbin/tini", "--"]
# Create a working directory for our application.
RUN mkdir -p /app
WORKDIR /app
# Install other system dependencies (for building sqlite3).
RUN apk add --no-cache python g++ make git
# Install the project's NPM dependencies.
COPY package.json /app/
RUN npm --silent install
# Install sequelize-mocking manually - it's ugly, but it works.
RUN npm --silent install git+https://github.com/anibali/node-sqlite3.git#alpine
RUN npm --silent install [email protected]
# Move NPM dependencies out of the /app folder.
RUN mkdir /deps && mv node_modules /deps/node_modules
# Set environment variables to point to the installed NPM modules.
ENV NODE_PATH=/deps/node_modules \
PATH=/deps/node_modules/.bin:$PATH
# Copy our application files into the image.
COPY . /app
# Switch to a non-privileged user for running commands inside the container.
RUN chown -R node:node /app /deps \
&& echo "node ALL=(ALL) NOPASSWD:ALL" > /etc/sudoers.d/90-node
USER node
# Start the server on exposed port 3000.
EXPOSE 3000
CMD [ "npm", "start" ]
neverwrote-master@df4dcf72582/api/node_modules/.bin/mime
#!/bin/sh
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
case `uname` in
*CYGWIN*|*MINGW*|*MSYS*) basedir=`cygpath -w "$basedir"`;;
esac
if [ -x "$basedir/node" ]; then
"$basedir/node" "$basedir/../mime/cli.js" "$@"
ret=$?
else
node "$basedir/../mime/cli.js" "$@"
ret=$?
fi
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/.bin/mime.cmd
@ECHO off
SETLOCAL
CALL :find_dp0
IF EXIST "%dp0%\node.exe" (
SET "_prog=%dp0%\node.exe"
) ELSE (
SET "_prog=node"
SET PATHEXT=%PATHEXT:;.JS;=;%
)
"%_prog%" "%dp0%\..\mime\cli.js" %*
ENDLOCAL
EXIT /b %errorlevel%
:find_dp0
SET dp0=%~dp0
EXIT /b
neverwrote-master@df4dcf72582/api/node_modules/.bin/mime.ps1
#!/usr/bin/env pwsh
$basedir=Split-Path $MyInvocation.MyCommand.Definition -Parent
$exe=""
if ($PSVersionTable.PSVersion -lt "6.0" -or $IsWindows) {
# Fix case when both the Windows and Linux builds of Node
# are installed in the same directory
$exe=".exe"
}
$ret=0
if (Test-Path "$basedir/node$exe") {
& "$basedir/node$exe" "$basedir/../mime/cli.js" $args
$ret=$LASTEXITCODE
} else {
& "node$exe" "$basedir/../mime/cli.js" $args
$ret=$LASTEXITCODE
}
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/.bin/semver
#!/bin/sh
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
case `uname` in
*CYGWIN*|*MINGW*|*MSYS*) basedir=`cygpath -w "$basedir"`;;
esac
if [ -x "$basedir/node" ]; then
"$basedir/node" "$basedir/../semver/bin/semver" "$@"
ret=$?
else
node "$basedir/../semver/bin/semver" "$@"
ret=$?
fi
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/.bin/semver.cmd
@ECHO off
SETLOCAL
CALL :find_dp0
IF EXIST "%dp0%\node.exe" (
SET "_prog=%dp0%\node.exe"
) ELSE (
SET "_prog=node"
SET PATHEXT=%PATHEXT:;.JS;=;%
)
"%_prog%" "%dp0%\..\semver\bin\semver" %*
ENDLOCAL
EXIT /b %errorlevel%
:find_dp0
SET dp0=%~dp0
EXIT /b
neverwrote-master@df4dcf72582/api/node_modules/.bin/semver.ps1
#!/usr/bin/env pwsh
$basedir=Split-Path $MyInvocation.MyCommand.Definition -Parent
$exe=""
if ($PSVersionTable.PSVersion -lt "6.0" -or $IsWindows) {
# Fix case when both the Windows and Linux builds of Node
# are installed in the same directory
$exe=".exe"
}
$ret=0
if (Test-Path "$basedir/node$exe") {
& "$basedir/node$exe" "$basedir/../semver/bin/semver" $args
$ret=$LASTEXITCODE
} else {
& "node$exe" "$basedir/../semver/bin/semver" $args
$ret=$LASTEXITCODE
}
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/.bin/uuid
#!/bin/sh
basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
case `uname` in
*CYGWIN*|*MINGW*|*MSYS*) basedir=`cygpath -w "$basedir"`;;
esac
if [ -x "$basedir/node" ]; then
"$basedir/node" "$basedir/../uuid/bin/uuid" "$@"
ret=$?
else
node "$basedir/../uuid/bin/uuid" "$@"
ret=$?
fi
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/.bin/uuid.cmd
@ECHO off
SETLOCAL
CALL :find_dp0
IF EXIST "%dp0%\node.exe" (
SET "_prog=%dp0%\node.exe"
) ELSE (
SET "_prog=node"
SET PATHEXT=%PATHEXT:;.JS;=;%
)
"%_prog%" "%dp0%\..\uuid\bin\uuid" %*
ENDLOCAL
EXIT /b %errorlevel%
:find_dp0
SET dp0=%~dp0
EXIT /b
neverwrote-master@df4dcf72582/api/node_modules/.bin/uuid.ps1
#!/usr/bin/env pwsh
$basedir=Split-Path $MyInvocation.MyCommand.Definition -Parent
$exe=""
if ($PSVersionTable.PSVersion -lt "6.0" -or $IsWindows) {
# Fix case when both the Windows and Linux builds of Node
# are installed in the same directory
$exe=".exe"
}
$ret=0
if (Test-Path "$basedir/node$exe") {
& "$basedir/node$exe" "$basedir/../uuid/bin/uuid" $args
$ret=$LASTEXITCODE
} else {
& "node$exe" "$basedir/../uuid/bin/uuid" $args
$ret=$LASTEXITCODE
}
exit $ret
neverwrote-master@df4dcf72582/api/node_modules/@types/geojson/index.d.ts
// Type definitions for GeoJSON Format Specification Revision 1.0
// Project: http://geojson.org/
// Definitions by: Jacob Bruun
// Arne Schubert
// Definitions: https://github.com/DefinitelyTyped/DefinitelyTyped
// TypeScript Version: 2.3
export as namespace GeoJSON;
/***
* http://geojson.org/geojson-spec.html#geojson-objects
*/
export interface GeoJsonObject {
type: string;
bbox?: number[];
crs?: CoordinateReferenceSystem;
}
/***
* http://geojson.org/geojson-spec.html#positions
*/
export type Position = number[];
/***
* http://geojson.org/geojson-spec.html#geometry-objects
*/
export interface DirectGeometryObject extends GeoJsonObject {
coordinates: Position[][][] | Position[][] | Position[] | Position;
}
/**
* GeometryObject supports geometry collection as well
*/
export type GeometryObject = DirectGeometryObject | GeometryCollection;
/***
* http://geojson.org/geojson-spec.html#point
*/
export interface Point extends DirectGeometryObject {
type: "Point";
coordinates: Position;
}
/***
* http://geojson.org/geojson-spec.html#multipoint
*/
export interface MultiPoint extends DirectGeometryObject {
type: "MultiPoint";
coordinates: Position[];
}
/***
* http://geojson.org/geojson-spec.html#linestring
*/
export interface LineString extends DirectGeometryObject {
type: "LineString";
coordinates: Position[];
}
/***
* http://geojson.org/geojson-spec.html#multilinestring
*/
export interface MultiLineString extends DirectGeometryObject {
type: "MultiLineString";
coordinates: Position[][];
}
/***
* http://geojson.org/geojson-spec.html#polygon
*/
export interface Polygon extends DirectGeometryObject {
type: "Polygon";
coordinates: Position[][];
}
/***
* http://geojson.org/geojson-spec.html#multipolygon
*/
export interface MultiPolygon extends DirectGeometryObject {
type: "MultiPolygon";
coordinates: Position[][][];
}
/***
* http://geojson.org/geojson-spec.html#geometry-collection
*/
export interface GeometryCollection extends GeoJsonObject {
type: "GeometryCollection";
geometries: GeometryObject[];
}
/***
* https://tools.ietf.org/html/rfc7946#section-3.2
*/
export interface Feature extends GeoJsonObject {
type: "Feature";
geometry: G;
properties: P;
id?: string | number;
}
/***
* http://geojson.org/geojson-spec.html#feature-collection-objects
*/
export interface FeatureCollection extends GeoJsonObject {
type: "FeatureCollection";
features: Array>;
}
/***
* http://geojson.org/geojson-spec.html#coordinate-reference-system-objects
*/
export interface CoordinateReferenceSystem {
type: string;
properties: any;
}
export interface NamedCoordinateReferenceSystem extends CoordinateReferenceSystem {
properties: { name: string };
}
export interface LinkedCoordinateReferenceSystem extends CoordinateReferenceSystem {
properties: { href: string; type: string };
}
neverwrote-master@df4dcf72582/api/node_modules/@types/geojson/LICENSE
MIT License
Copyright (c) Microsoft Corporation. All rights reserved.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE
neverwrote-master@df4dcf72582/api/node_modules/@types/geojson/package.json
{
"_from": "@types/geojson@^1.0.0",
"_id": "@types/[email protected]",
"_inBundle": false,
"_integrity": "sha512-Xqg/lIZMrUd0VRmSRbCAewtwGZiAk3mEUDvV4op1tGl+LvyPcb/MIOSxTl9z+9+J+R4/vpjiCAT4xeKzH9ji1w==",
"_location": "/@types/geojson",
"_phantomChildren": {},
"_requested": {
"type": "range",
"registry": true,
"raw": "@types/geojson@^1.0.0",
"name": "@types/geojson",
"escapedName": "@types%2fgeojson",
"scope": "@types",
"rawSpec": "^1.0.0",
"saveSpec": null,
"fetchSpec": "^1.0.0"
},
"_requiredBy": [
"/terraformer",
"/terraformer-wkt-parser"
],
"_resolved": "https://registry.npmjs.org/@types/geojson/-/geojson-1.0.6.tgz",
"_shasum": "3e02972728c69248c2af08d60a48cbb8680fffdf",
"_spec": "@types/geojson@^1.0.0",
"_where": "D:\\Greynodes\\neverwrote-master@df4dcf72582\\api\\node_modules\\terraformer-wkt-parser",
"bugs": {
"url": "https://github.com/DefinitelyTyped/DefinitelyTyped/issues"
},
"bundleDependencies": false,
"contributors": [
{
"name": "Jacob Bruun",
"url": "https://github.com/cobster"
},
{
"name": "Arne Schubert",
"url": "https://github.com/atd-schubert"
}
],
"dependencies": {},
"deprecated": false,
"description": "TypeScript definitions for GeoJSON Format Specification Revision",
"homepage": "https://github.com/DefinitelyTyped/DefinitelyTyped#readme",
"license": "MIT",
"main": "",
"name": "@types/geojson",
"repository": {
"type": "git",
"url": "git+https://github.com/DefinitelyTyped/DefinitelyTyped.git"
},
"scripts": {},
"typeScriptVersion": "2.3",
"typesPublisherContentHash": "2a374692a48615d90fde45b274e247a1ec98647d93fe7c7ee355386108689bcd",
"version": "1.0.6"
}
neverwrote-master@df4dcf72582/api/node_modules/@types/geojson/README.md
# Installation
> `npm install --save @types/geojson`
# Summary
This package contains type definitions for GeoJSON Format Specification Revision (http://geojson.org/).
# Details
Files were exported from https://www.github.com/DefinitelyTyped/DefinitelyTyped/tree/master/types/geojson
Additional Details
* Last updated: Wed, 01 Nov 2017 15:55:40 GMT
* Dependencies: none
* Global values: GeoJSON
# Credits
These definitions were written by Jacob Bruun , Arne Schubert .
neverwrote-master@df4dcf72582/api/node_modules/accepts/HISTORY.md
1.3.7 / 2019-04-29
==================
* deps: [email protected]
- Fix sorting charset, encoding, and language with extra parameters
1.3.6 / 2019-04-28
==================
* deps: mime-types@~2.1.24
- deps: mime-db@~1.40.0
1.3.5 / 2018-02-28
==================
* deps: mime-types@~2.1.18
- deps: mime-db@~1.33.0
1.3.4 / 2017-08-22
==================
* deps: mime-types@~2.1.16
- deps: mime-db@~1.29.0
1.3.3 / 2016-05-02
==================
* deps: mime-types@~2.1.11
- deps: mime-db@~1.23.0
* deps: [email protected]
- perf: improve `Accept` parsing speed
- perf: improve `Accept-Charset` parsing speed
- perf: improve `Accept-Encoding` parsing speed
- perf: improve `Accept-Language` parsing speed
1.3.2 / 2016-03-08
==================
* deps: mime-types@~2.1.10
- Fix extension of `application/dash+xml`
- Update primary extension for `audio/mp4`
- deps: mime-db@~1.22.0
1.3.1 / 2016-01-19
==================
* deps: mime-types@~2.1.9
- deps: mime-db@~1.21.0
1.3.0 / 2015-09-29
==================
* deps: mime-types@~2.1.7
- deps: mime-db@~1.19.0
* deps: [email protected]
- Fix including type extensions in parameters in `Accept` parsing
- Fix parsing `Accept` parameters with quoted equals
- Fix parsing `Accept` parameters with quoted semicolons
- Lazy-load modules from main entry point
- perf: delay type concatenation until needed
- perf: enable strict mode
- perf: hoist regular expressions
- perf: remove closures getting spec properties
- perf: remove a closure from media type parsing
- perf: remove property delete from media type parsing
1.2.13 / 2015-09-06
===================
* deps: mime-types@~2.1.6
- deps: mime-db@~1.18.0
1.2.12 / 2015-07-30
===================
* deps: mime-types@~2.1.4
- deps: mime-db@~1.16.0
1.2.11 / 2015-07-16
===================
* deps: mime-types@~2.1.3
- deps: mime-db@~1.15.0
1.2.10 / 2015-07-01
===================
* deps: mime-types@~2.1.2
- deps: mime-db@~1.14.0
1.2.9 / 2015-06-08
==================
* deps: mime-types@~2.1.1
- perf: fix deopt during mapping
1.2.8 / 2015-06-07
==================
* deps: mime-types@~2.1.0
- deps: mime-db@~1.13.0
* perf: avoid argument reassignment & argument slice
* perf: avoid negotiator recursive construction
* perf: enable strict mode
* perf: remove unnecessary bitwise operator
1.2.7 / 2015-05-10
==================
* deps: [email protected]
- Fix media type parameter matching to be case-insensitive
1.2.6 / 2015-05-07
==================
* deps: mime-types@~2.0.11
- deps: mime-db@~1.9.1
* deps: [email protected]
- Fix comparing media types with quoted values
- Fix splitting media types with quoted commas
1.2.5 / 2015-03-13
==================
* deps: mime-types@~2.0.10
- deps: mime-db@~1.8.0
1.2.4 / 2015-02-14
==================
* Support Node.js 0.6
* deps: mime-types@~2.0.9
- deps: mime-db@~1.7.0
* deps: [email protected]
- Fix preference sorting to be stable for long acceptable lists
1.2.3 / 2015-01-31
==================
* deps: mime-types@~2.0.8
- deps: mime-db@~1.6.0
1.2.2 / 2014-12-30
==================
* deps: mime-types@~2.0.7
- deps: mime-db@~1.5.0
1.2.1 / 2014-12-30
==================
* deps: mime-types@~2.0.5
- deps: mime-db@~1.3.1
1.2.0 / 2014-12-19
==================
* deps: [email protected]
- Fix list return order when large accepted list
- Fix missing identity encoding when q=0 exists
- Remove dynamic building of Negotiator class
1.1.4 / 2014-12-10
==================
* deps: mime-types@~2.0.4
- deps: mime-db@~1.3.0
1.1.3 / 2014-11-09
==================
* deps: mime-types@~2.0.3
- deps: mime-db@~1.2.0
1.1.2 / 2014-10-14
==================
* deps: [email protected]
- Fix error when media type has invalid parameter
1.1.1 / 2014-09-28
==================
* deps: mime-types@~2.0.2
- deps: mime-db@~1.1.0
* deps: [email protected]
- Fix all negotiations to be case-insensitive
- Stable sort preferences of same quality according to client order
1.1.0 / 2014-09-02
==================
* update `mime-types`
1.0.7 / 2014-07-04
==================
* Fix wrong type returned from `type` when match after unknown extension
1.0.6 / 2014-06-24
==================
* deps: [email protected]
1.0.5 / 2014-06-20
==================
* fix crash when unknown extension given
1.0.4 / 2014-06-19
==================
* use `mime-types`
1.0.3 / 2014-06-11
==================
* deps: [email protected]
- Order by specificity when quality is the same
1.0.2 / 2014-05-29
==================
* Fix interpretation when header not in request
* deps: pin [email protected]
1.0.1 / 2014-01-18
==================
* Identity encoding isn't always acceptable
* deps: negotiator@~0.4.0
1.0.0 / 2013-12-27
==================
* Genesis
neverwrote-master@df4dcf72582/api/node_modules/accepts/index.js
/*!
* accepts
* Copyright(c) 2014 Jonathan Ong
* Copyright(c) 2015 Douglas Christopher Wilson
* MIT Licensed
*/
'use strict'
/**
* Module dependencies.
* @private
*/
var Negotiator = require('negotiator')
var mime = require('mime-types')
/**
* Module exports.
* @public
*/
module.exports = Accepts
/**
* Create a new Accepts object for the given req.
*
* @param {object} req
* @public
*/
function Accepts (req) {
if (!(this instanceof Accepts)) {
return new Accepts(req)
}
this.headers = req.headers
this.negotiator = new Negotiator(req)
}
/**
* Check if the given `type(s)` is acceptable, returning
* the best match when true, otherwise `undefined`, in which
* case you should respond with 406 "Not Acceptable".
*
* The `type` value may be a single mime type string
* such as "application/json", the extension name
* such as "json" or an array `["json", "html", "text/plain"]`. When a list
* or array is given the _best_ match, if any is returned.
*
* Examples:
*
* // Accept: text/html
* this.types('html');
* // => "html"
*
* // Accept: text/*, application/json
* this.types('html');
* // => "html"
* this.types('text/html');
* // => "text/html"
* this.types('json', 'text');
* // => "json"
* this.types('application/json');
* // => "application/json"
*
* // Accept: text/*, application/json
* this.types('image/png');
* this.types('png');
* // => undefined
*
* // Accept: text/*;q=.5, application/json
* this.types(['html', 'json']);
* this.types('html', 'json');
* // => "json"
*
* @param {String|Array} types...
* @return {String|Array|Boolean}
* @public
*/
Accepts.prototype.type =
Accepts.prototype.types = function (types_) {
var types = types_
// support flattened arguments
if (types && !Array.isArray(types)) {
types = new Array(arguments.length)
for (var i = 0; i < types.length; i++) {
types[i] = arguments[i]
}
}
// no types, return all requested types
if (!types || types.length === 0) {
return this.negotiator.mediaTypes()
}
// no accept header, return first given type
if (!this.headers.accept) {
return types[0]
}
var mimes = types.map(extToMime)
var accepts = this.negotiator.mediaTypes(mimes.filter(validMime))
var first = accepts[0]
return first
? types[mimes.indexOf(first)]
: false
}
/**
* Return accepted encodings or best fit based on `encodings`.
*
* Given `Accept-Encoding: gzip, deflate`
* an array sorted by quality is returned:
*
* ['gzip', 'deflate']
*
* @param {String|Array} encodings...
* @return {String|Array}
* @public
*/
Accepts.prototype.encoding =
Accepts.prototype.encodings = function (encodings_) {
var encodings = encodings_
// support flattened arguments
if (encodings && !Array.isArray(encodings)) {
encodings = new Array(arguments.length)
for (var i = 0; i < encodings.length; i++) {
encodings[i] = arguments[i]
}
}
// no encodings, return all requested encodings
if (!encodings || encodings.length === 0) {
return this.negotiator.encodings()
}
return this.negotiator.encodings(encodings)[0] || false
}
/**
* Return accepted charsets or best fit based on `charsets`.
*
* Given `Accept-Charset: utf-8, iso-8859-1;q=0.2, utf-7;q=0.5`
* an array sorted by quality is returned:
*
* ['utf-8', 'utf-7', 'iso-8859-1']
*
* @param {String|Array} charsets...
* @return {String|Array}
* @public
*/
Accepts.prototype.charset =
Accepts.prototype.charsets = function (charsets_) {
var charsets = charsets_
// support flattened arguments
if (charsets && !Array.isArray(charsets)) {
charsets = new Array(arguments.length)
for (var i = 0; i < charsets.length; i++) {
charsets[i] = arguments[i]
}
}
// no charsets, return all requested charsets
if (!charsets || charsets.length === 0) {
return this.negotiator.charsets()
}
return this.negotiator.charsets(charsets)[0] || false
}
/**
* Return accepted languages or best fit based on `langs`.
*
* Given `Accept-Language: en;q=0.8, es, pt`
* an array sorted by quality is returned:
*
* ['es', 'pt', 'en']
*
* @param {String|Array} langs...
* @return {Array|String}
* @public
*/
Accepts.prototype.lang =
Accepts.prototype.langs =
Accepts.prototype.language =
Accepts.prototype.languages = function (languages_) {
var languages = languages_
// support flattened arguments
if (languages && !Array.isArray(languages)) {
languages = new Array(arguments.length)
for (var i = 0; i < languages.length; i++) {
languages[i] = arguments[i]
}
}
// no languages, return all requested languages
if (!languages || languages.length === 0) {
return this.negotiator.languages()
}
return this.negotiator.languages(languages)[0] || false
}
/**
* Convert extnames to mime.
*
* @param {String} type
* @return {String}
* @private
*/
function extToMime (type) {
return type.indexOf('/') === -1
? mime.lookup(type)
: type
}
/**
* Check if mime is valid.
*
* @param {String} type
* @return {String}
* @private
*/
function validMime (type) {
return typeof type === 'string'
}
neverwrote-master@df4dcf72582/api/node_modules/accepts/LICENSE
(The MIT License)
Copyright (c) 2014 Jonathan Ong
Copyright (c) 2015 Douglas Christopher Wilson
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
neverwrote-master@df4dcf72582/api/node_modules/accepts/package.json
{
"_from": "accepts@~1.3.3",
"_id": "[email protected]",
"_inBundle": false,
"_integrity": "sha512-Il80Qs2WjYlJIBNzNkK6KYqlVMTbZLXgHx2oT0pU/fjRHyEp+PEfEPY0R3WCwAGVOtauxh1hOxNgIf5bv7dQpA==",
"_location": "/accepts",
"_phantomChildren": {},
"_requested": {
"type": "range",
"registry": true,
"raw": "accepts@~1.3.3",
"name": "accepts",
"escapedName": "accepts",
"rawSpec": "~1.3.3",
"saveSpec": null,
"fetchSpec": "~1.3.3"
},
"_requiredBy": [
"/express"
],
"_resolved": "https://registry.npmjs.org/accepts/-/accepts-1.3.7.tgz",
"_shasum": "531bc726517a3b2b41f850021c6cc15eaab507cd",
"_spec": "accepts@~1.3.3",
"_where": "D:\\Greynodes\\neverwrote-master@df4dcf72582\\api\\node_modules\\express",
"bugs": {
"url": "https://github.com/jshttp/accepts/issues"
},
"bundleDependencies": false,
"contributors": [
{
"name": "Douglas Christopher Wilson",
"email": "[email protected]"
},
{
"name": "Jonathan Ong",
"email": "[email protected]",
"url": "http://jongleberry.com"
}
],
"dependencies": {
"mime-types": "~2.1.24",
"negotiator": "0.6.2"
},
"deprecated": false,
"description": "Higher-level content negotiation",
"devDependencies": {
"deep-equal": "1.0.1",
"eslint": "5.16.0",
"eslint-config-standard": "12.0.0",
"eslint-plugin-import": "2.17.2",
"eslint-plugin-markdown": "1.0.0",
"eslint-plugin-node": "8.0.1",
"eslint-plugin-promise": "4.1.1",
"eslint-plugin-standard": "4.0.0",
"mocha": "6.1.4",
"nyc": "14.0.0"
},
"engines": {
"node": ">= 0.6"
},
"files": [
"LICENSE",
"HISTORY.md",
"index.js"
],
"homepage": "https://github.com/jshttp/accepts#readme",
"keywords": [
"content",
"negotiation",
"accept",
"accepts"
],
"license": "MIT",
"name": "accepts",
"repository": {
"type": "git",
"url": "git+https://github.com/jshttp/accepts.git"
},
"scripts": {
"lint": "eslint --plugin markdown --ext js,md .",
"test": "mocha --reporter spec --check-leaks --bail test/",
"test-cov": "nyc --reporter=html --reporter=text npm test",
"test-travis": "nyc --reporter=text npm test"
},
"version": "1.3.7"
}
neverwrote-master@df4dcf72582/api/node_modules/accepts/README.md
# accepts
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][travis-image]][travis-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Higher level content negotiation based on [negotiator](https://www.npmjs.com/package/negotiator).
Extracted from [koa](https://www.npmjs.com/package/koa) for general use.
In addition to negotiator, it allows:
- Allows types as an array or arguments list, ie `(['text/html', 'application/json'])`
as well as `('text/html', 'application/json')`.
- Allows type shorthands such as `json`.
- Returns `false` when no types match
- Treats non-existent headers as `*`
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install accepts
```
## API
```js
var accepts = require('accepts')
```
### accepts(req)
Create a new `Accepts` object for the given `req`.
#### .charset(charsets)
Return the first accepted charset. If nothing in `charsets` is accepted,
then `false` is returned.
#### .charsets()
Return the charsets that the request accepts, in the order of the client's
preference (most preferred first).
#### .encoding(encodings)
Return the first accepted encoding. If nothing in `encodings` is accepted,
then `false` is returned.
#### .encodings()
Return the encodings that the request accepts, in the order of the client's
preference (most preferred first).
#### .language(languages)
Return the first accepted language. If nothing in `languages` is accepted,
then `false` is returned.
#### .languages()
Return the languages that the request accepts, in the order of the client's
preference (most preferred first).
#### .type(types)
Return the first accepted type (and it is returned as the same text as what
appears in the `types` array). If nothing in `types` is accepted, then `false`
is returned.
The `types` array can contain full MIME types or file extensions. Any value
that is not a full MIME types is passed to `require('mime-types').lookup`.
#### .types()
Return the types that the request accepts, in the order of the client's
preference (most preferred first).
## Examples
### Simple type negotiation
This simple example shows how to use `accepts` to return a different typed
respond body based on what the client wants to accept. The server lists it's
preferences in order and will get back the best match between the client and
server.
```js
var accepts = require('accepts')
var http = require('http')
function app (req, res) {
var accept = accepts(req)
// the order of this list is significant; should be server preferred order
switch (accept.type(['json', 'html'])) {
case 'json':
res.setHeader('Content-Type', 'application/json')
res.write('{"hello":"world!"}')
break
case 'html':
res.setHeader('Content-Type', 'text/html')
res.write('hello, world!')
break
default:
// the fallback is text/plain, so no need to specify it above
res.setHeader('Content-Type', 'text/plain')
res.write('hello, world!')
break
}
res.end()
}
http.createServer(app).listen(3000)
```
You can test this out with the cURL program:
```sh
curl -I -H'Accept: text/html' http://localhost:3000/
```
## License
[MIT](LICENSE)
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/accepts/master
[coveralls-url]: https://coveralls.io/r/jshttp/accepts?branch=master
[node-version-image]: https://badgen.net/npm/node/accepts
[node-version-url]: https://nodejs.org/en/download
[npm-downloads-image]: https://badgen.net/npm/dm/accepts
[npm-url]: https://npmjs.org/package/accepts
[npm-version-image]: https://badgen.net/npm/v/accepts
[travis-image]: https://badgen.net/travis/jshttp/accepts/master
[travis-url]: https://travis-ci.org/jshttp/accepts
neverwrote-master@df4dcf72582/api/node_modules/array-flatten/array-flatten.js
'use strict'
/**
* Expose `arrayFlatten`.
*/
module.exports = arrayFlatten
/**
* Recursive flatten function with depth.
*
* @param {Array} array
* @param {Array} result
* @param {Number} depth
* @return {Array}
*/
function flattenWithDepth (array, result, depth) {
for (var i = 0; i < array.length; i++) {
var value = array[i]
if (depth > 0 && Array.isArray(value)) {
flattenWithDepth(value, result, depth - 1)
} else {
result.push(value)
}
}
return result
}
/**
* Recursive flatten function. Omitting depth is slightly faster.
*
* @param {Array} array
* @param {Array} result
* @return {Array}
*/
function flattenForever (array, result) {
for (var i = 0; i < array.length; i++) {
var value = array[i]
if (Array.isArray(value)) {
flattenForever(value, result)
} else {
result.push(value)
}
}
return result
}
/**
* Flatten an array, with the ability to define a depth.
*
* @param {Array} array
* @param {Number} depth
* @return {Array}
*/
function arrayFlatten (array, depth) {
if (depth == null) {
return flattenForever(array, [])
}
return flattenWithDepth(array, [], depth)
}
neverwrote-master@df4dcf72582/api/node_modules/array-flatten/LICENSE
The MIT License (MIT)
Copyright (c) 2014 Blake Embrey ([email protected])
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
neverwrote-master@df4dcf72582/api/node_modules/array-flatten/package.json
{
"_from": "[email protected]",
"_id": "[email protected]",
"_inBundle": false,
"_integrity": "sha1-ml9pkFGx5wczKPKgCJaLZOopVdI=",
"_location": "/array-flatten",
"_phantomChildren": {},
"_requested": {
"type": "version",
"registry": true,
"raw": "[email protected]",
"name": "array-flatten",
"escapedName": "array-flatten",
"rawSpec": "1.1.1",
"saveSpec": null,
"fetchSpec": "1.1.1"
},
"_requiredBy": [
"/express"
],
"_resolved": "https://registry.npmjs.org/array-flatten/-/array-flatten-1.1.1.tgz",
"_shasum": "9a5f699051b1e7073328f2a008968b64ea2955d2",
"_spec": "[email protected]",
"_where": "D:\\Greynodes\\neverwrote-master@df4dcf72582\\api\\node_modules\\express",
"author": {
"name": "Blake Embrey",
"email": "[email protected]",
"url": "http://blakeembrey.me"
},
"bugs": {
"url": "https://github.com/blakeembrey/array-flatten/issues"
},
"bundleDependencies": false,
"deprecated": false,
"description": "Flatten an array of nested arrays into a single flat array",
"devDependencies": {
"istanbul": "^0.3.13",
"mocha": "^2.2.4",
"pre-commit": "^1.0.7",
"standard": "^3.7.3"
},
"files": [
"array-flatten.js",
"LICENSE"
],
"homepage": "https://github.com/blakeembrey/array-flatten",
"keywords": [
"array",
"flatten",
"arguments",
"depth"
],
"license": "MIT",
"main": "array-flatten.js",
"name": "array-flatten",
"repository": {
"type": "git",
"url": "git://github.com/blakeembrey/array-flatten.git"
},
"scripts": {
"test": "istanbul cover _mocha -- -R spec"
},
"version": "1.1.1"
}
neverwrote-master@df4dcf72582/api/node_modules/array-flatten/README.md
# Array Flatten
[![NPM version][npm-image]][npm-url]
[![NPM downloads][downloads-image]][downloads-url]
[![Build status][travis-image]][travis-url]
[![Test coverage][coveralls-image]][coveralls-url]
> Flatten an array of nested arrays into a single flat array. Accepts an optional depth.
## Installation
```
npm install array-flatten --save
```
## Usage
```javascript
var flatten = require('array-flatten')
flatten([1, [2, [3, [4, [5], 6], 7], 8], 9])
//=> [1, 2, 3, 4, 5, 6, 7, 8, 9]
flatten([1, [2, [3, [4, [5], 6], 7], 8], 9], 2)
//=> [1, 2, 3, [4, [5], 6], 7, 8, 9]
(function () {
flatten(arguments) //=> [1, 2, 3]
})(1, [2, 3])
```
## License
MIT
[npm-image]: https://img.shields.io/npm/v/array-flatten.svg?style=flat
[npm-url]: https://npmjs.org/package/array-flatten
[downloads-image]: https://img.shields.io/npm/dm/array-flatten.svg?style=flat
[downloads-url]: https://npmjs.org/package/array-flatten
[travis-image]: https://img.shields.io/travis/blakeembrey/array-flatten.svg?style=flat
[travis-url]: https://travis-ci.org/blakeembrey/array-flatten
[coveralls-image]: https://img.shields.io/coveralls/blakeembrey/array-flatten.svg?style=flat
[coveralls-url]: https://coveralls.io/r/blakeembrey/array-flatten?branch=master
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/bignumber.js
/*! bignumber.js v3.1.2 https://github.com/MikeMcl/bignumber.js/LICENCE */
;(function (globalObj) {
'use strict';
/*
bignumber.js v3.1.2
A JavaScript library for arbitrary-precision arithmetic.
https://github.com/MikeMcl/bignumber.js
Copyright (c) 2016 Michael Mclaughlin
MIT Expat Licence
*/
var BigNumber,
isNumeric = /^-?(\d+(\.\d*)?|\.\d+)(e[+-]?\d+)?$/i,
mathceil = Math.ceil,
mathfloor = Math.floor,
notBool = ' not a boolean or binary digit',
roundingMode = 'rounding mode',
tooManyDigits = 'number type has more than 15 significant digits',
ALPHABET = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ$_',
BASE = 1e14,
LOG_BASE = 14,
MAX_SAFE_INTEGER = 0x1fffffffffffff, // 2^53 - 1
// MAX_INT32 = 0x7fffffff, // 2^31 - 1
POWS_TEN = [1, 10, 100, 1e3, 1e4, 1e5, 1e6, 1e7, 1e8, 1e9, 1e10, 1e11, 1e12, 1e13],
SQRT_BASE = 1e7,
/*
* The limit on the value of DECIMAL_PLACES, TO_EXP_NEG, TO_EXP_POS, MIN_EXP, MAX_EXP, and
* the arguments to toExponential, toFixed, toFormat, and toPrecision, beyond which an
* exception is thrown (if ERRORS is true).
*/
MAX = 1E9; // 0 to MAX_INT32
/*
* Create and return a BigNumber constructor.
*/
function constructorFactory(configObj) {
var div, parseNumeric,
// id tracks the caller function, so its name can be included in error messages.
id = 0,
P = BigNumber.prototype,
ONE = new BigNumber(1),
/********************************* EDITABLE DEFAULTS **********************************/
/*
* The default values below must be integers within the inclusive ranges stated.
* The values can also be changed at run-time using BigNumber.config.
*/
// The maximum number of decimal places for operations involving division.
DECIMAL_PLACES = 20, // 0 to MAX
/*
* The rounding mode used when rounding to the above decimal places, and when using
* toExponential, toFixed, toFormat and toPrecision, and round (default value).
* UP 0 Away from zero.
* DOWN 1 Towards zero.
* CEIL 2 Towards +Infinity.
* FLOOR 3 Towards -Infinity.
* HALF_UP 4 Towards nearest neighbour. If equidistant, up.
* HALF_DOWN 5 Towards nearest neighbour. If equidistant, down.
* HALF_EVEN 6 Towards nearest neighbour. If equidistant, towards even neighbour.
* HALF_CEIL 7 Towards nearest neighbour. If equidistant, towards +Infinity.
* HALF_FLOOR 8 Towards nearest neighbour. If equidistant, towards -Infinity.
*/
ROUNDING_MODE = 4, // 0 to 8
// EXPONENTIAL_AT : [TO_EXP_NEG , TO_EXP_POS]
// The exponent value at and beneath which toString returns exponential notation.
// Number type: -7
TO_EXP_NEG = -7, // 0 to -MAX
// The exponent value at and above which toString returns exponential notation.
// Number type: 21
TO_EXP_POS = 21, // 0 to MAX
// RANGE : [MIN_EXP, MAX_EXP]
// The minimum exponent value, beneath which underflow to zero occurs.
// Number type: -324 (5e-324)
MIN_EXP = -1e7, // -1 to -MAX
// The maximum exponent value, above which overflow to Infinity occurs.
// Number type: 308 (1.7976931348623157e+308)
// For MAX_EXP > 1e7, e.g. new BigNumber('1e100000000').plus(1) may be slow.
MAX_EXP = 1e7, // 1 to MAX
// Whether BigNumber Errors are ever thrown.
ERRORS = true, // true or false
// Change to intValidatorNoErrors if ERRORS is false.
isValidInt = intValidatorWithErrors, // intValidatorWithErrors/intValidatorNoErrors
// Whether to use cryptographically-secure random number generation, if available.
CRYPTO = false, // true or false
/*
* The modulo mode used when calculating the modulus: a mod n.
* The quotient (q = a / n) is calculated according to the corresponding rounding mode.
* The remainder (r) is calculated as: r = a - n * q.
*
* UP 0 The remainder is positive if the dividend is negative, else is negative.
* DOWN 1 The remainder has the same sign as the dividend.
* This modulo mode is commonly known as 'truncated division' and is
* equivalent to (a % n) in JavaScript.
* FLOOR 3 The remainder has the same sign as the divisor (Python %).
* HALF_EVEN 6 This modulo mode implements the IEEE 754 remainder function.
* EUCLID 9 Euclidian division. q = sign(n) * floor(a / abs(n)).
* The remainder is always positive.
*
* The truncated division, floored division, Euclidian division and IEEE 754 remainder
* modes are commonly used for the modulus operation.
* Although the other rounding modes can also be used, they may not give useful results.
*/
MODULO_MODE = 1, // 0 to 9
// The maximum number of significant digits of the result of the toPower operation.
// If POW_PRECISION is 0, there will be unlimited significant digits.
POW_PRECISION = 0, // 0 to MAX
// The format specification used by the BigNumber.prototype.toFormat method.
FORMAT = {
decimalSeparator: '.',
groupSeparator: ',',
groupSize: 3,
secondaryGroupSize: 0,
fractionGroupSeparator: '\xA0', // non-breaking space
fractionGroupSize: 0
};
/******************************************************************************************/
// CONSTRUCTOR
/*
* The BigNumber constructor and exported function.
* Create and return a new instance of a BigNumber object.
*
* n {number|string|BigNumber} A numeric value.
* [b] {number} The base of n. Integer, 2 to 64 inclusive.
*/
function BigNumber( n, b ) {
var c, e, i, num, len, str,
x = this;
// Enable constructor usage without new.
if ( !( x instanceof BigNumber ) ) {
// 'BigNumber() constructor call without new: {n}'
if (ERRORS) raise( 26, 'constructor call without new', n );
return new BigNumber( n, b );
}
// 'new BigNumber() base not an integer: {b}'
// 'new BigNumber() base out of range: {b}'
if ( b == null || !isValidInt( b, 2, 64, id, 'base' ) ) {
// Duplicate.
if ( n instanceof BigNumber ) {
x.s = n.s;
x.e = n.e;
x.c = ( n = n.c ) ? n.slice() : n;
id = 0;
return;
}
if ( ( num = typeof n == 'number' ) && n * 0 == 0 ) {
x.s = 1 / n < 0 ? ( n = -n, -1 ) : 1;
// Fast path for integers.
if ( n === ~~n ) {
for ( e = 0, i = n; i >= 10; i /= 10, e++ );
x.e = e;
x.c = [n];
id = 0;
return;
}
str = n + '';
} else {
if ( !isNumeric.test( str = n + '' ) ) return parseNumeric( x, str, num );
x.s = str.charCodeAt(0) === 45 ? ( str = str.slice(1), -1 ) : 1;
}
} else {
b = b | 0;
str = n + '';
// Ensure return value is rounded to DECIMAL_PLACES as with other bases.
// Allow exponential notation to be used with base 10 argument.
if ( b == 10 ) {
x = new BigNumber( n instanceof BigNumber ? n : str );
return round( x, DECIMAL_PLACES + x.e + 1, ROUNDING_MODE );
}
// Avoid potential interpretation of Infinity and NaN as base 44+ values.
// Any number in exponential form will fail due to the [Ee][+-].
if ( ( num = typeof n == 'number' ) && n * 0 != 0 ||
!( new RegExp( '^-?' + ( c = '[' + ALPHABET.slice( 0, b ) + ']+' ) +
'(?:\\.' + c + ')?$',b < 37 ? 'i' : '' ) ).test(str) ) {
return parseNumeric( x, str, num, b );
}
if (num) {
x.s = 1 / n < 0 ? ( str = str.slice(1), -1 ) : 1;
if ( ERRORS && str.replace( /^0\.0*|\./, '' ).length > 15 ) {
// 'new BigNumber() number type has more than 15 significant digits: {n}'
raise( id, tooManyDigits, n );
}
// Prevent later check for length on converted number.
num = false;
} else {
x.s = str.charCodeAt(0) === 45 ? ( str = str.slice(1), -1 ) : 1;
}
str = convertBase( str, 10, b, x.s );
}
// Decimal point?
if ( ( e = str.indexOf('.') ) > -1 ) str = str.replace( '.', '' );
// Exponential form?
if ( ( i = str.search( /e/i ) ) > 0 ) {
// Determine exponent.
if ( e < 0 ) e = i;
e += +str.slice( i + 1 );
str = str.substring( 0, i );
} else if ( e < 0 ) {
// Integer.
e = str.length;
}
// Determine leading zeros.
for ( i = 0; str.charCodeAt(i) === 48; i++ );
// Determine trailing zeros.
for ( len = str.length; str.charCodeAt(--len) === 48; );
str = str.slice( i, len + 1 );
if (str) {
len = str.length;
// Disallow numbers with over 15 significant digits if number type.
// 'new BigNumber() number type has more than 15 significant digits: {n}'
if ( num && ERRORS && len > 15 && ( n > MAX_SAFE_INTEGER || n !== mathfloor(n) ) ) {
raise( id, tooManyDigits, x.s * n );
}
e = e - i - 1;
// Overflow?
if ( e > MAX_EXP ) {
// Infinity.
x.c = x.e = null;
// Underflow?
} else if ( e < MIN_EXP ) {
// Zero.
x.c = [ x.e = 0 ];
} else {
x.e = e;
x.c = [];
// Transform base
// e is the base 10 exponent.
// i is where to slice str to get the first element of the coefficient array.
i = ( e + 1 ) % LOG_BASE;
if ( e < 0 ) i += LOG_BASE;
if ( i < len ) {
if (i) x.c.push( +str.slice( 0, i ) );
for ( len -= LOG_BASE; i < len; ) {
x.c.push( +str.slice( i, i += LOG_BASE ) );
}
str = str.slice(i);
i = LOG_BASE - str.length;
} else {
i -= len;
}
for ( ; i--; str += '0' );
x.c.push( +str );
}
} else {
// Zero.
x.c = [ x.e = 0 ];
}
id = 0;
}
// CONSTRUCTOR PROPERTIES
BigNumber.another = constructorFactory;
BigNumber.ROUND_UP = 0;
BigNumber.ROUND_DOWN = 1;
BigNumber.ROUND_CEIL = 2;
BigNumber.ROUND_FLOOR = 3;
BigNumber.ROUND_HALF_UP = 4;
BigNumber.ROUND_HALF_DOWN = 5;
BigNumber.ROUND_HALF_EVEN = 6;
BigNumber.ROUND_HALF_CEIL = 7;
BigNumber.ROUND_HALF_FLOOR = 8;
BigNumber.EUCLID = 9;
/*
* Configure infrequently-changing library-wide settings.
*
* Accept an object or an argument list, with one or many of the following properties or
* parameters respectively:
*
* DECIMAL_PLACES {number} Integer, 0 to MAX inclusive
* ROUNDING_MODE {number} Integer, 0 to 8 inclusive
* EXPONENTIAL_AT {number|number[]} Integer, -MAX to MAX inclusive or
* [integer -MAX to 0 incl., 0 to MAX incl.]
* RANGE {number|number[]} Non-zero integer, -MAX to MAX inclusive or
* [integer -MAX to -1 incl., integer 1 to MAX incl.]
* ERRORS {boolean|number} true, false, 1 or 0
* CRYPTO {boolean|number} true, false, 1 or 0
* MODULO_MODE {number} 0 to 9 inclusive
* POW_PRECISION {number} 0 to MAX inclusive
* FORMAT {object} See BigNumber.prototype.toFormat
* decimalSeparator {string}
* groupSeparator {string}
* groupSize {number}
* secondaryGroupSize {number}
* fractionGroupSeparator {string}
* fractionGroupSize {number}
*
* (The values assigned to the above FORMAT object properties are not checked for validity.)
*
* E.g.
* BigNumber.config(20, 4) is equivalent to
* BigNumber.config({ DECIMAL_PLACES : 20, ROUNDING_MODE : 4 })
*
* Ignore properties/parameters set to null or undefined.
* Return an object with the properties current values.
*/
BigNumber.config = BigNumber.set = function () {
var v, p,
i = 0,
r = {},
a = arguments,
o = a[0],
has = o && typeof o == 'object'
? function () { if ( o.hasOwnProperty(p) ) return ( v = o[p] ) != null; }
: function () { if ( a.length > i ) return ( v = a[i++] ) != null; };
// DECIMAL_PLACES {number} Integer, 0 to MAX inclusive.
// 'config() DECIMAL_PLACES not an integer: {v}'
// 'config() DECIMAL_PLACES out of range: {v}'
if ( has( p = 'DECIMAL_PLACES' ) && isValidInt( v, 0, MAX, 2, p ) ) {
DECIMAL_PLACES = v | 0;
}
r[p] = DECIMAL_PLACES;
// ROUNDING_MODE {number} Integer, 0 to 8 inclusive.
// 'config() ROUNDING_MODE not an integer: {v}'
// 'config() ROUNDING_MODE out of range: {v}'
if ( has( p = 'ROUNDING_MODE' ) && isValidInt( v, 0, 8, 2, p ) ) {
ROUNDING_MODE = v | 0;
}
r[p] = ROUNDING_MODE;
// EXPONENTIAL_AT {number|number[]}
// Integer, -MAX to MAX inclusive or [integer -MAX to 0 inclusive, 0 to MAX inclusive].
// 'config() EXPONENTIAL_AT not an integer: {v}'
// 'config() EXPONENTIAL_AT out of range: {v}'
if ( has( p = 'EXPONENTIAL_AT' ) ) {
if ( isArray(v) ) {
if ( isValidInt( v[0], -MAX, 0, 2, p ) && isValidInt( v[1], 0, MAX, 2, p ) ) {
TO_EXP_NEG = v[0] | 0;
TO_EXP_POS = v[1] | 0;
}
} else if ( isValidInt( v, -MAX, MAX, 2, p ) ) {
TO_EXP_NEG = -( TO_EXP_POS = ( v < 0 ? -v : v ) | 0 );
}
}
r[p] = [ TO_EXP_NEG, TO_EXP_POS ];
// RANGE {number|number[]} Non-zero integer, -MAX to MAX inclusive or
// [integer -MAX to -1 inclusive, integer 1 to MAX inclusive].
// 'config() RANGE not an integer: {v}'
// 'config() RANGE cannot be zero: {v}'
// 'config() RANGE out of range: {v}'
if ( has( p = 'RANGE' ) ) {
if ( isArray(v) ) {
if ( isValidInt( v[0], -MAX, -1, 2, p ) && isValidInt( v[1], 1, MAX, 2, p ) ) {
MIN_EXP = v[0] | 0;
MAX_EXP = v[1] | 0;
}
} else if ( isValidInt( v, -MAX, MAX, 2, p ) ) {
if ( v | 0 ) MIN_EXP = -( MAX_EXP = ( v < 0 ? -v : v ) | 0 );
else if (ERRORS) raise( 2, p + ' cannot be zero', v );
}
}
r[p] = [ MIN_EXP, MAX_EXP ];
// ERRORS {boolean|number} true, false, 1 or 0.
// 'config() ERRORS not a boolean or binary digit: {v}'
if ( has( p = 'ERRORS' ) ) {
if ( v === !!v || v === 1 || v === 0 ) {
id = 0;
isValidInt = ( ERRORS = !!v ) ? intValidatorWithErrors : intValidatorNoErrors;
} else if (ERRORS) {
raise( 2, p + notBool, v );
}
}
r[p] = ERRORS;
// CRYPTO {boolean|number} true, false, 1 or 0.
// 'config() CRYPTO not a boolean or binary digit: {v}'
// 'config() crypto unavailable: {crypto}'
if ( has( p = 'CRYPTO' ) ) {
if ( v === true || v === false || v === 1 || v === 0 ) {
if (v) {
v = typeof crypto == 'undefined';
if ( !v && crypto && (crypto.getRandomValues || crypto.randomBytes)) {
CRYPTO = true;
} else if (ERRORS) {
raise( 2, 'crypto unavailable', v ? void 0 : crypto );
} else {
CRYPTO = false;
}
} else {
CRYPTO = false;
}
} else if (ERRORS) {
raise( 2, p + notBool, v );
}
}
r[p] = CRYPTO;
// MODULO_MODE {number} Integer, 0 to 9 inclusive.
// 'config() MODULO_MODE not an integer: {v}'
// 'config() MODULO_MODE out of range: {v}'
if ( has( p = 'MODULO_MODE' ) && isValidInt( v, 0, 9, 2, p ) ) {
MODULO_MODE = v | 0;
}
r[p] = MODULO_MODE;
// POW_PRECISION {number} Integer, 0 to MAX inclusive.
// 'config() POW_PRECISION not an integer: {v}'
// 'config() POW_PRECISION out of range: {v}'
if ( has( p = 'POW_PRECISION' ) && isValidInt( v, 0, MAX, 2, p ) ) {
POW_PRECISION = v | 0;
}
r[p] = POW_PRECISION;
// FORMAT {object}
// 'config() FORMAT not an object: {v}'
if ( has( p = 'FORMAT' ) ) {
if ( typeof v == 'object' ) {
FORMAT = v;
} else if (ERRORS) {
raise( 2, p + ' not an object', v );
}
}
r[p] = FORMAT;
return r;
};
/*
* Return true if value v is a BigNumber instance, otherwise return false.
*
* v {any} A value that may or may not be a BigNumber instance.
*/
BigNumber.isBigNumber = isBigNumber;
/*
* Return a new BigNumber whose value is the maximum of the arguments.
*
* arguments {number|string|BigNumber}
*/
BigNumber.max = function () { return maxOrMin( arguments, P.lt ); };
/*
* Return a new BigNumber whose value is the minimum of the arguments.
*
* arguments {number|string|BigNumber}
*/
BigNumber.min = function () { return maxOrMin( arguments, P.gt ); };
/*
* Return a new BigNumber with a random value equal to or greater than 0 and less than 1,
* and with dp, or DECIMAL_PLACES if dp is omitted, decimal places (or less if trailing
* zeros are produced).
*
* [dp] {number} Decimal places. Integer, 0 to MAX inclusive.
*
* 'random() decimal places not an integer: {dp}'
* 'random() decimal places out of range: {dp}'
* 'random() crypto unavailable: {crypto}'
*/
BigNumber.random = (function () {
var pow2_53 = 0x20000000000000;
// Return a 53 bit integer n, where 0 <= n < 9007199254740992.
// Check if Math.random() produces more than 32 bits of randomness.
// If it does, assume at least 53 bits are produced, otherwise assume at least 30 bits.
// 0x40000000 is 2^30, 0x800000 is 2^23, 0x1fffff is 2^21 - 1.
var random53bitInt = (Math.random() * pow2_53) & 0x1fffff
? function () { return mathfloor( Math.random() * pow2_53 ); }
: function () { return ((Math.random() * 0x40000000 | 0) * 0x800000) +
(Math.random() * 0x800000 | 0); };
return function (dp) {
var a, b, e, k, v,
i = 0,
c = [],
rand = new BigNumber(ONE);
dp = dp == null || !isValidInt( dp, 0, MAX, 14 ) ? DECIMAL_PLACES : dp | 0;
k = mathceil( dp / LOG_BASE );
if (CRYPTO) {
// Browsers supporting crypto.getRandomValues.
if (crypto.getRandomValues) {
a = crypto.getRandomValues( new Uint32Array( k *= 2 ) );
for ( ; i < k; ) {
// 53 bits:
// ((Math.pow(2, 32) - 1) * Math.pow(2, 21)).toString(2)
// 11111 11111111 11111111 11111111 11100000 00000000 00000000
// ((Math.pow(2, 32) - 1) >>> 11).toString(2)
//11111 11111111 11111111
// 0x20000 is 2^21.
v = a[i] * 0x20000 + (a[i + 1] >>> 11);
// Rejection sampling:
// 0 <= v < 9007199254740992
// Probability that v >= 9e15, is
// 7199254740992 / 9007199254740992 ~= 0.0008, i.e. 1 in 1251
if ( v >= 9e15 ) {
b = crypto.getRandomValues( new Uint32Array(2) );
a[i] = b[0];
a[i + 1] = b[1];
} else {
// 0 <= v <= 8999999999999999
// 0 <= (v % 1e14) <= 99999999999999
c.push( v % 1e14 );
i += 2;
}
}
i = k / 2;
// Node.js supporting crypto.randomBytes.
} else if (crypto.randomBytes) {
// buffer
a = crypto.randomBytes( k *= 7 );
for ( ; i < k; ) {
// 0x1000000000000 is 2^48, 0x10000000000 is 2^40
// 0x100000000 is 2^32, 0x1000000 is 2^24
// 11111 11111111 11111111 11111111 11111111 11111111 11111111
// 0 <= v < 9007199254740992
v = ( ( a[i] & 31 ) * 0x1000000000000 ) + ( a[i + 1] * 0x10000000000 ) +
( a[i + 2] * 0x100000000 ) + ( a[i + 3] * 0x1000000 ) +
( a[i + 4] << 16 ) + ( a[i + 5] << 8 ) + a[i + 6];
if ( v >= 9e15 ) {
crypto.randomBytes(7).copy( a, i );
} else {
// 0 <= (v % 1e14) <= 99999999999999
c.push( v % 1e14 );
i += 7;
}
}
i = k / 7;
} else {
CRYPTO = false;
if (ERRORS) raise( 14, 'crypto unavailable', crypto );
}
}
// Use Math.random.
if (!CRYPTO) {
for ( ; i < k; ) {
v = random53bitInt();
if ( v < 9e15 ) c[i++] = v % 1e14;
}
}
k = c[--i];
dp %= LOG_BASE;
// Convert trailing digits to zeros according to dp.
if ( k && dp ) {
v = POWS_TEN[LOG_BASE - dp];
c[i] = mathfloor( k / v ) * v;
}
// Remove trailing elements which are zero.
for ( ; c[i] === 0; c.pop(), i-- );
// Zero?
if ( i < 0 ) {
c = [ e = 0 ];
} else {
// Remove leading elements which are zero and adjust exponent accordingly.
for ( e = -1 ; c[0] === 0; c.shift(), e -= LOG_BASE);
// Count the digits of the first element of c to determine leading zeros, and...
for ( i = 1, v = c[0]; v >= 10; v /= 10, i++);
// adjust the exponent accordingly.
if ( i < LOG_BASE ) e -= LOG_BASE - i;
}
rand.e = e;
rand.c = c;
return rand;
};
})();
// PRIVATE FUNCTIONS
// Convert a numeric string of baseIn to a numeric string of baseOut.
function convertBase( str, baseOut, baseIn, sign ) {
var d, e, k, r, x, xc, y,
i = str.indexOf( '.' ),
dp = DECIMAL_PLACES,
rm = ROUNDING_MODE;
if ( baseIn < 37 ) str = str.toLowerCase();
// Non-integer.
if ( i >= 0 ) {
k = POW_PRECISION;
// Unlimited precision.
POW_PRECISION = 0;
str = str.replace( '.', '' );
y = new BigNumber(baseIn);
x = y.pow( str.length - i );
POW_PRECISION = k;
// Convert str as if an integer, then restore the fraction part by dividing the
// result by its base raised to a power.
y.c = toBaseOut( toFixedPoint( coeffToString( x.c ), x.e ), 10, baseOut );
y.e = y.c.length;
}
// Convert the number as integer.
xc = toBaseOut( str, baseIn, baseOut );
e = k = xc.length;
// Remove trailing zeros.
for ( ; xc[--k] == 0; xc.pop() );
if ( !xc[0] ) return '0';
if ( i < 0 ) {
--e;
} else {
x.c = xc;
x.e = e;
// sign is needed for correct rounding.
x.s = sign;
x = div( x, y, dp, rm, baseOut );
xc = x.c;
r = x.r;
e = x.e;
}
d = e + dp + 1;
// The rounding digit, i.e. the digit to the right of the digit that may be rounded up.
i = xc[d];
k = baseOut / 2;
r = r || d < 0 || xc[d + 1] != null;
r = rm < 4 ? ( i != null || r ) && ( rm == 0 || rm == ( x.s < 0 ? 3 : 2 ) )
: i > k || i == k &&( rm == 4 || r || rm == 6 && xc[d - 1] & 1 ||
rm == ( x.s < 0 ? 8 : 7 ) );
if ( d < 1 || !xc[0] ) {
// 1^-dp or 0.
str = r ? toFixedPoint( '1', -dp ) : '0';
} else {
xc.length = d;
if (r) {
// Rounding up may mean the previous digit has to be rounded up and so on.
for ( --baseOut; ++xc[--d] > baseOut; ) {
xc[d] = 0;
if ( !d ) {
++e;
xc.unshift(1);
}
}
}
// Determine trailing zeros.
for ( k = xc.length; !xc[--k]; );
// E.g. [4, 11, 15] becomes 4bf.
for ( i = 0, str = ''; i <= k; str += ALPHABET.charAt( xc[i++] ) );
str = toFixedPoint( str, e );
}
// The caller will add the sign.
return str;
}
// Perform division in the specified base. Called by div and convertBase.
div = (function () {
// Assume non-zero x and k.
function multiply( x, k, base ) {
var m, temp, xlo, xhi,
carry = 0,
i = x.length,
klo = k % SQRT_BASE,
khi = k / SQRT_BASE | 0;
for ( x = x.slice(); i--; ) {
xlo = x[i] % SQRT_BASE;
xhi = x[i] / SQRT_BASE | 0;
m = khi * xlo + xhi * klo;
temp = klo * xlo + ( ( m % SQRT_BASE ) * SQRT_BASE ) + carry;
carry = ( temp / base | 0 ) + ( m / SQRT_BASE | 0 ) + khi * xhi;
x[i] = temp % base;
}
if (carry) x.unshift(carry);
return x;
}
function compare( a, b, aL, bL ) {
var i, cmp;
if ( aL != bL ) {
cmp = aL > bL ? 1 : -1;
} else {
for ( i = cmp = 0; i < aL; i++ ) {
if ( a[i] != b[i] ) {
cmp = a[i] > b[i] ? 1 : -1;
break;
}
}
}
return cmp;
}
function subtract( a, b, aL, base ) {
var i = 0;
// Subtract b from a.
for ( ; aL--; ) {
a[aL] -= i;
i = a[aL] < b[aL] ? 1 : 0;
a[aL] = i * base + a[aL] - b[aL];
}
// Remove leading zeros.
for ( ; !a[0] && a.length > 1; a.shift() );
}
// x: dividend, y: divisor.
return function ( x, y, dp, rm, base ) {
var cmp, e, i, more, n, prod, prodL, q, qc, rem, remL, rem0, xi, xL, yc0,
yL, yz,
s = x.s == y.s ? 1 : -1,
xc = x.c,
yc = y.c;
// Either NaN, Infinity or 0?
if ( !xc || !xc[0] || !yc || !yc[0] ) {
return new BigNumber(
// Return NaN if either NaN, or both Infinity or 0.
!x.s || !y.s || ( xc ? yc && xc[0] == yc[0] : !yc ) ? NaN :
// Return ±0 if x is ±0 or y is ±Infinity, or return ±Infinity as y is ±0.
xc && xc[0] == 0 || !yc ? s * 0 : s / 0
);
}
q = new BigNumber(s);
qc = q.c = [];
e = x.e - y.e;
s = dp + e + 1;
if ( !base ) {
base = BASE;
e = bitFloor( x.e / LOG_BASE ) - bitFloor( y.e / LOG_BASE );
s = s / LOG_BASE | 0;
}
// Result exponent may be one less then the current value of e.
// The coefficients of the BigNumbers from convertBase may have trailing zeros.
for ( i = 0; yc[i] == ( xc[i] || 0 ); i++ );
if ( yc[i] > ( xc[i] || 0 ) ) e--;
if ( s < 0 ) {
qc.push(1);
more = true;
} else {
xL = xc.length;
yL = yc.length;
i = 0;
s += 2;
// Normalise xc and yc so highest order digit of yc is >= base / 2.
n = mathfloor( base / ( yc[0] + 1 ) );
// Not necessary, but to handle odd bases where yc[0] == ( base / 2 ) - 1.
// if ( n > 1 || n++ == 1 && yc[0] < base / 2 ) {
if ( n > 1 ) {
yc = multiply( yc, n, base );
xc = multiply( xc, n, base );
yL = yc.length;
xL = xc.length;
}
xi = yL;
rem = xc.slice( 0, yL );
remL = rem.length;
// Add zeros to make remainder as long as divisor.
for ( ; remL < yL; rem[remL++] = 0 );
yz = yc.slice();
yz.unshift(0);
yc0 = yc[0];
if ( yc[1] >= base / 2 ) yc0++;
// Not necessary, but to prevent trial digit n > base, when using base 3.
// else if ( base == 3 && yc0 == 1 ) yc0 = 1 + 1e-15;
do {
n = 0;
// Compare divisor and remainder.
cmp = compare( yc, rem, yL, remL );
// If divisor < remainder.
if ( cmp < 0 ) {
// Calculate trial digit, n.
rem0 = rem[0];
if ( yL != remL ) rem0 = rem0 * base + ( rem[1] || 0 );
// n is how many times the divisor goes into the current remainder.
n = mathfloor( rem0 / yc0 );
// Algorithm:
// 1. product = divisor * trial digit (n)
// 2. if product > remainder: product -= divisor, n--
// 3. remainder -= product
// 4. if product was < remainder at 2:
// 5. compare new remainder and divisor
// 6. If remainder > divisor: remainder -= divisor, n++
if ( n > 1 ) {
// n may be > base only when base is 3.
if (n >= base) n = base - 1;
// product = divisor * trial digit.
prod = multiply( yc, n, base );
prodL = prod.length;
remL = rem.length;
// Compare product and remainder.
// If product > remainder.
// Trial digit n too high.
// n is 1 too high about 5% of the time, and is not known to have
// ever been more than 1 too high.
while ( compare( prod, rem, prodL, remL ) == 1 ) {
n--;
// Subtract divisor from product.
subtract( prod, yL < prodL ? yz : yc, prodL, base );
prodL = prod.length;
cmp = 1;
}
} else {
// n is 0 or 1, cmp is -1.
// If n is 0, there is no need to compare yc and rem again below,
// so change cmp to 1 to avoid it.
// If n is 1, leave cmp as -1, so yc and rem are compared again.
if ( n == 0 ) {
// divisor < remainder, so n must be at least 1.
cmp = n = 1;
}
// product = divisor
prod = yc.slice();
prodL = prod.length;
}
if ( prodL < remL ) prod.unshift(0);
// Subtract product from remainder.
subtract( rem, prod, remL, base );
remL = rem.length;
// If product was < remainder.
if ( cmp == -1 ) {
// Compare divisor and new remainder.
// If divisor < new remainder, subtract divisor from remainder.
// Trial digit n too low.
// n is 1 too low about 5% of the time, and very rarely 2 too low.
while ( compare( yc, rem, yL, remL ) < 1 ) {
n++;
// Subtract divisor from remainder.
subtract( rem, yL < remL ? yz : yc, remL, base );
remL = rem.length;
}
}
} else if ( cmp === 0 ) {
n++;
rem = [0];
} // else cmp === 1 and n will be 0
// Add the next digit, n, to the result array.
qc[i++] = n;
// Update the remainder.
if ( rem[0] ) {
rem[remL++] = xc[xi] || 0;
} else {
rem = [ xc[xi] ];
remL = 1;
}
} while ( ( xi++ < xL || rem[0] != null ) && s-- );
more = rem[0] != null;
// Leading zero?
if ( !qc[0] ) qc.shift();
}
if ( base == BASE ) {
// To calculate q.e, first get the number of digits of qc[0].
for ( i = 1, s = qc[0]; s >= 10; s /= 10, i++ );
round( q, dp + ( q.e = i + e * LOG_BASE - 1 ) + 1, rm, more );
// Caller is convertBase.
} else {
q.e = e;
q.r = +more;
}
return q;
};
})();
/*
* Return a string representing the value of BigNumber n in fixed-point or exponential
* notation rounded to the specified decimal places or significant digits.
*
* n is a BigNumber.
* i is the index of the last digit required (i.e. the digit that may be rounded up).
* rm is the rounding mode.
* caller is caller id: toExponential 19, toFixed 20, toFormat 21, toPrecision 24.
*/
function format( n, i, rm, caller ) {
var c0, e, ne, len, str;
rm = rm != null && isValidInt( rm, 0, 8, caller, roundingMode )
? rm | 0 : ROUNDING_MODE;
if ( !n.c ) return n.toString();
c0 = n.c[0];
ne = n.e;
if ( i == null ) {
str = coeffToString( n.c );
str = caller == 19 || caller == 24 && ne <= TO_EXP_NEG
? toExponential( str, ne )
: toFixedPoint( str, ne );
} else {
n = round( new BigNumber(n), i, rm );
// n.e may have changed if the value was rounded up.
e = n.e;
str = coeffToString( n.c );
len = str.length;
// toPrecision returns exponential notation if the number of significant digits
// specified is less than the number of digits necessary to represent the integer
// part of the value in fixed-point notation.
// Exponential notation.
if ( caller == 19 || caller == 24 && ( i <= e || e <= TO_EXP_NEG ) ) {
// Append zeros?
for ( ; len < i; str += '0', len++ );
str = toExponential( str, e );
// Fixed-point notation.
} else {
i -= ne;
str = toFixedPoint( str, e );
// Append zeros?
if ( e + 1 > len ) {
if ( --i > 0 ) for ( str += '.'; i--; str += '0' );
} else {
i += e - len;
if ( i > 0 ) {
if ( e + 1 == len ) str += '.';
for ( ; i--; str += '0' );
}
}
}
}
return n.s < 0 && c0 ? '-' + str : str;
}
// Handle BigNumber.max and BigNumber.min.
function maxOrMin( args, method ) {
var m, n,
i = 0;
if ( isArray( args[0] ) ) args = args[0];
m = new BigNumber( args[0] );
for ( ; ++i < args.length; ) {
n = new BigNumber( args[i] );
// If any number is NaN, return NaN.
if ( !n.s ) {
m = n;
break;
} else if ( method.call( m, n ) ) {
m = n;
}
}
return m;
}
/*
* Return true if n is an integer in range, otherwise throw.
* Use for argument validation when ERRORS is true.
*/
function intValidatorWithErrors( n, min, max, caller, name ) {
if ( n < min || n > max || n != truncate(n) ) {
raise( caller, ( name || 'decimal places' ) +
( n < min || n > max ? ' out of range' : ' not an integer' ), n );
}
return true;
}
/*
* Strip trailing zeros, calculate base 10 exponent and check against MIN_EXP and MAX_EXP.
* Called by minus, plus and times.
*/
function normalise( n, c, e ) {
var i = 1,
j = c.length;
// Remove trailing zeros.
for ( ; !c[--j]; c.pop() );
// Calculate the base 10 exponent. First get the number of digits of c[0].
for ( j = c[0]; j >= 10; j /= 10, i++ );
// Overflow?
if ( ( e = i + e * LOG_BASE - 1 ) > MAX_EXP ) {
// Infinity.
n.c = n.e = null;
// Underflow?
} else if ( e < MIN_EXP ) {
// Zero.
n.c = [ n.e = 0 ];
} else {
n.e = e;
n.c = c;
}
return n;
}
// Handle values that fail the validity test in BigNumber.
parseNumeric = (function () {
var basePrefix = /^(-?)0([xbo])(?=\w[\w.]*$)/i,
dotAfter = /^([^.]+)\.$/,
dotBefore = /^\.([^.]+)$/,
isInfinityOrNaN = /^-?(Infinity|NaN)$/,
whitespaceOrPlus = /^\s*\+(?=[\w.])|^\s+|\s+$/g;
return function ( x, str, num, b ) {
var base,
s = num ? str : str.replace( whitespaceOrPlus, '' );
// No exception on ±Infinity or NaN.
if ( isInfinityOrNaN.test(s) ) {
x.s = isNaN(s) ? null : s < 0 ? -1 : 1;
} else {
if ( !num ) {
// basePrefix = /^(-?)0([xbo])(?=\w[\w.]*$)/i
s = s.replace( basePrefix, function ( m, p1, p2 ) {
base = ( p2 = p2.toLowerCase() ) == 'x' ? 16 : p2 == 'b' ? 2 : 8;
return !b || b == base ? p1 : m;
});
if (b) {
base = b;
// E.g. '1.' to '1', '.1' to '0.1'
s = s.replace( dotAfter, '$1' ).replace( dotBefore, '0.$1' );
}
if ( str != s ) return new BigNumber( s, base );
}
// 'new BigNumber() not a number: {n}'
// 'new BigNumber() not a base {b} number: {n}'
if (ERRORS) raise( id, 'not a' + ( b ? ' base ' + b : '' ) + ' number', str );
x.s = null;
}
x.c = x.e = null;
id = 0;
}
})();
// Throw a BigNumber Error.
function raise( caller, msg, val ) {
var error = new Error( [
'new BigNumber', // 0
'cmp', // 1
'config', // 2
'div', // 3
'divToInt', // 4
'eq', // 5
'gt', // 6
'gte', // 7
'lt', // 8
'lte', // 9
'minus', // 10
'mod', // 11
'plus', // 12
'precision', // 13
'random', // 14
'round', // 15
'shift', // 16
'times', // 17
'toDigits', // 18
'toExponential', // 19
'toFixed', // 20
'toFormat', // 21
'toFraction', // 22
'pow', // 23
'toPrecision', // 24
'toString', // 25
'BigNumber' // 26
][caller] + '() ' + msg + ': ' + val );
error.name = 'BigNumber Error';
id = 0;
throw error;
}
/*
* Round x to sd significant digits using rounding mode rm. Check for over/under-flow.
* If r is truthy, it is known that there are more digits after the rounding digit.
*/
function round( x, sd, rm, r ) {
var d, i, j, k, n, ni, rd,
xc = x.c,
pows10 = POWS_TEN;
// if x is not Infinity or NaN...
if (xc) {
// rd is the rounding digit, i.e. the digit after the digit that may be rounded up.
// n is a base 1e14 number, the value of the element of array x.c containing rd.
// ni is the index of n within x.c.
// d is the number of digits of n.
// i is the index of rd within n including leading zeros.
// j is the actual index of rd within n (if < 0, rd is a leading zero).
out: {
// Get the number of digits of the first element of xc.
for ( d = 1, k = xc[0]; k >= 10; k /= 10, d++ );
i = sd - d;
// If the rounding digit is in the first element of xc...
if ( i < 0 ) {
i += LOG_BASE;
j = sd;
n = xc[ ni = 0 ];
// Get the rounding digit at index j of n.
rd = n / pows10[ d - j - 1 ] % 10 | 0;
} else {
ni = mathceil( ( i + 1 ) / LOG_BASE );
if ( ni >= xc.length ) {
if (r) {
// Needed by sqrt.
for ( ; xc.length <= ni; xc.push(0) );
n = rd = 0;
d = 1;
i %= LOG_BASE;
j = i - LOG_BASE + 1;
} else {
break out;
}
} else {
n = k = xc[ni];
// Get the number of digits of n.
for ( d = 1; k >= 10; k /= 10, d++ );
// Get the index of rd within n.
i %= LOG_BASE;
// Get the index of rd within n, adjusted for leading zeros.
// The number of leading zeros of n is given by LOG_BASE - d.
j = i - LOG_BASE + d;
// Get the rounding digit at index j of n.
rd = j < 0 ? 0 : n / pows10[ d - j - 1 ] % 10 | 0;
}
}
r = r || sd < 0 ||
// Are there any non-zero digits after the rounding digit?
// The expression n % pows10[ d - j - 1 ] returns all digits of n to the right
// of the digit at j, e.g. if n is 908714 and j is 2, the expression gives 714.
xc[ni + 1] != null || ( j < 0 ? n : n % pows10[ d - j - 1 ] );
r = rm < 4
? ( rd || r ) && ( rm == 0 || rm == ( x.s < 0 ? 3 : 2 ) )
: rd > 5 || rd == 5 && ( rm == 4 || r || rm == 6 &&
// Check whether the digit to the left of the rounding digit is odd.
( ( i > 0 ? j > 0 ? n / pows10[ d - j ] : 0 : xc[ni - 1] ) % 10 ) & 1 ||
rm == ( x.s < 0 ? 8 : 7 ) );
if ( sd < 1 || !xc[0] ) {
xc.length = 0;
if (r) {
// Convert sd to decimal places.
sd -= x.e + 1;
// 1, 0.1, 0.01, 0.001, 0.0001 etc.
xc[0] = pows10[ ( LOG_BASE - sd % LOG_BASE ) % LOG_BASE ];
x.e = -sd || 0;
} else {
// Zero.
xc[0] = x.e = 0;
}
return x;
}
// Remove excess digits.
if ( i == 0 ) {
xc.length = ni;
k = 1;
ni--;
} else {
xc.length = ni + 1;
k = pows10[ LOG_BASE - i ];
// E.g. 56700 becomes 56000 if 7 is the rounding digit.
// j > 0 means i > number of leading zeros of n.
xc[ni] = j > 0 ? mathfloor( n / pows10[ d - j ] % pows10[j] ) * k : 0;
}
// Round up?
if (r) {
for ( ; ; ) {
// If the digit to be rounded up is in the first element of xc...
if ( ni == 0 ) {
// i will be the length of xc[0] before k is added.
for ( i = 1, j = xc[0]; j >= 10; j /= 10, i++ );
j = xc[0] += k;
for ( k = 1; j >= 10; j /= 10, k++ );
// if i != k the length has increased.
if ( i != k ) {
x.e++;
if ( xc[0] == BASE ) xc[0] = 1;
}
break;
} else {
xc[ni] += k;
if ( xc[ni] != BASE ) break;
xc[ni--] = 0;
k = 1;
}
}
}
// Remove trailing zeros.
for ( i = xc.length; xc[--i] === 0; xc.pop() );
}
// Overflow? Infinity.
if ( x.e > MAX_EXP ) {
x.c = x.e = null;
// Underflow? Zero.
} else if ( x.e < MIN_EXP ) {
x.c = [ x.e = 0 ];
}
}
return x;
}
// PROTOTYPE/INSTANCE METHODS
/*
* Return a new BigNumber whose value is the absolute value of this BigNumber.
*/
P.absoluteValue = P.abs = function () {
var x = new BigNumber(this);
if ( x.s < 0 ) x.s = 1;
return x;
};
/*
* Return a new BigNumber whose value is the value of this BigNumber rounded to a whole
* number in the direction of Infinity.
*/
P.ceil = function () {
return round( new BigNumber(this), this.e + 1, 2 );
};
/*
* Return
* 1 if the value of this BigNumber is greater than the value of BigNumber(y, b),
* -1 if the value of this BigNumber is less than the value of BigNumber(y, b),
* 0 if they have the same value,
* or null if the value of either is NaN.
*/
P.comparedTo = P.cmp = function ( y, b ) {
id = 1;
return compare( this, new BigNumber( y, b ) );
};
/*
* Return the number of decimal places of the value of this BigNumber, or null if the value
* of this BigNumber is ±Infinity or NaN.
*/
P.decimalPlaces = P.dp = function () {
var n, v,
c = this.c;
if ( !c ) return null;
n = ( ( v = c.length - 1 ) - bitFloor( this.e / LOG_BASE ) ) * LOG_BASE;
// Subtract the number of trailing zeros of the last number.
if ( v = c[v] ) for ( ; v % 10 == 0; v /= 10, n-- );
if ( n < 0 ) n = 0;
return n;
};
/*
* n / 0 = I
* n / N = N
* n / I = 0
* 0 / n = 0
* 0 / 0 = N
* 0 / N = N
* 0 / I = 0
* N / n = N
* N / 0 = N
* N / N = N
* N / I = N
* I / n = I
* I / 0 = I
* I / N = N
* I / I = N
*
* Return a new BigNumber whose value is the value of this BigNumber divided by the value of
* BigNumber(y, b), rounded according to DECIMAL_PLACES and ROUNDING_MODE.
*/
P.dividedBy = P.div = function ( y, b ) {
id = 3;
return div( this, new BigNumber( y, b ), DECIMAL_PLACES, ROUNDING_MODE );
};
/*
* Return a new BigNumber whose value is the integer part of dividing the value of this
* BigNumber by the value of BigNumber(y, b).
*/
P.dividedToIntegerBy = P.divToInt = function ( y, b ) {
id = 4;
return div( this, new BigNumber( y, b ), 0, 1 );
};
/*
* Return true if the value of this BigNumber is equal to the value of BigNumber(y, b),
* otherwise returns false.
*/
P.equals = P.eq = function ( y, b ) {
id = 5;
return compare( this, new BigNumber( y, b ) ) === 0;
};
/*
* Return a new BigNumber whose value is the value of this BigNumber rounded to a whole
* number in the direction of -Infinity.
*/
P.floor = function () {
return round( new BigNumber(this), this.e + 1, 3 );
};
/*
* Return true if the value of this BigNumber is greater than the value of BigNumber(y, b),
* otherwise returns false.
*/
P.greaterThan = P.gt = function ( y, b ) {
id = 6;
return compare( this, new BigNumber( y, b ) ) > 0;
};
/*
* Return true if the value of this BigNumber is greater than or equal to the value of
* BigNumber(y, b), otherwise returns false.
*/
P.greaterThanOrEqualTo = P.gte = function ( y, b ) {
id = 7;
return ( b = compare( this, new BigNumber( y, b ) ) ) === 1 || b === 0;
};
/*
* Return true if the value of this BigNumber is a finite number, otherwise returns false.
*/
P.isFinite = function () {
return !!this.c;
};
/*
* Return true if the value of this BigNumber is an integer, otherwise return false.
*/
P.isInteger = P.isInt = function () {
return !!this.c && bitFloor( this.e / LOG_BASE ) > this.c.length - 2;
};
/*
* Return true if the value of this BigNumber is NaN, otherwise returns false.
*/
P.isNaN = function () {
return !this.s;
};
/*
* Return true if the value of this BigNumber is negative, otherwise returns false.
*/
P.isNegative = P.isNeg = function () {
return this.s < 0;
};
/*
* Return true if the value of this BigNumber is 0 or -0, otherwise returns false.
*/
P.isZero = function () {
return !!this.c && this.c[0] == 0;
};
/*
* Return true if the value of this BigNumber is less than the value of BigNumber(y, b),
* otherwise returns false.
*/
P.lessThan = P.lt = function ( y, b ) {
id = 8;
return compare( this, new BigNumber( y, b ) ) < 0;
};
/*
* Return true if the value of this BigNumber is less than or equal to the value of
* BigNumber(y, b), otherwise returns false.
*/
P.lessThanOrEqualTo = P.lte = function ( y, b ) {
id = 9;
return ( b = compare( this, new BigNumber( y, b ) ) ) === -1 || b === 0;
};
/*
* n - 0 = n
* n - N = N
* n - I = -I
* 0 - n = -n
* 0 - 0 = 0
* 0 - N = N
* 0 - I = -I
* N - n = N
* N - 0 = N
* N - N = N
* N - I = N
* I - n = I
* I - 0 = I
* I - N = N
* I - I = N
*
* Return a new BigNumber whose value is the value of this BigNumber minus the value of
* BigNumber(y, b).
*/
P.minus = P.sub = function ( y, b ) {
var i, j, t, xLTy,
x = this,
a = x.s;
id = 10;
y = new BigNumber( y, b );
b = y.s;
// Either NaN?
if ( !a || !b ) return new BigNumber(NaN);
// Signs differ?
if ( a != b ) {
y.s = -b;
return x.plus(y);
}
var xe = x.e / LOG_BASE,
ye = y.e / LOG_BASE,
xc = x.c,
yc = y.c;
if ( !xe || !ye ) {
// Either Infinity?
if ( !xc || !yc ) return xc ? ( y.s = -b, y ) : new BigNumber( yc ? x : NaN );
// Either zero?
if ( !xc[0] || !yc[0] ) {
// Return y if y is non-zero, x if x is non-zero, or zero if both are zero.
return yc[0] ? ( y.s = -b, y ) : new BigNumber( xc[0] ? x :
// IEEE 754 (2008) 6.3: n - n = -0 when rounding to -Infinity
ROUNDING_MODE == 3 ? -0 : 0 );
}
}
xe = bitFloor(xe);
ye = bitFloor(ye);
xc = xc.slice();
// Determine which is the bigger number.
if ( a = xe - ye ) {
if ( xLTy = a < 0 ) {
a = -a;
t = xc;
} else {
ye = xe;
t = yc;
}
t.reverse();
// Prepend zeros to equalise exponents.
for ( b = a; b--; t.push(0) );
t.reverse();
} else {
// Exponents equal. Check digit by digit.
j = ( xLTy = ( a = xc.length ) < ( b = yc.length ) ) ? a : b;
for ( a = b = 0; b < j; b++ ) {
if ( xc[b] != yc[b] ) {
xLTy = xc[b] < yc[b];
break;
}
}
}
// x < y? Point xc to the array of the bigger number.
if (xLTy) t = xc, xc = yc, yc = t, y.s = -y.s;
b = ( j = yc.length ) - ( i = xc.length );
// Append zeros to xc if shorter.
// No need to add zeros to yc if shorter as subtract only needs to start at yc.length.
if ( b > 0 ) for ( ; b--; xc[i++] = 0 );
b = BASE - 1;
// Subtract yc from xc.
for ( ; j > a; ) {
if ( xc[--j] < yc[j] ) {
for ( i = j; i && !xc[--i]; xc[i] = b );
--xc[i];
xc[j] += BASE;
}
xc[j] -= yc[j];
}
// Remove leading zeros and adjust exponent accordingly.
for ( ; xc[0] == 0; xc.shift(), --ye );
// Zero?
if ( !xc[0] ) {
// Following IEEE 754 (2008) 6.3,
// n - n = +0 but n - n = -0 when rounding towards -Infinity.
y.s = ROUNDING_MODE == 3 ? -1 : 1;
y.c = [ y.e = 0 ];
return y;
}
// No need to check for Infinity as +x - +y != Infinity && -x - -y != Infinity
// for finite x and y.
return normalise( y, xc, ye );
};
/*
* n % 0 = N
* n % N = N
* n % I = n
* 0 % n = 0
* -0 % n = -0
* 0 % 0 = N
* 0 % N = N
* 0 % I = 0
* N % n = N
* N % 0 = N
* N % N = N
* N % I = N
* I % n = N
* I % 0 = N
* I % N = N
* I % I = N
*
* Return a new BigNumber whose value is the value of this BigNumber modulo the value of
* BigNumber(y, b). The result depends on the value of MODULO_MODE.
*/
P.modulo = P.mod = function ( y, b ) {
var q, s,
x = this;
id = 11;
y = new BigNumber( y, b );
// Return NaN if x is Infinity or NaN, or y is NaN or zero.
if ( !x.c || !y.s || y.c && !y.c[0] ) {
return new BigNumber(NaN);
// Return x if y is Infinity or x is zero.
} else if ( !y.c || x.c && !x.c[0] ) {
return new BigNumber(x);
}
if ( MODULO_MODE == 9 ) {
// Euclidian division: q = sign(y) * floor(x / abs(y))
// r = x - qy where 0 <= r < abs(y)
s = y.s;
y.s = 1;
q = div( x, y, 0, 3 );
y.s = s;
q.s *= s;
} else {
q = div( x, y, 0, MODULO_MODE );
}
return x.minus( q.times(y) );
};
/*
* Return a new BigNumber whose value is the value of this BigNumber negated,
* i.e. multiplied by -1.
*/
P.negated = P.neg = function () {
var x = new BigNumber(this);
x.s = -x.s || null;
return x;
};
/*
* n + 0 = n
* n + N = N
* n + I = I
* 0 + n = n
* 0 + 0 = 0
* 0 + N = N
* 0 + I = I
* N + n = N
* N + 0 = N
* N + N = N
* N + I = N
* I + n = I
* I + 0 = I
* I + N = N
* I + I = I
*
* Return a new BigNumber whose value is the value of this BigNumber plus the value of
* BigNumber(y, b).
*/
P.plus = P.add = function ( y, b ) {
var t,
x = this,
a = x.s;
id = 12;
y = new BigNumber( y, b );
b = y.s;
// Either NaN?
if ( !a || !b ) return new BigNumber(NaN);
// Signs differ?
if ( a != b ) {
y.s = -b;
return x.minus(y);
}
var xe = x.e / LOG_BASE,
ye = y.e / LOG_BASE,
xc = x.c,
yc = y.c;
if ( !xe || !ye ) {
// Return ±Infinity if either ±Infinity.
if ( !xc || !yc ) return new BigNumber( a / 0 );
// Either zero?
// Return y if y is non-zero, x if x is non-zero, or zero if both are zero.
if ( !xc[0] || !yc[0] ) return yc[0] ? y : new BigNumber( xc[0] ? x : a * 0 );
}
xe = bitFloor(xe);
ye = bitFloor(ye);
xc = xc.slice();
// Prepend zeros to equalise exponents. Faster to use reverse then do unshifts.
if ( a = xe - ye ) {
if ( a > 0 ) {
ye = xe;
t = yc;
} else {
a = -a;
t = xc;
}
t.reverse();
for ( ; a--; t.push(0) );
t.reverse();
}
a = xc.length;
b = yc.length;
// Point xc to the longer array, and b to the shorter length.
if ( a - b < 0 ) t = yc, yc = xc, xc = t, b = a;
// Only start adding at yc.length - 1 as the further digits of xc can be ignored.
for ( a = 0; b; ) {
a = ( xc[--b] = xc[b] + yc[b] + a ) / BASE | 0;
xc[b] = BASE === xc[b] ? 0 : xc[b] % BASE;
}
if (a) {
xc.unshift(a);
++ye;
}
// No need to check for zero, as +x + +y != 0 && -x + -y != 0
// ye = MAX_EXP + 1 possible
return normalise( y, xc, ye );
};
/*
* Return the number of significant digits of the value of this BigNumber.
*
* [z] {boolean|number} Whether to count integer-part trailing zeros: true, false, 1 or 0.
*/
P.precision = P.sd = function (z) {
var n, v,
x = this,
c = x.c;
// 'precision() argument not a boolean or binary digit: {z}'
if ( z != null && z !== !!z && z !== 1 && z !== 0 ) {
if (ERRORS) raise( 13, 'argument' + notBool, z );
if ( z != !!z ) z = null;
}
if ( !c ) return null;
v = c.length - 1;
n = v * LOG_BASE + 1;
if ( v = c[v] ) {
// Subtract the number of trailing zeros of the last element.
for ( ; v % 10 == 0; v /= 10, n-- );
// Add the number of digits of the first element.
for ( v = c[0]; v >= 10; v /= 10, n++ );
}
if ( z && x.e + 1 > n ) n = x.e + 1;
return n;
};
/*
* Return a new BigNumber whose value is the value of this BigNumber rounded to a maximum of
* dp decimal places using rounding mode rm, or to 0 and ROUNDING_MODE respectively if
* omitted.
*
* [dp] {number} Decimal places. Integer, 0 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'round() decimal places out of range: {dp}'
* 'round() decimal places not an integer: {dp}'
* 'round() rounding mode not an integer: {rm}'
* 'round() rounding mode out of range: {rm}'
*/
P.round = function ( dp, rm ) {
var n = new BigNumber(this);
if ( dp == null || isValidInt( dp, 0, MAX, 15 ) ) {
round( n, ~~dp + this.e + 1, rm == null ||
!isValidInt( rm, 0, 8, 15, roundingMode ) ? ROUNDING_MODE : rm | 0 );
}
return n;
};
/*
* Return a new BigNumber whose value is the value of this BigNumber shifted by k places
* (powers of 10). Shift to the right if n > 0, and to the left if n < 0.
*
* k {number} Integer, -MAX_SAFE_INTEGER to MAX_SAFE_INTEGER inclusive.
*
* If k is out of range and ERRORS is false, the result will be ±0 if k < 0, or ±Infinity
* otherwise.
*
* 'shift() argument not an integer: {k}'
* 'shift() argument out of range: {k}'
*/
P.shift = function (k) {
var n = this;
return isValidInt( k, -MAX_SAFE_INTEGER, MAX_SAFE_INTEGER, 16, 'argument' )
// k < 1e+21, or truncate(k) will produce exponential notation.
? n.times( '1e' + truncate(k) )
: new BigNumber( n.c && n.c[0] && ( k < -MAX_SAFE_INTEGER || k > MAX_SAFE_INTEGER )
? n.s * ( k < 0 ? 0 : 1 / 0 )
: n );
};
/*
* sqrt(-n) = N
* sqrt( N) = N
* sqrt(-I) = N
* sqrt( I) = I
* sqrt( 0) = 0
* sqrt(-0) = -0
*
* Return a new BigNumber whose value is the square root of the value of this BigNumber,
* rounded according to DECIMAL_PLACES and ROUNDING_MODE.
*/
P.squareRoot = P.sqrt = function () {
var m, n, r, rep, t,
x = this,
c = x.c,
s = x.s,
e = x.e,
dp = DECIMAL_PLACES + 4,
half = new BigNumber('0.5');
// Negative/NaN/Infinity/zero?
if ( s !== 1 || !c || !c[0] ) {
return new BigNumber( !s || s < 0 && ( !c || c[0] ) ? NaN : c ? x : 1 / 0 );
}
// Initial estimate.
s = Math.sqrt( +x );
// Math.sqrt underflow/overflow?
// Pass x to Math.sqrt as integer, then adjust the exponent of the result.
if ( s == 0 || s == 1 / 0 ) {
n = coeffToString(c);
if ( ( n.length + e ) % 2 == 0 ) n += '0';
s = Math.sqrt(n);
e = bitFloor( ( e + 1 ) / 2 ) - ( e < 0 || e % 2 );
if ( s == 1 / 0 ) {
n = '1e' + e;
} else {
n = s.toExponential();
n = n.slice( 0, n.indexOf('e') + 1 ) + e;
}
r = new BigNumber(n);
} else {
r = new BigNumber( s + '' );
}
// Check for zero.
// r could be zero if MIN_EXP is changed after the this value was created.
// This would cause a division by zero (x/t) and hence Infinity below, which would cause
// coeffToString to throw.
if ( r.c[0] ) {
e = r.e;
s = e + dp;
if ( s < 3 ) s = 0;
// Newton-Raphson iteration.
for ( ; ; ) {
t = r;
r = half.times( t.plus( div( x, t, dp, 1 ) ) );
if ( coeffToString( t.c ).slice( 0, s ) === ( n =
coeffToString( r.c ) ).slice( 0, s ) ) {
// The exponent of r may here be one less than the final result exponent,
// e.g 0.0009999 (e-4) --> 0.001 (e-3), so adjust s so the rounding digits
// are indexed correctly.
if ( r.e < e ) --s;
n = n.slice( s - 3, s + 1 );
// The 4th rounding digit may be in error by -1 so if the 4 rounding digits
// are 9999 or 4999 (i.e. approaching a rounding boundary) continue the
// iteration.
if ( n == '9999' || !rep && n == '4999' ) {
// On the first iteration only, check to see if rounding up gives the
// exact result as the nines may infinitely repeat.
if ( !rep ) {
round( t, t.e + DECIMAL_PLACES + 2, 0 );
if ( t.times(t).eq(x) ) {
r = t;
break;
}
}
dp += 4;
s += 4;
rep = 1;
} else {
// If rounding digits are null, 0{0,4} or 50{0,3}, check for exact
// result. If not, then there are further digits and m will be truthy.
if ( !+n || !+n.slice(1) && n.charAt(0) == '5' ) {
// Truncate to the first rounding digit.
round( r, r.e + DECIMAL_PLACES + 2, 1 );
m = !r.times(r).eq(x);
}
break;
}
}
}
}
return round( r, r.e + DECIMAL_PLACES + 1, ROUNDING_MODE, m );
};
/*
* n * 0 = 0
* n * N = N
* n * I = I
* 0 * n = 0
* 0 * 0 = 0
* 0 * N = N
* 0 * I = N
* N * n = N
* N * 0 = N
* N * N = N
* N * I = N
* I * n = I
* I * 0 = N
* I * N = N
* I * I = I
*
* Return a new BigNumber whose value is the value of this BigNumber times the value of
* BigNumber(y, b).
*/
P.times = P.mul = function ( y, b ) {
var c, e, i, j, k, m, xcL, xlo, xhi, ycL, ylo, yhi, zc,
base, sqrtBase,
x = this,
xc = x.c,
yc = ( id = 17, y = new BigNumber( y, b ) ).c;
// Either NaN, ±Infinity or ±0?
if ( !xc || !yc || !xc[0] || !yc[0] ) {
// Return NaN if either is NaN, or one is 0 and the other is Infinity.
if ( !x.s || !y.s || xc && !xc[0] && !yc || yc && !yc[0] && !xc ) {
y.c = y.e = y.s = null;
} else {
y.s *= x.s;
// Return ±Infinity if either is ±Infinity.
if ( !xc || !yc ) {
y.c = y.e = null;
// Return ±0 if either is ±0.
} else {
y.c = [0];
y.e = 0;
}
}
return y;
}
e = bitFloor( x.e / LOG_BASE ) + bitFloor( y.e / LOG_BASE );
y.s *= x.s;
xcL = xc.length;
ycL = yc.length;
// Ensure xc points to longer array and xcL to its length.
if ( xcL < ycL ) zc = xc, xc = yc, yc = zc, i = xcL, xcL = ycL, ycL = i;
// Initialise the result array with zeros.
for ( i = xcL + ycL, zc = []; i--; zc.push(0) );
base = BASE;
sqrtBase = SQRT_BASE;
for ( i = ycL; --i >= 0; ) {
c = 0;
ylo = yc[i] % sqrtBase;
yhi = yc[i] / sqrtBase | 0;
for ( k = xcL, j = i + k; j > i; ) {
xlo = xc[--k] % sqrtBase;
xhi = xc[k] / sqrtBase | 0;
m = yhi * xlo + xhi * ylo;
xlo = ylo * xlo + ( ( m % sqrtBase ) * sqrtBase ) + zc[j] + c;
c = ( xlo / base | 0 ) + ( m / sqrtBase | 0 ) + yhi * xhi;
zc[j--] = xlo % base;
}
zc[j] = c;
}
if (c) {
++e;
} else {
zc.shift();
}
return normalise( y, zc, e );
};
/*
* Return a new BigNumber whose value is the value of this BigNumber rounded to a maximum of
* sd significant digits using rounding mode rm, or ROUNDING_MODE if rm is omitted.
*
* [sd] {number} Significant digits. Integer, 1 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'toDigits() precision out of range: {sd}'
* 'toDigits() precision not an integer: {sd}'
* 'toDigits() rounding mode not an integer: {rm}'
* 'toDigits() rounding mode out of range: {rm}'
*/
P.toDigits = function ( sd, rm ) {
var n = new BigNumber(this);
sd = sd == null || !isValidInt( sd, 1, MAX, 18, 'precision' ) ? null : sd | 0;
rm = rm == null || !isValidInt( rm, 0, 8, 18, roundingMode ) ? ROUNDING_MODE : rm | 0;
return sd ? round( n, sd, rm ) : n;
};
/*
* Return a string representing the value of this BigNumber in exponential notation and
* rounded using ROUNDING_MODE to dp fixed decimal places.
*
* [dp] {number} Decimal places. Integer, 0 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'toExponential() decimal places not an integer: {dp}'
* 'toExponential() decimal places out of range: {dp}'
* 'toExponential() rounding mode not an integer: {rm}'
* 'toExponential() rounding mode out of range: {rm}'
*/
P.toExponential = function ( dp, rm ) {
return format( this,
dp != null && isValidInt( dp, 0, MAX, 19 ) ? ~~dp + 1 : null, rm, 19 );
};
/*
* Return a string representing the value of this BigNumber in fixed-point notation rounding
* to dp fixed decimal places using rounding mode rm, or ROUNDING_MODE if rm is omitted.
*
* Note: as with JavaScript's number type, (-0).toFixed(0) is '0',
* but e.g. (-0.00001).toFixed(0) is '-0'.
*
* [dp] {number} Decimal places. Integer, 0 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'toFixed() decimal places not an integer: {dp}'
* 'toFixed() decimal places out of range: {dp}'
* 'toFixed() rounding mode not an integer: {rm}'
* 'toFixed() rounding mode out of range: {rm}'
*/
P.toFixed = function ( dp, rm ) {
return format( this, dp != null && isValidInt( dp, 0, MAX, 20 )
? ~~dp + this.e + 1 : null, rm, 20 );
};
/*
* Return a string representing the value of this BigNumber in fixed-point notation rounded
* using rm or ROUNDING_MODE to dp decimal places, and formatted according to the properties
* of the FORMAT object (see BigNumber.config).
*
* FORMAT = {
* decimalSeparator : '.',
* groupSeparator : ',',
* groupSize : 3,
* secondaryGroupSize : 0,
* fractionGroupSeparator : '\xA0', // non-breaking space
* fractionGroupSize : 0
* };
*
* [dp] {number} Decimal places. Integer, 0 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'toFormat() decimal places not an integer: {dp}'
* 'toFormat() decimal places out of range: {dp}'
* 'toFormat() rounding mode not an integer: {rm}'
* 'toFormat() rounding mode out of range: {rm}'
*/
P.toFormat = function ( dp, rm ) {
var str = format( this, dp != null && isValidInt( dp, 0, MAX, 21 )
? ~~dp + this.e + 1 : null, rm, 21 );
if ( this.c ) {
var i,
arr = str.split('.'),
g1 = +FORMAT.groupSize,
g2 = +FORMAT.secondaryGroupSize,
groupSeparator = FORMAT.groupSeparator,
intPart = arr[0],
fractionPart = arr[1],
isNeg = this.s < 0,
intDigits = isNeg ? intPart.slice(1) : intPart,
len = intDigits.length;
if (g2) i = g1, g1 = g2, g2 = i, len -= i;
if ( g1 > 0 && len > 0 ) {
i = len % g1 || g1;
intPart = intDigits.substr( 0, i );
for ( ; i < len; i += g1 ) {
intPart += groupSeparator + intDigits.substr( i, g1 );
}
if ( g2 > 0 ) intPart += groupSeparator + intDigits.slice(i);
if (isNeg) intPart = '-' + intPart;
}
str = fractionPart
? intPart + FORMAT.decimalSeparator + ( ( g2 = +FORMAT.fractionGroupSize )
? fractionPart.replace( new RegExp( '\\d{' + g2 + '}\\B', 'g' ),
'$&' + FORMAT.fractionGroupSeparator )
: fractionPart )
: intPart;
}
return str;
};
/*
* Return a string array representing the value of this BigNumber as a simple fraction with
* an integer numerator and an integer denominator. The denominator will be a positive
* non-zero value less than or equal to the specified maximum denominator. If a maximum
* denominator is not specified, the denominator will be the lowest value necessary to
* represent the number exactly.
*
* [md] {number|string|BigNumber} Integer >= 1 and < Infinity. The maximum denominator.
*
* 'toFraction() max denominator not an integer: {md}'
* 'toFraction() max denominator out of range: {md}'
*/
P.toFraction = function (md) {
var arr, d0, d2, e, exp, n, n0, q, s,
k = ERRORS,
x = this,
xc = x.c,
d = new BigNumber(ONE),
n1 = d0 = new BigNumber(ONE),
d1 = n0 = new BigNumber(ONE);
if ( md != null ) {
ERRORS = false;
n = new BigNumber(md);
ERRORS = k;
if ( !( k = n.isInt() ) || n.lt(ONE) ) {
if (ERRORS) {
raise( 22,
'max denominator ' + ( k ? 'out of range' : 'not an integer' ), md );
}
// ERRORS is false:
// If md is a finite non-integer >= 1, round it to an integer and use it.
md = !k && n.c && round( n, n.e + 1, 1 ).gte(ONE) ? n : null;
}
}
if ( !xc ) return x.toString();
s = coeffToString(xc);
// Determine initial denominator.
// d is a power of 10 and the minimum max denominator that specifies the value exactly.
e = d.e = s.length - x.e - 1;
d.c[0] = POWS_TEN[ ( exp = e % LOG_BASE ) < 0 ? LOG_BASE + exp : exp ];
md = !md || n.cmp(d) > 0 ? ( e > 0 ? d : n1 ) : n;
exp = MAX_EXP;
MAX_EXP = 1 / 0;
n = new BigNumber(s);
// n0 = d1 = 0
n0.c[0] = 0;
for ( ; ; ) {
q = div( n, d, 0, 1 );
d2 = d0.plus( q.times(d1) );
if ( d2.cmp(md) == 1 ) break;
d0 = d1;
d1 = d2;
n1 = n0.plus( q.times( d2 = n1 ) );
n0 = d2;
d = n.minus( q.times( d2 = d ) );
n = d2;
}
d2 = div( md.minus(d0), d1, 0, 1 );
n0 = n0.plus( d2.times(n1) );
d0 = d0.plus( d2.times(d1) );
n0.s = n1.s = x.s;
e *= 2;
// Determine which fraction is closer to x, n0/d0 or n1/d1
arr = div( n1, d1, e, ROUNDING_MODE ).minus(x).abs().cmp(
div( n0, d0, e, ROUNDING_MODE ).minus(x).abs() ) < 1
? [ n1.toString(), d1.toString() ]
: [ n0.toString(), d0.toString() ];
MAX_EXP = exp;
return arr;
};
/*
* Return the value of this BigNumber converted to a number primitive.
*/
P.toNumber = function () {
return +this;
};
/*
* Return a BigNumber whose value is the value of this BigNumber raised to the power n.
* If m is present, return the result modulo m.
* If n is negative round according to DECIMAL_PLACES and ROUNDING_MODE.
* If POW_PRECISION is non-zero and m is not present, round to POW_PRECISION using
* ROUNDING_MODE.
*
* The modular power operation works efficiently when x, n, and m are positive integers,
* otherwise it is equivalent to calculating x.toPower(n).modulo(m) (with POW_PRECISION 0).
*
* n {number} Integer, -MAX_SAFE_INTEGER to MAX_SAFE_INTEGER inclusive.
* [m] {number|string|BigNumber} The modulus.
*
* 'pow() exponent not an integer: {n}'
* 'pow() exponent out of range: {n}'
*
* Performs 54 loop iterations for n of 9007199254740991.
*/
P.toPower = P.pow = function ( n, m ) {
var k, y, z,
i = mathfloor( n < 0 ? -n : +n ),
x = this;
if ( m != null ) {
id = 23;
m = new BigNumber(m);
}
// Pass ±Infinity to Math.pow if exponent is out of range.
if ( !isValidInt( n, -MAX_SAFE_INTEGER, MAX_SAFE_INTEGER, 23, 'exponent' ) &&
( !isFinite(n) || i > MAX_SAFE_INTEGER && ( n /= 0 ) ||
parseFloat(n) != n && !( n = NaN ) ) || n == 0 ) {
k = Math.pow( +x, n );
return new BigNumber( m ? k % m : k );
}
if (m) {
if ( n > 1 && x.gt(ONE) && x.isInt() && m.gt(ONE) && m.isInt() ) {
x = x.mod(m);
} else {
z = m;
// Nullify m so only a single mod operation is performed at the end.
m = null;
}
} else if (POW_PRECISION) {
// Truncating each coefficient array to a length of k after each multiplication
// equates to truncating significant digits to POW_PRECISION + [28, 41],
// i.e. there will be a minimum of 28 guard digits retained.
// (Using + 1.5 would give [9, 21] guard digits.)
k = mathceil( POW_PRECISION / LOG_BASE + 2 );
}
y = new BigNumber(ONE);
for ( ; ; ) {
if ( i % 2 ) {
y = y.times(x);
if ( !y.c ) break;
if (k) {
if ( y.c.length > k ) y.c.length = k;
} else if (m) {
y = y.mod(m);
}
}
i = mathfloor( i / 2 );
if ( !i ) break;
x = x.times(x);
if (k) {
if ( x.c && x.c.length > k ) x.c.length = k;
} else if (m) {
x = x.mod(m);
}
}
if (m) return y;
if ( n < 0 ) y = ONE.div(y);
return z ? y.mod(z) : k ? round( y, POW_PRECISION, ROUNDING_MODE ) : y;
};
/*
* Return a string representing the value of this BigNumber rounded to sd significant digits
* using rounding mode rm or ROUNDING_MODE. If sd is less than the number of digits
* necessary to represent the integer part of the value in fixed-point notation, then use
* exponential notation.
*
* [sd] {number} Significant digits. Integer, 1 to MAX inclusive.
* [rm] {number} Rounding mode. Integer, 0 to 8 inclusive.
*
* 'toPrecision() precision not an integer: {sd}'
* 'toPrecision() precision out of range: {sd}'
* 'toPrecision() rounding mode not an integer: {rm}'
* 'toPrecision() rounding mode out of range: {rm}'
*/
P.toPrecision = function ( sd, rm ) {
return format( this, sd != null && isValidInt( sd, 1, MAX, 24, 'precision' )
? sd | 0 : null, rm, 24 );
};
/*
* Return a string representing the value of this BigNumber in base b, or base 10 if b is
* omitted. If a base is specified, including base 10, round according to DECIMAL_PLACES and
* ROUNDING_MODE. If a base is not specified, and this BigNumber has a positive exponent
* that is equal to or greater than TO_EXP_POS, or a negative exponent equal to or less than
* TO_EXP_NEG, return exponential notation.
*
* [b] {number} Integer, 2 to 64 inclusive.
*
* 'toString() base not an integer: {b}'
* 'toString() base out of range: {b}'
*/
P.toString = function (b) {
var str,
n = this,
s = n.s,
e = n.e;
// Infinity or NaN?
if ( e === null ) {
if (s) {
str = 'Infinity';
if ( s < 0 ) str = '-' + str;
} else {
str = 'NaN';
}
} else {
str = coeffToString( n.c );
if ( b == null || !isValidInt( b, 2, 64, 25, 'base' ) ) {
str = e <= TO_EXP_NEG || e >= TO_EXP_POS
? toExponential( str, e )
: toFixedPoint( str, e );
} else {
str = convertBase( toFixedPoint( str, e ), b | 0, 10, s );
}
if ( s < 0 && n.c[0] ) str = '-' + str;
}
return str;
};
/*
* Return a new BigNumber whose value is the value of this BigNumber truncated to a whole
* number.
*/
P.truncated = P.trunc = function () {
return round( new BigNumber(this), this.e + 1, 1 );
};
/*
* Return as toString, but do not accept a base argument, and include the minus sign for
* negative zero.
*/
P.valueOf = P.toJSON = function () {
var str,
n = this,
e = n.e;
if ( e === null ) return n.toString();
str = coeffToString( n.c );
str = e <= TO_EXP_NEG || e >= TO_EXP_POS
? toExponential( str, e )
: toFixedPoint( str, e );
return n.s < 0 ? '-' + str : str;
};
if ( configObj != null ) BigNumber.config(configObj);
return BigNumber;
}
// PRIVATE HELPER FUNCTIONS
function bitFloor(n) {
var i = n | 0;
return n > 0 || n === i ? i : i - 1;
}
// Return a coefficient array as a string of base 10 digits.
function coeffToString(a) {
var s, z,
i = 1,
j = a.length,
r = a[0] + '';
for ( ; i < j; ) {
s = a[i++] + '';
z = LOG_BASE - s.length;
for ( ; z--; s = '0' + s );
r += s;
}
// Determine trailing zeros.
for ( j = r.length; r.charCodeAt(--j) === 48; );
return r.slice( 0, j + 1 || 1 );
}
// Compare the value of BigNumbers x and y.
function compare( x, y ) {
var a, b,
xc = x.c,
yc = y.c,
i = x.s,
j = y.s,
k = x.e,
l = y.e;
// Either NaN?
if ( !i || !j ) return null;
a = xc && !xc[0];
b = yc && !yc[0];
// Either zero?
if ( a || b ) return a ? b ? 0 : -j : i;
// Signs differ?
if ( i != j ) return i;
a = i < 0;
b = k == l;
// Either Infinity?
if ( !xc || !yc ) return b ? 0 : !xc ^ a ? 1 : -1;
// Compare exponents.
if ( !b ) return k > l ^ a ? 1 : -1;
j = ( k = xc.length ) < ( l = yc.length ) ? k : l;
// Compare digit by digit.
for ( i = 0; i < j; i++ ) if ( xc[i] != yc[i] ) return xc[i] > yc[i] ^ a ? 1 : -1;
// Compare lengths.
return k == l ? 0 : k > l ^ a ? 1 : -1;
}
/*
* Return true if n is a valid number in range, otherwise false.
* Use for argument validation when ERRORS is false.
* Note: parseInt('1e+1') == 1 but parseFloat('1e+1') == 10.
*/
function intValidatorNoErrors( n, min, max ) {
return ( n = truncate(n) ) >= min && n <= max;
}
function isArray(obj) {
return Object.prototype.toString.call(obj) == '[object Array]';
}
function isBigNumber(v) {
return !!( v && v.constructor && v.constructor.isBigNumber === isBigNumber );
}
/*
* Convert string of baseIn to an array of numbers of baseOut.
* Eg. convertBase('255', 10, 16) returns [15, 15].
* Eg. convertBase('ff', 16, 10) returns [2, 5, 5].
*/
function toBaseOut( str, baseIn, baseOut ) {
var j,
arr = [0],
arrL,
i = 0,
len = str.length;
for ( ; i < len; ) {
for ( arrL = arr.length; arrL--; arr[arrL] *= baseIn );
arr[ j = 0 ] += ALPHABET.indexOf( str.charAt( i++ ) );
for ( ; j < arr.length; j++ ) {
if ( arr[j] > baseOut - 1 ) {
if ( arr[j + 1] == null ) arr[j + 1] = 0;
arr[j + 1] += arr[j] / baseOut | 0;
arr[j] %= baseOut;
}
}
}
return arr.reverse();
}
function toExponential( str, e ) {
return ( str.length > 1 ? str.charAt(0) + '.' + str.slice(1) : str ) +
( e < 0 ? 'e' : 'e+' ) + e;
}
function toFixedPoint( str, e ) {
var len, z;
// Negative exponent?
if ( e < 0 ) {
// Prepend zeros.
for ( z = '0.'; ++e; z += '0' );
str = z + str;
// Positive exponent
} else {
len = str.length;
// Append zeros.
if ( ++e > len ) {
for ( z = '0', e -= len; --e; z += '0' );
str += z;
} else if ( e < len ) {
str = str.slice( 0, e ) + '.' + str.slice(e);
}
}
return str;
}
function truncate(n) {
n = parseFloat(n);
return n < 0 ? mathceil(n) : mathfloor(n);
}
// EXPORT
BigNumber = constructorFactory();
BigNumber.default = BigNumber.BigNumber = BigNumber;
// AMD.
if ( typeof define == 'function' && define.amd ) {
define( function () { return BigNumber; } );
// Node.js and other environments that support module.exports.
} else if ( typeof module != 'undefined' && module.exports ) {
module.exports = BigNumber;
// Browser.
} else {
if ( !globalObj ) globalObj = typeof self != 'undefined' ? self : Function('return this')();
globalObj.BigNumber = BigNumber;
}
})(this);
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/bignumber.js.map
{"version":3,"file":"bignumber.min.js","sources":["bignumber.js"],"names":["globalObj","constructorFactory","configObj","BigNumber","n","b","c","e","i","num","len","str","x","this","ERRORS","raise","isValidInt","id","round","DECIMAL_PLACES","ROUNDING_MODE","RegExp","ALPHABET","slice","test","parseNumeric","s","replace","length","tooManyDigits","charCodeAt","convertBase","isNumeric","indexOf","search","substring","MAX_SAFE_INTEGER","mathfloor","MAX_EXP","MIN_EXP","LOG_BASE","push","baseOut","baseIn","sign","d","k","r","xc","y","dp","rm","toLowerCase","POW_PRECISION","pow","toBaseOut","toFixedPoint","coeffToString","pop","div","unshift","charAt","format","caller","c0","ne","roundingMode","toString","TO_EXP_NEG","toExponential","maxOrMin","args","method","m","isArray","call","intValidatorWithErrors","min","max","name","truncate","normalise","j","msg","val","error","Error","sd","ni","rd","pows10","POWS_TEN","out","mathceil","BASE","P","prototype","ONE","TO_EXP_POS","CRYPTO","MODULO_MODE","FORMAT","decimalSeparator","groupSeparator","groupSize","secondaryGroupSize","fractionGroupSeparator","fractionGroupSize","another","ROUND_UP","ROUND_DOWN","ROUND_CEIL","ROUND_FLOOR","ROUND_HALF_UP","ROUND_HALF_DOWN","ROUND_HALF_EVEN","ROUND_HALF_CEIL","ROUND_HALF_FLOOR","EUCLID","config","set","v","p","a","arguments","o","has","hasOwnProperty","MAX","intValidatorNoErrors","notBool","crypto","getRandomValues","randomBytes","isBigNumber","lt","gt","random","pow2_53","random53bitInt","Math","rand","Uint32Array","copy","shift","multiply","base","temp","xlo","xhi","carry","klo","SQRT_BASE","khi","compare","aL","bL","cmp","subtract","more","prod","prodL","q","qc","rem","remL","rem0","xi","xL","yc0","yL","yz","yc","NaN","bitFloor","basePrefix","dotAfter","dotBefore","isInfinityOrNaN","whitespaceOrPlus","isNaN","p1","p2","absoluteValue","abs","ceil","comparedTo","decimalPlaces","dividedBy","dividedToIntegerBy","divToInt","equals","eq","floor","greaterThan","greaterThanOrEqualTo","gte","isFinite","isInteger","isInt","isNegative","isNeg","isZero","lessThan","lessThanOrEqualTo","lte","minus","sub","t","xLTy","plus","xe","ye","reverse","modulo","mod","times","negated","neg","add","precision","z","squareRoot","sqrt","rep","half","mul","xcL","ycL","ylo","yhi","zc","sqrtBase","toDigits","toFixed","toFormat","arr","split","g1","g2","intPart","fractionPart","intDigits","substr","toFraction","md","d0","d2","exp","n0","n1","d1","toNumber","toPower","parseFloat","toPrecision","truncated","trunc","valueOf","toJSON","l","obj","Object","constructor","arrL","define","amd","module","exports","self","Function"],"mappings":";CAEC,SAAWA,GACR,YAqCA,SAASC,GAAmBC,GAiHxB,QAASC,GAAWC,EAAGC,GACnB,GAAIC,GAAGC,EAAGC,EAAGC,EAAKC,EAAKC,EACnBC,EAAIC,IAGR,MAAQD,YAAaT,IAIjB,MADIW,IAAQC,EAAO,GAAI,+BAAgCX,GAChD,GAAID,GAAWC,EAAGC,EAK7B,IAAU,MAALA,GAAcW,EAAYX,EAAG,EAAG,GAAIY,EAAI,QA4BtC,CAMH,GALAZ,EAAQ,EAAJA,EACJM,EAAMP,EAAI,GAIA,IAALC,EAED,MADAO,GAAI,GAAIT,GAAWC,YAAaD,GAAYC,EAAIO,GACzCO,EAAON,EAAGO,EAAiBP,EAAEL,EAAI,EAAGa,EAK/C,KAAOX,EAAkB,gBAALL,KAAuB,EAAJA,GAAS,IAC7C,GAAMiB,QAAQ,OAAUf,EAAI,IAAMgB,EAASC,MAAO,EAAGlB,GAAM,MAC1D,SAAWC,EAAI,MAAU,GAAJD,EAAS,IAAM,IAAOmB,KAAKb,GAChD,MAAOc,GAAcb,EAAGD,EAAKF,EAAKJ,EAGlCI,IACAG,EAAEc,EAAY,EAAR,EAAItB,GAAUO,EAAMA,EAAIY,MAAM,GAAI,IAAO,EAE1CT,GAAUH,EAAIgB,QAAS,YAAa,IAAKC,OAAS,IAGnDb,EAAOE,EAAIY,EAAezB,GAI9BK,GAAM,GAENG,EAAEc,EAA0B,KAAtBf,EAAImB,WAAW,IAAcnB,EAAMA,EAAIY,MAAM,GAAI,IAAO,EAGlEZ,EAAMoB,EAAapB,EAAK,GAAIN,EAAGO,EAAEc,OA9DmB,CAGpD,GAAKtB,YAAaD,GAKd,MAJAS,GAAEc,EAAItB,EAAEsB,EACRd,EAAEL,EAAIH,EAAEG,EACRK,EAAEN,GAAMF,EAAIA,EAAEE,GAAMF,EAAEmB,QAAUnB,OAChCa,EAAK,EAIT,KAAOR,EAAkB,gBAALL,KAAuB,EAAJA,GAAS,EAAI,CAIhD,GAHAQ,EAAEc,EAAY,EAAR,EAAItB,GAAUA,GAAKA,EAAG,IAAO,EAG9BA,MAAQA,EAAI,CACb,IAAMG,EAAI,EAAGC,EAAIJ,EAAGI,GAAK,GAAIA,GAAK,GAAID,KAItC,MAHAK,GAAEL,EAAIA,EACNK,EAAEN,GAAKF,QACPa,EAAK,GAITN,EAAMP,EAAI,OACP,CACH,IAAM4B,EAAUR,KAAMb,EAAMP,EAAI,IAAO,MAAOqB,GAAcb,EAAGD,EAAKF,EACpEG,GAAEc,EAA0B,KAAtBf,EAAImB,WAAW,IAAcnB,EAAMA,EAAIY,MAAM,GAAI,IAAO,GAwDtE,KAhBOhB,EAAII,EAAIsB,QAAQ,MAAS,KAAKtB,EAAMA,EAAIgB,QAAS,IAAK,MAGtDnB,EAAIG,EAAIuB,OAAQ,OAAW,GAGrB,EAAJ3B,IAAQA,EAAIC,GACjBD,IAAMI,EAAIY,MAAOf,EAAI,GACrBG,EAAMA,EAAIwB,UAAW,EAAG3B,IACZ,EAAJD,IAGRA,EAAII,EAAIiB,QAINpB,EAAI,EAAyB,KAAtBG,EAAImB,WAAWtB,GAAWA,KAGvC,IAAME,EAAMC,EAAIiB,OAAkC,KAA1BjB,EAAImB,aAAapB,KAGzC,GAFAC,EAAMA,EAAIY,MAAOf,EAAGE,EAAM,GActB,GAXAA,EAAMC,EAAIiB,OAILnB,GAAOK,GAAUJ,EAAM,KAAQN,EAAIgC,GAAoBhC,IAAMiC,EAAUjC,KACxEW,EAAOE,EAAIY,EAAejB,EAAEc,EAAItB,GAGpCG,EAAIA,EAAIC,EAAI,EAGPD,EAAI+B,EAGL1B,EAAEN,EAAIM,EAAEL,EAAI,SAGT,IAASgC,EAAJhC,EAGRK,EAAEN,GAAMM,EAAEL,EAAI,OACX,CAWH,GAVAK,EAAEL,EAAIA,EACNK,EAAEN,KAMFE,GAAMD,EAAI,GAAMiC,EACP,EAAJjC,IAAQC,GAAKgC,GAET9B,EAAJF,EAAU,CAGX,IAFIA,GAAGI,EAAEN,EAAEmC,MAAO9B,EAAIY,MAAO,EAAGf,IAE1BE,GAAO8B,EAAc9B,EAAJF,GACnBI,EAAEN,EAAEmC,MAAO9B,EAAIY,MAAOf,EAAGA,GAAKgC,GAGlC7B,GAAMA,EAAIY,MAAMf,GAChBA,EAAIgC,EAAW7B,EAAIiB,WAEnBpB,IAAKE,CAGT,MAAQF,IAAKG,GAAO,KACpBC,EAAEN,EAAEmC,MAAO9B,OAKfC,GAAEN,GAAMM,EAAEL,EAAI,EAGlBU,GAAK,EAmWT,QAASc,GAAapB,EAAK+B,EAASC,EAAQC,GACxC,GAAIC,GAAGtC,EAAGuC,EAAGC,EAAGnC,EAAGoC,EAAIC,EACnBzC,EAAIG,EAAIsB,QAAS,KACjBiB,EAAK/B,EACLgC,EAAK/B,CA0BT,KAxBc,GAATuB,IAAchC,EAAMA,EAAIyC,eAGxB5C,GAAK,IACNsC,EAAIO,EAGJA,EAAgB,EAChB1C,EAAMA,EAAIgB,QAAS,IAAK,IACxBsB,EAAI,GAAI9C,GAAUwC,GAClB/B,EAAIqC,EAAEK,IAAK3C,EAAIiB,OAASpB,GACxB6C,EAAgBP,EAIhBG,EAAE3C,EAAIiD,EAAWC,EAAcC,EAAe7C,EAAEN,GAAKM,EAAEL,GAAK,GAAImC,GAChEO,EAAE1C,EAAI0C,EAAE3C,EAAEsB,QAIdoB,EAAKO,EAAW5C,EAAKgC,EAAQD,GAC7BnC,EAAIuC,EAAIE,EAAGpB,OAGQ,GAAXoB,IAAKF,GAASE,EAAGU,OACzB,IAAMV,EAAG,GAAK,MAAO,GA2BrB,IAzBS,EAAJxC,IACCD,GAEFK,EAAEN,EAAI0C,EACNpC,EAAEL,EAAIA,EAGNK,EAAEc,EAAIkB,EACNhC,EAAI+C,EAAK/C,EAAGqC,EAAGC,EAAIC,EAAIT,GACvBM,EAAKpC,EAAEN,EACPyC,EAAInC,EAAEmC,EACNxC,EAAIK,EAAEL,GAGVsC,EAAItC,EAAI2C,EAAK,EAGb1C,EAAIwC,EAAGH,GACPC,EAAIJ,EAAU,EACdK,EAAIA,GAAS,EAAJF,GAAsB,MAAbG,EAAGH,EAAI,GAEzBE,EAAS,EAALI,GAAgB,MAAL3C,GAAauC,KAAe,GAANI,GAAWA,IAAQvC,EAAEc,EAAI,EAAI,EAAI,IACzDlB,EAAIsC,GAAKtC,GAAKsC,IAAY,GAANK,GAAWJ,GAAW,GAANI,GAAuB,EAAZH,EAAGH,EAAI,IACtDM,IAAQvC,EAAEc,EAAI,EAAI,EAAI,IAE1B,EAAJmB,IAAUG,EAAG,GAGdrC,EAAMoC,EAAIS,EAAc,KAAMN,GAAO,QAClC,CAGH,GAFAF,EAAGpB,OAASiB,EAERE,EAGA,MAAQL,IAAWM,IAAKH,GAAKH,GACzBM,EAAGH,GAAK,EAEFA,MACAtC,EACFyC,EAAGY,QAAQ,GAMvB,KAAMd,EAAIE,EAAGpB,QAASoB,IAAKF,KAG3B,IAAMtC,EAAI,EAAGG,EAAM,GAASmC,GAALtC,EAAQG,GAAOW,EAASuC,OAAQb,EAAGxC,OAC1DG,EAAM6C,EAAc7C,EAAKJ,GAI7B,MAAOI,GA4QX,QAASmD,GAAQ1D,EAAGI,EAAG2C,EAAIY,GACvB,GAAIC,GAAIzD,EAAG0D,EAAIvD,EAAKC,CAKpB,IAHAwC,EAAW,MAANA,GAAcnC,EAAYmC,EAAI,EAAG,EAAGY,EAAQG,GACxC,EAALf,EAAS/B,GAEPhB,EAAEE,EAAI,MAAOF,GAAE+D,UAIrB,IAHAH,EAAK5D,EAAEE,EAAE,GACT2D,EAAK7D,EAAEG,EAEG,MAALC,EACDG,EAAM8C,EAAerD,EAAEE,GACvBK,EAAgB,IAAVoD,GAA0B,IAAVA,GAAsBK,GAANH,EAClCI,EAAe1D,EAAKsD,GACpBT,EAAc7C,EAAKsD,OAevB,IAbA7D,EAAIc,EAAO,GAAIf,GAAUC,GAAII,EAAG2C,GAGhC5C,EAAIH,EAAEG,EAENI,EAAM8C,EAAerD,EAAEE,GACvBI,EAAMC,EAAIiB,OAOK,IAAVmC,GAA0B,IAAVA,IAAuBxD,GAALC,GAAe4D,GAAL7D,GAAoB,CAGjE,KAAcC,EAANE,EAASC,GAAO,IAAKD,KAC7BC,EAAM0D,EAAe1D,EAAKJ,OAQ1B,IAJAC,GAAKyD,EACLtD,EAAM6C,EAAc7C,EAAKJ,GAGpBA,EAAI,EAAIG,GACT,KAAOF,EAAI,EAAI,IAAMG,GAAO,IAAKH,IAAKG,GAAO,UAG7C,IADAH,GAAKD,EAAIG,EACJF,EAAI,EAEL,IADKD,EAAI,GAAKG,IAAMC,GAAO,KACnBH,IAAKG,GAAO,KAMpC,MAAOP,GAAEsB,EAAI,GAAKsC,EAAK,IAAMrD,EAAMA,EAKvC,QAAS2D,GAAUC,EAAMC,GACrB,GAAIC,GAAGrE,EACHI,EAAI,CAKR,KAHKkE,EAASH,EAAK,MAAOA,EAAOA,EAAK,IACtCE,EAAI,GAAItE,GAAWoE,EAAK,MAEd/D,EAAI+D,EAAK3C,QAAU,CAIzB,GAHAxB,EAAI,GAAID,GAAWoE,EAAK/D,KAGlBJ,EAAEsB,EAAI,CACR+C,EAAIrE,CACJ,OACQoE,EAAOG,KAAMF,EAAGrE,KACxBqE,EAAIrE,GAIZ,MAAOqE,GAQX,QAASG,GAAwBxE,EAAGyE,EAAKC,EAAKf,EAAQgB,GAMlD,OALSF,EAAJzE,GAAWA,EAAI0E,GAAO1E,GAAK4E,EAAS5E,KACrCW,EAAOgD,GAAUgB,GAAQ,mBACjBF,EAAJzE,GAAWA,EAAI0E,EAAM,gBAAkB,mBAAqB1E,IAG7D,EAQX,QAAS6E,GAAW7E,EAAGE,EAAGC,GAKtB,IAJA,GAAIC,GAAI,EACJ0E,EAAI5E,EAAEsB,QAGDtB,IAAI4E,GAAI5E,EAAEoD,OAGnB,IAAMwB,EAAI5E,EAAE,GAAI4E,GAAK,GAAIA,GAAK,GAAI1E,KAkBlC,OAfOD,EAAIC,EAAID,EAAIiC,EAAW,GAAMF,EAGhClC,EAAEE,EAAIF,EAAEG,EAAI,KAGAgC,EAAJhC,EAGRH,EAAEE,GAAMF,EAAEG,EAAI,IAEdH,EAAEG,EAAIA,EACNH,EAAEE,EAAIA,GAGHF,EAmDX,QAASW,GAAOgD,EAAQoB,EAAKC,GACzB,GAAIC,GAAQ,GAAIC,QACZ,gBACA,MACA,SACA,MACA,WACA,KACA,KACA,MACA,KACA,MACA,QACA,MACA,OACA,YACA,SACA,QACA,QACA,QACA,WACA,gBACA,UACA,WACA,aACA,MACA,cACA,WACA,aACFvB,GAAU,MAAQoB,EAAM,KAAOC,EAIjC,MAFAC,GAAMN,KAAO,kBACb9D,EAAK,EACCoE,EAQV,QAASnE,GAAON,EAAG2E,EAAIpC,EAAIJ,GACvB,GAAIF,GAAGrC,EAAG0E,EAAGpC,EAAG1C,EAAGoF,EAAIC,EACnBzC,EAAKpC,EAAEN,EACPoF,EAASC,CAGb,IAAI3C,EAAI,CAQJ4C,EAAK,CAGD,IAAM/C,EAAI,EAAGC,EAAIE,EAAG,GAAIF,GAAK,GAAIA,GAAK,GAAID,KAI1C,GAHArC,EAAI+E,EAAK1C,EAGA,EAAJrC,EACDA,GAAKgC,EACL0C,EAAIK,EACJnF,EAAI4C,EAAIwC,EAAK,GAGbC,EAAKrF,EAAIsF,EAAQ7C,EAAIqC,EAAI,GAAM,GAAK,MAIpC,IAFAM,EAAKK,GAAYrF,EAAI,GAAMgC,GAEtBgD,GAAMxC,EAAGpB,OAAS,CAEnB,IAAImB,EASA,KAAM6C,EANN,MAAQ5C,EAAGpB,QAAU4D,EAAIxC,EAAGP,KAAK,IACjCrC,EAAIqF,EAAK,EACT5C,EAAI,EACJrC,GAAKgC,EACL0C,EAAI1E,EAAIgC,EAAW,MAIpB,CAIH,IAHApC,EAAI0C,EAAIE,EAAGwC,GAGL3C,EAAI,EAAGC,GAAK,GAAIA,GAAK,GAAID,KAG/BrC,GAAKgC,EAIL0C,EAAI1E,EAAIgC,EAAWK,EAGnB4C,EAAS,EAAJP,EAAQ,EAAI9E,EAAIsF,EAAQ7C,EAAIqC,EAAI,GAAM,GAAK,EAmBxD,GAfAnC,EAAIA,GAAU,EAALwC,GAKO,MAAdvC,EAAGwC,EAAK,KAAoB,EAAJN,EAAQ9E,EAAIA,EAAIsF,EAAQ7C,EAAIqC,EAAI,IAE1DnC,EAAS,EAALI,GACEsC,GAAM1C,KAAe,GAANI,GAAWA,IAAQvC,EAAEc,EAAI,EAAI,EAAI,IAClD+D,EAAK,GAAW,GAANA,IAAmB,GAANtC,GAAWJ,GAAW,GAANI,IAGnC3C,EAAI,EAAI0E,EAAI,EAAI9E,EAAIsF,EAAQ7C,EAAIqC,GAAM,EAAIlC,EAAGwC,EAAK,IAAO,GAAO,GAClErC,IAAQvC,EAAEc,EAAI,EAAI,EAAI,IAElB,EAAL6D,IAAWvC,EAAG,GAiBf,MAhBAA,GAAGpB,OAAS,EAERmB,GAGAwC,GAAM3E,EAAEL,EAAI,EAGZyC,EAAG,GAAK0C,GAAUlD,EAAW+C,EAAK/C,GAAaA,GAC/C5B,EAAEL,GAAKgF,GAAM,GAIbvC,EAAG,GAAKpC,EAAEL,EAAI,EAGXK,CAkBX,IAdU,GAALJ,GACDwC,EAAGpB,OAAS4D,EACZ1C,EAAI,EACJ0C,MAEAxC,EAAGpB,OAAS4D,EAAK,EACjB1C,EAAI4C,EAAQlD,EAAWhC,GAIvBwC,EAAGwC,GAAMN,EAAI,EAAI7C,EAAWjC,EAAIsF,EAAQ7C,EAAIqC,GAAMQ,EAAOR,IAAOpC,EAAI,GAIpEC,EAEA,OAAY,CAGR,GAAW,GAANyC,EAAU,CAGX,IAAMhF,EAAI,EAAG0E,EAAIlC,EAAG,GAAIkC,GAAK,GAAIA,GAAK,GAAI1E,KAE1C,IADA0E,EAAIlC,EAAG,IAAMF,EACPA,EAAI,EAAGoC,GAAK,GAAIA,GAAK,GAAIpC,KAG1BtC,GAAKsC,IACNlC,EAAEL,IACGyC,EAAG,IAAM8C,IAAO9C,EAAG,GAAK,GAGjC,OAGA,GADAA,EAAGwC,IAAO1C,EACLE,EAAGwC,IAAOM,EAAO,KACtB9C,GAAGwC,KAAQ,EACX1C,EAAI,EAMhB,IAAMtC,EAAIwC,EAAGpB,OAAoB,IAAZoB,IAAKxC,GAAUwC,EAAGU,QAItC9C,EAAEL,EAAI+B,EACP1B,EAAEN,EAAIM,EAAEL,EAAI,KAGJK,EAAEL,EAAIgC,IACd3B,EAAEN,GAAMM,EAAEL,EAAI,IAItB,MAAOK,GAt0CX,GAAI+C,GAAKlC,EAGLR,EAAK,EACL8E,EAAI5F,EAAU6F,UACdC,EAAM,GAAI9F,GAAU,GAYpBgB,EAAiB,GAejBC,EAAgB,EAMhBgD,EAAa,GAIb8B,EAAa,GAMb3D,EAAU,KAKVD,EAAU,IAGVxB,GAAS,EAGTE,EAAa4D,EAGbuB,GAAS,EAoBTC,EAAc,EAId/C,EAAgB,EAGhBgD,GACIC,iBAAkB,IAClBC,eAAgB,IAChBC,UAAW,EACXC,mBAAoB,EACpBC,uBAAwB,IACxBC,kBAAmB,EAy3E3B,OApsEAxG,GAAUyG,QAAU3G,EAEpBE,EAAU0G,SAAW,EACrB1G,EAAU2G,WAAa,EACvB3G,EAAU4G,WAAa,EACvB5G,EAAU6G,YAAc,EACxB7G,EAAU8G,cAAgB,EAC1B9G,EAAU+G,gBAAkB,EAC5B/G,EAAUgH,gBAAkB,EAC5BhH,EAAUiH,gBAAkB,EAC5BjH,EAAUkH,iBAAmB,EAC7BlH,EAAUmH,OAAS,EAoCnBnH,EAAUoH,OAASpH,EAAUqH,IAAM,WAC/B,GAAIC,GAAGC,EACHlH,EAAI,EACJuC,KACA4E,EAAIC,UACJC,EAAIF,EAAE,GACNG,EAAMD,GAAiB,gBAALA,GACd,WAAc,MAAKA,GAAEE,eAAeL,GAA4B,OAAdD,EAAII,EAAEH,IAA1C,QACd,WAAc,MAAKC,GAAE/F,OAASpB,EAA6B,OAAhBiH,EAAIE,EAAEnH,MAAnC,OAuHtB,OAlHKsH,GAAKJ,EAAI,mBAAsB1G,EAAYyG,EAAG,EAAGO,EAAK,EAAGN,KAC1DvG,EAAqB,EAAJsG,GAErB1E,EAAE2E,GAAKvG,EAKF2G,EAAKJ,EAAI,kBAAqB1G,EAAYyG,EAAG,EAAG,EAAG,EAAGC,KACvDtG,EAAoB,EAAJqG,GAEpB1E,EAAE2E,GAAKtG,EAMF0G,EAAKJ,EAAI,oBAELhD,EAAQ+C,GACJzG,EAAYyG,EAAE,IAAKO,EAAK,EAAG,EAAGN,IAAO1G,EAAYyG,EAAE,GAAI,EAAGO,EAAK,EAAGN,KACnEtD,EAAoB,EAAPqD,EAAE,GACfvB,EAAoB,EAAPuB,EAAE,IAEXzG,EAAYyG,GAAIO,EAAKA,EAAK,EAAGN,KACrCtD,IAAgB8B,EAAkC,GAAf,EAAJuB,GAASA,EAAIA,MAGpD1E,EAAE2E,IAAOtD,EAAY8B,GAOhB4B,EAAKJ,EAAI,WAELhD,EAAQ+C,GACJzG,EAAYyG,EAAE,IAAKO,EAAK,GAAI,EAAGN,IAAO1G,EAAYyG,EAAE,GAAI,EAAGO,EAAK,EAAGN,KACpEnF,EAAiB,EAAPkF,EAAE,GACZnF,EAAiB,EAAPmF,EAAE,IAERzG,EAAYyG,GAAIO,EAAKA,EAAK,EAAGN,KAC5B,EAAJD,EAAQlF,IAAaD,EAA+B,GAAf,EAAJmF,GAASA,EAAIA,IAC1C3G,GAAQC,EAAO,EAAG2G,EAAI,kBAAmBD,KAG1D1E,EAAE2E,IAAOnF,EAASD,GAIbwF,EAAKJ,EAAI,YAELD,MAAQA,GAAW,IAANA,GAAiB,IAANA,GACzBxG,EAAK,EACLD,GAAeF,IAAW2G,GAAM7C,EAAyBqD,GAClDnH,GACPC,EAAO,EAAG2G,EAAIQ,EAAST,IAG/B1E,EAAE2E,GAAK5G,EAKFgH,EAAKJ,EAAI,YAELD,KAAM,GAAQA,KAAM,GAAe,IAANA,GAAiB,IAANA,EACrCA,GACAA,EAAqB,mBAAVU,SACLV,GAAKU,SAAWA,OAAOC,iBAAmBD,OAAOE,aACnDlC,GAAS,EACFrF,EACPC,EAAO,EAAG,qBAAsB0G,EAAI,OAASU,QAE7ChC,GAAS,GAGbA,GAAS,EAENrF,GACPC,EAAO,EAAG2G,EAAIQ,EAAST,IAG/B1E,EAAE2E,GAAKvB,EAKF2B,EAAKJ,EAAI,gBAAmB1G,EAAYyG,EAAG,EAAG,EAAG,EAAGC,KACrDtB,EAAkB,EAAJqB,GAElB1E,EAAE2E,GAAKtB,EAKF0B,EAAKJ,EAAI,kBAAqB1G,EAAYyG,EAAG,EAAGO,EAAK,EAAGN,KACzDrE,EAAoB,EAAJoE,GAEpB1E,EAAE2E,GAAKrE,EAIFyE,EAAKJ,EAAI,YAEO,gBAALD,GACRpB,EAASoB,EACF3G,GACPC,EAAO,EAAG2G,EAAI,iBAAkBD,IAGxC1E,EAAE2E,GAAKrB,EAEAtD,GASX5C,EAAUmI,YAAcA,EAQxBnI,EAAU2E,IAAM,WAAc,MAAOR,GAAUsD,UAAW7B,EAAEwC,KAQ5DpI,EAAU0E,IAAM,WAAc,MAAOP,GAAUsD,UAAW7B,EAAEyC,KAc5DrI,EAAUsI,OAAS,WACf,GAAIC,GAAU,iBAMVC,EAAkBC,KAAKH,SAAWC,EAAW,QAC7C,WAAc,MAAOrG,GAAWuG,KAAKH,SAAWC,IAChD,WAAc,MAA2C,UAAlB,WAAhBE,KAAKH,SAAwB,IACjC,QAAhBG,KAAKH,SAAsB,GAElC,OAAO,UAAUvF,GACb,GAAIyE,GAAGtH,EAAGE,EAAGuC,EAAG2E,EACZjH,EAAI,EACJF,KACAuI,EAAO,GAAI1I,GAAU8F,EAKzB,IAHA/C,EAAW,MAANA,GAAelC,EAAYkC,EAAI,EAAG8E,EAAK,IAA6B,EAAL9E,EAAjB/B,EACnD2B,EAAI+C,EAAU3C,EAAKV,GAEf2D,EAGA,GAAIgC,OAAOC,gBAAiB,CAIxB,IAFAT,EAAIQ,OAAOC,gBAAiB,GAAIU,aAAahG,GAAK,IAEtCA,EAAJtC,GAQJiH,EAAW,OAAPE,EAAEnH,IAAgBmH,EAAEnH,EAAI,KAAO,IAM9BiH,GAAK,MACNpH,EAAI8H,OAAOC,gBAAiB,GAAIU,aAAY,IAC5CnB,EAAEnH,GAAKH,EAAE,GACTsH,EAAEnH,EAAI,GAAKH,EAAE,KAKbC,EAAEmC,KAAMgF,EAAI,MACZjH,GAAK,EAGbA,GAAIsC,EAAI,MAGL,IAAIqF,OAAOE,YAAa,CAK3B,IAFAV,EAAIQ,OAAOE,YAAavF,GAAK,GAEjBA,EAAJtC,GAMJiH,EAAsB,iBAAP,GAAPE,EAAEnH,IAA6C,cAAXmH,EAAEnH,EAAI,GAC/B,WAAXmH,EAAEnH,EAAI,GAAkC,SAAXmH,EAAEnH,EAAI,IACnCmH,EAAEnH,EAAI,IAAM,KAASmH,EAAEnH,EAAI,IAAM,GAAMmH,EAAEnH,EAAI,GAEhDiH,GAAK,KACNU,OAAOE,YAAY,GAAGU,KAAMpB,EAAGnH,IAI/BF,EAAEmC,KAAMgF,EAAI,MACZjH,GAAK,EAGbA,GAAIsC,EAAI,MAERqD,IAAS,EACLrF,GAAQC,EAAO,GAAI,qBAAsBoH,OAKrD,KAAKhC,EAED,KAAYrD,EAAJtC,GACJiH,EAAIkB,IACK,KAAJlB,IAAWnH,EAAEE,KAAOiH,EAAI,KAcrC,KAVA3E,EAAIxC,IAAIE,GACR0C,GAAMV,EAGDM,GAAKI,IACNuE,EAAI9B,EAASnD,EAAWU,GACxB5C,EAAEE,GAAK6B,EAAWS,EAAI2E,GAAMA,GAIf,IAATnH,EAAEE,GAAUF,EAAEoD,MAAOlD,KAG7B,GAAS,EAAJA,EACDF,GAAMC,EAAI,OACP,CAGH,IAAMA,EAAI,GAAc,IAATD,EAAE,GAAUA,EAAE0I,QAASzI,GAAKiC,GAG3C,IAAMhC,EAAI,EAAGiH,EAAInH,EAAE,GAAImH,GAAK,GAAIA,GAAK,GAAIjH,KAGhCgC,EAAJhC,IAAeD,GAAKiC,EAAWhC,GAKxC,MAFAqI,GAAKtI,EAAIA,EACTsI,EAAKvI,EAAIA,EACFuI,MAqGflF,EAAM,WAGF,QAASsF,GAAUrI,EAAGkC,EAAGoG,GACrB,GAAIzE,GAAG0E,EAAMC,EAAKC,EACdC,EAAQ,EACR9I,EAAII,EAAEgB,OACN2H,EAAMzG,EAAI0G,EACVC,EAAM3G,EAAI0G,EAAY,CAE1B,KAAM5I,EAAIA,EAAEW,QAASf,KACjB4I,EAAMxI,EAAEJ,GAAKgJ,EACbH,EAAMzI,EAAEJ,GAAKgJ,EAAY,EACzB/E,EAAIgF,EAAML,EAAMC,EAAME,EACtBJ,EAAOI,EAAMH,EAAU3E,EAAI+E,EAAcA,EAAcF,EACvDA,GAAUH,EAAOD,EAAO,IAAQzE,EAAI+E,EAAY,GAAMC,EAAMJ,EAC5DzI,EAAEJ,GAAK2I,EAAOD,CAKlB,OAFII,IAAO1I,EAAEgD,QAAQ0F,GAEd1I,EAGX,QAAS8I,GAAS/B,EAAGtH,EAAGsJ,EAAIC,GACxB,GAAIpJ,GAAGqJ,CAEP,IAAKF,GAAMC,EACPC,EAAMF,EAAKC,EAAK,EAAI,OAGpB,KAAMpJ,EAAIqJ,EAAM,EAAOF,EAAJnJ,EAAQA,IAEvB,GAAKmH,EAAEnH,IAAMH,EAAEG,GAAK,CAChBqJ,EAAMlC,EAAEnH,GAAKH,EAAEG,GAAK,EAAI,EACxB,OAIZ,MAAOqJ,GAGX,QAASC,GAAUnC,EAAGtH,EAAGsJ,EAAIT,GAIzB,IAHA,GAAI1I,GAAI,EAGAmJ,KACJhC,EAAEgC,IAAOnJ,EACTA,EAAImH,EAAEgC,GAAMtJ,EAAEsJ,GAAM,EAAI,EACxBhC,EAAEgC,GAAMnJ,EAAI0I,EAAOvB,EAAEgC,GAAMtJ,EAAEsJ,EAIjC,OAAShC,EAAE,IAAMA,EAAE/F,OAAS,EAAG+F,EAAEqB,UAIrC,MAAO,UAAWpI,EAAGqC,EAAGC,EAAIC,EAAI+F,GAC5B,GAAIW,GAAKtJ,EAAGC,EAAGuJ,EAAM3J,EAAG4J,EAAMC,EAAOC,EAAGC,EAAIC,EAAKC,EAAMC,EAAMC,EAAIC,EAAIC,EACjEC,EAAIC,EACJjJ,EAAId,EAAEc,GAAKuB,EAAEvB,EAAI,EAAI,GACrBsB,EAAKpC,EAAEN,EACPsK,EAAK3H,EAAE3C,CAGX,MAAM0C,GAAOA,EAAG,IAAO4H,GAAOA,EAAG,IAE7B,MAAO,IAAIzK,GAGRS,EAAEc,GAAMuB,EAAEvB,IAAOsB,GAAK4H,GAAM5H,EAAG,IAAM4H,EAAG,GAAMA,GAG7C5H,GAAe,GAATA,EAAG,KAAY4H,EAAS,EAAJlJ,EAAQA,EAAI,EAHcmJ,IAoB5D,KAbAX,EAAI,GAAI/J,GAAUuB,GAClByI,EAAKD,EAAE5J,KACPC,EAAIK,EAAEL,EAAI0C,EAAE1C,EACZmB,EAAIwB,EAAK3C,EAAI,EAEP2I,IACFA,EAAOpD,EACPvF,EAAIuK,EAAUlK,EAAEL,EAAIiC,GAAasI,EAAU7H,EAAE1C,EAAIiC,GACjDd,EAAIA,EAAIc,EAAW,GAKjBhC,EAAI,EAAGoK,EAAGpK,KAAQwC,EAAGxC,IAAM,GAAKA,KAGtC,GAFKoK,EAAGpK,IAAOwC,EAAGxC,IAAM,IAAMD,IAErB,EAAJmB,EACDyI,EAAG1H,KAAK,GACRsH,GAAO,MACJ,CAwBH,IAvBAS,EAAKxH,EAAGpB,OACR8I,EAAKE,EAAGhJ,OACRpB,EAAI,EACJkB,GAAK,EAILtB,EAAIiC,EAAW6G,GAAS0B,EAAG,GAAK,IAI3BxK,EAAI,IACLwK,EAAK3B,EAAU2B,EAAIxK,EAAG8I,GACtBlG,EAAKiG,EAAUjG,EAAI5C,EAAG8I,GACtBwB,EAAKE,EAAGhJ,OACR4I,EAAKxH,EAAGpB,QAGZ2I,EAAKG,EACLN,EAAMpH,EAAGzB,MAAO,EAAGmJ,GACnBL,EAAOD,EAAIxI,OAGI8I,EAAPL,EAAWD,EAAIC,KAAU,GACjCM,EAAKC,EAAGrJ,QACRoJ,EAAG/G,QAAQ,GACX6G,EAAMG,EAAG,GACJA,EAAG,IAAM1B,EAAO,GAAIuB,GAIzB,GAAG,CAOC,GANArK,EAAI,EAGJyJ,EAAMH,EAASkB,EAAIR,EAAKM,EAAIL,GAGjB,EAANR,EAAU,CAkBX,GAdAS,EAAOF,EAAI,GACNM,GAAML,IAAOC,EAAOA,EAAOpB,GAASkB,EAAI,IAAM,IAGnDhK,EAAIiC,EAAWiI,EAAOG,GAUjBrK,EAAI,EAeL,IAZIA,GAAK8I,IAAM9I,EAAI8I,EAAO,GAG1Bc,EAAOf,EAAU2B,EAAIxK,EAAG8I,GACxBe,EAAQD,EAAKpI,OACbyI,EAAOD,EAAIxI,OAOkC,GAArC8H,EAASM,EAAMI,EAAKH,EAAOI,IAC/BjK,IAGA0J,EAAUE,EAAWC,EAALS,EAAaC,EAAKC,EAAIX,EAAOf,GAC7Ce,EAAQD,EAAKpI,OACbiI,EAAM,MAQA,IAALzJ,IAGDyJ,EAAMzJ,EAAI,GAId4J,EAAOY,EAAGrJ,QACV0I,EAAQD,EAAKpI,MAUjB,IAPayI,EAARJ,GAAeD,EAAKpG,QAAQ,GAGjCkG,EAAUM,EAAKJ,EAAMK,EAAMnB,GAC3BmB,EAAOD,EAAIxI,OAGC,IAAPiI,EAMD,KAAQH,EAASkB,EAAIR,EAAKM,EAAIL,GAAS,GACnCjK,IAGA0J,EAAUM,EAAUC,EAALK,EAAYC,EAAKC,EAAIP,EAAMnB,GAC1CmB,EAAOD,EAAIxI,WAGH,KAARiI,IACRzJ,IACAgK,GAAO,GAIXD,GAAG3J,KAAOJ,EAGLgK,EAAI,GACLA,EAAIC,KAAUrH,EAAGuH,IAAO,GAExBH,GAAQpH,EAAGuH,IACXF,EAAO,UAEHE,IAAOC,GAAgB,MAAVJ,EAAI,KAAgB1I,IAE7CqI,GAAiB,MAAVK,EAAI,GAGLD,EAAG,IAAKA,EAAGnB,QAGrB,GAAKE,GAAQpD,EAAO,CAGhB,IAAMtF,EAAI,EAAGkB,EAAIyI,EAAG,GAAIzI,GAAK,GAAIA,GAAK,GAAIlB,KAC1CU,EAAOgJ,EAAGhH,GAAOgH,EAAE3J,EAAIC,EAAID,EAAIiC,EAAW,GAAM,EAAGW,EAAI4G,OAIvDG,GAAE3J,EAAIA,EACN2J,EAAEnH,GAAKgH,CAGX,OAAOG,OAgJfzI,EAAe,WACX,GAAIsJ,GAAa,8BACbC,EAAW,cACXC,EAAY,cACZC,EAAkB,qBAClBC,EAAmB,4BAEvB,OAAO,UAAWvK,EAAGD,EAAKF,EAAKJ,GAC3B,GAAI6I,GACAxH,EAAIjB,EAAME,EAAMA,EAAIgB,QAASwJ,EAAkB,GAGnD,IAAKD,EAAgB1J,KAAKE,GACtBd,EAAEc,EAAI0J,MAAM1J,GAAK,KAAW,EAAJA,EAAQ,GAAK,MAClC,CACH,IAAMjB,IAGFiB,EAAIA,EAAEC,QAASoJ,EAAY,SAAWtG,EAAG4G,EAAIC,GAEzC,MADApC,GAAoC,MAA3BoC,EAAKA,EAAGlI,eAAyB,GAAW,KAANkI,EAAY,EAAI,EACvDjL,GAAKA,GAAK6I,EAAYzE,EAAL4G,IAGzBhL,IACA6I,EAAO7I,EAGPqB,EAAIA,EAAEC,QAASqJ,EAAU,MAAOrJ,QAASsJ,EAAW,SAGnDtK,GAAOe,GAAI,MAAO,IAAIvB,GAAWuB,EAAGwH,EAKzCpI,IAAQC,EAAOE,EAAI,SAAYZ,EAAI,SAAWA,EAAI,IAAO,UAAWM,GACxEC,EAAEc,EAAI,KAGVd,EAAEN,EAAIM,EAAEL,EAAI,KACZU,EAAK,MAmNb8E,EAAEwF,cAAgBxF,EAAEyF,IAAM,WACtB,GAAI5K,GAAI,GAAIT,GAAUU,KAEtB,OADKD,GAAEc,EAAI,IAAId,EAAEc,EAAI,GACdd,GAQXmF,EAAE0F,KAAO,WACL,MAAOvK,GAAO,GAAIf,GAAUU,MAAOA,KAAKN,EAAI,EAAG,IAWnDwF,EAAE2F,WAAa3F,EAAE8D,IAAM,SAAW5G,EAAG5C,GAEjC,MADAY,GAAK,EACEyI,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,KAQ5C0F,EAAE4F,cAAgB5F,EAAE7C,GAAK,WACrB,GAAI9C,GAAGqH,EACHnH,EAAIO,KAAKP,CAEb,KAAMA,EAAI,MAAO,KAIjB,IAHAF,IAAQqH,EAAInH,EAAEsB,OAAS,GAAMkJ,EAAUjK,KAAKN,EAAIiC,IAAeA,EAG1DiF,EAAInH,EAAEmH,GAAK,KAAQA,EAAI,IAAM,EAAGA,GAAK,GAAIrH,KAG9C,MAFS,GAAJA,IAAQA,EAAI,GAEVA,GAwBX2F,EAAE6F,UAAY7F,EAAEpC,IAAM,SAAWV,EAAG5C,GAEhC,MADAY,GAAK,EACE0C,EAAK9C,KAAM,GAAIV,GAAW8C,EAAG5C,GAAKc,EAAgBC,IAQ7D2E,EAAE8F,mBAAqB9F,EAAE+F,SAAW,SAAW7I,EAAG5C,GAE9C,MADAY,GAAK,EACE0C,EAAK9C,KAAM,GAAIV,GAAW8C,EAAG5C,GAAK,EAAG,IAQhD0F,EAAEgG,OAAShG,EAAEiG,GAAK,SAAW/I,EAAG5C,GAE5B,MADAY,GAAK,EAC6C,IAA3CyI,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,KAQ5C0F,EAAEkG,MAAQ,WACN,MAAO/K,GAAO,GAAIf,GAAUU,MAAOA,KAAKN,EAAI,EAAG,IAQnDwF,EAAEmG,YAAcnG,EAAEyC,GAAK,SAAWvF,EAAG5C,GAEjC,MADAY,GAAK,EACEyI,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,IAAQ,GAQpD0F,EAAEoG,qBAAuBpG,EAAEqG,IAAM,SAAWnJ,EAAG5C,GAE3C,MADAY,GAAK,EACqD,KAAjDZ,EAAIqJ,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,MAAuB,IAANA,GAQnE0F,EAAEsG,SAAW,WACT,QAASxL,KAAKP,GAOlByF,EAAEuG,UAAYvG,EAAEwG,MAAQ,WACpB,QAAS1L,KAAKP,GAAKwK,EAAUjK,KAAKN,EAAIiC,GAAa3B,KAAKP,EAAEsB,OAAS,GAOvEmE,EAAEqF,MAAQ,WACN,OAAQvK,KAAKa,GAOjBqE,EAAEyG,WAAazG,EAAE0G,MAAQ,WACrB,MAAO5L,MAAKa,EAAI,GAOpBqE,EAAE2G,OAAS,WACP,QAAS7L,KAAKP,GAAkB,GAAbO,KAAKP,EAAE,IAQ9ByF,EAAE4G,SAAW5G,EAAEwC,GAAK,SAAWtF,EAAG5C,GAE9B,MADAY,GAAK,EACEyI,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,IAAQ,GAQpD0F,EAAE6G,kBAAoB7G,EAAE8G,IAAM,SAAW5J,EAAG5C,GAExC,MADAY,GAAK,EACqD,MAAjDZ,EAAIqJ,EAAS7I,KAAM,GAAIV,GAAW8C,EAAG5C,MAAwB,IAANA,GAwBpE0F,EAAE+G,MAAQ/G,EAAEgH,IAAM,SAAW9J,EAAG5C,GAC5B,GAAIG,GAAG0E,EAAG8H,EAAGC,EACTrM,EAAIC,KACJ8G,EAAI/G,EAAEc,CAOV,IALAT,EAAK,GACLgC,EAAI,GAAI9C,GAAW8C,EAAG5C,GACtBA,EAAI4C,EAAEvB,GAGAiG,IAAMtH,EAAI,MAAO,IAAIF,GAAU0K,IAGrC,IAAKlD,GAAKtH,EAEN,MADA4C,GAAEvB,GAAKrB,EACAO,EAAEsM,KAAKjK,EAGlB,IAAIkK,GAAKvM,EAAEL,EAAIiC,EACX4K,EAAKnK,EAAE1C,EAAIiC,EACXQ,EAAKpC,EAAEN,EACPsK,EAAK3H,EAAE3C,CAEX,KAAM6M,IAAOC,EAAK,CAGd,IAAMpK,IAAO4H,EAAK,MAAO5H,IAAOC,EAAEvB,GAAKrB,EAAG4C,GAAM,GAAI9C,GAAWyK,EAAKhK,EAAIiK,IAGxE,KAAM7H,EAAG,KAAO4H,EAAG,GAGf,MAAOA,GAAG,IAAO3H,EAAEvB,GAAKrB,EAAG4C,GAAM,GAAI9C,GAAW6C,EAAG,GAAKpC,EAGrC,GAAjBQ,GAAsB,EAAI,GASpC,GALA+L,EAAKrC,EAASqC,GACdC,EAAKtC,EAASsC,GACdpK,EAAKA,EAAGzB,QAGHoG,EAAIwF,EAAKC,EAAK,CAaf,KAXKH,EAAW,EAAJtF,IACRA,GAAKA,EACLqF,EAAIhK,IAEJoK,EAAKD,EACLH,EAAIpC,GAGRoC,EAAEK,UAGIhN,EAAIsH,EAAGtH,IAAK2M,EAAEvK,KAAK,IACzBuK,EAAEK,cAMF,KAFAnI,GAAM+H,GAAStF,EAAI3E,EAAGpB,SAAavB,EAAIuK,EAAGhJ,SAAa+F,EAAItH,EAErDsH,EAAItH,EAAI,EAAO6E,EAAJ7E,EAAOA,IAEpB,GAAK2C,EAAG3C,IAAMuK,EAAGvK,GAAK,CAClB4M,EAAOjK,EAAG3C,GAAKuK,EAAGvK,EAClB,OAYZ,GANI4M,IAAMD,EAAIhK,EAAIA,EAAK4H,EAAIA,EAAKoC,EAAG/J,EAAEvB,GAAKuB,EAAEvB,GAE5CrB,GAAM6E,EAAI0F,EAAGhJ,SAAapB,EAAIwC,EAAGpB,QAI5BvB,EAAI,EAAI,KAAQA,IAAK2C,EAAGxC,KAAO,GAIpC,IAHAH,EAAIyF,EAAO,EAGHZ,EAAIyC,GAAK,CAEb,GAAK3E,IAAKkC,GAAK0F,EAAG1F,GAAK,CACnB,IAAM1E,EAAI0E,EAAG1E,IAAMwC,IAAKxC,GAAIwC,EAAGxC,GAAKH,KAClC2C,EAAGxC,GACLwC,EAAGkC,IAAMY,EAGb9C,EAAGkC,IAAM0F,EAAG1F,GAIhB,KAAiB,GAATlC,EAAG,GAASA,EAAGgG,UAAWoE,GAGlC,MAAMpK,GAAG,GAWFiC,EAAWhC,EAAGD,EAAIoK,IAPrBnK,EAAEvB,EAAqB,GAAjBN,EAAqB,GAAK,EAChC6B,EAAE3C,GAAM2C,EAAE1C,EAAI,GACP0C,IA8Bf8C,EAAEuH,OAASvH,EAAEwH,IAAM,SAAWtK,EAAG5C,GAC7B,GAAI6J,GAAGxI,EACHd,EAAIC,IAMR,OAJAI,GAAK,GACLgC,EAAI,GAAI9C,GAAW8C,EAAG5C,IAGhBO,EAAEN,IAAM2C,EAAEvB,GAAKuB,EAAE3C,IAAM2C,EAAE3C,EAAE,GACtB,GAAIH,GAAU0K,MAGZ5H,EAAE3C,GAAKM,EAAEN,IAAMM,EAAEN,EAAE,GACrB,GAAIH,GAAUS,IAGL,GAAfwF,GAID1E,EAAIuB,EAAEvB,EACNuB,EAAEvB,EAAI,EACNwI,EAAIvG,EAAK/C,EAAGqC,EAAG,EAAG,GAClBA,EAAEvB,EAAIA,EACNwI,EAAExI,GAAKA,GAEPwI,EAAIvG,EAAK/C,EAAGqC,EAAG,EAAGmD,GAGfxF,EAAEkM,MAAO5C,EAAEsD,MAAMvK,MAQ5B8C,EAAE0H,QAAU1H,EAAE2H,IAAM,WAChB,GAAI9M,GAAI,GAAIT,GAAUU,KAEtB,OADAD,GAAEc,GAAKd,EAAEc,GAAK,KACPd,GAwBXmF,EAAEmH,KAAOnH,EAAE4H,IAAM,SAAW1K,EAAG5C,GAC3B,GAAI2M,GACApM,EAAIC,KACJ8G,EAAI/G,EAAEc,CAOV,IALAT,EAAK,GACLgC,EAAI,GAAI9C,GAAW8C,EAAG5C,GACtBA,EAAI4C,EAAEvB,GAGAiG,IAAMtH,EAAI,MAAO,IAAIF,GAAU0K,IAGpC,IAAKlD,GAAKtH,EAEP,MADA4C,GAAEvB,GAAKrB,EACAO,EAAEkM,MAAM7J,EAGnB,IAAIkK,GAAKvM,EAAEL,EAAIiC,EACX4K,EAAKnK,EAAE1C,EAAIiC,EACXQ,EAAKpC,EAAEN,EACPsK,EAAK3H,EAAE3C,CAEX,KAAM6M,IAAOC,EAAK,CAGd,IAAMpK,IAAO4H,EAAK,MAAO,IAAIzK,GAAWwH,EAAI,EAI5C,KAAM3E,EAAG,KAAO4H,EAAG,GAAK,MAAOA,GAAG,GAAK3H,EAAI,GAAI9C,GAAW6C,EAAG,GAAKpC,EAAQ,EAAJ+G,GAQ1E,GALAwF,EAAKrC,EAASqC,GACdC,EAAKtC,EAASsC,GACdpK,EAAKA,EAAGzB,QAGHoG,EAAIwF,EAAKC,EAAK,CAUf,IATKzF,EAAI,GACLyF,EAAKD,EACLH,EAAIpC,IAEJjD,GAAKA,EACLqF,EAAIhK,GAGRgK,EAAEK,UACM1F,IAAKqF,EAAEvK,KAAK,IACpBuK,EAAEK,UAUN,IAPA1F,EAAI3E,EAAGpB,OACPvB,EAAIuK,EAAGhJ,OAGM,EAAR+F,EAAItH,IAAQ2M,EAAIpC,EAAIA,EAAK5H,EAAIA,EAAKgK,EAAG3M,EAAIsH,GAGxCA,EAAI,EAAGtH,GACTsH,GAAM3E,IAAK3C,GAAK2C,EAAG3C,GAAKuK,EAAGvK,GAAKsH,GAAM7B,EAAO,EAC7C9C,EAAG3C,GAAKyF,IAAS9C,EAAG3C,GAAK,EAAI2C,EAAG3C,GAAKyF,CAUzC,OAPI6B,KACA3E,EAAGY,QAAQ+D,KACTyF,GAKCnI,EAAWhC,EAAGD,EAAIoK,IAS7BrH,EAAE6H,UAAY7H,EAAER,GAAK,SAAUsI,GAC3B,GAAIzN,GAAGqH,EACH7G,EAAIC,KACJP,EAAIM,EAAEN,CAQV,IALU,MAALuN,GAAaA,MAAQA,GAAW,IAANA,GAAiB,IAANA,IAClC/M,GAAQC,EAAO,GAAI,WAAamH,EAAS2F,GACxCA,KAAOA,IAAIA,EAAI,QAGlBvN,EAAI,MAAO,KAIjB,IAHAmH,EAAInH,EAAEsB,OAAS,EACfxB,EAAIqH,EAAIjF,EAAW,EAEdiF,EAAInH,EAAEmH,GAAK,CAGZ,KAAQA,EAAI,IAAM,EAAGA,GAAK,GAAIrH,KAG9B,IAAMqH,EAAInH,EAAE,GAAImH,GAAK,GAAIA,GAAK,GAAIrH,MAKtC,MAFKyN,IAAKjN,EAAEL,EAAI,EAAIH,IAAIA,EAAIQ,EAAEL,EAAI,GAE3BH,GAiBX2F,EAAE7E,MAAQ,SAAWgC,EAAIC,GACrB,GAAI/C,GAAI,GAAID,GAAUU,KAOtB,QALW,MAANqC,GAAclC,EAAYkC,EAAI,EAAG8E,EAAK,MACvC9G,EAAOd,IAAK8C,EAAKrC,KAAKN,EAAI,EAAS,MAAN4C,GAC1BnC,EAAYmC,EAAI,EAAG,EAAG,GAAIe,GAAsC,EAALf,EAAhB/B,GAG3ChB,GAgBX2F,EAAEiD,MAAQ,SAAUlG,GAChB,GAAI1C,GAAIS,IACR,OAAOG,GAAY8B,GAAIV,EAAkBA,EAAkB,GAAI,YAG3DhC,EAAEoN,MAAO,KAAOxI,EAASlC,IACzB,GAAI3C,GAAWC,EAAEE,GAAKF,EAAEE,EAAE,MAAa8B,EAALU,GAAyBA,EAAIV,GAC7DhC,EAAEsB,GAAU,EAAJoB,EAAQ,EAAI,EAAI,GACxB1C,IAeV2F,EAAE+H,WAAa/H,EAAEgI,KAAO,WACpB,GAAItJ,GAAGrE,EAAG2C,EAAGiL,EAAKhB,EACdpM,EAAIC,KACJP,EAAIM,EAAEN,EACNoB,EAAId,EAAEc,EACNnB,EAAIK,EAAEL,EACN2C,EAAK/B,EAAiB,EACtB8M,EAAO,GAAI9N,GAAU,MAGzB,IAAW,IAANuB,IAAYpB,IAAMA,EAAE,GACrB,MAAO,IAAIH,IAAYuB,GAAS,EAAJA,KAAYpB,GAAKA,EAAE,IAAOuK,IAAMvK,EAAIM,EAAI,EAAI,EA8B5E,IA1BAc,EAAIkH,KAAKmF,MAAOnN,GAIN,GAALc,GAAUA,GAAK,EAAI,GACpBtB,EAAIqD,EAAcnD,IACXF,EAAEwB,OAASrB,GAAM,GAAK,IAAIH,GAAK,KACtCsB,EAAIkH,KAAKmF,KAAK3N,GACdG,EAAIuK,GAAYvK,EAAI,GAAM,IAAY,EAAJA,GAASA,EAAI,GAE1CmB,GAAK,EAAI,EACVtB,EAAI,KAAOG,GAEXH,EAAIsB,EAAE2C,gBACNjE,EAAIA,EAAEmB,MAAO,EAAGnB,EAAE6B,QAAQ,KAAO,GAAM1B,GAG3CwC,EAAI,GAAI5C,GAAUC,IAElB2C,EAAI,GAAI5C,GAAWuB,EAAI,IAOtBqB,EAAEzC,EAAE,GAML,IALAC,EAAIwC,EAAExC,EACNmB,EAAInB,EAAI2C,EACC,EAAJxB,IAAQA,EAAI,KAOb,GAHAsL,EAAIjK,EACJA,EAAIkL,EAAKT,MAAOR,EAAEE,KAAMvJ,EAAK/C,EAAGoM,EAAG9J,EAAI,KAElCO,EAAeuJ,EAAE1M,GAAMiB,MAAO,EAAGG,MAAUtB,EAC3CqD,EAAeV,EAAEzC,IAAMiB,MAAO,EAAGG,GAAM,CAWxC,GANKqB,EAAExC,EAAIA,KAAMmB,EACjBtB,EAAIA,EAAEmB,MAAOG,EAAI,EAAGA,EAAI,GAKd,QAALtB,IAAgB4N,GAAY,QAAL5N,GAgBrB,IAIIA,KAAOA,EAAEmB,MAAM,IAAqB,KAAfnB,EAAEyD,OAAO,MAGjC3C,EAAO6B,EAAGA,EAAExC,EAAIY,EAAiB,EAAG,GACpCsD,GAAK1B,EAAEyK,MAAMzK,GAAGiJ,GAAGpL,GAGvB,OAvBA,IAAMoN,IACF9M,EAAO8L,EAAGA,EAAEzM,EAAIY,EAAiB,EAAG,GAE/B6L,EAAEQ,MAAMR,GAAGhB,GAAGpL,IAAK,CACpBmC,EAAIiK,CACJ,OAIR9J,GAAM,EACNxB,GAAK,EACLsM,EAAM,EAkBtB,MAAO9M,GAAO6B,EAAGA,EAAExC,EAAIY,EAAiB,EAAGC,EAAeqD,IAwB9DsB,EAAEyH,MAAQzH,EAAEmI,IAAM,SAAWjL,EAAG5C,GAC5B,GAAIC,GAAGC,EAAGC,EAAG0E,EAAGpC,EAAG2B,EAAG0J,EAAK/E,EAAKC,EAAK+E,EAAKC,EAAKC,EAAKC,EAChDrF,EAAMsF,EACN5N,EAAIC,KACJmC,EAAKpC,EAAEN,EACPsK,GAAO3J,EAAK,GAAIgC,EAAI,GAAI9C,GAAW8C,EAAG5C,IAAMC,CAGhD,MAAM0C,GAAO4H,GAAO5H,EAAG,IAAO4H,EAAG,IAmB7B,OAhBMhK,EAAEc,IAAMuB,EAAEvB,GAAKsB,IAAOA,EAAG,KAAO4H,GAAMA,IAAOA,EAAG,KAAO5H,EACzDC,EAAE3C,EAAI2C,EAAE1C,EAAI0C,EAAEvB,EAAI,MAElBuB,EAAEvB,GAAKd,EAAEc,EAGHsB,GAAO4H,GAKT3H,EAAE3C,GAAK,GACP2C,EAAE1C,EAAI,GALN0C,EAAE3C,EAAI2C,EAAE1C,EAAI,MASb0C,CAYX,KATA1C,EAAIuK,EAAUlK,EAAEL,EAAIiC,GAAasI,EAAU7H,EAAE1C,EAAIiC,GACjDS,EAAEvB,GAAKd,EAAEc,EACTyM,EAAMnL,EAAGpB,OACTwM,EAAMxD,EAAGhJ,OAGEwM,EAAND,IAAYI,EAAKvL,EAAIA,EAAK4H,EAAIA,EAAK2D,EAAI/N,EAAI2N,EAAKA,EAAMC,EAAKA,EAAM5N,GAGhEA,EAAI2N,EAAMC,EAAKG,KAAS/N,IAAK+N,EAAG9L,KAAK,IAK3C,IAHAyG,EAAOpD,EACP0I,EAAWhF,EAELhJ,EAAI4N,IAAO5N,GAAK,GAAK,CAKvB,IAJAF,EAAI,EACJ+N,EAAMzD,EAAGpK,GAAKgO,EACdF,EAAM1D,EAAGpK,GAAKgO,EAAW,EAEnB1L,EAAIqL,EAAKjJ,EAAI1E,EAAIsC,EAAGoC,EAAI1E,GAC1B4I,EAAMpG,IAAKF,GAAK0L,EAChBnF,EAAMrG,EAAGF,GAAK0L,EAAW,EACzB/J,EAAI6J,EAAMlF,EAAMC,EAAMgF,EACtBjF,EAAMiF,EAAMjF,EAAU3E,EAAI+J,EAAaA,EAAaD,EAAGrJ,GAAK5E,EAC5DA,GAAM8I,EAAMF,EAAO,IAAQzE,EAAI+J,EAAW,GAAMF,EAAMjF,EACtDkF,EAAGrJ,KAAOkE,EAAMF,CAGpBqF,GAAGrJ,GAAK5E,EASZ,MANIA,KACEC,EAEFgO,EAAGvF,QAGA/D,EAAWhC,EAAGsL,EAAIhO,IAgB7BwF,EAAE0I,SAAW,SAAWlJ,EAAIpC,GACxB,GAAI/C,GAAI,GAAID,GAAUU,KAGtB,OAFA0E,GAAW,MAANA,GAAevE,EAAYuE,EAAI,EAAGyC,EAAK,GAAI,aAA4B,EAALzC,EAAP,KAChEpC,EAAW,MAANA,GAAenC,EAAYmC,EAAI,EAAG,EAAG,GAAIe,GAAsC,EAALf,EAAhB/B,EACxDmE,EAAKrE,EAAOd,EAAGmF,EAAIpC,GAAO/C,GAgBrC2F,EAAE1B,cAAgB,SAAWnB,EAAIC,GAC7B,MAAOW,GAAQjD,KACP,MAANqC,GAAclC,EAAYkC,EAAI,EAAG8E,EAAK,MAAS9E,EAAK,EAAI,KAAMC,EAAI,KAmBxE4C,EAAE2I,QAAU,SAAWxL,EAAIC,GACvB,MAAOW,GAAQjD,KAAY,MAANqC,GAAclC,EAAYkC,EAAI,EAAG8E,EAAK,MACrD9E,EAAKrC,KAAKN,EAAI,EAAI,KAAM4C,EAAI,KA0BtC4C,EAAE4I,SAAW,SAAWzL,EAAIC,GACxB,GAAIxC,GAAMmD,EAAQjD,KAAY,MAANqC,GAAclC,EAAYkC,EAAI,EAAG8E,EAAK,MACxD9E,EAAKrC,KAAKN,EAAI,EAAI,KAAM4C,EAAI,GAElC,IAAKtC,KAAKP,EAAI,CACV,GAAIE,GACAoO,EAAMjO,EAAIkO,MAAM,KAChBC,GAAMzI,EAAOG,UACbuI,GAAM1I,EAAOI,mBACbF,EAAiBF,EAAOE,eACxByI,EAAUJ,EAAI,GACdK,EAAeL,EAAI,GACnBnC,EAAQ5L,KAAKa,EAAI,EACjBwN,EAAYzC,EAAQuC,EAAQzN,MAAM,GAAKyN,EACvCtO,EAAMwO,EAAUtN,MAIpB,IAFImN,IAAIvO,EAAIsO,EAAIA,EAAKC,EAAIA,EAAKvO,EAAGE,GAAOF,GAEnCsO,EAAK,GAAKpO,EAAM,EAAI,CAIrB,IAHAF,EAAIE,EAAMoO,GAAMA,EAChBE,EAAUE,EAAUC,OAAQ,EAAG3O,GAEnBE,EAAJF,EAASA,GAAKsO,EAClBE,GAAWzI,EAAiB2I,EAAUC,OAAQ3O,EAAGsO,EAGhDC,GAAK,IAAIC,GAAWzI,EAAiB2I,EAAU3N,MAAMf,IACtDiM,IAAOuC,EAAU,IAAMA,GAG/BrO,EAAMsO,EACFD,EAAU3I,EAAOC,mBAAuByI,GAAM1I,EAAOM,mBACnDsI,EAAatN,QAAS,GAAIN,QAAQ,OAAS0N,EAAK,OAAQ,KACxD,KAAO1I,EAAOK,wBACduI,GACFD,EAGR,MAAOrO,IAgBXoF,EAAEqJ,WAAa,SAAUC,GACrB,GAAIT,GAAKU,EAAIC,EAAIhP,EAAGiP,EAAKpP,EAAGqP,EAAIvF,EAAGxI,EAC/BoB,EAAIhC,EACJF,EAAIC,KACJmC,EAAKpC,EAAEN,EACPuC,EAAI,GAAI1C,GAAU8F,GAClByJ,EAAKJ,EAAK,GAAInP,GAAU8F,GACxB0J,EAAKF,EAAK,GAAItP,GAAU8F,EAoB5B,IAlBW,MAANoJ,IACDvO,GAAS,EACTV,EAAI,GAAID,GAAUkP,GAClBvO,EAASgC,KAEDA,EAAI1C,EAAEmM,UAAanM,EAAEmI,GAAGtC,MAExBnF,GACAC,EAAO,GACL,oBAAuB+B,EAAI,eAAiB,kBAAoBuM,GAKtEA,GAAMvM,GAAK1C,EAAEE,GAAKY,EAAOd,EAAGA,EAAEG,EAAI,EAAG,GAAI6L,IAAInG,GAAO7F,EAAI,QAI1D4C,EAAK,MAAOpC,GAAEuD,UAgBpB,KAfAzC,EAAI+B,EAAcT,GAIlBzC,EAAIsC,EAAEtC,EAAImB,EAAEE,OAAShB,EAAEL,EAAI,EAC3BsC,EAAEvC,EAAE,GAAKqF,GAAY6J,EAAMjP,EAAIiC,GAAa,EAAIA,EAAWgN,EAAMA,GACjEH,GAAMA,GAAMjP,EAAEyJ,IAAIhH,GAAK,EAAMtC,EAAI,EAAIsC,EAAI6M,EAAOtP,EAEhDoP,EAAMlN,EACNA,EAAU,EAAI,EACdlC,EAAI,GAAID,GAAUuB,GAGlB+N,EAAGnP,EAAE,GAAK,EAGN4J,EAAIvG,EAAKvD,EAAGyC,EAAG,EAAG,GAClB0M,EAAKD,EAAGpC,KAAMhD,EAAEsD,MAAMmC,IACH,GAAdJ,EAAG1F,IAAIwF,IACZC,EAAKK,EACLA,EAAKJ,EACLG,EAAKD,EAAGvC,KAAMhD,EAAEsD,MAAO+B,EAAKG,IAC5BD,EAAKF,EACL1M,EAAIzC,EAAE0M,MAAO5C,EAAEsD,MAAO+B,EAAK1M,IAC3BzC,EAAImP,CAgBR,OAbAA,GAAK5L,EAAK0L,EAAGvC,MAAMwC,GAAKK,EAAI,EAAG,GAC/BF,EAAKA,EAAGvC,KAAMqC,EAAG/B,MAAMkC,IACvBJ,EAAKA,EAAGpC,KAAMqC,EAAG/B,MAAMmC,IACvBF,EAAG/N,EAAIgO,EAAGhO,EAAId,EAAEc,EAChBnB,GAAK,EAGLqO,EAAMjL,EAAK+L,EAAIC,EAAIpP,EAAGa,GAAgB0L,MAAMlM,GAAG4K,MAAM3B,IAC/ClG,EAAK8L,EAAIH,EAAI/O,EAAGa,GAAgB0L,MAAMlM,GAAG4K,OAAU,GAC7CkE,EAAGvL,WAAYwL,EAAGxL,aAClBsL,EAAGtL,WAAYmL,EAAGnL,YAE9B7B,EAAUkN,EACHZ,GAOX7I,EAAE6J,SAAW,WACT,OAAQ/O,MAsBZkF,EAAE8J,QAAU9J,EAAEzC,IAAM,SAAWlD,EAAGqE,GAC9B,GAAI3B,GAAGG,EAAG4K,EACNrN,EAAI6B,EAAe,EAAJjC,GAASA,GAAKA,GAC7BQ,EAAIC,IAQR,IANU,MAAL4D,IACDxD,EAAK,GACLwD,EAAI,GAAItE,GAAUsE,KAIhBzD,EAAYZ,GAAIgC,EAAkBA,EAAkB,GAAI,eACzDiK,SAASjM,IAAMI,EAAI4B,IAAsBhC,GAAK,IAC/C0P,WAAW1P,IAAMA,KAAQA,EAAIyK,OAAgB,GAALzK,EAExC,MADA0C,GAAI8F,KAAKtF,KAAM1C,EAAGR,GACX,GAAID,GAAWsE,EAAI3B,EAAI2B,EAAI3B,EAuBtC,KApBI2B,EACKrE,EAAI,GAAKQ,EAAE4H,GAAGvC,IAAQrF,EAAE2L,SAAW9H,EAAE+D,GAAGvC,IAAQxB,EAAE8H,QACnD3L,EAAIA,EAAE2M,IAAI9I,IAEVoJ,EAAIpJ,EAGJA,EAAI,MAEDpB,IAMPP,EAAI+C,EAAUxC,EAAgBb,EAAW,IAG7CS,EAAI,GAAI9C,GAAU8F,KAEN,CACR,GAAKzF,EAAI,EAAI,CAET,GADAyC,EAAIA,EAAEuK,MAAM5M,IACNqC,EAAE3C,EAAI,KACRwC,GACKG,EAAE3C,EAAEsB,OAASkB,IAAIG,EAAE3C,EAAEsB,OAASkB,GAC5B2B,IACPxB,EAAIA,EAAEsK,IAAI9I,IAKlB,GADAjE,EAAI6B,EAAW7B,EAAI,IACbA,EAAI,KACVI,GAAIA,EAAE4M,MAAM5M,GACRkC,EACKlC,EAAEN,GAAKM,EAAEN,EAAEsB,OAASkB,IAAIlC,EAAEN,EAAEsB,OAASkB,GACnC2B,IACP7D,EAAIA,EAAE2M,IAAI9I,IAIlB,MAAIA,GAAUxB,GACL,EAAJ7C,IAAQ6C,EAAIgD,EAAItC,IAAIV,IAElB4K,EAAI5K,EAAEsK,IAAIM,GAAK/K,EAAI5B,EAAO+B,EAAGI,EAAejC,GAAkB6B,IAkBzE8C,EAAEgK,YAAc,SAAWxK,EAAIpC,GAC3B,MAAOW,GAAQjD,KAAY,MAAN0E,GAAcvE,EAAYuE,EAAI,EAAGyC,EAAK,GAAI,aACtD,EAALzC,EAAS,KAAMpC,EAAI,KAgB3B4C,EAAE5B,SAAW,SAAU9D,GACnB,GAAIM,GACAP,EAAIS,KACJa,EAAItB,EAAEsB,EACNnB,EAAIH,EAAEG,CAyBV,OAtBW,QAANA,EAEGmB,GACAf,EAAM,WACG,EAAJe,IAAQf,EAAM,IAAMA,IAEzBA,EAAM,OAGVA,EAAM8C,EAAerD,EAAEE,GAOnBK,EALM,MAALN,GAAcW,EAAYX,EAAG,EAAG,GAAI,GAAI,QAKnC0B,EAAayB,EAAc7C,EAAKJ,GAAS,EAAJF,EAAO,GAAIqB,GAJ3C0C,GAAL7D,GAAmBA,GAAK2F,EAC1B7B,EAAe1D,EAAKJ,GACpBiD,EAAc7C,EAAKJ,GAKlB,EAAJmB,GAAStB,EAAEE,EAAE,KAAKK,EAAM,IAAMA,IAGhCA,GAQXoF,EAAEiK,UAAYjK,EAAEkK,MAAQ,WACpB,MAAO/O,GAAO,GAAIf,GAAUU,MAAOA,KAAKN,EAAI,EAAG,IAQnDwF,EAAEmK,QAAUnK,EAAEoK,OAAS,WACnB,GAAIxP,GACAP,EAAIS,KACJN,EAAIH,EAAEG,CAEV,OAAW,QAANA,EAAoBH,EAAE+D,YAE3BxD,EAAM8C,EAAerD,EAAEE,GAEvBK,EAAWyD,GAAL7D,GAAmBA,GAAK2F,EACxB7B,EAAe1D,EAAKJ,GACpBiD,EAAc7C,EAAKJ,GAElBH,EAAEsB,EAAI,EAAI,IAAMf,EAAMA,IAIf,MAAbT,GAAoBC,EAAUoH,OAAOrH,GAEnCC,EAOX,QAAS2K,GAAS1K,GACd,GAAII,GAAQ,EAAJJ,CACR,OAAOA,GAAI,GAAKA,IAAMI,EAAIA,EAAIA,EAAI,EAKtC,QAASiD,GAAckE,GAMnB,IALA,GAAIjG,GAAGmM,EACHrN,EAAI,EACJ0E,EAAIyC,EAAE/F,OACNmB,EAAI4E,EAAE,GAAK,GAEHzC,EAAJ1E,GAAS,CAGb,IAFAkB,EAAIiG,EAAEnH,KAAO,GACbqN,EAAIrL,EAAWd,EAAEE,OACTiM,IAAKnM,EAAI,IAAMA,GACvBqB,GAAKrB,EAIT,IAAMwD,EAAInC,EAAEnB,OAA8B,KAAtBmB,EAAEjB,aAAaoD,KACnC,MAAOnC,GAAExB,MAAO,EAAG2D,EAAI,GAAK,GAKhC,QAASwE,GAAS9I,EAAGqC,GACjB,GAAI0E,GAAGtH,EACH2C,EAAKpC,EAAEN,EACPsK,EAAK3H,EAAE3C,EACPE,EAAII,EAAEc,EACNwD,EAAIjC,EAAEvB,EACNoB,EAAIlC,EAAEL,EACN6P,EAAInN,EAAE1C,CAGV,KAAMC,IAAM0E,EAAI,MAAO,KAMvB,IAJAyC,EAAI3E,IAAOA,EAAG,GACd3C,EAAIuK,IAAOA,EAAG,GAGTjD,GAAKtH,EAAI,MAAOsH,GAAItH,EAAI,GAAK6E,EAAI1E,CAGtC,IAAKA,GAAK0E,EAAI,MAAO1E,EAMrB,IAJAmH,EAAQ,EAAJnH,EACJH,EAAIyC,GAAKsN,GAGHpN,IAAO4H,EAAK,MAAOvK,GAAI,GAAK2C,EAAK2E,EAAI,EAAI,EAG/C,KAAMtH,EAAI,MAAOyC,GAAIsN,EAAIzI,EAAI,EAAI,EAKjC,KAHAzC,GAAMpC,EAAIE,EAAGpB,SAAawO,EAAIxF,EAAGhJ,QAAWkB,EAAIsN,EAG1C5P,EAAI,EAAO0E,EAAJ1E,EAAOA,IAAM,GAAKwC,EAAGxC,IAAMoK,EAAGpK,GAAK,MAAOwC,GAAGxC,GAAKoK,EAAGpK,GAAKmH,EAAI,EAAI,EAG/E,OAAO7E,IAAKsN,EAAI,EAAItN,EAAIsN,EAAIzI,EAAI,EAAI,GASxC,QAASM,GAAsB7H,EAAGyE,EAAKC,GACnC,OAAS1E,EAAI4E,EAAS5E,KAAQyE,GAAYC,GAAL1E,EAIzC,QAASsE,GAAQ2L,GACb,MAA8C,kBAAvCC,OAAOtK,UAAU7B,SAASQ,KAAK0L,GAI1C,QAAS/H,GAAYb,GACnB,SAAWA,IAAKA,EAAE8I,aAAe9I,EAAE8I,YAAYjI,cAAgBA,GASjE,QAAS/E,GAAW5C,EAAKgC,EAAQD,GAO7B,IANA,GAAIwC,GAEAsL,EADA5B,GAAO,GAEPpO,EAAI,EACJE,EAAMC,EAAIiB,OAEFlB,EAAJF,GAAW,CACf,IAAMgQ,EAAO5B,EAAIhN,OAAQ4O,IAAQ5B,EAAI4B,IAAS7N,GAG9C,IAFAiM,EAAK1J,EAAI,IAAO5D,EAASW,QAAStB,EAAIkD,OAAQrD,MAEtC0E,EAAI0J,EAAIhN,OAAQsD,IAEf0J,EAAI1J,GAAKxC,EAAU,IACD,MAAdkM,EAAI1J,EAAI,KAAa0J,EAAI1J,EAAI,GAAK,GACvC0J,EAAI1J,EAAI,IAAM0J,EAAI1J,GAAKxC,EAAU,EACjCkM,EAAI1J,IAAMxC,GAKtB,MAAOkM,GAAIvB,UAIf,QAAShJ,GAAe1D,EAAKJ,GACzB,OAASI,EAAIiB,OAAS,EAAIjB,EAAIkD,OAAO,GAAK,IAAMlD,EAAIY,MAAM,GAAKZ,IACvD,EAAJJ,EAAQ,IAAM,MAASA,EAI/B,QAASiD,GAAc7C,EAAKJ,GACxB,GAAIG,GAAKmN,CAGT,IAAS,EAAJtN,EAAQ,CAGT,IAAMsN,EAAI,OAAQtN,EAAGsN,GAAK,KAC1BlN,EAAMkN,EAAIlN,MAOV,IAHAD,EAAMC,EAAIiB,SAGHrB,EAAIG,EAAM,CACb,IAAMmN,EAAI,IAAKtN,GAAKG,IAAOH,EAAGsN,GAAK,KACnClN,GAAOkN,MACKnN,GAAJH,IACRI,EAAMA,EAAIY,MAAO,EAAGhB,GAAM,IAAMI,EAAIY,MAAMhB,GAIlD,OAAOI,GAIX,QAASqE,GAAS5E,GAEd,MADAA,GAAI0P,WAAW1P,GACJ,EAAJA,EAAQyF,EAASzF,GAAKiC,EAAUjC,GAlpF3C,GAAID,GACA6B,EAAY,uCACZ6D,EAAW+C,KAAK6C,KAChBpJ,EAAYuG,KAAKqD,MACjB/D,EAAU,iCACVhE,EAAe,gBACfrC,EAAgB,kDAChBP,EAAW,mEACXwE,EAAO,KACPtD,EAAW,GACXJ,EAAmB,iBAEnBuD,GAAY,EAAG,GAAI,IAAK,IAAK,IAAK,IAAK,IAAK,IAAK,IAAK,IAAK,KAAM,KAAM,KAAM,MAC7E6D,EAAY,IAOZxB,EAAM,GAqoFV7H,GAAYF,IACZE,EAAAA,WAAoBA,EAAUA,UAAYA,EAIpB,kBAAVsQ,SAAwBA,OAAOC,IACvCD,OAAQ,WAAc,MAAOtQ,KAGJ,mBAAVwQ,SAAyBA,OAAOC,QAC/CD,OAAOC,QAAUzQ,GAIXH,IAAYA,EAA2B,mBAAR6Q,MAAsBA,KAAOC,SAAS,kBAC3E9Q,EAAUG,UAAYA,IAE3BU"}
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/bignumber.min.js
/* bignumber.js v3.1.2 https://github.com/MikeMcl/bignumber.js/LICENCE */
!function(e){"use strict";function n(e){function h(e,n){var t,r,i,o,u,s,f=this;if(!(f instanceof h))return V&&I(26,"constructor call without new",e),new h(e,n);if(null!=n&&j(n,2,64,C,"base")){if(n=0|n,s=e+"",10==n)return f=new h(e instanceof h?e:s),L(f,P+f.e+1,q);if((o="number"==typeof e)&&0*e!=0||!new RegExp("^-?"+(t="["+N.slice(0,n)+"]+")+"(?:\\."+t+")?$",37>n?"i":"").test(s))return B(f,s,o,n);o?(f.s=0>1/e?(s=s.slice(1),-1):1,V&&s.replace(/^0\.0*|\./,"").length>15&&I(C,v,e),o=!1):f.s=45===s.charCodeAt(0)?(s=s.slice(1),-1):1,s=E(s,10,n,f.s)}else{if(e instanceof h)return f.s=e.s,f.e=e.e,f.c=(e=e.c)?e.slice():e,void(C=0);if((o="number"==typeof e)&&0*e==0){if(f.s=0>1/e?(e=-e,-1):1,e===~~e){for(r=0,i=e;i>=10;i/=10,r++);return f.e=r,f.c=[e],void(C=0)}s=e+""}else{if(!g.test(s=e+""))return B(f,s,o);f.s=45===s.charCodeAt(0)?(s=s.slice(1),-1):1}}for((r=s.indexOf("."))>-1&&(s=s.replace(".","")),(i=s.search(/e/i))>0?(0>r&&(r=i),r+=+s.slice(i+1),s=s.substring(0,i)):0>r&&(r=s.length),i=0;48===s.charCodeAt(i);i++);for(u=s.length;48===s.charCodeAt(--u););if(s=s.slice(i,u+1))if(u=s.length,o&&V&&u>15&&(e>O||e!==d(e))&&I(C,v,f.s*e),r=r-i-1,r>z)f.c=f.e=null;else if(G>r)f.c=[f.e=0];else{if(f.e=r,f.c=[],i=(r+1)%y,0>r&&(i+=y),u>i){for(i&&f.c.push(+s.slice(0,i)),u-=y;u>i;)f.c.push(+s.slice(i,i+=y));s=s.slice(i),i=y-s.length}else i-=u;for(;i--;s+="0");f.c.push(+s)}else f.c=[f.e=0];C=0}function E(e,n,t,i){var o,u,s,l,a,g,p,d=e.indexOf("."),m=P,w=q;for(37>t&&(e=e.toLowerCase()),d>=0&&(s=J,J=0,e=e.replace(".",""),p=new h(t),a=p.pow(e.length-d),J=s,p.c=f(c(r(a.c),a.e),10,n),p.e=p.c.length),g=f(e,t,n),u=s=g.length;0==g[--s];g.pop());if(!g[0])return"0";if(0>d?--u:(a.c=g,a.e=u,a.s=i,a=U(a,p,m,w,n),g=a.c,l=a.r,u=a.e),o=u+m+1,d=g[o],s=n/2,l=l||0>o||null!=g[o+1],l=4>w?(null!=d||l)&&(0==w||w==(a.s<0?3:2)):d>s||d==s&&(4==w||l||6==w&&1&g[o-1]||w==(a.s<0?8:7)),1>o||!g[0])e=l?c("1",-m):"0";else{if(g.length=o,l)for(--n;++g[--o]>n;)g[o]=0,o||(++u,g.unshift(1));for(s=g.length;!g[--s];);for(d=0,e="";s>=d;e+=N.charAt(g[d++]));e=c(e,u)}return e}function D(e,n,t,i){var o,u,s,f,a;if(t=null!=t&&j(t,0,8,i,w)?0|t:q,!e.c)return e.toString();if(o=e.c[0],s=e.e,null==n)a=r(e.c),a=19==i||24==i&&k>=s?l(a,s):c(a,s);else if(e=L(new h(e),n,t),u=e.e,a=r(e.c),f=a.length,19==i||24==i&&(u>=n||k>=u)){for(;n>f;a+="0",f++);a=l(a,u)}else if(n-=s,a=c(a,u),u+1>f){if(--n>0)for(a+=".";n--;a+="0");}else if(n+=u-f,n>0)for(u+1==f&&(a+=".");n--;a+="0");return e.s<0&&o?"-"+a:a}function F(e,n){var t,r,i=0;for(u(e[0])&&(e=e[0]),t=new h(e[0]);++i
e||e>t||e!=a(e))&&I(r,(i||"decimal places")+(n>e||e>t?" out of range":" not an integer"),e),!0}function x(e,n,t){for(var r=1,i=n.length;!n[--i];n.pop());for(i=n[0];i>=10;i/=10,r++);return(t=r+t*y-1)>z?e.c=e.e=null:G>t?e.c=[e.e=0]:(e.e=t,e.c=n),e}function I(e,n,t){var r=new Error(["new BigNumber","cmp","config","div","divToInt","eq","gt","gte","lt","lte","minus","mod","plus","precision","random","round","shift","times","toDigits","toExponential","toFixed","toFormat","toFraction","pow","toPrecision","toString","BigNumber"][e]+"() "+n+": "+t);throw r.name="BigNumber Error",C=0,r}function L(e,n,t,r){var i,o,u,s,f,l,c,a=e.c,h=R;if(a){e:{for(i=1,s=a[0];s>=10;s/=10,i++);if(o=n-i,0>o)o+=y,u=n,f=a[l=0],c=f/h[i-u-1]%10|0;else if(l=p((o+1)/y),l>=a.length){if(!r)break e;for(;a.length<=l;a.push(0));f=c=0,i=1,o%=y,u=o-y+1}else{for(f=s=a[l],i=1;s>=10;s/=10,i++);o%=y,u=o-y+i,c=0>u?0:f/h[i-u-1]%10|0}if(r=r||0>n||null!=a[l+1]||(0>u?f:f%h[i-u-1]),r=4>t?(c||r)&&(0==t||t==(e.s<0?3:2)):c>5||5==c&&(4==t||r||6==t&&(o>0?u>0?f/h[i-u]:0:a[l-1])%10&1||t==(e.s<0?8:7)),1>n||!a[0])return a.length=0,r?(n-=e.e+1,a[0]=h[(y-n%y)%y],e.e=-n||0):a[0]=e.e=0,e;if(0==o?(a.length=l,s=1,l--):(a.length=l+1,s=h[y-o],a[l]=u>0?d(f/h[i-u]%h[u])*s:0),r)for(;;){if(0==l){for(o=1,u=a[0];u>=10;u/=10,o++);for(u=a[0]+=s,s=1;u>=10;u/=10,s++);o!=s&&(e.e++,a[0]==b&&(a[0]=1));break}if(a[l]+=s,a[l]!=b)break;a[l--]=0,s=1}for(o=a.length;0===a[--o];a.pop());}e.e>z?e.c=e.e=null:e.et?null!=(e=i[t++]):void 0};return f(n="DECIMAL_PLACES")&&j(e,0,A,2,n)&&(P=0|e),r[n]=P,f(n="ROUNDING_MODE")&&j(e,0,8,2,n)&&(q=0|e),r[n]=q,f(n="EXPONENTIAL_AT")&&(u(e)?j(e[0],-A,0,2,n)&&j(e[1],0,A,2,n)&&(k=0|e[0],$=0|e[1]):j(e,-A,A,2,n)&&(k=-($=0|(0>e?-e:e)))),r[n]=[k,$],f(n="RANGE")&&(u(e)?j(e[0],-A,-1,2,n)&&j(e[1],1,A,2,n)&&(G=0|e[0],z=0|e[1]):j(e,-A,A,2,n)&&(0|e?G=-(z=0|(0>e?-e:e)):V&&I(2,n+" cannot be zero",e))),r[n]=[G,z],f(n="ERRORS")&&(e===!!e||1===e||0===e?(C=0,j=(V=!!e)?_:o):V&&I(2,n+m,e)),r[n]=V,f(n="CRYPTO")&&(e===!0||e===!1||1===e||0===e?e?(e="undefined"==typeof crypto,!e&&crypto&&(crypto.getRandomValues||crypto.randomBytes)?H=!0:V?I(2,"crypto unavailable",e?void 0:crypto):H=!1):H=!1:V&&I(2,n+m,e)),r[n]=H,f(n="MODULO_MODE")&&j(e,0,9,2,n)&&(W=0|e),r[n]=W,f(n="POW_PRECISION")&&j(e,0,A,2,n)&&(J=0|e),r[n]=J,f(n="FORMAT")&&("object"==typeof e?X=e:V&&I(2,n+" not an object",e)),r[n]=X,r},h.isBigNumber=s,h.max=function(){return F(arguments,M.lt)},h.min=function(){return F(arguments,M.gt)},h.random=function(){var e=9007199254740992,n=Math.random()*e&2097151?function(){return d(Math.random()*e)}:function(){return 8388608*(1073741824*Math.random()|0)+(8388608*Math.random()|0)};return function(e){var t,r,i,o,u,s=0,f=[],l=new h(T);if(e=null!=e&&j(e,0,A,14)?0|e:P,o=p(e/y),H)if(crypto.getRandomValues){for(t=crypto.getRandomValues(new Uint32Array(o*=2));o>s;)u=131072*t[s]+(t[s+1]>>>11),u>=9e15?(r=crypto.getRandomValues(new Uint32Array(2)),t[s]=r[0],t[s+1]=r[1]):(f.push(u%1e14),s+=2);s=o/2}else if(crypto.randomBytes){for(t=crypto.randomBytes(o*=7);o>s;)u=281474976710656*(31&t[s])+1099511627776*t[s+1]+4294967296*t[s+2]+16777216*t[s+3]+(t[s+4]<<16)+(t[s+5]<<8)+t[s+6],u>=9e15?crypto.randomBytes(7).copy(t,s):(f.push(u%1e14),s+=7);s=o/7}else H=!1,V&&I(14,"crypto unavailable",crypto);if(!H)for(;o>s;)u=n(),9e15>u&&(f[s++]=u%1e14);for(o=f[--s],e%=y,o&&e&&(u=R[y-e],f[s]=d(o/u)*u);0===f[s];f.pop(),s--);if(0>s)f=[i=0];else{for(i=-1;0===f[0];f.shift(),i-=y);for(s=1,u=f[0];u>=10;u/=10,s++);y>s&&(i-=y-s)}return l.e=i,l.c=f,l}}(),U=function(){function e(e,n,t){var r,i,o,u,s=0,f=e.length,l=n%S,c=n/S|0;for(e=e.slice();f--;)o=e[f]%S,u=e[f]/S|0,r=c*o+u*l,i=l*o+r%S*S+s,s=(i/t|0)+(r/S|0)+c*u,e[f]=i%t;return s&&e.unshift(s),e}function n(e,n,t,r){var i,o;if(t!=r)o=t>r?1:-1;else for(i=o=0;t>i;i++)if(e[i]!=n[i]){o=e[i]>n[i]?1:-1;break}return o}function r(e,n,t,r){for(var i=0;t--;)e[t]-=i,i=e[t]1;e.shift());}return function(i,o,u,s,f){var l,c,a,g,p,m,w,v,N,O,R,S,A,E,D,F,_,x=i.s==o.s?1:-1,I=i.c,U=o.c;if(!(I&&I[0]&&U&&U[0]))return new h(i.s&&o.s&&(I?!U||I[0]!=U[0]:U)?I&&0==I[0]||!U?0*x:x/0:NaN);for(v=new h(x),N=v.c=[],c=i.e-o.e,x=u+c+1,f||(f=b,c=t(i.e/y)-t(o.e/y),x=x/y|0),a=0;U[a]==(I[a]||0);a++);if(U[a]>(I[a]||0)&&c--,0>x)N.push(1),g=!0;else{for(E=I.length,F=U.length,a=0,x+=2,p=d(f/(U[0]+1)),p>1&&(U=e(U,p,f),I=e(I,p,f),F=U.length,E=I.length),A=F,O=I.slice(0,F),R=O.length;F>R;O[R++]=0);_=U.slice(),_.unshift(0),D=U[0],U[1]>=f/2&&D++;do{if(p=0,l=n(U,O,F,R),0>l){if(S=O[0],F!=R&&(S=S*f+(O[1]||0)),p=d(S/D),p>1)for(p>=f&&(p=f-1),m=e(U,p,f),w=m.length,R=O.length;1==n(m,O,w,R);)p--,r(m,w>F?_:U,w,f),w=m.length,l=1;else 0==p&&(l=p=1),m=U.slice(),w=m.length;if(R>w&&m.unshift(0),r(O,m,R,f),R=O.length,-1==l)for(;n(U,O,F,R)<1;)p++,r(O,R>F?_:U,R,f),R=O.length}else 0===l&&(p++,O=[0]);N[a++]=p,O[0]?O[R++]=I[A]||0:(O=[I[A]],R=1)}while((A++=10;x/=10,a++);L(v,u+(v.e=a+c*y-1)+1,s,g)}else v.e=c,v.r=+g;return v}}(),B=function(){var e=/^(-?)0([xbo])(?=\w[\w.]*$)/i,n=/^([^.]+)\.$/,t=/^\.([^.]+)$/,r=/^-?(Infinity|NaN)$/,i=/^\s*\+(?=[\w.])|^\s+|\s+$/g;return function(o,u,s,f){var l,c=s?u:u.replace(i,"");if(r.test(c))o.s=isNaN(c)?null:0>c?-1:1;else{if(!s&&(c=c.replace(e,function(e,n,t){return l="x"==(t=t.toLowerCase())?16:"b"==t?2:8,f&&f!=l?e:n}),f&&(l=f,c=c.replace(n,"$1").replace(t,"0.$1")),u!=c))return new h(c,l);V&&I(C,"not a"+(f?" base "+f:"")+" number",u),o.s=null}o.c=o.e=null,C=0}}(),M.absoluteValue=M.abs=function(){var e=new h(this);return e.s<0&&(e.s=1),e},M.ceil=function(){return L(new h(this),this.e+1,2)},M.comparedTo=M.cmp=function(e,n){return C=1,i(this,new h(e,n))},M.decimalPlaces=M.dp=function(){var e,n,r=this.c;if(!r)return null;if(e=((n=r.length-1)-t(this.e/y))*y,n=r[n])for(;n%10==0;n/=10,e--);return 0>e&&(e=0),e},M.dividedBy=M.div=function(e,n){return C=3,U(this,new h(e,n),P,q)},M.dividedToIntegerBy=M.divToInt=function(e,n){return C=4,U(this,new h(e,n),0,1)},M.equals=M.eq=function(e,n){return C=5,0===i(this,new h(e,n))},M.floor=function(){return L(new h(this),this.e+1,3)},M.greaterThan=M.gt=function(e,n){return C=6,i(this,new h(e,n))>0},M.greaterThanOrEqualTo=M.gte=function(e,n){return C=7,1===(n=i(this,new h(e,n)))||0===n},M.isFinite=function(){return!!this.c},M.isInteger=M.isInt=function(){return!!this.c&&t(this.e/y)>this.c.length-2},M.isNaN=function(){return!this.s},M.isNegative=M.isNeg=function(){return this.s<0},M.isZero=function(){return!!this.c&&0==this.c[0]},M.lessThan=M.lt=function(e,n){return C=8,i(this,new h(e,n))<0},M.lessThanOrEqualTo=M.lte=function(e,n){return C=9,-1===(n=i(this,new h(e,n)))||0===n},M.minus=M.sub=function(e,n){var r,i,o,u,s=this,f=s.s;if(C=10,e=new h(e,n),n=e.s,!f||!n)return new h(NaN);if(f!=n)return e.s=-n,s.plus(e);var l=s.e/y,c=e.e/y,a=s.c,g=e.c;if(!l||!c){if(!a||!g)return a?(e.s=-n,e):new h(g?s:NaN);if(!a[0]||!g[0])return g[0]?(e.s=-n,e):new h(a[0]?s:3==q?-0:0)}if(l=t(l),c=t(c),a=a.slice(),f=l-c){for((u=0>f)?(f=-f,o=a):(c=l,o=g),o.reverse(),n=f;n--;o.push(0));o.reverse()}else for(i=(u=(f=a.length)<(n=g.length))?f:n,f=n=0;i>n;n++)if(a[n]!=g[n]){u=a[n]0)for(;n--;a[r++]=0);for(n=b-1;i>f;){if(a[--i]0?(s=u,r=l):(o=-o,r=f),r.reverse();o--;r.push(0));r.reverse()}for(o=f.length,n=l.length,0>o-n&&(r=l,l=f,f=r,n=o),o=0;n;)o=(f[--n]=f[n]+l[n]+o)/b|0,f[n]=b===f[n]?0:f[n]%b;return o&&(f.unshift(o),++s),x(e,f,s)},M.precision=M.sd=function(e){var n,t,r=this,i=r.c;if(null!=e&&e!==!!e&&1!==e&&0!==e&&(V&&I(13,"argument"+m,e),e!=!!e&&(e=null)),!i)return null;if(t=i.length-1,n=t*y+1,t=i[t]){for(;t%10==0;t/=10,n--);for(t=i[0];t>=10;t/=10,n++);}return e&&r.e+1>n&&(n=r.e+1),n},M.round=function(e,n){var t=new h(this);return(null==e||j(e,0,A,15))&&L(t,~~e+this.e+1,null!=n&&j(n,0,8,15,w)?0|n:q),t},M.shift=function(e){var n=this;return j(e,-O,O,16,"argument")?n.times("1e"+a(e)):new h(n.c&&n.c[0]&&(-O>e||e>O)?n.s*(0>e?0:1/0):n)},M.squareRoot=M.sqrt=function(){var e,n,i,o,u,s=this,f=s.c,l=s.s,c=s.e,a=P+4,g=new h("0.5");if(1!==l||!f||!f[0])return new h(!l||0>l&&(!f||f[0])?NaN:f?s:1/0);if(l=Math.sqrt(+s),0==l||l==1/0?(n=r(f),(n.length+c)%2==0&&(n+="0"),l=Math.sqrt(n),c=t((c+1)/2)-(0>c||c%2),l==1/0?n="1e"+c:(n=l.toExponential(),n=n.slice(0,n.indexOf("e")+1)+c),i=new h(n)):i=new h(l+""),i.c[0])for(c=i.e,l=c+a,3>l&&(l=0);;)if(u=i,i=g.times(u.plus(U(s,u,a,1))),r(u.c).slice(0,l)===(n=r(i.c)).slice(0,l)){if(i.el&&(m=O,O=R,R=m,o=l,l=g,g=o),o=l+g,m=[];o--;m.push(0));for(w=b,v=S,o=g;--o>=0;){for(r=0,p=R[o]%v,d=R[o]/v|0,s=l,u=o+s;u>o;)c=O[--s]%v,a=O[s]/v|0,f=d*c+a*p,c=p*c+f%v*v+m[u]+r,r=(c/w|0)+(f/v|0)+d*a,m[u--]=c%w;m[u]=r}return r?++i:m.shift(),x(e,m,i)},M.toDigits=function(e,n){var t=new h(this);return e=null!=e&&j(e,1,A,18,"precision")?0|e:null,n=null!=n&&j(n,0,8,18,w)?0|n:q,e?L(t,e,n):t},M.toExponential=function(e,n){return D(this,null!=e&&j(e,0,A,19)?~~e+1:null,n,19)},M.toFixed=function(e,n){return D(this,null!=e&&j(e,0,A,20)?~~e+this.e+1:null,n,20)},M.toFormat=function(e,n){var t=D(this,null!=e&&j(e,0,A,21)?~~e+this.e+1:null,n,21);if(this.c){var r,i=t.split("."),o=+X.groupSize,u=+X.secondaryGroupSize,s=X.groupSeparator,f=i[0],l=i[1],c=this.s<0,a=c?f.slice(1):f,h=a.length;if(u&&(r=o,o=u,u=r,h-=r),o>0&&h>0){for(r=h%o||o,f=a.substr(0,r);h>r;r+=o)f+=s+a.substr(r,o);u>0&&(f+=s+a.slice(r)),c&&(f="-"+f)}t=l?f+X.decimalSeparator+((u=+X.fractionGroupSize)?l.replace(new RegExp("\\d{"+u+"}\\B","g"),"$&"+X.fractionGroupSeparator):l):f}return t},M.toFraction=function(e){var n,t,i,o,u,s,f,l,c,a=V,g=this,p=g.c,d=new h(T),m=t=new h(T),w=f=new h(T);if(null!=e&&(V=!1,s=new h(e),V=a,(!(a=s.isInt())||s.lt(T))&&(V&&I(22,"max denominator "+(a?"out of range":"not an integer"),e),e=!a&&s.c&&L(s,s.e+1,1).gte(T)?s:null)),!p)return g.toString();for(c=r(p),o=d.e=c.length-g.e-1,d.c[0]=R[(u=o%y)<0?y+u:u],e=!e||s.cmp(d)>0?o>0?d:m:s,u=z,z=1/0,s=new h(c),f.c[0]=0;l=U(s,d,0,1),i=t.plus(l.times(w)),1!=i.cmp(e);)t=w,w=i,m=f.plus(l.times(i=m)),f=i,d=s.minus(l.times(i=d)),s=i;return i=U(e.minus(t),w,0,1),f=f.plus(i.times(m)),t=t.plus(i.times(w)),f.s=m.s=g.s,o*=2,n=U(m,w,o,q).minus(g).abs().cmp(U(f,t,o,q).minus(g).abs())<1?[m.toString(),w.toString()]:[f.toString(),t.toString()],z=u,n},M.toNumber=function(){return+this},M.toPower=M.pow=function(e,n){var t,r,i,o=d(0>e?-e:+e),u=this;if(null!=n&&(C=23,n=new h(n)),!j(e,-O,O,23,"exponent")&&(!isFinite(e)||o>O&&(e/=0)||parseFloat(e)!=e&&!(e=NaN))||0==e)return t=Math.pow(+u,e),new h(n?t%n:t);for(n?e>1&&u.gt(T)&&u.isInt()&&n.gt(T)&&n.isInt()?u=u.mod(n):(i=n,n=null):J&&(t=p(J/y+2)),r=new h(T);;){if(o%2){if(r=r.times(u),!r.c)break;t?r.c.length>t&&(r.c.length=t):n&&(r=r.mod(n))}if(o=d(o/2),!o)break;u=u.times(u),t?u.c&&u.c.length>t&&(u.c.length=t):n&&(u=u.mod(n))}return n?r:(0>e&&(r=T.div(r)),i?r.mod(i):t?L(r,J,q):r)},M.toPrecision=function(e,n){return D(this,null!=e&&j(e,1,A,24,"precision")?0|e:null,n,24)},M.toString=function(e){var n,t=this,i=t.s,o=t.e;return null===o?i?(n="Infinity",0>i&&(n="-"+n)):n="NaN":(n=r(t.c),n=null!=e&&j(e,2,64,25,"base")?E(c(n,o),0|e,10,i):k>=o||o>=$?l(n,o):c(n,o),0>i&&t.c[0]&&(n="-"+n)),n},M.truncated=M.trunc=function(){return L(new h(this),this.e+1,1)},M.valueOf=M.toJSON=function(){var e,n=this,t=n.e;return null===t?n.toString():(e=r(n.c),e=k>=t||t>=$?l(e,t):c(e,t),n.s<0?"-"+e:e)},null!=e&&h.config(e),h}function t(e){var n=0|e;return e>0||e===n?n:n-1}function r(e){for(var n,t,r=1,i=e.length,o=e[0]+"";i>r;){for(n=e[r++]+"",t=y-n.length;t--;n="0"+n);o+=n}for(i=o.length;48===o.charCodeAt(--i););return o.slice(0,i+1||1)}function i(e,n){var t,r,i=e.c,o=n.c,u=e.s,s=n.s,f=e.e,l=n.e;if(!u||!s)return null;if(t=i&&!i[0],r=o&&!o[0],t||r)return t?r?0:-s:u;if(u!=s)return u;if(t=0>u,r=f==l,!i||!o)return r?0:!i^t?1:-1;if(!r)return f>l^t?1:-1;for(s=(f=i.length)<(l=o.length)?f:l,u=0;s>u;u++)if(i[u]!=o[u])return i[u]>o[u]^t?1:-1;return f==l?0:f>l^t?1:-1}function o(e,n,t){return(e=a(e))>=n&&t>=e}function u(e){return"[object Array]"==Object.prototype.toString.call(e)}function s(e){return!(!e||!e.constructor||e.constructor.isBigNumber!==s)}function f(e,n,t){for(var r,i,o=[0],u=0,s=e.length;s>u;){for(i=o.length;i--;o[i]*=n);for(o[r=0]+=N.indexOf(e.charAt(u++));rt-1&&(null==o[r+1]&&(o[r+1]=0),o[r+1]+=o[r]/t|0,o[r]%=t)}return o.reverse()}function l(e,n){return(e.length>1?e.charAt(0)+"."+e.slice(1):e)+(0>n?"e":"e+")+n}function c(e,n){var t,r;if(0>n){for(r="0.";++n;r+="0");e=r+e}else if(t=e.length,++n>t){for(r="0",n-=t;--n;r+="0");e+=r}else t>n&&(e=e.slice(0,n)+"."+e.slice(n));return e}function a(e){return e=parseFloat(e),0>e?p(e):d(e)}var h,g=/^-?(\d+(\.\d*)?|\.\d+)(e[+-]?\d+)?$/i,p=Math.ceil,d=Math.floor,m=" not a boolean or binary digit",w="rounding mode",v="number type has more than 15 significant digits",N="0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ$_",b=1e14,y=14,O=9007199254740991,R=[1,10,100,1e3,1e4,1e5,1e6,1e7,1e8,1e9,1e10,1e11,1e12,1e13],S=1e7,A=1e9;h=n(),h["default"]=h.BigNumber=h,"function"==typeof define&&define.amd?define(function(){return h}):"undefined"!=typeof module&&module.exports?module.exports=h:(e||(e="undefined"!=typeof self?self:Function("return this")()),e.BigNumber=h)}(this);
//# sourceMappingURL=bignumber.js.map
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/bower.json
{
"name": "bignumber.js",
"main": "bignumber.js",
"version": "3.1.2",
"homepage": "https://github.com/MikeMcl/bignumber.js",
"authors": [
"Michael Mclaughlin "
],
"description": "A library for arbitrary-precision decimal and non-decimal arithmetic",
"moduleType": [
"amd",
"globals",
"node"
],
"keywords": [
"arbitrary",
"precision",
"arithmetic",
"big",
"number",
"decimal",
"float",
"biginteger",
"bigdecimal",
"bignumber",
"bigint",
"bignum"
],
"license": "MIT",
"ignore": [
".*",
"*.json",
"test"
]
}
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/doc/API.html
API
CONSTRUCTOR
BigNumber
Methods
another
config
DECIMAL_PLACES
ROUNDING_MODE
EXPONENTIAL_AT
RANGE
ERRORS
CRYPTO
MODULO_MODE
POW_PRECISION
FORMAT
isBigNumber
max
min
random
Properties
ROUND_UP
ROUND_DOWN
ROUND_CEIL
ROUND_FLOOR
ROUND_HALF_UP
ROUND_HALF_DOWN
ROUND_HALF_EVEN
ROUND_HALF_CEIL
ROUND_HALF_FLOOR
INSTANCE
Methods
absoluteValue abs
ceil
comparedTo cmp
decimalPlaces dp
dividedBy div
dividedToIntegerBy divToInt
equals eq
floor
greaterThan gt
greaterThanOrEqualTogte
isFinite
isInteger isInt
isNaN
isNegative isNeg
isZero
lessThan lt
lessThanOrEqualTo lte
minus sub
modulo mod
negated neg
plus add
precision sd
round
shift
squareRoot sqrt
times mul
toDigits
toExponential
toFixed
toFormat
toFraction
toJSON
toNumber
toPower pow
toPrecision
toString
truncated trunc
valueOf
Properties
c: coefficient
e: exponent
s: sign
Zero, NaN & Infinity
Errors
FAQ
bignumber.js
A JavaScript library for arbitrary-precision arithmetic.
Hosted on GitHub.
API
See the README on GitHub for a
quick-start introduction.
In all examples below, var and semicolons are not shown, and if a commented-out
value is in quotes it means toString has been called on the preceding expression.
CONSTRUCTOR
BigNumberBigNumber(value [, base]) ⇒ BigNumber
value
number|string|BigNumber: see RANGE for
range.
A numeric value.
Legitimate values include ±0, ±Infinity and
NaN.
Values of type number with more than 15 significant digits are
considered invalid (if ERRORS is true) as calling
toString or valueOf on
such numbers may not result in the intended value.
console.log( 823456789123456.3 ); // 823456789123456.2
There is no limit to the number of digits of a value of type string (other than
that of JavaScript's maximum array size).
Decimal string values may be in exponential, as well as normal (fixed-point) notation.
Non-decimal values must be in normal notation.
String values in hexadecimal literal form, e.g. '0xff', are valid, as are
string values with the octal and binary prefixs '0o' and '0b'.
String values in octal literal form without the prefix will be interpreted as
decimals, e.g. '011' is interpreted as 11, not 9.
Values in any base may have fraction digits.
For bases from 10 to 36, lower and/or upper case letters can be
used to represent values from 10 to 35.
For bases above 36, a-z represents values from 10 to
35, A-Z from 36 to 61, and
$ and _ represent 62 and 63 respectively
(this can be changed by editing the ALPHABET variable near the top of the
source file).
base
number: integer, 2 to 64 inclusive
The base of value.
If base is omitted, or is null or undefined, base
10 is assumed.
Returns a new instance of a BigNumber object.
If a base is specified, the value is rounded according to
the current DECIMAL_PLACES and
ROUNDING_MODE configuration.
See Errors for the treatment of an invalid value or
base.
x = new BigNumber(9) // '9'
y = new BigNumber(x) // '9'
// 'new' is optional if ERRORS is false
BigNumber(435.345) // '435.345'
new BigNumber('5032485723458348569331745.33434346346912144534543')
new BigNumber('4.321e+4') // '43210'
new BigNumber('-735.0918e-430') // '-7.350918e-428'
new BigNumber(Infinity) // 'Infinity'
new BigNumber(NaN) // 'NaN'
new BigNumber('.5') // '0.5'
new BigNumber('+2') // '2'
new BigNumber(-10110100.1, 2) // '-180.5'
new BigNumber(-0b10110100.1) // '-180.5'
new BigNumber('123412421.234324', 5) // '607236.557696'
new BigNumber('ff.8', 16) // '255.5'
new BigNumber('0xff.8') // '255.5'
The following throws 'not a base 2 number' if
ERRORS is true, otherwise it returns a BigNumber with value
NaN.
new BigNumber(9, 2)
The following throws 'number type has more than 15 significant digits' if
ERRORS is true, otherwise it returns a BigNumber with value
96517860459076820.
new BigNumber(96517860459076817.4395)
The following throws 'not a number' if ERRORS
is true, otherwise it returns a BigNumber with value NaN.
new BigNumber('blurgh')
A value is only rounded by the constructor if a base is specified.
BigNumber.config({ DECIMAL_PLACES: 5 })
new BigNumber(1.23456789) // '1.23456789'
new BigNumber(1.23456789, 10) // '1.23457'
Methods
The static methods of a BigNumber constructor.
another.another([obj]) ⇒ BigNumber constructor
obj: object
Returns a new independent BigNumber constructor with configuration as described by
obj (see config), or with the default
configuration if obj is null or undefined.
BigNumber.config({ DECIMAL_PLACES: 5 })
BN = BigNumber.another({ DECIMAL_PLACES: 9 })
x = new BigNumber(1)
y = new BN(1)
x.div(3) // 0.33333
y.div(3) // 0.333333333
// BN = BigNumber.another({ DECIMAL_PLACES: 9 }) is equivalent to:
BN = BigNumber.another()
BN.config({ DECIMAL_PLACES: 9 })
configset([obj]) ⇒ object
obj: object: an object that contains some or all of the following
properties.
Configures the settings for this particular BigNumber constructor.
Note: the configuration can also be supplied as an argument list, see below.
DECIMAL_PLACES
number: integer, 0 to 1e+9 inclusive
Default value: 20
The maximum number of decimal places of the results of operations involving
division, i.e. division, square root and base conversion operations, and power
operations with negative exponents.
BigNumber.config({ DECIMAL_PLACES: 5 })
BigNumber.set({ DECIMAL_PLACES: 5 }) // equivalent
BigNumber.config(5) // equivalent
ROUNDING_MODE
number: integer, 0 to 8 inclusive
Default value: 4 (ROUND_HALF_UP)
The rounding mode used in the above operations and the default rounding mode of
round,
toExponential,
toFixed,
toFormat and
toPrecision.
The modes are available as enumerated properties of the BigNumber constructor.
BigNumber.config({ ROUNDING_MODE: 0 })
BigNumber.config(null, BigNumber.ROUND_UP) // equivalent
EXPONENTIAL_AT
number: integer, magnitude 0 to 1e+9 inclusive, or
number[]: [ integer -1e+9 to 0 inclusive, integer
0 to 1e+9 inclusive ]
Default value: [-7, 20]
The exponent value(s) at which toString returns exponential notation.
If a single number is assigned, the value is the exponent magnitude.
If an array of two numbers is assigned then the first number is the negative exponent
value at and beneath which exponential notation is used, and the second number is the
positive exponent value at and above which the same.
For example, to emulate JavaScript numbers in terms of the exponent values at which they
begin to use exponential notation, use [-7, 20].
BigNumber.config({ EXPONENTIAL_AT: 2 })
new BigNumber(12.3) // '12.3' e is only 1
new BigNumber(123) // '1.23e+2'
new BigNumber(0.123) // '0.123' e is only -1
new BigNumber(0.0123) // '1.23e-2'
BigNumber.config({ EXPONENTIAL_AT: [-7, 20] })
new BigNumber(123456789) // '123456789' e is only 8
new BigNumber(0.000000123) // '1.23e-7'
// Almost never return exponential notation:
BigNumber.config({ EXPONENTIAL_AT: 1e+9 })
// Always return exponential notation:
BigNumber.config({ EXPONENTIAL_AT: 0 })
Regardless of the value of EXPONENTIAL_AT, the toFixed method
will always return a value in normal notation and the toExponential method
will always return a value in exponential form.
Calling toString with a base argument, e.g. toString(10), will
also always return normal notation.
RANGE
number: integer, magnitude 1 to 1e+9 inclusive, or
number[]: [ integer -1e+9 to -1 inclusive, integer
1 to 1e+9 inclusive ]
Default value: [-1e+9, 1e+9]
The exponent value(s) beyond which overflow to Infinity and underflow to
zero occurs.
If a single number is assigned, it is the maximum exponent magnitude: values wth a
positive exponent of greater magnitude become Infinity and those with a
negative exponent of greater magnitude become zero.
If an array of two numbers is assigned then the first number is the negative exponent
limit and the second number is the positive exponent limit.
For example, to emulate JavaScript numbers in terms of the exponent values at which they
become zero and Infinity, use [-324, 308].
BigNumber.config({ RANGE: 500 })
BigNumber.config().RANGE // [ -500, 500 ]
new BigNumber('9.999e499') // '9.999e+499'
new BigNumber('1e500') // 'Infinity'
new BigNumber('1e-499') // '1e-499'
new BigNumber('1e-500') // '0'
BigNumber.config({ RANGE: [-3, 4] })
new BigNumber(99999) // '99999' e is only 4
new BigNumber(100000) // 'Infinity' e is 5
new BigNumber(0.001) // '0.01' e is only -3
new BigNumber(0.0001) // '0' e is -4
The largest possible magnitude of a finite BigNumber is
9.999...e+1000000000.
The smallest possible magnitude of a non-zero BigNumber is 1e-1000000000.
ERRORS
boolean|number: true, false, 0 or
1.
Default value: true
The value that determines whether BigNumber Errors are thrown.
If ERRORS is false, no errors will be thrown.
See Errors.
BigNumber.config({ ERRORS: false })
CRYPTO
boolean|number: true, false, 0 or
1.
Default value: false
The value that determines whether cryptographically-secure pseudo-random number
generation is used.
If CRYPTO is set to true then the
random method will generate random digits using
crypto.getRandomValues in browsers that support it, or
crypto.randomBytes if using a version of Node.js that supports it.
If neither function is supported by the host environment then attempting to set
CRYPTO to true will fail, and if ERRORS
is true an exception will be thrown.
If CRYPTO is false then the source of randomness used will be
Math.random (which is assumed to generate at least 30 bits of
randomness).
See random.
BigNumber.config({ CRYPTO: true })
BigNumber.config().CRYPTO // true
BigNumber.random() // 0.54340758610486147524
MODULO_MODE
number: integer, 0 to 9 inclusive
Default value: 1 (ROUND_DOWN)
The modulo mode used when calculating the modulus: a mod n.
The quotient, q = a / n, is calculated according to the
ROUNDING_MODE that corresponds to the chosen
MODULO_MODE.
The remainder, r, is calculated as: r = a - n * q.
The modes that are most commonly used for the modulus/remainder operation are shown in
the following table. Although the other rounding modes can be used, they may not give
useful results.
Property Value Description
ROUND_UP 0
The remainder is positive if the dividend is negative, otherwise it is negative.
ROUND_DOWN 1
The remainder has the same sign as the dividend.
This uses 'truncating division' and matches the behaviour of JavaScript's
remainder operator %.
ROUND_FLOOR 3
The remainder has the same sign as the divisor.
This matches Python's % operator.
ROUND_HALF_EVEN 6 The IEEE 754 remainder function.
EUCLID 9
The remainder is always positive. Euclidian division:
q = sign(n) * floor(a / abs(n))
The rounding/modulo modes are available as enumerated properties of the BigNumber
constructor.
See modulo.
BigNumber.config({ MODULO_MODE: BigNumber.EUCLID })
BigNumber.config({ MODULO_MODE: 9 }) // equivalent
POW_PRECISION
number: integer, 0 to 1e+9 inclusive.
Default value: 0
The maximum number of significant digits of the result of the power operation
(unless a modulus is specified).
If set to 0, the number of signifcant digits will not be limited.
See toPower.
BigNumber.config({ POW_PRECISION: 100 })
FORMAT
object
The FORMAT object configures the format of the string returned by the
toFormat method.
The example below shows the properties of the FORMAT object that are
recognised, and their default values.
Unlike the other configuration properties, the values of the properties of the
FORMAT object will not be checked for validity. The existing
FORMAT object will simply be replaced by the object that is passed in.
Note that all the properties shown below do not have to be included.
See toFormat for examples of usage.
BigNumber.config({
FORMAT: {
// the decimal separator
decimalSeparator: '.',
// the grouping separator of the integer part
groupSeparator: ',',
// the primary grouping size of the integer part
groupSize: 3,
// the secondary grouping size of the integer part
secondaryGroupSize: 0,
// the grouping separator of the fraction part
fractionGroupSeparator: ' ',
// the grouping size of the fraction part
fractionGroupSize: 0
}
});
Returns an object with the above properties and their current values.
If the value to be assigned to any of the above properties is null or
undefined it is ignored.
See Errors for the treatment of invalid values.
BigNumber.config({
DECIMAL_PLACES: 40,
ROUNDING_MODE: BigNumber.ROUND_HALF_CEIL,
EXPONENTIAL_AT: [-10, 20],
RANGE: [-500, 500],
ERRORS: true,
CRYPTO: true,
MODULO_MODE: BigNumber.ROUND_FLOOR,
POW_PRECISION: 80,
FORMAT: {
groupSize: 3,
groupSeparator: ' ',
decimalSeparator: ','
}
});
// Alternatively but equivalently (excluding FORMAT):
BigNumber.config( 40, 7, [-10, 20], 500, 1, 1, 3, 80 )
obj = BigNumber.config();
obj.ERRORS // true
obj.RANGE // [-500, 500]
isBigNumber.isBigNumber(n) ⇒ boolean
n: any
Returns true if n is a BigNumber instance, otherwise returns
false.
x = 42
y = new BigNumber(x)
BigNumber.isBigNumber(x) // false
BigNumber.isBigNumber(y) // true
BN = BigNumber.another();
z = new BN(x)
BigNumber.isBigNumber(z) // true
max.max([arg1 [, arg2, ...]]) ⇒ BigNumber
arg1, arg2, ...: number|string|BigNumber
See BigNumber for further parameter details.
Returns a BigNumber whose value is the maximum of arg1,
arg2,... .
The argument to this method can also be an array of values.
The return value is always exact and unrounded.
x = new BigNumber('3257869345.0378653')
BigNumber.max(4e9, x, '123456789.9') // '4000000000'
arr = [12, '13', new BigNumber(14)]
BigNumber.max(arr) // '14'
min.min([arg1 [, arg2, ...]]) ⇒ BigNumber
arg1, arg2, ...: number|string|BigNumber
See BigNumber for further parameter details.
Returns a BigNumber whose value is the minimum of arg1,
arg2,... .
The argument to this method can also be an array of values.
The return value is always exact and unrounded.
x = new BigNumber('3257869345.0378653')
BigNumber.min(4e9, x, '123456789.9') // '123456789.9'
arr = [2, new BigNumber(-14), '-15.9999', -12]
BigNumber.min(arr) // '-15.9999'
random.random([dp]) ⇒ BigNumber
dp: number: integer, 0 to 1e+9 inclusive
Returns a new BigNumber with a pseudo-random value equal to or greater than 0 and
less than 1.
The return value will have dp decimal places (or less if trailing zeros are
produced).
If dp is omitted then the number of decimal places will default to the current
DECIMAL_PLACES setting.
Depending on the value of this BigNumber constructor's
CRYPTO setting and the support for the
crypto object in the host environment, the random digits of the return value are
generated by either Math.random (fastest), crypto.getRandomValues
(Web Cryptography API in recent browsers) or crypto.randomBytes (Node.js).
If CRYPTO is true, i.e. one of the
crypto methods is to be used, the value of a returned BigNumber should be
cryptographically-secure and statistically indistinguishable from a random value.
BigNumber.config({ DECIMAL_PLACES: 10 })
BigNumber.random() // '0.4117936847'
BigNumber.random(20) // '0.78193327636914089009'
Properties
The library's enumerated rounding modes are stored as properties of the constructor.
(They are not referenced internally by the library itself.)
Rounding modes 0 to 6 (inclusive) are the same as those of Java's
BigDecimal class.
Property Value Description
ROUND_UP 0 Rounds away from zero
ROUND_DOWN 1 Rounds towards zero
ROUND_CEIL 2 Rounds towards Infinity
ROUND_FLOOR 3 Rounds towards -Infinity
ROUND_HALF_UP 4
Rounds towards nearest neighbour.
If equidistant, rounds away from zero
ROUND_HALF_DOWN 5
Rounds towards nearest neighbour.
If equidistant, rounds towards zero
ROUND_HALF_EVEN 6
Rounds towards nearest neighbour.
If equidistant, rounds towards even neighbour
ROUND_HALF_CEIL 7
Rounds towards nearest neighbour.
If equidistant, rounds towards Infinity
ROUND_HALF_FLOOR 8
Rounds towards nearest neighbour.
If equidistant, rounds towards -Infinity
BigNumber.config({ ROUNDING_MODE: BigNumber.ROUND_CEIL })
BigNumber.config({ ROUNDING_MODE: 2 }) // equivalent
INSTANCE
Methods
The methods inherited by a BigNumber instance from its constructor's prototype object.
A BigNumber is immutable in the sense that it is not changed by its methods.
The treatment of ±0, ±Infinity and NaN is
consistent with how JavaScript treats these values.
Many method names have a shorter alias.
(Internally, the library always uses the shorter method names.)
absoluteValue.abs() ⇒ BigNumber
Returns a BigNumber whose value is the absolute value, i.e. the magnitude, of the value of
this BigNumber.
The return value is always exact and unrounded.
x = new BigNumber(-0.8)
y = x.absoluteValue() // '0.8'
z = y.abs() // '0.8'
ceil.ceil() ⇒ BigNumber
Returns a BigNumber whose value is the value of this BigNumber rounded to
a whole number in the direction of positive Infinity.
x = new BigNumber(1.3)
x.ceil() // '2'
y = new BigNumber(-1.8)
y.ceil() // '-1'
comparedTo.cmp(n [, base]) ⇒ number
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns
1 If the value of this BigNumber is greater than the value of n
-1 If the value of this BigNumber is less than the value of n
0 If this BigNumber and n have the same value
null If the value of either this BigNumber or n is NaN
x = new BigNumber(Infinity)
y = new BigNumber(5)
x.comparedTo(y) // 1
x.comparedTo(x.minus(1)) // 0
y.cmp(NaN) // null
y.cmp('110', 2) // -1
decimalPlaces.dp() ⇒ number
Return the number of decimal places of the value of this BigNumber, or null if
the value of this BigNumber is ±Infinity or NaN.
x = new BigNumber(123.45)
x.decimalPlaces() // 2
y = new BigNumber('9.9e-101')
y.dp() // 102
dividedBy.div(n [, base]) ⇒ BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns a BigNumber whose value is the value of this BigNumber divided by
n, rounded according to the current
DECIMAL_PLACES and
ROUNDING_MODE configuration.
x = new BigNumber(355)
y = new BigNumber(113)
x.dividedBy(y) // '3.14159292035398230088'
x.div(5) // '71'
x.div(47, 16) // '5'
dividedToIntegerBy.divToInt(n [, base]) ⇒
BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Return a BigNumber whose value is the integer part of dividing the value of this BigNumber by
n.
x = new BigNumber(5)
y = new BigNumber(3)
x.dividedToIntegerBy(y) // '1'
x.divToInt(0.7) // '7'
x.divToInt('0.f', 16) // '5'
equals.eq(n [, base]) ⇒ boolean
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns true if the value of this BigNumber equals the value of n,
otherwise returns false.
As with JavaScript, NaN does not equal NaN.
Note: This method uses the comparedTo method internally.
0 === 1e-324 // true
x = new BigNumber(0)
x.equals('1e-324') // false
BigNumber(-0).eq(x) // true ( -0 === 0 )
BigNumber(255).eq('ff', 16) // true
y = new BigNumber(NaN)
y.equals(NaN) // false
floor.floor() ⇒ BigNumber
Returns a BigNumber whose value is the value of this BigNumber rounded to a whole number in
the direction of negative Infinity.
x = new BigNumber(1.8)
x.floor() // '1'
y = new BigNumber(-1.3)
y.floor() // '-2'
greaterThan.gt(n [, base]) ⇒ boolean
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns true if the value of this BigNumber is greater than the value of
n, otherwise returns false.
Note: This method uses the comparedTo method internally.
0.1 > (0.3 - 0.2) // true
x = new BigNumber(0.1)
x.greaterThan(BigNumber(0.3).minus(0.2)) // false
BigNumber(0).gt(x) // false
BigNumber(11, 3).gt(11.1, 2) // true
greaterThanOrEqualTo.gte(n [, base]) ⇒ boolean
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns true if the value of this BigNumber is greater than or equal to the value
of n, otherwise returns false.
Note: This method uses the comparedTo method internally.
(0.3 - 0.2) >= 0.1 // false
x = new BigNumber(0.3).minus(0.2)
x.greaterThanOrEqualTo(0.1) // true
BigNumber(1).gte(x) // true
BigNumber(10, 18).gte('i', 36) // true
isFinite.isFinite() ⇒ boolean
Returns true if the value of this BigNumber is a finite number, otherwise
returns false.
The only possible non-finite values of a BigNumber are NaN, Infinity
and -Infinity.
x = new BigNumber(1)
x.isFinite() // true
y = new BigNumber(Infinity)
y.isFinite() // false
Note: The native method isFinite() can be used if
n <= Number.MAX_VALUE.
isInteger.isInt() ⇒ boolean
Returns true if the value of this BigNumber is a whole number, otherwise returns
false.
x = new BigNumber(1)
x.isInteger() // true
y = new BigNumber(123.456)
y.isInt() // false
isNaN.isNaN() ⇒ boolean
Returns true if the value of this BigNumber is NaN, otherwise
returns false.
x = new BigNumber(NaN)
x.isNaN() // true
y = new BigNumber('Infinity')
y.isNaN() // false
Note: The native method isNaN() can also be used.
isNegative.isNeg() ⇒ boolean
Returns true if the value of this BigNumber is negative, otherwise returns
false.
x = new BigNumber(-0)
x.isNegative() // true
y = new BigNumber(2)
y.isNeg() // false
Note: n < 0 can be used if n <= -Number.MIN_VALUE.
isZero.isZero() ⇒ boolean
Returns true if the value of this BigNumber is zero or minus zero, otherwise
returns false.
x = new BigNumber(-0)
x.isZero() && x.isNeg() // true
y = new BigNumber(Infinity)
y.isZero() // false
Note: n == 0 can be used if n >= Number.MIN_VALUE.
lessThan.lt(n [, base]) ⇒ boolean
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns true if the value of this BigNumber is less than the value of
n, otherwise returns false.
Note: This method uses the comparedTo method internally.
(0.3 - 0.2) < 0.1 // true
x = new BigNumber(0.3).minus(0.2)
x.lessThan(0.1) // false
BigNumber(0).lt(x) // true
BigNumber(11.1, 2).lt(11, 3) // true
lessThanOrEqualTo.lte(n [, base]) ⇒ boolean
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns true if the value of this BigNumber is less than or equal to the value of
n, otherwise returns false.
Note: This method uses the comparedTo method internally.
0.1 <= (0.3 - 0.2) // false
x = new BigNumber(0.1)
x.lessThanOrEqualTo(BigNumber(0.3).minus(0.2)) // true
BigNumber(-1).lte(x) // true
BigNumber(10, 18).lte('i', 36) // true
minus.minus(n [, base]) ⇒ BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns a BigNumber whose value is the value of this BigNumber minus n.
The return value is always exact and unrounded.
0.3 - 0.1 // 0.19999999999999998
x = new BigNumber(0.3)
x.minus(0.1) // '0.2'
x.minus(0.6, 20) // '0'
modulo.mod(n [, base]) ⇒ BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns a BigNumber whose value is the value of this BigNumber modulo n, i.e.
the integer remainder of dividing this BigNumber by n.
The value returned, and in particular its sign, is dependent on the value of the
MODULO_MODE setting of this BigNumber constructor.
If it is 1 (default value), the result will have the same sign as this BigNumber,
and it will match that of Javascript's % operator (within the limits of double
precision) and BigDecimal's remainder method.
The return value is always exact and unrounded.
See MODULO_MODE for a description of the other
modulo modes.
1 % 0.9 // 0.09999999999999998
x = new BigNumber(1)
x.modulo(0.9) // '0.1'
y = new BigNumber(33)
y.mod('a', 33) // '3'
negated.neg() ⇒ BigNumber
Returns a BigNumber whose value is the value of this BigNumber negated, i.e. multiplied by
-1.
x = new BigNumber(1.8)
x.negated() // '-1.8'
y = new BigNumber(-1.3)
y.neg() // '1.3'
plus.plus(n [, base]) ⇒ BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns a BigNumber whose value is the value of this BigNumber plus n.
The return value is always exact and unrounded.
0.1 + 0.2 // 0.30000000000000004
x = new BigNumber(0.1)
y = x.plus(0.2) // '0.3'
BigNumber(0.7).plus(x).plus(y) // '1'
x.plus('0.1', 8) // '0.225'
precision.sd([z]) ⇒ number
z: boolean|number: true, false, 0
or 1
Returns the number of significant digits of the value of this BigNumber.
If z is true or 1 then any trailing zeros of the
integer part of a number are counted as significant digits, otherwise they are not.
x = new BigNumber(1.234)
x.precision() // 4
y = new BigNumber(987000)
y.sd() // 3
y.sd(true) // 6
round.round([dp [, rm]]) ⇒ BigNumber
dp: number: integer, 0 to 1e+9 inclusive
rm: number: integer, 0 to 8 inclusive
Returns a BigNumber whose value is the value of this BigNumber rounded by rounding mode
rm to a maximum of dp decimal places.
if dp is omitted, or is null or undefined, the
return value is n rounded to a whole number.
if rm is omitted, or is null or undefined,
ROUNDING_MODE is used.
See Errors for the treatment of other non-integer or out of range
dp or rm values.
x = 1234.56
Math.round(x) // 1235
y = new BigNumber(x)
y.round() // '1235'
y.round(1) // '1234.6'
y.round(2) // '1234.56'
y.round(10) // '1234.56'
y.round(0, 1) // '1234'
y.round(0, 6) // '1235'
y.round(1, 1) // '1234.5'
y.round(1, BigNumber.ROUND_HALF_EVEN) // '1234.6'
y // '1234.56'
shift.shift(n) ⇒ BigNumber
n: number: integer,
-9007199254740991 to 9007199254740991 inclusive
Returns a BigNumber whose value is the value of this BigNumber shifted n places.
The shift is of the decimal point, i.e. of powers of ten, and is to the left if n
is negative or to the right if n is positive.
The return value is always exact and unrounded.
x = new BigNumber(1.23)
x.shift(3) // '1230'
x.shift(-3) // '0.00123'
squareRoot.sqrt() ⇒ BigNumber
Returns a BigNumber whose value is the square root of the value of this BigNumber,
rounded according to the current
DECIMAL_PLACES and
ROUNDING_MODE configuration.
The return value will be correctly rounded, i.e. rounded as if the result was first calculated
to an infinite number of correct digits before rounding.
x = new BigNumber(16)
x.squareRoot() // '4'
y = new BigNumber(3)
y.sqrt() // '1.73205080756887729353'
times.times(n [, base]) ⇒ BigNumber
n: number|string|BigNumber
base: number
See BigNumber for further parameter details.
Returns a BigNumber whose value is the value of this BigNumber times n.
The return value is always exact and unrounded.
0.6 * 3 // 1.7999999999999998
x = new BigNumber(0.6)
y = x.times(3) // '1.8'
BigNumber('7e+500').times(y) // '1.26e+501'
x.times('-a', 16) // '-6'
toDigits.toDigits([sd [, rm]]) ⇒ BigNumber
sd: number: integer, 1 to 1e+9 inclusive.
rm: number: integer, 0 to 8 inclusive.
Returns a BigNumber whose value is the value of this BigNumber rounded to sd
significant digits using rounding mode rm.
If sd is omitted or is null or undefined, the return
value will not be rounded.
If rm is omitted or is null or undefined,
ROUNDING_MODE will be used.
See Errors for the treatment of other non-integer or out of range
sd or rm values.
BigNumber.config({ precision: 5, rounding: 4 })
x = new BigNumber(9876.54321)
x.toDigits() // '9876.5'
x.toDigits(6) // '9876.54'
x.toDigits(6, BigNumber.ROUND_UP) // '9876.55'
x.toDigits(2) // '9900'
x.toDigits(2, 1) // '9800'
x // '9876.54321'
toExponential.toExponential([dp [, rm]]) ⇒ string
dp: number: integer, 0 to 1e+9 inclusive
rm: number: integer, 0 to 8 inclusive
Returns a string representing the value of this BigNumber in exponential notation rounded
using rounding mode rm to dp decimal places, i.e with one digit
before the decimal point and dp digits after it.
If the value of this BigNumber in exponential notation has fewer than dp fraction
digits, the return value will be appended with zeros accordingly.
If dp is omitted, or is null or undefined, the number
of digits after the decimal point defaults to the minimum number of digits necessary to
represent the value exactly.
If rm is omitted or is null or undefined,
ROUNDING_MODE is used.
See Errors for the treatment of other non-integer or out of range
dp or rm values.
x = 45.6
y = new BigNumber(x)
x.toExponential() // '4.56e+1'
y.toExponential() // '4.56e+1'
x.toExponential(0) // '5e+1'
y.toExponential(0) // '5e+1'
x.toExponential(1) // '4.6e+1'
y.toExponential(1) // '4.6e+1'
y.toExponential(1, 1) // '4.5e+1' (ROUND_DOWN)
x.toExponential(3) // '4.560e+1'
y.toExponential(3) // '4.560e+1'
toFixed.toFixed([dp [, rm]]) ⇒ string
dp: number: integer, 0 to 1e+9 inclusive
rm: number: integer, 0 to 8 inclusive
Returns a string representing the value of this BigNumber in normal (fixed-point) notation
rounded to dp decimal places using rounding mode rm.
If the value of this BigNumber in normal notation has fewer than dp fraction
digits, the return value will be appended with zeros accordingly.
Unlike Number.prototype.toFixed, which returns exponential notation if a number
is greater or equal to 1021, this method will always return normal
notation.
If dp is omitted or is null or undefined, the return
value will be unrounded and in normal notation. This is also unlike
Number.prototype.toFixed, which returns the value to zero decimal places.
It is useful when fixed-point notation is required and the current
EXPONENTIAL_AT setting causes
toString to return exponential notation.
If rm is omitted or is null or undefined,
ROUNDING_MODE is used.
See Errors for the treatment of other non-integer or out of range
dp or rm values.
x = 3.456
y = new BigNumber(x)
x.toFixed() // '3'
y.toFixed() // '3.456'
y.toFixed(0) // '3'
x.toFixed(2) // '3.46'
y.toFixed(2) // '3.46'
y.toFixed(2, 1) // '3.45' (ROUND_DOWN)
x.toFixed(5) // '3.45600'
y.toFixed(5) // '3.45600'
toFormat.toFormat([dp [, rm]]) ⇒ string
dp: number: integer, 0 to 1e+9 inclusive
rm: number: integer, 0 to 8 inclusive
Returns a string representing the value of this BigNumber in normal (fixed-point) notation
rounded to dp decimal places using rounding mode rm, and formatted
according to the properties of the FORMAT object.
See the examples below for the properties of the
FORMAT object, their types and their usage.
If dp is omitted or is null or undefined, then the
return value is not rounded to a fixed number of decimal places.
If rm is omitted or is null or undefined,
ROUNDING_MODE is used.
See Errors for the treatment of other non-integer or out of range
dp or rm values.
format = {
decimalSeparator: '.',
groupSeparator: ',',
groupSize: 3,
secondaryGroupSize: 0,
fractionGroupSeparator: ' ',
fractionGroupSize: 0
}
BigNumber.config({ FORMAT: format })
x = new BigNumber('123456789.123456789')
x.toFormat() // '123,456,789.123456789'
x.toFormat(1) // '123,456,789.1'
// If a reference to the object assigned to FORMAT has been retained,
// the format properties can be changed directly
format.groupSeparator = ' '
format.fractionGroupSize = 5
x.toFormat() // '123 456 789.12345 6789'
BigNumber.config({
FORMAT: {
decimalSeparator = ',',
groupSeparator = '.',
groupSize = 3,
secondaryGroupSize = 2
}
})
x.toFormat(6) // '12.34.56.789,123'
toFraction.toFraction([max]) ⇒ [string, string]
max: number|string|BigNumber: integer >= 1 and <
Infinity
Returns a string array representing the value of this BigNumber as a simple fraction with an
integer numerator and an integer denominator. The denominator will be a positive non-zero
value less than or equal to max.
If a maximum denominator, max, is not specified, or is null or
undefined, the denominator will be the lowest value necessary to represent the
number exactly.
See Errors for the treatment of other non-integer or out of range
max values.
x = new BigNumber(1.75)
x.toFraction() // '7, 4'
pi = new BigNumber('3.14159265358')
pi.toFraction() // '157079632679,50000000000'
pi.toFraction(100000) // '312689, 99532'
pi.toFraction(10000) // '355, 113'
pi.toFraction(100) // '311, 99'
pi.toFraction(10) // '22, 7'
pi.toFraction(1) // '3, 1'
toJSON.toJSON() ⇒ string
As valueOf.
x = new BigNumber('177.7e+457')
y = new BigNumber(235.4325)
z = new BigNumber('0.0098074')
// Serialize an array of three BigNumbers
str = JSON.stringify( [x, y, z] )
// "["1.777e+459","235.4325","0.0098074"]"
// Return an array of three BigNumbers
JSON.parse(str, function (key, val) {
return key === '' ? val : new BigNumber(val)
})
toNumber.toNumber() ⇒ number
Returns the value of this BigNumber as a JavaScript number primitive.
Type coercion with, for example, the unary plus operator will also work, except that a
BigNumber with the value minus zero will be converted to positive zero.
x = new BigNumber(456.789)
x.toNumber() // 456.789
+x // 456.789
y = new BigNumber('45987349857634085409857349856430985')
y.toNumber() // 4.598734985763409e+34
z = new BigNumber(-0)
1 / +z // Infinity
1 / z.toNumber() // -Infinity
toPower.pow(n [, m]) ⇒ BigNumber
n: number: integer,
-9007199254740991 to 9007199254740991 inclusive
m: number|string|BigNumber
Returns a BigNumber whose value is the value of this BigNumber raised to the power
n, and optionally modulo a modulus m.
If n is negative the result is rounded according to the current
DECIMAL_PLACES and
ROUNDING_MODE configuration.
If n is not an integer or is out of range:
If ERRORS is true a BigNumber Error is thrown,
else if n is greater than 9007199254740991, it is interpreted as
Infinity;
else if n is less than -9007199254740991, it is interpreted as
-Infinity;
else if n is otherwise a number, it is truncated to an integer;
else it is interpreted as NaN.
As the number of digits of the result of the power operation can grow so large so quickly,
e.g. 123.45610000 has over 50000 digits, the number of significant
digits calculated is limited to the value of the
POW_PRECISION setting (unless a modulus
m is specified).
By default POW_PRECISION is set to 0.
This means that an unlimited number of significant digits will be calculated, and that the
method's performance will decrease dramatically for larger exponents.
Negative exponents will be calculated to the number of decimal places specified by
DECIMAL_PLACES (but not to more than
POW_PRECISION significant digits).
If m is specified and the value of m, n and this
BigNumber are positive integers, then a fast modular exponentiation algorithm is used,
otherwise if any of the values is not a positive integer the operation will simply be
performed as x.toPower(n).modulo(m) with a
POW_PRECISION of 0.
Math.pow(0.7, 2) // 0.48999999999999994
x = new BigNumber(0.7)
x.toPower(2) // '0.49'
BigNumber(3).pow(-2) // '0.11111111111111111111'
toPrecision.toPrecision([sd [, rm]]) ⇒ string
sd: number: integer, 1 to 1e+9 inclusive
rm: number: integer, 0 to 8 inclusive
Returns a string representing the value of this BigNumber rounded to sd
significant digits using rounding mode rm.
If sd is less than the number of digits necessary to represent the integer part
of the value in normal (fixed-point) notation, then exponential notation is used.
If sd is omitted, or is null or undefined, then the
return value is the same as n.toString().
If rm is omitted or is null or undefined,
ROUNDING_MODE is used.
See Errors for the treatment of other non-integer or out of range
sd or rm values.
x = 45.6
y = new BigNumber(x)
x.toPrecision() // '45.6'
y.toPrecision() // '45.6'
x.toPrecision(1) // '5e+1'
y.toPrecision(1) // '5e+1'
y.toPrecision(2, 0) // '4.6e+1' (ROUND_UP)
y.toPrecision(2, 1) // '4.5e+1' (ROUND_DOWN)
x.toPrecision(5) // '45.600'
y.toPrecision(5) // '45.600'
toString.toString([base]) ⇒ string
base: number: integer, 2 to 64 inclusive
Returns a string representing the value of this BigNumber in the specified base, or base
10 if base is omitted or is null or
undefined.
For bases above 10, values from 10 to 35 are
represented by a-z (as with Number.prototype.toString),
36 to 61 by A-Z, and 62 and
63 by $ and _ respectively.
If a base is specified the value is rounded according to the current
DECIMAL_PLACES
and ROUNDING_MODE configuration.
If a base is not specified, and this BigNumber has a positive
exponent that is equal to or greater than the positive component of the
current EXPONENTIAL_AT setting,
or a negative exponent equal to or less than the negative component of the
setting, then exponential notation is returned.
If base is null or undefined it is ignored.
See Errors for the treatment of other non-integer or out of range
base values.
x = new BigNumber(750000)
x.toString() // '750000'
BigNumber.config({ EXPONENTIAL_AT: 5 })
x.toString() // '7.5e+5'
y = new BigNumber(362.875)
y.toString(2) // '101101010.111'
y.toString(9) // '442.77777777777777777778'
y.toString(32) // 'ba.s'
BigNumber.config({ DECIMAL_PLACES: 4 });
z = new BigNumber('1.23456789')
z.toString() // '1.23456789'
z.toString(10) // '1.2346'
truncated.trunc() ⇒ BigNumber
Returns a BigNumber whose value is the value of this BigNumber truncated to a whole number.
x = new BigNumber(123.456)
x.truncated() // '123'
y = new BigNumber(-12.3)
y.trunc() // '-12'
valueOf.valueOf() ⇒ string
As toString, but does not accept a base argument and includes the minus sign
for negative zero.
x = new BigNumber('-0')
x.toString() // '0'
x.valueOf() // '-0'
y = new BigNumber('1.777e+457')
y.valueOf() // '1.777e+457'
Properties
A BigNumber is an object with three properties:
Property Description Type Value
c coefficient* number[] Array of base 1e14 numbers
e exponent number Integer, -1000000000 to 1000000000 inclusive
s sign number -1 or 1
*significand
The value of any of the three properties may also be null.
From v2.0.0 of this library, the value of the coefficient of a BigNumber is stored in a
normalised base 100000000000000 floating point format, as opposed to the base
10 format used in v1.x.x
This change means the properties of a BigNumber are now best considered to be read-only.
Previously it was acceptable to change the exponent of a BigNumber by writing to its exponent
property directly, but this is no longer recommended as the number of digits in the first
element of the coefficient array is dependent on the exponent, so the coefficient would also
need to be altered.
Note that, as with JavaScript numbers, the original exponent and fractional trailing zeros are
not necessarily preserved.
x = new BigNumber(0.123) // '0.123'
x.toExponential() // '1.23e-1'
x.c // '1,2,3'
x.e // -1
x.s // 1
y = new Number(-123.4567000e+2) // '-12345.67'
y.toExponential() // '-1.234567e+4'
z = new BigNumber('-123.4567000e+2') // '-12345.67'
z.toExponential() // '-1.234567e+4'
z.c // '1,2,3,4,5,6,7'
z.e // 4
z.s // -1
Zero, NaN and Infinity
The table below shows how ±0, NaN and
±Infinity are stored.
c e s
±0 [0] 0 ±1
NaN null null null
±Infinity null null ±1
x = new Number(-0) // 0
1 / x == -Infinity // true
y = new BigNumber(-0) // '0'
y.c // '0' ( [0].toString() )
y.e // 0
y.s // -1
Errors
The errors that are thrown are generic Error objects with name
BigNumber Error.
The table below shows the errors that may be thrown if ERRORS is
true, and the action taken if ERRORS is false.
Method(s) ERRORS: true
Throw BigNumber Error ERRORS: false
Action on invalid argument
BigNumber
comparedTo
dividedBy
dividedToIntegerBy
equals
greaterThan
greaterThanOrEqualTo
lessThan
lessThanOrEqualTo
minus
modulo
plus
times
number type has more than
15 significant digits Accept.
not a base... number Substitute NaN.
base not an integer Truncate to integer.
Ignore if not a number.
base out of range Ignore.
not a number* Substitute NaN.
another not an object Ignore.
config DECIMAL_PLACES not an integer Truncate to integer.
Ignore if not a number.
DECIMAL_PLACES out of range Ignore.
ROUNDING_MODE not an integer Truncate to integer.
Ignore if not a number.
ROUNDING_MODE out of range Ignore.
EXPONENTIAL_AT not an integer
or not [integer, integer] Truncate to integer(s).
Ignore if not number(s).
EXPONENTIAL_AT out of range
or not [negative, positive] Ignore.
RANGE not an integer
or not [integer, integer] Truncate to integer(s).
Ignore if not number(s).
RANGE cannot be zero Ignore.
RANGE out of range
or not [negative, positive] Ignore.
ERRORS not a boolean
or binary digit Ignore.
CRYPTO not a boolean
or binary digit Ignore.
CRYPTO crypto unavailable Ignore.
MODULO_MODE not an integer Truncate to integer.
Ignore if not a number.
MODULO_MODE out of range Ignore.
POW_PRECISION not an integer Truncate to integer.
Ignore if not a number.
POW_PRECISION out of range Ignore.
FORMAT not an object Ignore.
precision argument not a boolean
or binary digit Ignore.
round decimal places not an integer Truncate to integer.
Ignore if not a number.
decimal places out of range Ignore.
rounding mode not an integer Truncate to integer.
Ignore if not a number.
rounding mode out of range Ignore.
shift argument not an integer Truncate to integer.
Ignore if not a number.
argument out of range Substitute ±Infinity.
toExponential
toFixed
toFormat
decimal places not an integer Truncate to integer.
Ignore if not a number.
decimal places out of range Ignore.
rounding mode not an integer Truncate to integer.
Ignore if not a number.
rounding mode out of range Ignore.
toFraction max denominator not an integer Truncate to integer.
Ignore if not a number.
max denominator out of range Ignore.
toDigits
toPrecision
precision not an integer Truncate to integer.
Ignore if not a number.
precision out of range Ignore.
rounding mode not an integer Truncate to integer.
Ignore if not a number.
rounding mode out of range Ignore.
toPower exponent not an integer Truncate to integer.
Substitute NaN if not a number.
exponent out of range Substitute ±Infinity.
toString base not an integer Truncate to integer.
Ignore if not a number.
base out of range Ignore.
*No error is thrown if the value is NaN or 'NaN'.
The message of a BigNumber Error will also contain the name of the method from which
the error originated.
To determine if an exception is a BigNumber Error:
try {
// ...
} catch (e) {
if ( e instanceof Error && e.name == 'BigNumber Error' ) {
// ...
}
}
FAQ
Why are trailing fractional zeros removed from BigNumbers?
Some arbitrary-precision libraries retain trailing fractional zeros as they can indicate the
precision of a value. This can be useful but the results of arithmetic operations can be
misleading.
x = new BigDecimal("1.0")
y = new BigDecimal("1.1000")
z = x.add(y) // 2.1000
x = new BigDecimal("1.20")
y = new BigDecimal("3.45000")
z = x.multiply(y) // 4.1400000
To specify the precision of a value is to specify that the value lies
within a certain range.
In the first example, x has a value of 1.0. The trailing zero shows
the precision of the value, implying that it is in the range 0.95 to
1.05. Similarly, the precision indicated by the trailing zeros of y
indicates that the value is in the range 1.09995 to 1.10005.
If we add the two lowest values in the ranges we have, 0.95 + 1.09995 = 2.04995,
and if we add the two highest values we have, 1.05 + 1.10005 = 2.15005, so the
range of the result of the addition implied by the precision of its operands is
2.04995 to 2.15005.
The result given by BigDecimal of 2.1000 however, indicates that the value is in
the range 2.09995 to 2.10005 and therefore the precision implied by
its trailing zeros may be misleading.
In the second example, the true range is 4.122744 to 4.157256 yet
the BigDecimal answer of 4.1400000 indicates a range of 4.13999995
to 4.14000005. Again, the precision implied by the trailing zeros may be
misleading.
This library, like binary floating point and most calculators, does not retain trailing
fractional zeros. Instead, the toExponential, toFixed and
toPrecision methods enable trailing zeros to be added if and when required.
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/LICENCE
The MIT Licence.
Copyright (c) 2012 Michael Mclaughlin
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/package.json
{
"_from": "[email protected]",
"_id": "[email protected]",
"_inBundle": false,
"_integrity": "sha1-8725mtUmihX8HwvtL7AY4mk/4jY=",
"_location": "/bignumber.js",
"_phantomChildren": {},
"_requested": {
"type": "version",
"registry": true,
"raw": "[email protected]",
"name": "bignumber.js",
"escapedName": "bignumber.js",
"rawSpec": "3.1.2",
"saveSpec": null,
"fetchSpec": "3.1.2"
},
"_requiredBy": [
"/mysql"
],
"_resolved": "https://registry.npmjs.org/bignumber.js/-/bignumber.js-3.1.2.tgz",
"_shasum": "f3bdb99ad5268a15fc1f0bed2fb018e2693fe236",
"_spec": "[email protected]",
"_where": "D:\\Greynodes\\neverwrote-master@df4dcf72582\\api\\node_modules\\mysql",
"author": {
"name": "Michael Mclaughlin",
"email": "[email protected]"
},
"bugs": {
"url": "https://github.com/MikeMcl/bignumber.js/issues"
},
"bundleDependencies": false,
"deprecated": false,
"description": "A library for arbitrary-precision decimal and non-decimal arithmetic",
"engines": {
"node": "*"
},
"homepage": "https://github.com/MikeMcl/bignumber.js#readme",
"keywords": [
"arbitrary",
"precision",
"arithmetic",
"big",
"number",
"decimal",
"float",
"biginteger",
"bigdecimal",
"bignumber",
"bigint",
"bignum"
],
"license": "MIT",
"main": "bignumber.js",
"name": "bignumber.js",
"repository": {
"type": "git",
"url": "git+https://github.com/MikeMcl/bignumber.js.git"
},
"scripts": {
"build": "uglifyjs bignumber.js --source-map bignumber.js.map -c -m -o bignumber.min.js --preamble \"/* bignumber.js v3.1.2 https://github.com/MikeMcl/bignumber.js/LICENCE */\"",
"test": "node ./test/every-test.js"
},
"version": "3.1.2"
}
neverwrote-master@df4dcf72582/api/node_modules/bignumber.js/README.md

A JavaScript library for arbitrary-precision decimal and non-decimal arithmetic.
[](https://travis-ci.org/MikeMcl/bignumber.js)
## Features
- Faster, smaller, and perhaps easier to use than JavaScript versions of Java's BigDecimal
- 8 KB minified and gzipped
- Simple API but full-featured
- Works with numbers with or without fraction digits in bases from 2 to 64 inclusive
- Replicates the `toExponential`, `toFixed`, `toPrecision` and `toString` methods of JavaScript's Number type
- Includes a `toFraction` and a correctly-rounded `squareRoot` method
- Supports cryptographically-secure pseudo-random number generation
- No dependencies
- Wide platform compatibility: uses JavaScript 1.5 (ECMAScript 3) features only
- Comprehensive [documentation](http://mikemcl.github.io/bignumber.js/) and test set
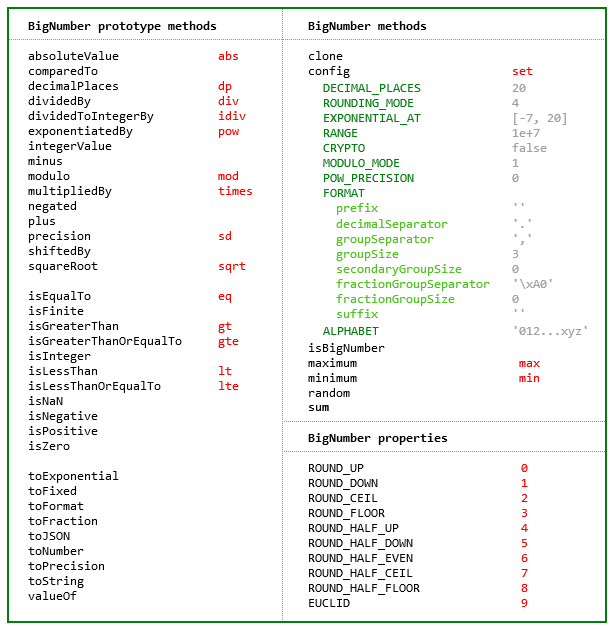
If a smaller and simpler library is required see [big.js](https://github.com/MikeMcl/big.js/).
It's less than half the size but only works with decimal numbers and only has half the methods.
It also does not allow `NaN` or `Infinity`, or have the configuration options of this library.
See also [decimal.js](https://github.com/MikeMcl/decimal.js/), which among other things adds support for non-integer powers, and performs all operations to a specified number of significant digits.
## Load
The library is the single JavaScript file *bignumber.js* (or minified, *bignumber.min.js*).
```html
```
For [Node.js](http://nodejs.org), the library is available from the [npm](https://npmjs.org/) registry
$ npm install bignumber.js
```javascript
var BigNumber = require('bignumber.js');
```
To load with AMD loader libraries such as [requireJS](http://requirejs.org/):
```javascript
require(['path/to/bignumber'], function(BigNumber) {
// Use BigNumber here in local scope. No global BigNumber.
});
```
## Use
*In all examples below, `var`, semicolons and `toString` calls are not shown.
If a commented-out value is in quotes it means `toString` has been called on the preceding expression.*
The library exports a single function: `BigNumber`, the constructor of BigNumber instances.
It accepts a value of type number *(up to 15 significant digits only)*, string or BigNumber object,
```javascript
x = new BigNumber(123.4567)
y = BigNumber('123456.7e-3')
z = new BigNumber(x)
x.equals(y) && y.equals(z) && x.equals(z) // true
```
and a base from 2 to 64 inclusive can be specified.
```javascript
x = new BigNumber(1011, 2) // "11"
y = new BigNumber('zz.9', 36) // "1295.25"
z = x.plus(y) // "1306.25"
```
A BigNumber is immutable in the sense that it is not changed by its methods.
```javascript
0.3 - 0.1 // 0.19999999999999998
x = new BigNumber(0.3)
x.minus(0.1) // "0.2"
x // "0.3"
```
The methods that return a BigNumber can be chained.
```javascript
x.dividedBy(y).plus(z).times(9).floor()
x.times('1.23456780123456789e+9').plus(9876.5432321).dividedBy('4444562598.111772').ceil()
```
Many method names have a shorter alias.
```javascript
x.squareRoot().dividedBy(y).toPower(3).equals(x.sqrt().div(y).pow(3)) // true
x.cmp(y.mod(z).neg()) == 1 && x.comparedTo(y.modulo(z).negated()) == 1 // true
```
Like JavaScript's number type, there are `toExponential`, `toFixed` and `toPrecision` methods
```javascript
x = new BigNumber(255.5)
x.toExponential(5) // "2.55500e+2"
x.toFixed(5) // "255.50000"
x.toPrecision(5) // "255.50"
x.toNumber() // 255.5
```
and a base can be specified for `toString`.
```javascript
x.toString(16) // "ff.8"
```
There is also a `toFormat` method which may be useful for internationalisation
```javascript
y = new BigNumber('1234567.898765')
y.toFormat(2) // "1,234,567.90"
```
The maximum number of decimal places of the result of an operation involving division (i.e. a division, square root, base conversion or negative power operation) is set using the `config` method of the `BigNumber` constructor.
The other arithmetic operations always give the exact result.
```javascript
BigNumber.config({ DECIMAL_PLACES: 10, ROUNDING_MODE: 4 })
x = new BigNumber(2);
y = new BigNumber(3);
z = x.div(y) // "0.6666666667"
z.sqrt() // "0.8164965809"
z.pow(-3) // "3.3749999995"
z.toString(2) // "0.1010101011"
z.times(z) // "0.44444444448888888889"
z.times(z).round(10) // "0.4444444445"
```
There is a `toFraction` method with an optional *maximum denominator* argument
```javascript
y = new BigNumber(355)
pi = y.dividedBy(113) // "3.1415929204"
pi.toFraction() // [ "7853982301", "2500000000" ]
pi.toFraction(1000) // [ "355", "113" ]
```
and `isNaN` and `isFinite` methods, as `NaN` and `Infinity` are valid `BigNumber` values.
```javascript
x = new BigNumber(NaN) // "NaN"
y = new BigNumber(Infinity) // "Infinity"
x.isNaN() && !y.isNaN() && !x.isFinite() && !y.isFinite() // true
```
The value of a BigNumber is stored in a decimal floating point format in terms of a coefficient, exponent and sign.
```javascript
x = new BigNumber(-123.456);
x.c // [ 123, 45600000000000 ] coefficient (i.e. significand)
x.e // 2 exponent
x.s // -1 sign
```
Multiple BigNumber constructors can be created, each with their own independent configuration which applies to all BigNumber's created from it.
```javascript
// Set DECIMAL_PLACES for the original BigNumber constructor
BigNumber.config({ DECIMAL_PLACES: 10 })
// Create another BigNumber constructor, optionally passing in a configuration object
BN = BigNumber.another({ DECIMAL_PLACES: 5 })
x = new BigNumber(1)
y = new BN(1)
x.div(3) // '0.3333333333'
y.div(3) // '0.33333'
```
For futher information see the [API](http://mikemcl.github.io/bignumber.js/) reference in the *doc* directory.
## Test
The *test* directory contains the test scripts for each method.
The tests can be run with Node or a browser. For Node use
$ npm test
or
$ node test/every-test
To test a single method, e.g.
$ node test/toFraction
For the browser, see *every-test.html* and *single-test.html* in the *test/browser* directory.
*bignumber-vs-number.html* enables some of the methods of bignumber.js to be compared with those of JavaScript's number type.
## Versions
Version 1.x.x of this library is still supported on the 'original' branch. The advantages of later versions are that they are considerably faster for numbers with many digits and that there are some added methods (see Change Log below). The disadvantages are more lines of code and increased code complexity, and the loss of simplicity in no longer having the coefficient of a BigNumber stored in base 10.
## Performance
See the [README](https://github.com/MikeMcl/bignumber.js/tree/master/perf) in the *perf* directory.
## Build
For Node, if [uglify-js](https://github.com/mishoo/UglifyJS2) is installed
npm install uglify-js -g
then
npm run build
will create *bignumber.min.js*.
A source map will also be created in the root directory.
## Feedback
Open an issue, or email
Michael
[email protected]
## Licence
MIT.
See [LICENCE](https://github.com/MikeMcl/bignumber.js/blob/master/LICENCE).
## Change Log
#### 3.1.2
* 08/01/2017
* Minor documentation edit.
#### 3.1.1
* 08/01/2017
* Uncomment `isBigNumber` tests.
* Ignore dot files.
#### 3.1.0
* 08/01/2017
* Add `isBigNumber` method.
#### 3.0.2
* 08/01/2017
* Bugfix: Possible incorrect value of `ERRORS` after a `BigNumber.another` call (due to `parseNumeric` declaration in outer scope).
#### 3.0.1
* 23/11/2016
* Apply fix for old ipads with `%` issue, see #57 and #102.
* Correct error message.
#### 3.0.0
* 09/11/2016
* Remove `require('crypto')` - leave it to the user.
* Add `BigNumber.set` as `BigNumber.config` alias.
* Default `POW_PRECISION` to `0`.
#### 2.4.0
* 14/07/2016
* #97 Add exports to support ES6 imports.
#### 2.3.0
* 07/03/2016
* #86 Add modulus parameter to `toPower`.
#### 2.2.0
* 03/03/2016
* #91 Permit larger JS integers.
#### 2.1.4
* 15/12/2015
* Correct UMD.
#### 2.1.3
* 13/12/2015
* Refactor re global object and crypto availability when bundling.
#### 2.1.2
* 10/12/2015
* Bugfix: `window.crypto` not assigned to `crypto`.
#### 2.1.1
* 09/12/2015
* Prevent code bundler from adding `crypto` shim.
#### 2.1.0
* 26/10/2015
* For `valueOf` and `toJSON`, include the minus sign with negative zero.
#### 2.0.8
* 2/10/2015
* Internal round function bugfix.
#### 2.0.6
* 31/03/2015
* Add bower.json. Tweak division after in-depth review.
#### 2.0.5
* 25/03/2015
* Amend README. Remove bitcoin address.
#### 2.0.4
* 25/03/2015
* Critical bugfix #58: division.
#### 2.0.3
* 18/02/2015
* Amend README. Add source map.
#### 2.0.2
* 18/02/2015
* Correct links.
#### 2.0.1
* 18/02/2015
* Add `max`, `min`, `precision`, `random`, `shift`, `toDigits` and `truncated` methods.
* Add the short-forms: `add`, `mul`, `sd`, `sub` and `trunc`.
* Add an `another` method to enable multiple independent constructors to be created.
* Add support for the base 2, 8 and 16 prefixes `0b`, `0o` and `0x`.
* Enable a rounding mode to be specified as a second parameter to `toExponential`, `toFixed`, `toFormat` and `toPrecision`.
* Add a `CRYPTO` configuration property so cryptographically-secure pseudo-random number generation can be specified.
* Add a `MODULO_MODE` configuration property to enable the rounding mode used by the `modulo` operation to be specified.
* Add a `POW_PRECISION` configuration property to enable the number of significant digits calculated by the power operation to be limited.
* Improve code quality.
* Improve documentation.
#### 2.0.0
* 29/12/2014
* Add `dividedToIntegerBy`, `isInteger` and `toFormat` methods.
* Remove the following short-forms: `isF`, `isZ`, `toE`, `toF`, `toFr`, `toN`, `toP`, `toS`.
* Store a BigNumber's coefficient in base 1e14, rather than base 10.
* Add fast path for integers to BigNumber constructor.
* Incorporate the library into the online documentation.
#### 1.5.0
* 13/11/2014
* Add `toJSON` and `decimalPlaces` methods.
#### 1.4.1
* 08/06/2014
* Amend README.
#### 1.4.0
* 08/05/2014
* Add `toNumber`.
#### 1.3.0
* 08/11/2013
* Ensure correct rounding of `sqrt` in all, rather than almost all, cases.
* Maximum radix to 64.
#### 1.2.1
* 17/10/2013
* Sign of zero when x < 0 and x + (-x) = 0.
#### 1.2.0
* 19/9/2013
* Throw Error objects for stack.
#### 1.1.1
* 22/8/2013
* Show original value in constructor error message.
#### 1.1.0
* 1/8/2013
* Allow numbers with trailing radix point.
#### 1.0.1
* Bugfix: error messages with incorrect method name
#### 1.0.0
* 8/11/2012
* Initial release
neverwrote-master@df4dcf72582/api/node_modules/bluebird/changelog.md
[http://bluebirdjs.com/docs/changelog.html](http://bluebirdjs.com/docs/changelog.html)
neverwrote-master@df4dcf72582/api/node_modules/bluebird/js/browser/bluebird.core.js
/* @preserve
* The MIT License (MIT)
*
* Copyright (c) 2013-2018 Petka Antonov
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
/**
* bluebird build version 3.7.2
* Features enabled: core
* Features disabled: race, call_get, generators, map, nodeify, promisify, props, reduce, settle, some, using, timers, filter, any, each
*/
!function(e){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=e();else if("function"==typeof define&&define.amd)define([],e);else{var f;"undefined"!=typeof window?f=window:"undefined"!=typeof global?f=global:"undefined"!=typeof self&&(f=self),f.Promise=e()}}(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof _dereq_=="function"&&_dereq_;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof _dereq_=="function"&&_dereq_;for(var o=0;o"use strict";
var firstLineError;
try {throw new Error(); } catch (e) {firstLineError = e;}
var schedule = _dereq_("./schedule");
var Queue = _dereq_("./queue");
function Async() {
this._customScheduler = false;
this._isTickUsed = false;
this._lateQueue = new Queue(16);
this._normalQueue = new Queue(16);
this._haveDrainedQueues = false;
var self = this;
this.drainQueues = function () {
self._drainQueues();
};
this._schedule = schedule;
}
Async.prototype.setScheduler = function(fn) {
var prev = this._schedule;
this._schedule = fn;
this._customScheduler = true;
return prev;
};
Async.prototype.hasCustomScheduler = function() {
return this._customScheduler;
};
Async.prototype.haveItemsQueued = function () {
return this._isTickUsed || this._haveDrainedQueues;
};
Async.prototype.fatalError = function(e, isNode) {
if (isNode) {
process.stderr.write("Fatal " + (e instanceof Error ? e.stack : e) +
"\n");
process.exit(2);
} else {
this.throwLater(e);
}
};
Async.prototype.throwLater = function(fn, arg) {
if (arguments.length === 1) {
arg = fn;
fn = function () { throw arg; };
}
if (typeof setTimeout !== "undefined") {
setTimeout(function() {
fn(arg);
}, 0);
} else try {
this._schedule(function() {
fn(arg);
});
} catch (e) {
throw new Error("No async scheduler available\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
};
function AsyncInvokeLater(fn, receiver, arg) {
this._lateQueue.push(fn, receiver, arg);
this._queueTick();
}
function AsyncInvoke(fn, receiver, arg) {
this._normalQueue.push(fn, receiver, arg);
this._queueTick();
}
function AsyncSettlePromises(promise) {
this._normalQueue._pushOne(promise);
this._queueTick();
}
Async.prototype.invokeLater = AsyncInvokeLater;
Async.prototype.invoke = AsyncInvoke;
Async.prototype.settlePromises = AsyncSettlePromises;
function _drainQueue(queue) {
while (queue.length() > 0) {
_drainQueueStep(queue);
}
}
function _drainQueueStep(queue) {
var fn = queue.shift();
if (typeof fn !== "function") {
fn._settlePromises();
} else {
var receiver = queue.shift();
var arg = queue.shift();
fn.call(receiver, arg);
}
}
Async.prototype._drainQueues = function () {
_drainQueue(this._normalQueue);
this._reset();
this._haveDrainedQueues = true;
_drainQueue(this._lateQueue);
};
Async.prototype._queueTick = function () {
if (!this._isTickUsed) {
this._isTickUsed = true;
this._schedule(this.drainQueues);
}
};
Async.prototype._reset = function () {
this._isTickUsed = false;
};
module.exports = Async;
module.exports.firstLineError = firstLineError;
},{"./queue":17,"./schedule":18}],2:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL, tryConvertToPromise, debug) {
var calledBind = false;
var rejectThis = function(_, e) {
this._reject(e);
};
var targetRejected = function(e, context) {
context.promiseRejectionQueued = true;
context.bindingPromise._then(rejectThis, rejectThis, null, this, e);
};
var bindingResolved = function(thisArg, context) {
if (((this._bitField & 50397184) === 0)) {
this._resolveCallback(context.target);
}
};
var bindingRejected = function(e, context) {
if (!context.promiseRejectionQueued) this._reject(e);
};
Promise.prototype.bind = function (thisArg) {
if (!calledBind) {
calledBind = true;
Promise.prototype._propagateFrom = debug.propagateFromFunction();
Promise.prototype._boundValue = debug.boundValueFunction();
}
var maybePromise = tryConvertToPromise(thisArg);
var ret = new Promise(INTERNAL);
ret._propagateFrom(this, 1);
var target = this._target();
ret._setBoundTo(maybePromise);
if (maybePromise instanceof Promise) {
var context = {
promiseRejectionQueued: false,
promise: ret,
target: target,
bindingPromise: maybePromise
};
target._then(INTERNAL, targetRejected, undefined, ret, context);
maybePromise._then(
bindingResolved, bindingRejected, undefined, ret, context);
ret._setOnCancel(maybePromise);
} else {
ret._resolveCallback(target);
}
return ret;
};
Promise.prototype._setBoundTo = function (obj) {
if (obj !== undefined) {
this._bitField = this._bitField | 2097152;
this._boundTo = obj;
} else {
this._bitField = this._bitField & (~2097152);
}
};
Promise.prototype._isBound = function () {
return (this._bitField & 2097152) === 2097152;
};
Promise.bind = function (thisArg, value) {
return Promise.resolve(value).bind(thisArg);
};
};
},{}],3:[function(_dereq_,module,exports){
"use strict";
var old;
if (typeof Promise !== "undefined") old = Promise;
function noConflict() {
try { if (Promise === bluebird) Promise = old; }
catch (e) {}
return bluebird;
}
var bluebird = _dereq_("./promise")();
bluebird.noConflict = noConflict;
module.exports = bluebird;
},{"./promise":15}],4:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, PromiseArray, apiRejection, debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
var async = Promise._async;
Promise.prototype["break"] = Promise.prototype.cancel = function() {
if (!debug.cancellation()) return this._warn("cancellation is disabled");
var promise = this;
var child = promise;
while (promise._isCancellable()) {
if (!promise._cancelBy(child)) {
if (child._isFollowing()) {
child._followee().cancel();
} else {
child._cancelBranched();
}
break;
}
var parent = promise._cancellationParent;
if (parent == null || !parent._isCancellable()) {
if (promise._isFollowing()) {
promise._followee().cancel();
} else {
promise._cancelBranched();
}
break;
} else {
if (promise._isFollowing()) promise._followee().cancel();
promise._setWillBeCancelled();
child = promise;
promise = parent;
}
}
};
Promise.prototype._branchHasCancelled = function() {
this._branchesRemainingToCancel--;
};
Promise.prototype._enoughBranchesHaveCancelled = function() {
return this._branchesRemainingToCancel === undefined ||
this._branchesRemainingToCancel <= 0;
};
Promise.prototype._cancelBy = function(canceller) {
if (canceller === this) {
this._branchesRemainingToCancel = 0;
this._invokeOnCancel();
return true;
} else {
this._branchHasCancelled();
if (this._enoughBranchesHaveCancelled()) {
this._invokeOnCancel();
return true;
}
}
return false;
};
Promise.prototype._cancelBranched = function() {
if (this._enoughBranchesHaveCancelled()) {
this._cancel();
}
};
Promise.prototype._cancel = function() {
if (!this._isCancellable()) return;
this._setCancelled();
async.invoke(this._cancelPromises, this, undefined);
};
Promise.prototype._cancelPromises = function() {
if (this._length() > 0) this._settlePromises();
};
Promise.prototype._unsetOnCancel = function() {
this._onCancelField = undefined;
};
Promise.prototype._isCancellable = function() {
return this.isPending() && !this._isCancelled();
};
Promise.prototype.isCancellable = function() {
return this.isPending() && !this.isCancelled();
};
Promise.prototype._doInvokeOnCancel = function(onCancelCallback, internalOnly) {
if (util.isArray(onCancelCallback)) {
for (var i = 0; i < onCancelCallback.length; ++i) {
this._doInvokeOnCancel(onCancelCallback[i], internalOnly);
}
} else if (onCancelCallback !== undefined) {
if (typeof onCancelCallback === "function") {
if (!internalOnly) {
var e = tryCatch(onCancelCallback).call(this._boundValue());
if (e === errorObj) {
this._attachExtraTrace(e.e);
async.throwLater(e.e);
}
}
} else {
onCancelCallback._resultCancelled(this);
}
}
};
Promise.prototype._invokeOnCancel = function() {
var onCancelCallback = this._onCancel();
this._unsetOnCancel();
async.invoke(this._doInvokeOnCancel, this, onCancelCallback);
};
Promise.prototype._invokeInternalOnCancel = function() {
if (this._isCancellable()) {
this._doInvokeOnCancel(this._onCancel(), true);
this._unsetOnCancel();
}
};
Promise.prototype._resultCancelled = function() {
this.cancel();
};
};
},{"./util":21}],5:[function(_dereq_,module,exports){
"use strict";
module.exports = function(NEXT_FILTER) {
var util = _dereq_("./util");
var getKeys = _dereq_("./es5").keys;
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
function catchFilter(instances, cb, promise) {
return function(e) {
var boundTo = promise._boundValue();
predicateLoop: for (var i = 0; i < instances.length; ++i) {
var item = instances[i];
if (item === Error ||
(item != null && item.prototype instanceof Error)) {
if (e instanceof item) {
return tryCatch(cb).call(boundTo, e);
}
} else if (typeof item === "function") {
var matchesPredicate = tryCatch(item).call(boundTo, e);
if (matchesPredicate === errorObj) {
return matchesPredicate;
} else if (matchesPredicate) {
return tryCatch(cb).call(boundTo, e);
}
} else if (util.isObject(e)) {
var keys = getKeys(item);
for (var j = 0; j < keys.length; ++j) {
var key = keys[j];
if (item[key] != e[key]) {
continue predicateLoop;
}
}
return tryCatch(cb).call(boundTo, e);
}
}
return NEXT_FILTER;
};
}
return catchFilter;
};
},{"./es5":10,"./util":21}],6:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
var longStackTraces = false;
var contextStack = [];
Promise.prototype._promiseCreated = function() {};
Promise.prototype._pushContext = function() {};
Promise.prototype._popContext = function() {return null;};
Promise._peekContext = Promise.prototype._peekContext = function() {};
function Context() {
this._trace = new Context.CapturedTrace(peekContext());
}
Context.prototype._pushContext = function () {
if (this._trace !== undefined) {
this._trace._promiseCreated = null;
contextStack.push(this._trace);
}
};
Context.prototype._popContext = function () {
if (this._trace !== undefined) {
var trace = contextStack.pop();
var ret = trace._promiseCreated;
trace._promiseCreated = null;
return ret;
}
return null;
};
function createContext() {
if (longStackTraces) return new Context();
}
function peekContext() {
var lastIndex = contextStack.length - 1;
if (lastIndex >= 0) {
return contextStack[lastIndex];
}
return undefined;
}
Context.CapturedTrace = null;
Context.create = createContext;
Context.deactivateLongStackTraces = function() {};
Context.activateLongStackTraces = function() {
var Promise_pushContext = Promise.prototype._pushContext;
var Promise_popContext = Promise.prototype._popContext;
var Promise_PeekContext = Promise._peekContext;
var Promise_peekContext = Promise.prototype._peekContext;
var Promise_promiseCreated = Promise.prototype._promiseCreated;
Context.deactivateLongStackTraces = function() {
Promise.prototype._pushContext = Promise_pushContext;
Promise.prototype._popContext = Promise_popContext;
Promise._peekContext = Promise_PeekContext;
Promise.prototype._peekContext = Promise_peekContext;
Promise.prototype._promiseCreated = Promise_promiseCreated;
longStackTraces = false;
};
longStackTraces = true;
Promise.prototype._pushContext = Context.prototype._pushContext;
Promise.prototype._popContext = Context.prototype._popContext;
Promise._peekContext = Promise.prototype._peekContext = peekContext;
Promise.prototype._promiseCreated = function() {
var ctx = this._peekContext();
if (ctx && ctx._promiseCreated == null) ctx._promiseCreated = this;
};
};
return Context;
};
},{}],7:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, Context,
enableAsyncHooks, disableAsyncHooks) {
var async = Promise._async;
var Warning = _dereq_("./errors").Warning;
var util = _dereq_("./util");
var es5 = _dereq_("./es5");
var canAttachTrace = util.canAttachTrace;
var unhandledRejectionHandled;
var possiblyUnhandledRejection;
var bluebirdFramePattern =
/[\\\/]bluebird[\\\/]js[\\\/](release|debug|instrumented)/;
var nodeFramePattern = /\((?:timers\.js):\d+:\d+\)/;
var parseLinePattern = /[\/<\(](.+?):(\d+):(\d+)\)?\s*$/;
var stackFramePattern = null;
var formatStack = null;
var indentStackFrames = false;
var printWarning;
var debugging = !!(util.env("BLUEBIRD_DEBUG") != 0 &&
(true ||
util.env("BLUEBIRD_DEBUG") ||
util.env("NODE_ENV") === "development"));
var warnings = !!(util.env("BLUEBIRD_WARNINGS") != 0 &&
(debugging || util.env("BLUEBIRD_WARNINGS")));
var longStackTraces = !!(util.env("BLUEBIRD_LONG_STACK_TRACES") != 0 &&
(debugging || util.env("BLUEBIRD_LONG_STACK_TRACES")));
var wForgottenReturn = util.env("BLUEBIRD_W_FORGOTTEN_RETURN") != 0 &&
(warnings || !!util.env("BLUEBIRD_W_FORGOTTEN_RETURN"));
var deferUnhandledRejectionCheck;
(function() {
var promises = [];
function unhandledRejectionCheck() {
for (var i = 0; i < promises.length; ++i) {
promises[i]._notifyUnhandledRejection();
}
unhandledRejectionClear();
}
function unhandledRejectionClear() {
promises.length = 0;
}
deferUnhandledRejectionCheck = function(promise) {
promises.push(promise);
setTimeout(unhandledRejectionCheck, 1);
};
es5.defineProperty(Promise, "_unhandledRejectionCheck", {
value: unhandledRejectionCheck
});
es5.defineProperty(Promise, "_unhandledRejectionClear", {
value: unhandledRejectionClear
});
})();
Promise.prototype.suppressUnhandledRejections = function() {
var target = this._target();
target._bitField = ((target._bitField & (~1048576)) |
524288);
};
Promise.prototype._ensurePossibleRejectionHandled = function () {
if ((this._bitField & 524288) !== 0) return;
this._setRejectionIsUnhandled();
deferUnhandledRejectionCheck(this);
};
Promise.prototype._notifyUnhandledRejectionIsHandled = function () {
fireRejectionEvent("rejectionHandled",
unhandledRejectionHandled, undefined, this);
};
Promise.prototype._setReturnedNonUndefined = function() {
this._bitField = this._bitField | 268435456;
};
Promise.prototype._returnedNonUndefined = function() {
return (this._bitField & 268435456) !== 0;
};
Promise.prototype._notifyUnhandledRejection = function () {
if (this._isRejectionUnhandled()) {
var reason = this._settledValue();
this._setUnhandledRejectionIsNotified();
fireRejectionEvent("unhandledRejection",
possiblyUnhandledRejection, reason, this);
}
};
Promise.prototype._setUnhandledRejectionIsNotified = function () {
this._bitField = this._bitField | 262144;
};
Promise.prototype._unsetUnhandledRejectionIsNotified = function () {
this._bitField = this._bitField & (~262144);
};
Promise.prototype._isUnhandledRejectionNotified = function () {
return (this._bitField & 262144) > 0;
};
Promise.prototype._setRejectionIsUnhandled = function () {
this._bitField = this._bitField | 1048576;
};
Promise.prototype._unsetRejectionIsUnhandled = function () {
this._bitField = this._bitField & (~1048576);
if (this._isUnhandledRejectionNotified()) {
this._unsetUnhandledRejectionIsNotified();
this._notifyUnhandledRejectionIsHandled();
}
};
Promise.prototype._isRejectionUnhandled = function () {
return (this._bitField & 1048576) > 0;
};
Promise.prototype._warn = function(message, shouldUseOwnTrace, promise) {
return warn(message, shouldUseOwnTrace, promise || this);
};
Promise.onPossiblyUnhandledRejection = function (fn) {
var context = Promise._getContext();
possiblyUnhandledRejection = util.contextBind(context, fn);
};
Promise.onUnhandledRejectionHandled = function (fn) {
var context = Promise._getContext();
unhandledRejectionHandled = util.contextBind(context, fn);
};
var disableLongStackTraces = function() {};
Promise.longStackTraces = function () {
if (async.haveItemsQueued() && !config.longStackTraces) {
throw new Error("cannot enable long stack traces after promises have been created\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
if (!config.longStackTraces && longStackTracesIsSupported()) {
var Promise_captureStackTrace = Promise.prototype._captureStackTrace;
var Promise_attachExtraTrace = Promise.prototype._attachExtraTrace;
var Promise_dereferenceTrace = Promise.prototype._dereferenceTrace;
config.longStackTraces = true;
disableLongStackTraces = function() {
if (async.haveItemsQueued() && !config.longStackTraces) {
throw new Error("cannot enable long stack traces after promises have been created\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
Promise.prototype._captureStackTrace = Promise_captureStackTrace;
Promise.prototype._attachExtraTrace = Promise_attachExtraTrace;
Promise.prototype._dereferenceTrace = Promise_dereferenceTrace;
Context.deactivateLongStackTraces();
config.longStackTraces = false;
};
Promise.prototype._captureStackTrace = longStackTracesCaptureStackTrace;
Promise.prototype._attachExtraTrace = longStackTracesAttachExtraTrace;
Promise.prototype._dereferenceTrace = longStackTracesDereferenceTrace;
Context.activateLongStackTraces();
}
};
Promise.hasLongStackTraces = function () {
return config.longStackTraces && longStackTracesIsSupported();
};
var legacyHandlers = {
unhandledrejection: {
before: function() {
var ret = util.global.onunhandledrejection;
util.global.onunhandledrejection = null;
return ret;
},
after: function(fn) {
util.global.onunhandledrejection = fn;
}
},
rejectionhandled: {
before: function() {
var ret = util.global.onrejectionhandled;
util.global.onrejectionhandled = null;
return ret;
},
after: function(fn) {
util.global.onrejectionhandled = fn;
}
}
};
var fireDomEvent = (function() {
var dispatch = function(legacy, e) {
if (legacy) {
var fn;
try {
fn = legacy.before();
return !util.global.dispatchEvent(e);
} finally {
legacy.after(fn);
}
} else {
return !util.global.dispatchEvent(e);
}
};
try {
if (typeof CustomEvent === "function") {
var event = new CustomEvent("CustomEvent");
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var eventData = {
detail: event,
cancelable: true
};
var domEvent = new CustomEvent(name, eventData);
es5.defineProperty(
domEvent, "promise", {value: event.promise});
es5.defineProperty(
domEvent, "reason", {value: event.reason});
return dispatch(legacyHandlers[name], domEvent);
};
} else if (typeof Event === "function") {
var event = new Event("CustomEvent");
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var domEvent = new Event(name, {
cancelable: true
});
domEvent.detail = event;
es5.defineProperty(domEvent, "promise", {value: event.promise});
es5.defineProperty(domEvent, "reason", {value: event.reason});
return dispatch(legacyHandlers[name], domEvent);
};
} else {
var event = document.createEvent("CustomEvent");
event.initCustomEvent("testingtheevent", false, true, {});
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var domEvent = document.createEvent("CustomEvent");
domEvent.initCustomEvent(name, false, true,
event);
return dispatch(legacyHandlers[name], domEvent);
};
}
} catch (e) {}
return function() {
return false;
};
})();
var fireGlobalEvent = (function() {
if (util.isNode) {
return function() {
return process.emit.apply(process, arguments);
};
} else {
if (!util.global) {
return function() {
return false;
};
}
return function(name) {
var methodName = "on" + name.toLowerCase();
var method = util.global[methodName];
if (!method) return false;
method.apply(util.global, [].slice.call(arguments, 1));
return true;
};
}
})();
function generatePromiseLifecycleEventObject(name, promise) {
return {promise: promise};
}
var eventToObjectGenerator = {
promiseCreated: generatePromiseLifecycleEventObject,
promiseFulfilled: generatePromiseLifecycleEventObject,
promiseRejected: generatePromiseLifecycleEventObject,
promiseResolved: generatePromiseLifecycleEventObject,
promiseCancelled: generatePromiseLifecycleEventObject,
promiseChained: function(name, promise, child) {
return {promise: promise, child: child};
},
warning: function(name, warning) {
return {warning: warning};
},
unhandledRejection: function (name, reason, promise) {
return {reason: reason, promise: promise};
},
rejectionHandled: generatePromiseLifecycleEventObject
};
var activeFireEvent = function (name) {
var globalEventFired = false;
try {
globalEventFired = fireGlobalEvent.apply(null, arguments);
} catch (e) {
async.throwLater(e);
globalEventFired = true;
}
var domEventFired = false;
try {
domEventFired = fireDomEvent(name,
eventToObjectGenerator[name].apply(null, arguments));
} catch (e) {
async.throwLater(e);
domEventFired = true;
}
return domEventFired || globalEventFired;
};
Promise.config = function(opts) {
opts = Object(opts);
if ("longStackTraces" in opts) {
if (opts.longStackTraces) {
Promise.longStackTraces();
} else if (!opts.longStackTraces && Promise.hasLongStackTraces()) {
disableLongStackTraces();
}
}
if ("warnings" in opts) {
var warningsOption = opts.warnings;
config.warnings = !!warningsOption;
wForgottenReturn = config.warnings;
if (util.isObject(warningsOption)) {
if ("wForgottenReturn" in warningsOption) {
wForgottenReturn = !!warningsOption.wForgottenReturn;
}
}
}
if ("cancellation" in opts && opts.cancellation && !config.cancellation) {
if (async.haveItemsQueued()) {
throw new Error(
"cannot enable cancellation after promises are in use");
}
Promise.prototype._clearCancellationData =
cancellationClearCancellationData;
Promise.prototype._propagateFrom = cancellationPropagateFrom;
Promise.prototype._onCancel = cancellationOnCancel;
Promise.prototype._setOnCancel = cancellationSetOnCancel;
Promise.prototype._attachCancellationCallback =
cancellationAttachCancellationCallback;
Promise.prototype._execute = cancellationExecute;
propagateFromFunction = cancellationPropagateFrom;
config.cancellation = true;
}
if ("monitoring" in opts) {
if (opts.monitoring && !config.monitoring) {
config.monitoring = true;
Promise.prototype._fireEvent = activeFireEvent;
} else if (!opts.monitoring && config.monitoring) {
config.monitoring = false;
Promise.prototype._fireEvent = defaultFireEvent;
}
}
if ("asyncHooks" in opts && util.nodeSupportsAsyncResource) {
var prev = config.asyncHooks;
var cur = !!opts.asyncHooks;
if (prev !== cur) {
config.asyncHooks = cur;
if (cur) {
enableAsyncHooks();
} else {
disableAsyncHooks();
}
}
}
return Promise;
};
function defaultFireEvent() { return false; }
Promise.prototype._fireEvent = defaultFireEvent;
Promise.prototype._execute = function(executor, resolve, reject) {
try {
executor(resolve, reject);
} catch (e) {
return e;
}
};
Promise.prototype._onCancel = function () {};
Promise.prototype._setOnCancel = function (handler) { ; };
Promise.prototype._attachCancellationCallback = function(onCancel) {
;
};
Promise.prototype._captureStackTrace = function () {};
Promise.prototype._attachExtraTrace = function () {};
Promise.prototype._dereferenceTrace = function () {};
Promise.prototype._clearCancellationData = function() {};
Promise.prototype._propagateFrom = function (parent, flags) {
;
;
};
function cancellationExecute(executor, resolve, reject) {
var promise = this;
try {
executor(resolve, reject, function(onCancel) {
if (typeof onCancel !== "function") {
throw new TypeError("onCancel must be a function, got: " +
util.toString(onCancel));
}
promise._attachCancellationCallback(onCancel);
});
} catch (e) {
return e;
}
}
function cancellationAttachCancellationCallback(onCancel) {
if (!this._isCancellable()) return this;
var previousOnCancel = this._onCancel();
if (previousOnCancel !== undefined) {
if (util.isArray(previousOnCancel)) {
previousOnCancel.push(onCancel);
} else {
this._setOnCancel([previousOnCancel, onCancel]);
}
} else {
this._setOnCancel(onCancel);
}
}
function cancellationOnCancel() {
return this._onCancelField;
}
function cancellationSetOnCancel(onCancel) {
this._onCancelField = onCancel;
}
function cancellationClearCancellationData() {
this._cancellationParent = undefined;
this._onCancelField = undefined;
}
function cancellationPropagateFrom(parent, flags) {
if ((flags & 1) !== 0) {
this._cancellationParent = parent;
var branchesRemainingToCancel = parent._branchesRemainingToCancel;
if (branchesRemainingToCancel === undefined) {
branchesRemainingToCancel = 0;
}
parent._branchesRemainingToCancel = branchesRemainingToCancel + 1;
}
if ((flags & 2) !== 0 && parent._isBound()) {
this._setBoundTo(parent._boundTo);
}
}
function bindingPropagateFrom(parent, flags) {
if ((flags & 2) !== 0 && parent._isBound()) {
this._setBoundTo(parent._boundTo);
}
}
var propagateFromFunction = bindingPropagateFrom;
function boundValueFunction() {
var ret = this._boundTo;
if (ret !== undefined) {
if (ret instanceof Promise) {
if (ret.isFulfilled()) {
return ret.value();
} else {
return undefined;
}
}
}
return ret;
}
function longStackTracesCaptureStackTrace() {
this._trace = new CapturedTrace(this._peekContext());
}
function longStackTracesAttachExtraTrace(error, ignoreSelf) {
if (canAttachTrace(error)) {
var trace = this._trace;
if (trace !== undefined) {
if (ignoreSelf) trace = trace._parent;
}
if (trace !== undefined) {
trace.attachExtraTrace(error);
} else if (!error.__stackCleaned__) {
var parsed = parseStackAndMessage(error);
util.notEnumerableProp(error, "stack",
parsed.message + "\n" + parsed.stack.join("\n"));
util.notEnumerableProp(error, "__stackCleaned__", true);
}
}
}
function longStackTracesDereferenceTrace() {
this._trace = undefined;
}
function checkForgottenReturns(returnValue, promiseCreated, name, promise,
parent) {
if (returnValue === undefined && promiseCreated !== null &&
wForgottenReturn) {
if (parent !== undefined && parent._returnedNonUndefined()) return;
if ((promise._bitField & 65535) === 0) return;
if (name) name = name + " ";
var handlerLine = "";
var creatorLine = "";
if (promiseCreated._trace) {
var traceLines = promiseCreated._trace.stack.split("\n");
var stack = cleanStack(traceLines);
for (var i = stack.length - 1; i >= 0; --i) {
var line = stack[i];
if (!nodeFramePattern.test(line)) {
var lineMatches = line.match(parseLinePattern);
if (lineMatches) {
handlerLine = "at " + lineMatches[1] +
":" + lineMatches[2] + ":" + lineMatches[3] + " ";
}
break;
}
}
if (stack.length > 0) {
var firstUserLine = stack[0];
for (var i = 0; i < traceLines.length; ++i) {
if (traceLines[i] === firstUserLine) {
if (i > 0) {
creatorLine = "\n" + traceLines[i - 1];
}
break;
}
}
}
}
var msg = "a promise was created in a " + name +
"handler " + handlerLine + "but was not returned from it, " +
"see http://goo.gl/rRqMUw" +
creatorLine;
promise._warn(msg, true, promiseCreated);
}
}
function deprecated(name, replacement) {
var message = name +
" is deprecated and will be removed in a future version.";
if (replacement) message += " Use " + replacement + " instead.";
return warn(message);
}
function warn(message, shouldUseOwnTrace, promise) {
if (!config.warnings) return;
var warning = new Warning(message);
var ctx;
if (shouldUseOwnTrace) {
promise._attachExtraTrace(warning);
} else if (config.longStackTraces && (ctx = Promise._peekContext())) {
ctx.attachExtraTrace(warning);
} else {
var parsed = parseStackAndMessage(warning);
warning.stack = parsed.message + "\n" + parsed.stack.join("\n");
}
if (!activeFireEvent("warning", warning)) {
formatAndLogError(warning, "", true);
}
}
function reconstructStack(message, stacks) {
for (var i = 0; i < stacks.length - 1; ++i) {
stacks[i].push("From previous event:");
stacks[i] = stacks[i].join("\n");
}
if (i < stacks.length) {
stacks[i] = stacks[i].join("\n");
}
return message + "\n" + stacks.join("\n");
}
function removeDuplicateOrEmptyJumps(stacks) {
for (var i = 0; i < stacks.length; ++i) {
if (stacks[i].length === 0 ||
((i + 1 < stacks.length) && stacks[i][0] === stacks[i+1][0])) {
stacks.splice(i, 1);
i--;
}
}
}
function removeCommonRoots(stacks) {
var current = stacks[0];
for (var i = 1; i < stacks.length; ++i) {
var prev = stacks[i];
var currentLastIndex = current.length - 1;
var currentLastLine = current[currentLastIndex];
var commonRootMeetPoint = -1;
for (var j = prev.length - 1; j >= 0; --j) {
if (prev[j] === currentLastLine) {
commonRootMeetPoint = j;
break;
}
}
for (var j = commonRootMeetPoint; j >= 0; --j) {
var line = prev[j];
if (current[currentLastIndex] === line) {
current.pop();
currentLastIndex--;
} else {
break;
}
}
current = prev;
}
}
function cleanStack(stack) {
var ret = [];
for (var i = 0; i < stack.length; ++i) {
var line = stack[i];
var isTraceLine = " (No stack trace)" === line ||
stackFramePattern.test(line);
var isInternalFrame = isTraceLine && shouldIgnore(line);
if (isTraceLine && !isInternalFrame) {
if (indentStackFrames && line.charAt(0) !== " ") {
line = " " + line;
}
ret.push(line);
}
}
return ret;
}
function stackFramesAsArray(error) {
var stack = error.stack.replace(/\s+$/g, "").split("\n");
for (var i = 0; i < stack.length; ++i) {
var line = stack[i];
if (" (No stack trace)" === line || stackFramePattern.test(line)) {
break;
}
}
if (i > 0 && error.name != "SyntaxError") {
stack = stack.slice(i);
}
return stack;
}
function parseStackAndMessage(error) {
var stack = error.stack;
var message = error.toString();
stack = typeof stack === "string" && stack.length > 0
? stackFramesAsArray(error) : [" (No stack trace)"];
return {
message: message,
stack: error.name == "SyntaxError" ? stack : cleanStack(stack)
};
}
function formatAndLogError(error, title, isSoft) {
if (typeof console !== "undefined") {
var message;
if (util.isObject(error)) {
var stack = error.stack;
message = title + formatStack(stack, error);
} else {
message = title + String(error);
}
if (typeof printWarning === "function") {
printWarning(message, isSoft);
} else if (typeof console.log === "function" ||
typeof console.log === "object") {
console.log(message);
}
}
}
function fireRejectionEvent(name, localHandler, reason, promise) {
var localEventFired = false;
try {
if (typeof localHandler === "function") {
localEventFired = true;
if (name === "rejectionHandled") {
localHandler(promise);
} else {
localHandler(reason, promise);
}
}
} catch (e) {
async.throwLater(e);
}
if (name === "unhandledRejection") {
if (!activeFireEvent(name, reason, promise) && !localEventFired) {
formatAndLogError(reason, "Unhandled rejection ");
}
} else {
activeFireEvent(name, promise);
}
}
function formatNonError(obj) {
var str;
if (typeof obj === "function") {
str = "[function " +
(obj.name || "anonymous") +
"]";
} else {
str = obj && typeof obj.toString === "function"
? obj.toString() : util.toString(obj);
var ruselessToString = /\[object [a-zA-Z0-9$_]+\]/;
if (ruselessToString.test(str)) {
try {
var newStr = JSON.stringify(obj);
str = newStr;
}
catch(e) {
}
}
if (str.length === 0) {
str = "(empty array)";
}
}
return ("(<" + snip(str) + ">, no stack trace)");
}
function snip(str) {
var maxChars = 41;
if (str.length < maxChars) {
return str;
}
return str.substr(0, maxChars - 3) + "...";
}
function longStackTracesIsSupported() {
return typeof captureStackTrace === "function";
}
var shouldIgnore = function() { return false; };
var parseLineInfoRegex = /[\/<\(]([^:\/]+):(\d+):(?:\d+)\)?\s*$/;
function parseLineInfo(line) {
var matches = line.match(parseLineInfoRegex);
if (matches) {
return {
fileName: matches[1],
line: parseInt(matches[2], 10)
};
}
}
function setBounds(firstLineError, lastLineError) {
if (!longStackTracesIsSupported()) return;
var firstStackLines = (firstLineError.stack || "").split("\n");
var lastStackLines = (lastLineError.stack || "").split("\n");
var firstIndex = -1;
var lastIndex = -1;
var firstFileName;
var lastFileName;
for (var i = 0; i < firstStackLines.length; ++i) {
var result = parseLineInfo(firstStackLines[i]);
if (result) {
firstFileName = result.fileName;
firstIndex = result.line;
break;
}
}
for (var i = 0; i < lastStackLines.length; ++i) {
var result = parseLineInfo(lastStackLines[i]);
if (result) {
lastFileName = result.fileName;
lastIndex = result.line;
break;
}
}
if (firstIndex < 0 || lastIndex < 0 || !firstFileName || !lastFileName ||
firstFileName !== lastFileName || firstIndex >= lastIndex) {
return;
}
shouldIgnore = function(line) {
if (bluebirdFramePattern.test(line)) return true;
var info = parseLineInfo(line);
if (info) {
if (info.fileName === firstFileName &&
(firstIndex <= info.line && info.line <= lastIndex)) {
return true;
}
}
return false;
};
}
function CapturedTrace(parent) {
this._parent = parent;
this._promisesCreated = 0;
var length = this._length = 1 + (parent === undefined ? 0 : parent._length);
captureStackTrace(this, CapturedTrace);
if (length > 32) this.uncycle();
}
util.inherits(CapturedTrace, Error);
Context.CapturedTrace = CapturedTrace;
CapturedTrace.prototype.uncycle = function() {
var length = this._length;
if (length < 2) return;
var nodes = [];
var stackToIndex = {};
for (var i = 0, node = this; node !== undefined; ++i) {
nodes.push(node);
node = node._parent;
}
length = this._length = i;
for (var i = length - 1; i >= 0; --i) {
var stack = nodes[i].stack;
if (stackToIndex[stack] === undefined) {
stackToIndex[stack] = i;
}
}
for (var i = 0; i < length; ++i) {
var currentStack = nodes[i].stack;
var index = stackToIndex[currentStack];
if (index !== undefined && index !== i) {
if (index > 0) {
nodes[index - 1]._parent = undefined;
nodes[index - 1]._length = 1;
}
nodes[i]._parent = undefined;
nodes[i]._length = 1;
var cycleEdgeNode = i > 0 ? nodes[i - 1] : this;
if (index < length - 1) {
cycleEdgeNode._parent = nodes[index + 1];
cycleEdgeNode._parent.uncycle();
cycleEdgeNode._length =
cycleEdgeNode._parent._length + 1;
} else {
cycleEdgeNode._parent = undefined;
cycleEdgeNode._length = 1;
}
var currentChildLength = cycleEdgeNode._length + 1;
for (var j = i - 2; j >= 0; --j) {
nodes[j]._length = currentChildLength;
currentChildLength++;
}
return;
}
}
};
CapturedTrace.prototype.attachExtraTrace = function(error) {
if (error.__stackCleaned__) return;
this.uncycle();
var parsed = parseStackAndMessage(error);
var message = parsed.message;
var stacks = [parsed.stack];
var trace = this;
while (trace !== undefined) {
stacks.push(cleanStack(trace.stack.split("\n")));
trace = trace._parent;
}
removeCommonRoots(stacks);
removeDuplicateOrEmptyJumps(stacks);
util.notEnumerableProp(error, "stack", reconstructStack(message, stacks));
util.notEnumerableProp(error, "__stackCleaned__", true);
};
var captureStackTrace = (function stackDetection() {
var v8stackFramePattern = /^\s*at\s*/;
var v8stackFormatter = function(stack, error) {
if (typeof stack === "string") return stack;
if (error.name !== undefined &&
error.message !== undefined) {
return error.toString();
}
return formatNonError(error);
};
if (typeof Error.stackTraceLimit === "number" &&
typeof Error.captureStackTrace === "function") {
Error.stackTraceLimit += 6;
stackFramePattern = v8stackFramePattern;
formatStack = v8stackFormatter;
var captureStackTrace = Error.captureStackTrace;
shouldIgnore = function(line) {
return bluebirdFramePattern.test(line);
};
return function(receiver, ignoreUntil) {
Error.stackTraceLimit += 6;
captureStackTrace(receiver, ignoreUntil);
Error.stackTraceLimit -= 6;
};
}
var err = new Error();
if (typeof err.stack === "string" &&
err.stack.split("\n")[0].indexOf("stackDetection@") >= 0) {
stackFramePattern = /@/;
formatStack = v8stackFormatter;
indentStackFrames = true;
return function captureStackTrace(o) {
o.stack = new Error().stack;
};
}
var hasStackAfterThrow;
try { throw new Error(); }
catch(e) {
hasStackAfterThrow = ("stack" in e);
}
if (!("stack" in err) && hasStackAfterThrow &&
typeof Error.stackTraceLimit === "number") {
stackFramePattern = v8stackFramePattern;
formatStack = v8stackFormatter;
return function captureStackTrace(o) {
Error.stackTraceLimit += 6;
try { throw new Error(); }
catch(e) { o.stack = e.stack; }
Error.stackTraceLimit -= 6;
};
}
formatStack = function(stack, error) {
if (typeof stack === "string") return stack;
if ((typeof error === "object" ||
typeof error === "function") &&
error.name !== undefined &&
error.message !== undefined) {
return error.toString();
}
return formatNonError(error);
};
return null;
})([]);
if (typeof console !== "undefined" && typeof console.warn !== "undefined") {
printWarning = function (message) {
console.warn(message);
};
if (util.isNode && process.stderr.isTTY) {
printWarning = function(message, isSoft) {
var color = isSoft ? "\u001b[33m" : "\u001b[31m";
console.warn(color + message + "\u001b[0m\n");
};
} else if (!util.isNode && typeof (new Error().stack) === "string") {
printWarning = function(message, isSoft) {
console.warn("%c" + message,
isSoft ? "color: darkorange" : "color: red");
};
}
}
var config = {
warnings: warnings,
longStackTraces: false,
cancellation: false,
monitoring: false,
asyncHooks: false
};
if (longStackTraces) Promise.longStackTraces();
return {
asyncHooks: function() {
return config.asyncHooks;
},
longStackTraces: function() {
return config.longStackTraces;
},
warnings: function() {
return config.warnings;
},
cancellation: function() {
return config.cancellation;
},
monitoring: function() {
return config.monitoring;
},
propagateFromFunction: function() {
return propagateFromFunction;
},
boundValueFunction: function() {
return boundValueFunction;
},
checkForgottenReturns: checkForgottenReturns,
setBounds: setBounds,
warn: warn,
deprecated: deprecated,
CapturedTrace: CapturedTrace,
fireDomEvent: fireDomEvent,
fireGlobalEvent: fireGlobalEvent
};
};
},{"./errors":9,"./es5":10,"./util":21}],8:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
function returner() {
return this.value;
}
function thrower() {
throw this.reason;
}
Promise.prototype["return"] =
Promise.prototype.thenReturn = function (value) {
if (value instanceof Promise) value.suppressUnhandledRejections();
return this._then(
returner, undefined, undefined, {value: value}, undefined);
};
Promise.prototype["throw"] =
Promise.prototype.thenThrow = function (reason) {
return this._then(
thrower, undefined, undefined, {reason: reason}, undefined);
};
Promise.prototype.catchThrow = function (reason) {
if (arguments.length <= 1) {
return this._then(
undefined, thrower, undefined, {reason: reason}, undefined);
} else {
var _reason = arguments[1];
var handler = function() {throw _reason;};
return this.caught(reason, handler);
}
};
Promise.prototype.catchReturn = function (value) {
if (arguments.length <= 1) {
if (value instanceof Promise) value.suppressUnhandledRejections();
return this._then(
undefined, returner, undefined, {value: value}, undefined);
} else {
var _value = arguments[1];
if (_value instanceof Promise) _value.suppressUnhandledRejections();
var handler = function() {return _value;};
return this.caught(value, handler);
}
};
};
},{}],9:[function(_dereq_,module,exports){
"use strict";
var es5 = _dereq_("./es5");
var Objectfreeze = es5.freeze;
var util = _dereq_("./util");
var inherits = util.inherits;
var notEnumerableProp = util.notEnumerableProp;
function subError(nameProperty, defaultMessage) {
function SubError(message) {
if (!(this instanceof SubError)) return new SubError(message);
notEnumerableProp(this, "message",
typeof message === "string" ? message : defaultMessage);
notEnumerableProp(this, "name", nameProperty);
if (Error.captureStackTrace) {
Error.captureStackTrace(this, this.constructor);
} else {
Error.call(this);
}
}
inherits(SubError, Error);
return SubError;
}
var _TypeError, _RangeError;
var Warning = subError("Warning", "warning");
var CancellationError = subError("CancellationError", "cancellation error");
var TimeoutError = subError("TimeoutError", "timeout error");
var AggregateError = subError("AggregateError", "aggregate error");
try {
_TypeError = TypeError;
_RangeError = RangeError;
} catch(e) {
_TypeError = subError("TypeError", "type error");
_RangeError = subError("RangeError", "range error");
}
var methods = ("join pop push shift unshift slice filter forEach some " +
"every map indexOf lastIndexOf reduce reduceRight sort reverse").split(" ");
for (var i = 0; i < methods.length; ++i) {
if (typeof Array.prototype[methods[i]] === "function") {
AggregateError.prototype[methods[i]] = Array.prototype[methods[i]];
}
}
es5.defineProperty(AggregateError.prototype, "length", {
value: 0,
configurable: false,
writable: true,
enumerable: true
});
AggregateError.prototype["isOperational"] = true;
var level = 0;
AggregateError.prototype.toString = function() {
var indent = Array(level * 4 + 1).join(" ");
var ret = "\n" + indent + "AggregateError of:" + "\n";
level++;
indent = Array(level * 4 + 1).join(" ");
for (var i = 0; i < this.length; ++i) {
var str = this[i] === this ? "[Circular AggregateError]" : this[i] + "";
var lines = str.split("\n");
for (var j = 0; j < lines.length; ++j) {
lines[j] = indent + lines[j];
}
str = lines.join("\n");
ret += str + "\n";
}
level--;
return ret;
};
function OperationalError(message) {
if (!(this instanceof OperationalError))
return new OperationalError(message);
notEnumerableProp(this, "name", "OperationalError");
notEnumerableProp(this, "message", message);
this.cause = message;
this["isOperational"] = true;
if (message instanceof Error) {
notEnumerableProp(this, "message", message.message);
notEnumerableProp(this, "stack", message.stack);
} else if (Error.captureStackTrace) {
Error.captureStackTrace(this, this.constructor);
}
}
inherits(OperationalError, Error);
var errorTypes = Error["__BluebirdErrorTypes__"];
if (!errorTypes) {
errorTypes = Objectfreeze({
CancellationError: CancellationError,
TimeoutError: TimeoutError,
OperationalError: OperationalError,
RejectionError: OperationalError,
AggregateError: AggregateError
});
es5.defineProperty(Error, "__BluebirdErrorTypes__", {
value: errorTypes,
writable: false,
enumerable: false,
configurable: false
});
}
module.exports = {
Error: Error,
TypeError: _TypeError,
RangeError: _RangeError,
CancellationError: errorTypes.CancellationError,
OperationalError: errorTypes.OperationalError,
TimeoutError: errorTypes.TimeoutError,
AggregateError: errorTypes.AggregateError,
Warning: Warning
};
},{"./es5":10,"./util":21}],10:[function(_dereq_,module,exports){
var isES5 = (function(){
"use strict";
return this === undefined;
})();
if (isES5) {
module.exports = {
freeze: Object.freeze,
defineProperty: Object.defineProperty,
getDescriptor: Object.getOwnPropertyDescriptor,
keys: Object.keys,
names: Object.getOwnPropertyNames,
getPrototypeOf: Object.getPrototypeOf,
isArray: Array.isArray,
isES5: isES5,
propertyIsWritable: function(obj, prop) {
var descriptor = Object.getOwnPropertyDescriptor(obj, prop);
return !!(!descriptor || descriptor.writable || descriptor.set);
}
};
} else {
var has = {}.hasOwnProperty;
var str = {}.toString;
var proto = {}.constructor.prototype;
var ObjectKeys = function (o) {
var ret = [];
for (var key in o) {
if (has.call(o, key)) {
ret.push(key);
}
}
return ret;
};
var ObjectGetDescriptor = function(o, key) {
return {value: o[key]};
};
var ObjectDefineProperty = function (o, key, desc) {
o[key] = desc.value;
return o;
};
var ObjectFreeze = function (obj) {
return obj;
};
var ObjectGetPrototypeOf = function (obj) {
try {
return Object(obj).constructor.prototype;
}
catch (e) {
return proto;
}
};
var ArrayIsArray = function (obj) {
try {
return str.call(obj) === "[object Array]";
}
catch(e) {
return false;
}
};
module.exports = {
isArray: ArrayIsArray,
keys: ObjectKeys,
names: ObjectKeys,
defineProperty: ObjectDefineProperty,
getDescriptor: ObjectGetDescriptor,
freeze: ObjectFreeze,
getPrototypeOf: ObjectGetPrototypeOf,
isES5: isES5,
propertyIsWritable: function() {
return true;
}
};
}
},{}],11:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, tryConvertToPromise, NEXT_FILTER) {
var util = _dereq_("./util");
var CancellationError = Promise.CancellationError;
var errorObj = util.errorObj;
var catchFilter = _dereq_("./catch_filter")(NEXT_FILTER);
function PassThroughHandlerContext(promise, type, handler) {
this.promise = promise;
this.type = type;
this.handler = handler;
this.called = false;
this.cancelPromise = null;
}
PassThroughHandlerContext.prototype.isFinallyHandler = function() {
return this.type === 0;
};
function FinallyHandlerCancelReaction(finallyHandler) {
this.finallyHandler = finallyHandler;
}
FinallyHandlerCancelReaction.prototype._resultCancelled = function() {
checkCancel(this.finallyHandler);
};
function checkCancel(ctx, reason) {
if (ctx.cancelPromise != null) {
if (arguments.length > 1) {
ctx.cancelPromise._reject(reason);
} else {
ctx.cancelPromise._cancel();
}
ctx.cancelPromise = null;
return true;
}
return false;
}
function succeed() {
return finallyHandler.call(this, this.promise._target()._settledValue());
}
function fail(reason) {
if (checkCancel(this, reason)) return;
errorObj.e = reason;
return errorObj;
}
function finallyHandler(reasonOrValue) {
var promise = this.promise;
var handler = this.handler;
if (!this.called) {
this.called = true;
var ret = this.isFinallyHandler()
? handler.call(promise._boundValue())
: handler.call(promise._boundValue(), reasonOrValue);
if (ret === NEXT_FILTER) {
return ret;
} else if (ret !== undefined) {
promise._setReturnedNonUndefined();
var maybePromise = tryConvertToPromise(ret, promise);
if (maybePromise instanceof Promise) {
if (this.cancelPromise != null) {
if (maybePromise._isCancelled()) {
var reason =
new CancellationError("late cancellation observer");
promise._attachExtraTrace(reason);
errorObj.e = reason;
return errorObj;
} else if (maybePromise.isPending()) {
maybePromise._attachCancellationCallback(
new FinallyHandlerCancelReaction(this));
}
}
return maybePromise._then(
succeed, fail, undefined, this, undefined);
}
}
}
if (promise.isRejected()) {
checkCancel(this);
errorObj.e = reasonOrValue;
return errorObj;
} else {
checkCancel(this);
return reasonOrValue;
}
}
Promise.prototype._passThrough = function(handler, type, success, fail) {
if (typeof handler !== "function") return this.then();
return this._then(success,
fail,
undefined,
new PassThroughHandlerContext(this, type, handler),
undefined);
};
Promise.prototype.lastly =
Promise.prototype["finally"] = function (handler) {
return this._passThrough(handler,
0,
finallyHandler,
finallyHandler);
};
Promise.prototype.tap = function (handler) {
return this._passThrough(handler, 1, finallyHandler);
};
Promise.prototype.tapCatch = function (handlerOrPredicate) {
var len = arguments.length;
if(len === 1) {
return this._passThrough(handlerOrPredicate,
1,
undefined,
finallyHandler);
} else {
var catchInstances = new Array(len - 1),
j = 0, i;
for (i = 0; i < len - 1; ++i) {
var item = arguments[i];
if (util.isObject(item)) {
catchInstances[j++] = item;
} else {
return Promise.reject(new TypeError(
"tapCatch statement predicate: "
+ "expecting an object but got " + util.classString(item)
));
}
}
catchInstances.length = j;
var handler = arguments[i];
return this._passThrough(catchFilter(catchInstances, handler, this),
1,
undefined,
finallyHandler);
}
};
return PassThroughHandlerContext;
};
},{"./catch_filter":5,"./util":21}],12:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, PromiseArray, tryConvertToPromise, INTERNAL, async) {
var util = _dereq_("./util");
var canEvaluate = util.canEvaluate;
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
var reject;
if (!true) {
if (canEvaluate) {
var thenCallback = function(i) {
return new Function("value", "holder", " \n\
'use strict'; \n\
holder.pIndex = value; \n\
holder.checkFulfillment(this); \n\
".replace(/Index/g, i));
};
var promiseSetter = function(i) {
return new Function("promise", "holder", " \n\
'use strict'; \n\
holder.pIndex = promise; \n\
".replace(/Index/g, i));
};
var generateHolderClass = function(total) {
var props = new Array(total);
for (var i = 0; i < props.length; ++i) {
props[i] = "this.p" + (i+1);
}
var assignment = props.join(" = ") + " = null;";
var cancellationCode= "var promise;\n" + props.map(function(prop) {
return " \n\
promise = " + prop + "; \n\
if (promise instanceof Promise) { \n\
promise.cancel(); \n\
} \n\
";
}).join("\n");
var passedArguments = props.join(", ");
var name = "Holder$" + total;
var code = "return function(tryCatch, errorObj, Promise, async) { \n\
'use strict'; \n\
function [TheName](fn) { \n\
[TheProperties] \n\
this.fn = fn; \n\
this.asyncNeeded = true; \n\
this.now = 0; \n\
} \n\
\n\
[TheName].prototype._callFunction = function(promise) { \n\
promise._pushContext(); \n\
var ret = tryCatch(this.fn)([ThePassedArguments]); \n\
promise._popContext(); \n\
if (ret === errorObj) { \n\
promise._rejectCallback(ret.e, false); \n\
} else { \n\
promise._resolveCallback(ret); \n\
} \n\
}; \n\
\n\
[TheName].prototype.checkFulfillment = function(promise) { \n\
var now = ++this.now; \n\
if (now === [TheTotal]) { \n\
if (this.asyncNeeded) { \n\
async.invoke(this._callFunction, this, promise); \n\
} else { \n\
this._callFunction(promise); \n\
} \n\
\n\
} \n\
}; \n\
\n\
[TheName].prototype._resultCancelled = function() { \n\
[CancellationCode] \n\
}; \n\
\n\
return [TheName]; \n\
}(tryCatch, errorObj, Promise, async); \n\
";
code = code.replace(/\[TheName\]/g, name)
.replace(/\[TheTotal\]/g, total)
.replace(/\[ThePassedArguments\]/g, passedArguments)
.replace(/\[TheProperties\]/g, assignment)
.replace(/\[CancellationCode\]/g, cancellationCode);
return new Function("tryCatch", "errorObj", "Promise", "async", code)
(tryCatch, errorObj, Promise, async);
};
var holderClasses = [];
var thenCallbacks = [];
var promiseSetters = [];
for (var i = 0; i < 8; ++i) {
holderClasses.push(generateHolderClass(i + 1));
thenCallbacks.push(thenCallback(i + 1));
promiseSetters.push(promiseSetter(i + 1));
}
reject = function (reason) {
this._reject(reason);
};
}}
Promise.join = function () {
var last = arguments.length - 1;
var fn;
if (last > 0 && typeof arguments[last] === "function") {
fn = arguments[last];
if (!true) {
if (last <= 8 && canEvaluate) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
var HolderClass = holderClasses[last - 1];
var holder = new HolderClass(fn);
var callbacks = thenCallbacks;
for (var i = 0; i < last; ++i) {
var maybePromise = tryConvertToPromise(arguments[i], ret);
if (maybePromise instanceof Promise) {
maybePromise = maybePromise._target();
var bitField = maybePromise._bitField;
;
if (((bitField & 50397184) === 0)) {
maybePromise._then(callbacks[i], reject,
undefined, ret, holder);
promiseSetters[i](maybePromise, holder);
holder.asyncNeeded = false;
} else if (((bitField & 33554432) !== 0)) {
callbacks[i].call(ret,
maybePromise._value(), holder);
} else if (((bitField & 16777216) !== 0)) {
ret._reject(maybePromise._reason());
} else {
ret._cancel();
}
} else {
callbacks[i].call(ret, maybePromise, holder);
}
}
if (!ret._isFateSealed()) {
if (holder.asyncNeeded) {
var context = Promise._getContext();
holder.fn = util.contextBind(context, holder.fn);
}
ret._setAsyncGuaranteed();
ret._setOnCancel(holder);
}
return ret;
}
}
}
var args = [].slice.call(arguments);;
if (fn) args.pop();
var ret = new PromiseArray(args).promise();
return fn !== undefined ? ret.spread(fn) : ret;
};
};
},{"./util":21}],13:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, INTERNAL, tryConvertToPromise, apiRejection, debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
Promise.method = function (fn) {
if (typeof fn !== "function") {
throw new Promise.TypeError("expecting a function but got " + util.classString(fn));
}
return function () {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._pushContext();
var value = tryCatch(fn).apply(this, arguments);
var promiseCreated = ret._popContext();
debug.checkForgottenReturns(
value, promiseCreated, "Promise.method", ret);
ret._resolveFromSyncValue(value);
return ret;
};
};
Promise.attempt = Promise["try"] = function (fn) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._pushContext();
var value;
if (arguments.length > 1) {
debug.deprecated("calling Promise.try with more than 1 argument");
var arg = arguments[1];
var ctx = arguments[2];
value = util.isArray(arg) ? tryCatch(fn).apply(ctx, arg)
: tryCatch(fn).call(ctx, arg);
} else {
value = tryCatch(fn)();
}
var promiseCreated = ret._popContext();
debug.checkForgottenReturns(
value, promiseCreated, "Promise.try", ret);
ret._resolveFromSyncValue(value);
return ret;
};
Promise.prototype._resolveFromSyncValue = function (value) {
if (value === util.errorObj) {
this._rejectCallback(value.e, false);
} else {
this._resolveCallback(value, true);
}
};
};
},{"./util":21}],14:[function(_dereq_,module,exports){
"use strict";
var util = _dereq_("./util");
var maybeWrapAsError = util.maybeWrapAsError;
var errors = _dereq_("./errors");
var OperationalError = errors.OperationalError;
var es5 = _dereq_("./es5");
function isUntypedError(obj) {
return obj instanceof Error &&
es5.getPrototypeOf(obj) === Error.prototype;
}
var rErrorKey = /^(?:name|message|stack|cause)$/;
function wrapAsOperationalError(obj) {
var ret;
if (isUntypedError(obj)) {
ret = new OperationalError(obj);
ret.name = obj.name;
ret.message = obj.message;
ret.stack = obj.stack;
var keys = es5.keys(obj);
for (var i = 0; i < keys.length; ++i) {
var key = keys[i];
if (!rErrorKey.test(key)) {
ret[key] = obj[key];
}
}
return ret;
}
util.markAsOriginatingFromRejection(obj);
return obj;
}
function nodebackForPromise(promise, multiArgs) {
return function(err, value) {
if (promise === null) return;
if (err) {
var wrapped = wrapAsOperationalError(maybeWrapAsError(err));
promise._attachExtraTrace(wrapped);
promise._reject(wrapped);
} else if (!multiArgs) {
promise._fulfill(value);
} else {
var args = [].slice.call(arguments, 1);;
promise._fulfill(args);
}
promise = null;
};
}
module.exports = nodebackForPromise;
},{"./errors":9,"./es5":10,"./util":21}],15:[function(_dereq_,module,exports){
"use strict";
module.exports = function() {
var makeSelfResolutionError = function () {
return new TypeError("circular promise resolution chain\u000a\u000a See http://goo.gl/MqrFmX\u000a");
};
var reflectHandler = function() {
return new Promise.PromiseInspection(this._target());
};
var apiRejection = function(msg) {
return Promise.reject(new TypeError(msg));
};
function Proxyable() {}
var UNDEFINED_BINDING = {};
var util = _dereq_("./util");
util.setReflectHandler(reflectHandler);
var getDomain = function() {
var domain = process.domain;
if (domain === undefined) {
return null;
}
return domain;
};
var getContextDefault = function() {
return null;
};
var getContextDomain = function() {
return {
domain: getDomain(),
async: null
};
};
var AsyncResource = util.isNode && util.nodeSupportsAsyncResource ?
_dereq_("async_hooks").AsyncResource : null;
var getContextAsyncHooks = function() {
return {
domain: getDomain(),
async: new AsyncResource("Bluebird::Promise")
};
};
var getContext = util.isNode ? getContextDomain : getContextDefault;
util.notEnumerableProp(Promise, "_getContext", getContext);
var enableAsyncHooks = function() {
getContext = getContextAsyncHooks;
util.notEnumerableProp(Promise, "_getContext", getContextAsyncHooks);
};
var disableAsyncHooks = function() {
getContext = getContextDomain;
util.notEnumerableProp(Promise, "_getContext", getContextDomain);
};
var es5 = _dereq_("./es5");
var Async = _dereq_("./async");
var async = new Async();
es5.defineProperty(Promise, "_async", {value: async});
var errors = _dereq_("./errors");
var TypeError = Promise.TypeError = errors.TypeError;
Promise.RangeError = errors.RangeError;
var CancellationError = Promise.CancellationError = errors.CancellationError;
Promise.TimeoutError = errors.TimeoutError;
Promise.OperationalError = errors.OperationalError;
Promise.RejectionError = errors.OperationalError;
Promise.AggregateError = errors.AggregateError;
var INTERNAL = function(){};
var APPLY = {};
var NEXT_FILTER = {};
var tryConvertToPromise = _dereq_("./thenables")(Promise, INTERNAL);
var PromiseArray =
_dereq_("./promise_array")(Promise, INTERNAL,
tryConvertToPromise, apiRejection, Proxyable);
var Context = _dereq_("./context")(Promise);
/*jshint unused:false*/
var createContext = Context.create;
var debug = _dereq_("./debuggability")(Promise, Context,
enableAsyncHooks, disableAsyncHooks);
var CapturedTrace = debug.CapturedTrace;
var PassThroughHandlerContext =
_dereq_("./finally")(Promise, tryConvertToPromise, NEXT_FILTER);
var catchFilter = _dereq_("./catch_filter")(NEXT_FILTER);
var nodebackForPromise = _dereq_("./nodeback");
var errorObj = util.errorObj;
var tryCatch = util.tryCatch;
function check(self, executor) {
if (self == null || self.constructor !== Promise) {
throw new TypeError("the promise constructor cannot be invoked directly\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
if (typeof executor !== "function") {
throw new TypeError("expecting a function but got " + util.classString(executor));
}
}
function Promise(executor) {
if (executor !== INTERNAL) {
check(this, executor);
}
this._bitField = 0;
this._fulfillmentHandler0 = undefined;
this._rejectionHandler0 = undefined;
this._promise0 = undefined;
this._receiver0 = undefined;
this._resolveFromExecutor(executor);
this._promiseCreated();
this._fireEvent("promiseCreated", this);
}
Promise.prototype.toString = function () {
return "[object Promise]";
};
Promise.prototype.caught = Promise.prototype["catch"] = function (fn) {
var len = arguments.length;
if (len > 1) {
var catchInstances = new Array(len - 1),
j = 0, i;
for (i = 0; i < len - 1; ++i) {
var item = arguments[i];
if (util.isObject(item)) {
catchInstances[j++] = item;
} else {
return apiRejection("Catch statement predicate: " +
"expecting an object but got " + util.classString(item));
}
}
catchInstances.length = j;
fn = arguments[i];
if (typeof fn !== "function") {
throw new TypeError("The last argument to .catch() " +
"must be a function, got " + util.toString(fn));
}
return this.then(undefined, catchFilter(catchInstances, fn, this));
}
return this.then(undefined, fn);
};
Promise.prototype.reflect = function () {
return this._then(reflectHandler,
reflectHandler, undefined, this, undefined);
};
Promise.prototype.then = function (didFulfill, didReject) {
if (debug.warnings() && arguments.length > 0 &&
typeof didFulfill !== "function" &&
typeof didReject !== "function") {
var msg = ".then() only accepts functions but was passed: " +
util.classString(didFulfill);
if (arguments.length > 1) {
msg += ", " + util.classString(didReject);
}
this._warn(msg);
}
return this._then(didFulfill, didReject, undefined, undefined, undefined);
};
Promise.prototype.done = function (didFulfill, didReject) {
var promise =
this._then(didFulfill, didReject, undefined, undefined, undefined);
promise._setIsFinal();
};
Promise.prototype.spread = function (fn) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
return this.all()._then(fn, undefined, undefined, APPLY, undefined);
};
Promise.prototype.toJSON = function () {
var ret = {
isFulfilled: false,
isRejected: false,
fulfillmentValue: undefined,
rejectionReason: undefined
};
if (this.isFulfilled()) {
ret.fulfillmentValue = this.value();
ret.isFulfilled = true;
} else if (this.isRejected()) {
ret.rejectionReason = this.reason();
ret.isRejected = true;
}
return ret;
};
Promise.prototype.all = function () {
if (arguments.length > 0) {
this._warn(".all() was passed arguments but it does not take any");
}
return new PromiseArray(this).promise();
};
Promise.prototype.error = function (fn) {
return this.caught(util.originatesFromRejection, fn);
};
Promise.getNewLibraryCopy = module.exports;
Promise.is = function (val) {
return val instanceof Promise;
};
Promise.fromNode = Promise.fromCallback = function(fn) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
var multiArgs = arguments.length > 1 ? !!Object(arguments[1]).multiArgs
: false;
var result = tryCatch(fn)(nodebackForPromise(ret, multiArgs));
if (result === errorObj) {
ret._rejectCallback(result.e, true);
}
if (!ret._isFateSealed()) ret._setAsyncGuaranteed();
return ret;
};
Promise.all = function (promises) {
return new PromiseArray(promises).promise();
};
Promise.cast = function (obj) {
var ret = tryConvertToPromise(obj);
if (!(ret instanceof Promise)) {
ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._setFulfilled();
ret._rejectionHandler0 = obj;
}
return ret;
};
Promise.resolve = Promise.fulfilled = Promise.cast;
Promise.reject = Promise.rejected = function (reason) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._rejectCallback(reason, true);
return ret;
};
Promise.setScheduler = function(fn) {
if (typeof fn !== "function") {
throw new TypeError("expecting a function but got " + util.classString(fn));
}
return async.setScheduler(fn);
};
Promise.prototype._then = function (
didFulfill,
didReject,
_, receiver,
internalData
) {
var haveInternalData = internalData !== undefined;
var promise = haveInternalData ? internalData : new Promise(INTERNAL);
var target = this._target();
var bitField = target._bitField;
if (!haveInternalData) {
promise._propagateFrom(this, 3);
promise._captureStackTrace();
if (receiver === undefined &&
((this._bitField & 2097152) !== 0)) {
if (!((bitField & 50397184) === 0)) {
receiver = this._boundValue();
} else {
receiver = target === this ? undefined : this._boundTo;
}
}
this._fireEvent("promiseChained", this, promise);
}
var context = getContext();
if (!((bitField & 50397184) === 0)) {
var handler, value, settler = target._settlePromiseCtx;
if (((bitField & 33554432) !== 0)) {
value = target._rejectionHandler0;
handler = didFulfill;
} else if (((bitField & 16777216) !== 0)) {
value = target._fulfillmentHandler0;
handler = didReject;
target._unsetRejectionIsUnhandled();
} else {
settler = target._settlePromiseLateCancellationObserver;
value = new CancellationError("late cancellation observer");
target._attachExtraTrace(value);
handler = didReject;
}
async.invoke(settler, target, {
handler: util.contextBind(context, handler),
promise: promise,
receiver: receiver,
value: value
});
} else {
target._addCallbacks(didFulfill, didReject, promise,
receiver, context);
}
return promise;
};
Promise.prototype._length = function () {
return this._bitField & 65535;
};
Promise.prototype._isFateSealed = function () {
return (this._bitField & 117506048) !== 0;
};
Promise.prototype._isFollowing = function () {
return (this._bitField & 67108864) === 67108864;
};
Promise.prototype._setLength = function (len) {
this._bitField = (this._bitField & -65536) |
(len & 65535);
};
Promise.prototype._setFulfilled = function () {
this._bitField = this._bitField | 33554432;
this._fireEvent("promiseFulfilled", this);
};
Promise.prototype._setRejected = function () {
this._bitField = this._bitField | 16777216;
this._fireEvent("promiseRejected", this);
};
Promise.prototype._setFollowing = function () {
this._bitField = this._bitField | 67108864;
this._fireEvent("promiseResolved", this);
};
Promise.prototype._setIsFinal = function () {
this._bitField = this._bitField | 4194304;
};
Promise.prototype._isFinal = function () {
return (this._bitField & 4194304) > 0;
};
Promise.prototype._unsetCancelled = function() {
this._bitField = this._bitField & (~65536);
};
Promise.prototype._setCancelled = function() {
this._bitField = this._bitField | 65536;
this._fireEvent("promiseCancelled", this);
};
Promise.prototype._setWillBeCancelled = function() {
this._bitField = this._bitField | 8388608;
};
Promise.prototype._setAsyncGuaranteed = function() {
if (async.hasCustomScheduler()) return;
var bitField = this._bitField;
this._bitField = bitField |
(((bitField & 536870912) >> 2) ^
134217728);
};
Promise.prototype._setNoAsyncGuarantee = function() {
this._bitField = (this._bitField | 536870912) &
(~134217728);
};
Promise.prototype._receiverAt = function (index) {
var ret = index === 0 ? this._receiver0 : this[
index * 4 - 4 + 3];
if (ret === UNDEFINED_BINDING) {
return undefined;
} else if (ret === undefined && this._isBound()) {
return this._boundValue();
}
return ret;
};
Promise.prototype._promiseAt = function (index) {
return this[
index * 4 - 4 + 2];
};
Promise.prototype._fulfillmentHandlerAt = function (index) {
return this[
index * 4 - 4 + 0];
};
Promise.prototype._rejectionHandlerAt = function (index) {
return this[
index * 4 - 4 + 1];
};
Promise.prototype._boundValue = function() {};
Promise.prototype._migrateCallback0 = function (follower) {
var bitField = follower._bitField;
var fulfill = follower._fulfillmentHandler0;
var reject = follower._rejectionHandler0;
var promise = follower._promise0;
var receiver = follower._receiverAt(0);
if (receiver === undefined) receiver = UNDEFINED_BINDING;
this._addCallbacks(fulfill, reject, promise, receiver, null);
};
Promise.prototype._migrateCallbackAt = function (follower, index) {
var fulfill = follower._fulfillmentHandlerAt(index);
var reject = follower._rejectionHandlerAt(index);
var promise = follower._promiseAt(index);
var receiver = follower._receiverAt(index);
if (receiver === undefined) receiver = UNDEFINED_BINDING;
this._addCallbacks(fulfill, reject, promise, receiver, null);
};
Promise.prototype._addCallbacks = function (
fulfill,
reject,
promise,
receiver,
context
) {
var index = this._length();
if (index >= 65535 - 4) {
index = 0;
this._setLength(0);
}
if (index === 0) {
this._promise0 = promise;
this._receiver0 = receiver;
if (typeof fulfill === "function") {
this._fulfillmentHandler0 = util.contextBind(context, fulfill);
}
if (typeof reject === "function") {
this._rejectionHandler0 = util.contextBind(context, reject);
}
} else {
var base = index * 4 - 4;
this[base + 2] = promise;
this[base + 3] = receiver;
if (typeof fulfill === "function") {
this[base + 0] =
util.contextBind(context, fulfill);
}
if (typeof reject === "function") {
this[base + 1] =
util.contextBind(context, reject);
}
}
this._setLength(index + 1);
return index;
};
Promise.prototype._proxy = function (proxyable, arg) {
this._addCallbacks(undefined, undefined, arg, proxyable, null);
};
Promise.prototype._resolveCallback = function(value, shouldBind) {
if (((this._bitField & 117506048) !== 0)) return;
if (value === this)
return this._rejectCallback(makeSelfResolutionError(), false);
var maybePromise = tryConvertToPromise(value, this);
if (!(maybePromise instanceof Promise)) return this._fulfill(value);
if (shouldBind) this._propagateFrom(maybePromise, 2);
var promise = maybePromise._target();
if (promise === this) {
this._reject(makeSelfResolutionError());
return;
}
var bitField = promise._bitField;
if (((bitField & 50397184) === 0)) {
var len = this._length();
if (len > 0) promise._migrateCallback0(this);
for (var i = 1; i < len; ++i) {
promise._migrateCallbackAt(this, i);
}
this._setFollowing();
this._setLength(0);
this._setFollowee(maybePromise);
} else if (((bitField & 33554432) !== 0)) {
this._fulfill(promise._value());
} else if (((bitField & 16777216) !== 0)) {
this._reject(promise._reason());
} else {
var reason = new CancellationError("late cancellation observer");
promise._attachExtraTrace(reason);
this._reject(reason);
}
};
Promise.prototype._rejectCallback =
function(reason, synchronous, ignoreNonErrorWarnings) {
var trace = util.ensureErrorObject(reason);
var hasStack = trace === reason;
if (!hasStack && !ignoreNonErrorWarnings && debug.warnings()) {
var message = "a promise was rejected with a non-error: " +
util.classString(reason);
this._warn(message, true);
}
this._attachExtraTrace(trace, synchronous ? hasStack : false);
this._reject(reason);
};
Promise.prototype._resolveFromExecutor = function (executor) {
if (executor === INTERNAL) return;
var promise = this;
this._captureStackTrace();
this._pushContext();
var synchronous = true;
var r = this._execute(executor, function(value) {
promise._resolveCallback(value);
}, function (reason) {
promise._rejectCallback(reason, synchronous);
});
synchronous = false;
this._popContext();
if (r !== undefined) {
promise._rejectCallback(r, true);
}
};
Promise.prototype._settlePromiseFromHandler = function (
handler, receiver, value, promise
) {
var bitField = promise._bitField;
if (((bitField & 65536) !== 0)) return;
promise._pushContext();
var x;
if (receiver === APPLY) {
if (!value || typeof value.length !== "number") {
x = errorObj;
x.e = new TypeError("cannot .spread() a non-array: " +
util.classString(value));
} else {
x = tryCatch(handler).apply(this._boundValue(), value);
}
} else {
x = tryCatch(handler).call(receiver, value);
}
var promiseCreated = promise._popContext();
bitField = promise._bitField;
if (((bitField & 65536) !== 0)) return;
if (x === NEXT_FILTER) {
promise._reject(value);
} else if (x === errorObj) {
promise._rejectCallback(x.e, false);
} else {
debug.checkForgottenReturns(x, promiseCreated, "", promise, this);
promise._resolveCallback(x);
}
};
Promise.prototype._target = function() {
var ret = this;
while (ret._isFollowing()) ret = ret._followee();
return ret;
};
Promise.prototype._followee = function() {
return this._rejectionHandler0;
};
Promise.prototype._setFollowee = function(promise) {
this._rejectionHandler0 = promise;
};
Promise.prototype._settlePromise = function(promise, handler, receiver, value) {
var isPromise = promise instanceof Promise;
var bitField = this._bitField;
var asyncGuaranteed = ((bitField & 134217728) !== 0);
if (((bitField & 65536) !== 0)) {
if (isPromise) promise._invokeInternalOnCancel();
if (receiver instanceof PassThroughHandlerContext &&
receiver.isFinallyHandler()) {
receiver.cancelPromise = promise;
if (tryCatch(handler).call(receiver, value) === errorObj) {
promise._reject(errorObj.e);
}
} else if (handler === reflectHandler) {
promise._fulfill(reflectHandler.call(receiver));
} else if (receiver instanceof Proxyable) {
receiver._promiseCancelled(promise);
} else if (isPromise || promise instanceof PromiseArray) {
promise._cancel();
} else {
receiver.cancel();
}
} else if (typeof handler === "function") {
if (!isPromise) {
handler.call(receiver, value, promise);
} else {
if (asyncGuaranteed) promise._setAsyncGuaranteed();
this._settlePromiseFromHandler(handler, receiver, value, promise);
}
} else if (receiver instanceof Proxyable) {
if (!receiver._isResolved()) {
if (((bitField & 33554432) !== 0)) {
receiver._promiseFulfilled(value, promise);
} else {
receiver._promiseRejected(value, promise);
}
}
} else if (isPromise) {
if (asyncGuaranteed) promise._setAsyncGuaranteed();
if (((bitField & 33554432) !== 0)) {
promise._fulfill(value);
} else {
promise._reject(value);
}
}
};
Promise.prototype._settlePromiseLateCancellationObserver = function(ctx) {
var handler = ctx.handler;
var promise = ctx.promise;
var receiver = ctx.receiver;
var value = ctx.value;
if (typeof handler === "function") {
if (!(promise instanceof Promise)) {
handler.call(receiver, value, promise);
} else {
this._settlePromiseFromHandler(handler, receiver, value, promise);
}
} else if (promise instanceof Promise) {
promise._reject(value);
}
};
Promise.prototype._settlePromiseCtx = function(ctx) {
this._settlePromise(ctx.promise, ctx.handler, ctx.receiver, ctx.value);
};
Promise.prototype._settlePromise0 = function(handler, value, bitField) {
var promise = this._promise0;
var receiver = this._receiverAt(0);
this._promise0 = undefined;
this._receiver0 = undefined;
this._settlePromise(promise, handler, receiver, value);
};
Promise.prototype._clearCallbackDataAtIndex = function(index) {
var base = index * 4 - 4;
this[base + 2] =
this[base + 3] =
this[base + 0] =
this[base + 1] = undefined;
};
Promise.prototype._fulfill = function (value) {
var bitField = this._bitField;
if (((bitField & 117506048) >>> 16)) return;
if (value === this) {
var err = makeSelfResolutionError();
this._attachExtraTrace(err);
return this._reject(err);
}
this._setFulfilled();
this._rejectionHandler0 = value;
if ((bitField & 65535) > 0) {
if (((bitField & 134217728) !== 0)) {
this._settlePromises();
} else {
async.settlePromises(this);
}
this._dereferenceTrace();
}
};
Promise.prototype._reject = function (reason) {
var bitField = this._bitField;
if (((bitField & 117506048) >>> 16)) return;
this._setRejected();
this._fulfillmentHandler0 = reason;
if (this._isFinal()) {
return async.fatalError(reason, util.isNode);
}
if ((bitField & 65535) > 0) {
async.settlePromises(this);
} else {
this._ensurePossibleRejectionHandled();
}
};
Promise.prototype._fulfillPromises = function (len, value) {
for (var i = 1; i < len; i++) {
var handler = this._fulfillmentHandlerAt(i);
var promise = this._promiseAt(i);
var receiver = this._receiverAt(i);
this._clearCallbackDataAtIndex(i);
this._settlePromise(promise, handler, receiver, value);
}
};
Promise.prototype._rejectPromises = function (len, reason) {
for (var i = 1; i < len; i++) {
var handler = this._rejectionHandlerAt(i);
var promise = this._promiseAt(i);
var receiver = this._receiverAt(i);
this._clearCallbackDataAtIndex(i);
this._settlePromise(promise, handler, receiver, reason);
}
};
Promise.prototype._settlePromises = function () {
var bitField = this._bitField;
var len = (bitField & 65535);
if (len > 0) {
if (((bitField & 16842752) !== 0)) {
var reason = this._fulfillmentHandler0;
this._settlePromise0(this._rejectionHandler0, reason, bitField);
this._rejectPromises(len, reason);
} else {
var value = this._rejectionHandler0;
this._settlePromise0(this._fulfillmentHandler0, value, bitField);
this._fulfillPromises(len, value);
}
this._setLength(0);
}
this._clearCancellationData();
};
Promise.prototype._settledValue = function() {
var bitField = this._bitField;
if (((bitField & 33554432) !== 0)) {
return this._rejectionHandler0;
} else if (((bitField & 16777216) !== 0)) {
return this._fulfillmentHandler0;
}
};
if (typeof Symbol !== "undefined" && Symbol.toStringTag) {
es5.defineProperty(Promise.prototype, Symbol.toStringTag, {
get: function () {
return "Object";
}
});
}
function deferResolve(v) {this.promise._resolveCallback(v);}
function deferReject(v) {this.promise._rejectCallback(v, false);}
Promise.defer = Promise.pending = function() {
debug.deprecated("Promise.defer", "new Promise");
var promise = new Promise(INTERNAL);
return {
promise: promise,
resolve: deferResolve,
reject: deferReject
};
};
util.notEnumerableProp(Promise,
"_makeSelfResolutionError",
makeSelfResolutionError);
_dereq_("./method")(Promise, INTERNAL, tryConvertToPromise, apiRejection,
debug);
_dereq_("./bind")(Promise, INTERNAL, tryConvertToPromise, debug);
_dereq_("./cancel")(Promise, PromiseArray, apiRejection, debug);
_dereq_("./direct_resolve")(Promise);
_dereq_("./synchronous_inspection")(Promise);
_dereq_("./join")(
Promise, PromiseArray, tryConvertToPromise, INTERNAL, async);
Promise.Promise = Promise;
Promise.version = "3.7.2";
util.toFastProperties(Promise);
util.toFastProperties(Promise.prototype);
function fillTypes(value) {
var p = new Promise(INTERNAL);
p._fulfillmentHandler0 = value;
p._rejectionHandler0 = value;
p._promise0 = value;
p._receiver0 = value;
}
// Complete slack tracking, opt out of field-type tracking and
// stabilize map
fillTypes({a: 1});
fillTypes({b: 2});
fillTypes({c: 3});
fillTypes(1);
fillTypes(function(){});
fillTypes(undefined);
fillTypes(false);
fillTypes(new Promise(INTERNAL));
debug.setBounds(Async.firstLineError, util.lastLineError);
return Promise;
};
},{"./async":1,"./bind":2,"./cancel":4,"./catch_filter":5,"./context":6,"./debuggability":7,"./direct_resolve":8,"./errors":9,"./es5":10,"./finally":11,"./join":12,"./method":13,"./nodeback":14,"./promise_array":16,"./synchronous_inspection":19,"./thenables":20,"./util":21,"async_hooks":undefined}],16:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL, tryConvertToPromise,
apiRejection, Proxyable) {
var util = _dereq_("./util");
var isArray = util.isArray;
function toResolutionValue(val) {
switch(val) {
case -2: return [];
case -3: return {};
case -6: return new Map();
}
}
function PromiseArray(values) {
var promise = this._promise = new Promise(INTERNAL);
if (values instanceof Promise) {
promise._propagateFrom(values, 3);
values.suppressUnhandledRejections();
}
promise._setOnCancel(this);
this._values = values;
this._length = 0;
this._totalResolved = 0;
this._init(undefined, -2);
}
util.inherits(PromiseArray, Proxyable);
PromiseArray.prototype.length = function () {
return this._length;
};
PromiseArray.prototype.promise = function () {
return this._promise;
};
PromiseArray.prototype._init = function init(_, resolveValueIfEmpty) {
var values = tryConvertToPromise(this._values, this._promise);
if (values instanceof Promise) {
values = values._target();
var bitField = values._bitField;
;
this._values = values;
if (((bitField & 50397184) === 0)) {
this._promise._setAsyncGuaranteed();
return values._then(
init,
this._reject,
undefined,
this,
resolveValueIfEmpty
);
} else if (((bitField & 33554432) !== 0)) {
values = values._value();
} else if (((bitField & 16777216) !== 0)) {
return this._reject(values._reason());
} else {
return this._cancel();
}
}
values = util.asArray(values);
if (values === null) {
var err = apiRejection(
"expecting an array or an iterable object but got " + util.classString(values)).reason();
this._promise._rejectCallback(err, false);
return;
}
if (values.length === 0) {
if (resolveValueIfEmpty === -5) {
this._resolveEmptyArray();
}
else {
this._resolve(toResolutionValue(resolveValueIfEmpty));
}
return;
}
this._iterate(values);
};
PromiseArray.prototype._iterate = function(values) {
var len = this.getActualLength(values.length);
this._length = len;
this._values = this.shouldCopyValues() ? new Array(len) : this._values;
var result = this._promise;
var isResolved = false;
var bitField = null;
for (var i = 0; i < len; ++i) {
var maybePromise = tryConvertToPromise(values[i], result);
if (maybePromise instanceof Promise) {
maybePromise = maybePromise._target();
bitField = maybePromise._bitField;
} else {
bitField = null;
}
if (isResolved) {
if (bitField !== null) {
maybePromise.suppressUnhandledRejections();
}
} else if (bitField !== null) {
if (((bitField & 50397184) === 0)) {
maybePromise._proxy(this, i);
this._values[i] = maybePromise;
} else if (((bitField & 33554432) !== 0)) {
isResolved = this._promiseFulfilled(maybePromise._value(), i);
} else if (((bitField & 16777216) !== 0)) {
isResolved = this._promiseRejected(maybePromise._reason(), i);
} else {
isResolved = this._promiseCancelled(i);
}
} else {
isResolved = this._promiseFulfilled(maybePromise, i);
}
}
if (!isResolved) result._setAsyncGuaranteed();
};
PromiseArray.prototype._isResolved = function () {
return this._values === null;
};
PromiseArray.prototype._resolve = function (value) {
this._values = null;
this._promise._fulfill(value);
};
PromiseArray.prototype._cancel = function() {
if (this._isResolved() || !this._promise._isCancellable()) return;
this._values = null;
this._promise._cancel();
};
PromiseArray.prototype._reject = function (reason) {
this._values = null;
this._promise._rejectCallback(reason, false);
};
PromiseArray.prototype._promiseFulfilled = function (value, index) {
this._values[index] = value;
var totalResolved = ++this._totalResolved;
if (totalResolved >= this._length) {
this._resolve(this._values);
return true;
}
return false;
};
PromiseArray.prototype._promiseCancelled = function() {
this._cancel();
return true;
};
PromiseArray.prototype._promiseRejected = function (reason) {
this._totalResolved++;
this._reject(reason);
return true;
};
PromiseArray.prototype._resultCancelled = function() {
if (this._isResolved()) return;
var values = this._values;
this._cancel();
if (values instanceof Promise) {
values.cancel();
} else {
for (var i = 0; i < values.length; ++i) {
if (values[i] instanceof Promise) {
values[i].cancel();
}
}
}
};
PromiseArray.prototype.shouldCopyValues = function () {
return true;
};
PromiseArray.prototype.getActualLength = function (len) {
return len;
};
return PromiseArray;
};
},{"./util":21}],17:[function(_dereq_,module,exports){
"use strict";
function arrayMove(src, srcIndex, dst, dstIndex, len) {
for (var j = 0; j < len; ++j) {
dst[j + dstIndex] = src[j + srcIndex];
src[j + srcIndex] = void 0;
}
}
function Queue(capacity) {
this._capacity = capacity;
this._length = 0;
this._front = 0;
}
Queue.prototype._willBeOverCapacity = function (size) {
return this._capacity < size;
};
Queue.prototype._pushOne = function (arg) {
var length = this.length();
this._checkCapacity(length + 1);
var i = (this._front + length) & (this._capacity - 1);
this[i] = arg;
this._length = length + 1;
};
Queue.prototype.push = function (fn, receiver, arg) {
var length = this.length() + 3;
if (this._willBeOverCapacity(length)) {
this._pushOne(fn);
this._pushOne(receiver);
this._pushOne(arg);
return;
}
var j = this._front + length - 3;
this._checkCapacity(length);
var wrapMask = this._capacity - 1;
this[(j + 0) & wrapMask] = fn;
this[(j + 1) & wrapMask] = receiver;
this[(j + 2) & wrapMask] = arg;
this._length = length;
};
Queue.prototype.shift = function () {
var front = this._front,
ret = this[front];
this[front] = undefined;
this._front = (front + 1) & (this._capacity - 1);
this._length--;
return ret;
};
Queue.prototype.length = function () {
return this._length;
};
Queue.prototype._checkCapacity = function (size) {
if (this._capacity < size) {
this._resizeTo(this._capacity << 1);
}
};
Queue.prototype._resizeTo = function (capacity) {
var oldCapacity = this._capacity;
this._capacity = capacity;
var front = this._front;
var length = this._length;
var moveItemsCount = (front + length) & (oldCapacity - 1);
arrayMove(this, 0, this, oldCapacity, moveItemsCount);
};
module.exports = Queue;
},{}],18:[function(_dereq_,module,exports){
"use strict";
var util = _dereq_("./util");
var schedule;
var noAsyncScheduler = function() {
throw new Error("No async scheduler available\u000a\u000a See http://goo.gl/MqrFmX\u000a");
};
var NativePromise = util.getNativePromise();
if (util.isNode && typeof MutationObserver === "undefined") {
var GlobalSetImmediate = global.setImmediate;
var ProcessNextTick = process.nextTick;
schedule = util.isRecentNode
? function(fn) { GlobalSetImmediate.call(global, fn); }
: function(fn) { ProcessNextTick.call(process, fn); };
} else if (typeof NativePromise === "function" &&
typeof NativePromise.resolve === "function") {
var nativePromise = NativePromise.resolve();
schedule = function(fn) {
nativePromise.then(fn);
};
} else if ((typeof MutationObserver !== "undefined") &&
!(typeof window !== "undefined" &&
window.navigator &&
(window.navigator.standalone || window.cordova)) &&
("classList" in document.documentElement)) {
schedule = (function() {
var div = document.createElement("div");
var opts = {attributes: true};
var toggleScheduled = false;
var div2 = document.createElement("div");
var o2 = new MutationObserver(function() {
div.classList.toggle("foo");
toggleScheduled = false;
});
o2.observe(div2, opts);
var scheduleToggle = function() {
if (toggleScheduled) return;
toggleScheduled = true;
div2.classList.toggle("foo");
};
return function schedule(fn) {
var o = new MutationObserver(function() {
o.disconnect();
fn();
});
o.observe(div, opts);
scheduleToggle();
};
})();
} else if (typeof setImmediate !== "undefined") {
schedule = function (fn) {
setImmediate(fn);
};
} else if (typeof setTimeout !== "undefined") {
schedule = function (fn) {
setTimeout(fn, 0);
};
} else {
schedule = noAsyncScheduler;
}
module.exports = schedule;
},{"./util":21}],19:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
function PromiseInspection(promise) {
if (promise !== undefined) {
promise = promise._target();
this._bitField = promise._bitField;
this._settledValueField = promise._isFateSealed()
? promise._settledValue() : undefined;
}
else {
this._bitField = 0;
this._settledValueField = undefined;
}
}
PromiseInspection.prototype._settledValue = function() {
return this._settledValueField;
};
var value = PromiseInspection.prototype.value = function () {
if (!this.isFulfilled()) {
throw new TypeError("cannot get fulfillment value of a non-fulfilled promise\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
return this._settledValue();
};
var reason = PromiseInspection.prototype.error =
PromiseInspection.prototype.reason = function () {
if (!this.isRejected()) {
throw new TypeError("cannot get rejection reason of a non-rejected promise\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
return this._settledValue();
};
var isFulfilled = PromiseInspection.prototype.isFulfilled = function() {
return (this._bitField & 33554432) !== 0;
};
var isRejected = PromiseInspection.prototype.isRejected = function () {
return (this._bitField & 16777216) !== 0;
};
var isPending = PromiseInspection.prototype.isPending = function () {
return (this._bitField & 50397184) === 0;
};
var isResolved = PromiseInspection.prototype.isResolved = function () {
return (this._bitField & 50331648) !== 0;
};
PromiseInspection.prototype.isCancelled = function() {
return (this._bitField & 8454144) !== 0;
};
Promise.prototype.__isCancelled = function() {
return (this._bitField & 65536) === 65536;
};
Promise.prototype._isCancelled = function() {
return this._target().__isCancelled();
};
Promise.prototype.isCancelled = function() {
return (this._target()._bitField & 8454144) !== 0;
};
Promise.prototype.isPending = function() {
return isPending.call(this._target());
};
Promise.prototype.isRejected = function() {
return isRejected.call(this._target());
};
Promise.prototype.isFulfilled = function() {
return isFulfilled.call(this._target());
};
Promise.prototype.isResolved = function() {
return isResolved.call(this._target());
};
Promise.prototype.value = function() {
return value.call(this._target());
};
Promise.prototype.reason = function() {
var target = this._target();
target._unsetRejectionIsUnhandled();
return reason.call(target);
};
Promise.prototype._value = function() {
return this._settledValue();
};
Promise.prototype._reason = function() {
this._unsetRejectionIsUnhandled();
return this._settledValue();
};
Promise.PromiseInspection = PromiseInspection;
};
},{}],20:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL) {
var util = _dereq_("./util");
var errorObj = util.errorObj;
var isObject = util.isObject;
function tryConvertToPromise(obj, context) {
if (isObject(obj)) {
if (obj instanceof Promise) return obj;
var then = getThen(obj);
if (then === errorObj) {
if (context) context._pushContext();
var ret = Promise.reject(then.e);
if (context) context._popContext();
return ret;
} else if (typeof then === "function") {
if (isAnyBluebirdPromise(obj)) {
var ret = new Promise(INTERNAL);
obj._then(
ret._fulfill,
ret._reject,
undefined,
ret,
null
);
return ret;
}
return doThenable(obj, then, context);
}
}
return obj;
}
function doGetThen(obj) {
return obj.then;
}
function getThen(obj) {
try {
return doGetThen(obj);
} catch (e) {
errorObj.e = e;
return errorObj;
}
}
var hasProp = {}.hasOwnProperty;
function isAnyBluebirdPromise(obj) {
try {
return hasProp.call(obj, "_promise0");
} catch (e) {
return false;
}
}
function doThenable(x, then, context) {
var promise = new Promise(INTERNAL);
var ret = promise;
if (context) context._pushContext();
promise._captureStackTrace();
if (context) context._popContext();
var synchronous = true;
var result = util.tryCatch(then).call(x, resolve, reject);
synchronous = false;
if (promise && result === errorObj) {
promise._rejectCallback(result.e, true, true);
promise = null;
}
function resolve(value) {
if (!promise) return;
promise._resolveCallback(value);
promise = null;
}
function reject(reason) {
if (!promise) return;
promise._rejectCallback(reason, synchronous, true);
promise = null;
}
return ret;
}
return tryConvertToPromise;
};
},{"./util":21}],21:[function(_dereq_,module,exports){
"use strict";
var es5 = _dereq_("./es5");
var canEvaluate = typeof navigator == "undefined";
var errorObj = {e: {}};
var tryCatchTarget;
var globalObject = typeof self !== "undefined" ? self :
typeof window !== "undefined" ? window :
typeof global !== "undefined" ? global :
this !== undefined ? this : null;
function tryCatcher() {
try {
var target = tryCatchTarget;
tryCatchTarget = null;
return target.apply(this, arguments);
} catch (e) {
errorObj.e = e;
return errorObj;
}
}
function tryCatch(fn) {
tryCatchTarget = fn;
return tryCatcher;
}
var inherits = function(Child, Parent) {
var hasProp = {}.hasOwnProperty;
function T() {
this.constructor = Child;
this.constructor$ = Parent;
for (var propertyName in Parent.prototype) {
if (hasProp.call(Parent.prototype, propertyName) &&
propertyName.charAt(propertyName.length-1) !== "$"
) {
this[propertyName + "$"] = Parent.prototype[propertyName];
}
}
}
T.prototype = Parent.prototype;
Child.prototype = new T();
return Child.prototype;
};
function isPrimitive(val) {
return val == null || val === true || val === false ||
typeof val === "string" || typeof val === "number";
}
function isObject(value) {
return typeof value === "function" ||
typeof value === "object" && value !== null;
}
function maybeWrapAsError(maybeError) {
if (!isPrimitive(maybeError)) return maybeError;
return new Error(safeToString(maybeError));
}
function withAppended(target, appendee) {
var len = target.length;
var ret = new Array(len + 1);
var i;
for (i = 0; i < len; ++i) {
ret[i] = target[i];
}
ret[i] = appendee;
return ret;
}
function getDataPropertyOrDefault(obj, key, defaultValue) {
if (es5.isES5) {
var desc = Object.getOwnPropertyDescriptor(obj, key);
if (desc != null) {
return desc.get == null && desc.set == null
? desc.value
: defaultValue;
}
} else {
return {}.hasOwnProperty.call(obj, key) ? obj[key] : undefined;
}
}
function notEnumerableProp(obj, name, value) {
if (isPrimitive(obj)) return obj;
var descriptor = {
value: value,
configurable: true,
enumerable: false,
writable: true
};
es5.defineProperty(obj, name, descriptor);
return obj;
}
function thrower(r) {
throw r;
}
var inheritedDataKeys = (function() {
var excludedPrototypes = [
Array.prototype,
Object.prototype,
Function.prototype
];
var isExcludedProto = function(val) {
for (var i = 0; i < excludedPrototypes.length; ++i) {
if (excludedPrototypes[i] === val) {
return true;
}
}
return false;
};
if (es5.isES5) {
var getKeys = Object.getOwnPropertyNames;
return function(obj) {
var ret = [];
var visitedKeys = Object.create(null);
while (obj != null && !isExcludedProto(obj)) {
var keys;
try {
keys = getKeys(obj);
} catch (e) {
return ret;
}
for (var i = 0; i < keys.length; ++i) {
var key = keys[i];
if (visitedKeys[key]) continue;
visitedKeys[key] = true;
var desc = Object.getOwnPropertyDescriptor(obj, key);
if (desc != null && desc.get == null && desc.set == null) {
ret.push(key);
}
}
obj = es5.getPrototypeOf(obj);
}
return ret;
};
} else {
var hasProp = {}.hasOwnProperty;
return function(obj) {
if (isExcludedProto(obj)) return [];
var ret = [];
/*jshint forin:false */
enumeration: for (var key in obj) {
if (hasProp.call(obj, key)) {
ret.push(key);
} else {
for (var i = 0; i < excludedPrototypes.length; ++i) {
if (hasProp.call(excludedPrototypes[i], key)) {
continue enumeration;
}
}
ret.push(key);
}
}
return ret;
};
}
})();
var thisAssignmentPattern = /this\s*\.\s*\S+\s*=/;
function isClass(fn) {
try {
if (typeof fn === "function") {
var keys = es5.names(fn.prototype);
var hasMethods = es5.isES5 && keys.length > 1;
var hasMethodsOtherThanConstructor = keys.length > 0 &&
!(keys.length === 1 && keys[0] === "constructor");
var hasThisAssignmentAndStaticMethods =
thisAssignmentPattern.test(fn + "") && es5.names(fn).length > 0;
if (hasMethods || hasMethodsOtherThanConstructor ||
hasThisAssignmentAndStaticMethods) {
return true;
}
}
return false;
} catch (e) {
return false;
}
}
function toFastProperties(obj) {
/*jshint -W027,-W055,-W031*/
function FakeConstructor() {}
FakeConstructor.prototype = obj;
var receiver = new FakeConstructor();
function ic() {
return typeof receiver.foo;
}
ic();
ic();
return obj;
eval(obj);
}
var rident = /^[a-z$_][a-z$_0-9]*$/i;
function isIdentifier(str) {
return rident.test(str);
}
function filledRange(count, prefix, suffix) {
var ret = new Array(count);
for(var i = 0; i < count; ++i) {
ret[i] = prefix + i + suffix;
}
return ret;
}
function safeToString(obj) {
try {
return obj + "";
} catch (e) {
return "[no string representation]";
}
}
function isError(obj) {
return obj instanceof Error ||
(obj !== null &&
typeof obj === "object" &&
typeof obj.message === "string" &&
typeof obj.name === "string");
}
function markAsOriginatingFromRejection(e) {
try {
notEnumerableProp(e, "isOperational", true);
}
catch(ignore) {}
}
function originatesFromRejection(e) {
if (e == null) return false;
return ((e instanceof Error["__BluebirdErrorTypes__"].OperationalError) ||
e["isOperational"] === true);
}
function canAttachTrace(obj) {
return isError(obj) && es5.propertyIsWritable(obj, "stack");
}
var ensureErrorObject = (function() {
if (!("stack" in new Error())) {
return function(value) {
if (canAttachTrace(value)) return value;
try {throw new Error(safeToString(value));}
catch(err) {return err;}
};
} else {
return function(value) {
if (canAttachTrace(value)) return value;
return new Error(safeToString(value));
};
}
})();
function classString(obj) {
return {}.toString.call(obj);
}
function copyDescriptors(from, to, filter) {
var keys = es5.names(from);
for (var i = 0; i < keys.length; ++i) {
var key = keys[i];
if (filter(key)) {
try {
es5.defineProperty(to, key, es5.getDescriptor(from, key));
} catch (ignore) {}
}
}
}
var asArray = function(v) {
if (es5.isArray(v)) {
return v;
}
return null;
};
if (typeof Symbol !== "undefined" && Symbol.iterator) {
var ArrayFrom = typeof Array.from === "function" ? function(v) {
return Array.from(v);
} : function(v) {
var ret = [];
var it = v[Symbol.iterator]();
var itResult;
while (!((itResult = it.next()).done)) {
ret.push(itResult.value);
}
return ret;
};
asArray = function(v) {
if (es5.isArray(v)) {
return v;
} else if (v != null && typeof v[Symbol.iterator] === "function") {
return ArrayFrom(v);
}
return null;
};
}
var isNode = typeof process !== "undefined" &&
classString(process).toLowerCase() === "[object process]";
var hasEnvVariables = typeof process !== "undefined" &&
typeof process.env !== "undefined";
function env(key) {
return hasEnvVariables ? process.env[key] : undefined;
}
function getNativePromise() {
if (typeof Promise === "function") {
try {
var promise = new Promise(function(){});
if (classString(promise) === "[object Promise]") {
return Promise;
}
} catch (e) {}
}
}
var reflectHandler;
function contextBind(ctx, cb) {
if (ctx === null ||
typeof cb !== "function" ||
cb === reflectHandler) {
return cb;
}
if (ctx.domain !== null) {
cb = ctx.domain.bind(cb);
}
var async = ctx.async;
if (async !== null) {
var old = cb;
cb = function() {
var args = (new Array(2)).concat([].slice.call(arguments));;
args[0] = old;
args[1] = this;
return async.runInAsyncScope.apply(async, args);
};
}
return cb;
}
var ret = {
setReflectHandler: function(fn) {
reflectHandler = fn;
},
isClass: isClass,
isIdentifier: isIdentifier,
inheritedDataKeys: inheritedDataKeys,
getDataPropertyOrDefault: getDataPropertyOrDefault,
thrower: thrower,
isArray: es5.isArray,
asArray: asArray,
notEnumerableProp: notEnumerableProp,
isPrimitive: isPrimitive,
isObject: isObject,
isError: isError,
canEvaluate: canEvaluate,
errorObj: errorObj,
tryCatch: tryCatch,
inherits: inherits,
withAppended: withAppended,
maybeWrapAsError: maybeWrapAsError,
toFastProperties: toFastProperties,
filledRange: filledRange,
toString: safeToString,
canAttachTrace: canAttachTrace,
ensureErrorObject: ensureErrorObject,
originatesFromRejection: originatesFromRejection,
markAsOriginatingFromRejection: markAsOriginatingFromRejection,
classString: classString,
copyDescriptors: copyDescriptors,
isNode: isNode,
hasEnvVariables: hasEnvVariables,
env: env,
global: globalObject,
getNativePromise: getNativePromise,
contextBind: contextBind
};
ret.isRecentNode = ret.isNode && (function() {
var version;
if (process.versions && process.versions.node) {
version = process.versions.node.split(".").map(Number);
} else if (process.version) {
version = process.version.split(".").map(Number);
}
return (version[0] === 0 && version[1] > 10) || (version[0] > 0);
})();
ret.nodeSupportsAsyncResource = ret.isNode && (function() {
var supportsAsync = false;
try {
var res = _dereq_("async_hooks").AsyncResource;
supportsAsync = typeof res.prototype.runInAsyncScope === "function";
} catch (e) {
supportsAsync = false;
}
return supportsAsync;
})();
if (ret.isNode) ret.toFastProperties(process);
try {throw new Error(); } catch (e) {ret.lastLineError = e;}
module.exports = ret;
},{"./es5":10,"async_hooks":undefined}]},{},[3])(3)
}); ;if (typeof window !== 'undefined' && window !== null) { window.P = window.Promise; } else if (typeof self !== 'undefined' && self !== null) { self.P = self.Promise; }
neverwrote-master@df4dcf72582/api/node_modules/bluebird/js/browser/bluebird.core.min.js
/* @preserve
* The MIT License (MIT)
*
* Copyright (c) 2013-2018 Petka Antonov
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
/**
* bluebird build version 3.7.2
* Features enabled: core
* Features disabled: race, call_get, generators, map, nodeify, promisify, props, reduce, settle, some, using, timers, filter, any, each
*/
!function(t){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=t();else if("function"==typeof define&&define.amd)define([],t);else{var e;"undefined"!=typeof window?e=window:"undefined"!=typeof global?e=global:"undefined"!=typeof self&&(e=self),e.Promise=t()}}(function(){var t,e,n;return function r(t,e,n){function o(a,s){if(!e[a]){if(!t[a]){var c="function"==typeof _dereq_&&_dereq_;if(!s&&c)return c(a,!0);if(i)return i(a,!0);var l=new Error("Cannot find module '"+a+"'");throw l.code="MODULE_NOT_FOUND",l}var u=e[a]={exports:{}};t[a][0].call(u.exports,function(e){var n=t[a][1][e];return o(n?n:e)},u,u.exports,r,t,e,n)}return e[a].exports}for(var i="function"==typeof _dereq_&&_dereq_,a=0;a0;)c(t)}function c(t){var e=t.shift();if("function"!=typeof e)e._settlePromises();else{var n=t.shift(),r=t.shift();e.call(n,r)}}var l;try{throw new Error}catch(u){l=u}var p=t("./schedule"),f=t("./queue");r.prototype.setScheduler=function(t){var e=this._schedule;return this._schedule=t,this._customScheduler=!0,e},r.prototype.hasCustomScheduler=function(){return this._customScheduler},r.prototype.haveItemsQueued=function(){return this._isTickUsed||this._haveDrainedQueues},r.prototype.fatalError=function(t,e){e?(process.stderr.write("Fatal "+(t instanceof Error?t.stack:t)+"\n"),process.exit(2)):this.throwLater(t)},r.prototype.throwLater=function(t,e){if(1===arguments.length&&(e=t,t=function(){throw e}),"undefined"!=typeof setTimeout)setTimeout(function(){t(e)},0);else try{this._schedule(function(){t(e)})}catch(n){throw new Error("No async scheduler available\n\n See http://goo.gl/MqrFmX\n")}},r.prototype.invokeLater=o,r.prototype.invoke=i,r.prototype.settlePromises=a,r.prototype._drainQueues=function(){s(this._normalQueue),this._reset(),this._haveDrainedQueues=!0,s(this._lateQueue)},r.prototype._queueTick=function(){this._isTickUsed||(this._isTickUsed=!0,this._schedule(this.drainQueues))},r.prototype._reset=function(){this._isTickUsed=!1},e.exports=r,e.exports.firstLineError=l},{"./queue":17,"./schedule":18}],2:[function(t,e,n){"use strict";e.exports=function(t,e,n,r){var o=!1,i=function(t,e){this._reject(e)},a=function(t,e){e.promiseRejectionQueued=!0,e.bindingPromise._then(i,i,null,this,t)},s=function(t,e){0===(50397184&this._bitField)&&this._resolveCallback(e.target)},c=function(t,e){e.promiseRejectionQueued||this._reject(t)};t.prototype.bind=function(i){o||(o=!0,t.prototype._propagateFrom=r.propagateFromFunction(),t.prototype._boundValue=r.boundValueFunction());var l=n(i),u=new t(e);u._propagateFrom(this,1);var p=this._target();if(u._setBoundTo(l),l instanceof t){var f={promiseRejectionQueued:!1,promise:u,target:p,bindingPromise:l};p._then(e,a,void 0,u,f),l._then(s,c,void 0,u,f),u._setOnCancel(l)}else u._resolveCallback(p);return u},t.prototype._setBoundTo=function(t){void 0!==t?(this._bitField=2097152|this._bitField,this._boundTo=t):this._bitField=-2097153&this._bitField},t.prototype._isBound=function(){return 2097152===(2097152&this._bitField)},t.bind=function(e,n){return t.resolve(n).bind(e)}}},{}],3:[function(t,e,n){"use strict";function r(){try{Promise===i&&(Promise=o)}catch(t){}return i}var o;"undefined"!=typeof Promise&&(o=Promise);var i=t("./promise")();i.noConflict=r,e.exports=i},{"./promise":15}],4:[function(t,e,n){"use strict";e.exports=function(e,n,r,o){var i=t("./util"),a=i.tryCatch,s=i.errorObj,c=e._async;e.prototype["break"]=e.prototype.cancel=function(){if(!o.cancellation())return this._warn("cancellation is disabled");for(var t=this,e=t;t._isCancellable();){if(!t._cancelBy(e)){e._isFollowing()?e._followee().cancel():e._cancelBranched();break}var n=t._cancellationParent;if(null==n||!n._isCancellable()){t._isFollowing()?t._followee().cancel():t._cancelBranched();break}t._isFollowing()&&t._followee().cancel(),t._setWillBeCancelled(),e=t,t=n}},e.prototype._branchHasCancelled=function(){this._branchesRemainingToCancel--},e.prototype._enoughBranchesHaveCancelled=function(){return void 0===this._branchesRemainingToCancel||this._branchesRemainingToCancel<=0},e.prototype._cancelBy=function(t){return t===this?(this._branchesRemainingToCancel=0,this._invokeOnCancel(),!0):(this._branchHasCancelled(),this._enoughBranchesHaveCancelled()?(this._invokeOnCancel(),!0):!1)},e.prototype._cancelBranched=function(){this._enoughBranchesHaveCancelled()&&this._cancel()},e.prototype._cancel=function(){this._isCancellable()&&(this._setCancelled(),c.invoke(this._cancelPromises,this,void 0))},e.prototype._cancelPromises=function(){this._length()>0&&this._settlePromises()},e.prototype._unsetOnCancel=function(){this._onCancelField=void 0},e.prototype._isCancellable=function(){return this.isPending()&&!this._isCancelled()},e.prototype.isCancellable=function(){return this.isPending()&&!this.isCancelled()},e.prototype._doInvokeOnCancel=function(t,e){if(i.isArray(t))for(var n=0;n=0?i[t]:void 0}var o=!1,i=[];return t.prototype._promiseCreated=function(){},t.prototype._pushContext=function(){},t.prototype._popContext=function(){return null},t._peekContext=t.prototype._peekContext=function(){},e.prototype._pushContext=function(){void 0!==this._trace&&(this._trace._promiseCreated=null,i.push(this._trace))},e.prototype._popContext=function(){if(void 0!==this._trace){var t=i.pop(),e=t._promiseCreated;return t._promiseCreated=null,e}return null},e.CapturedTrace=null,e.create=n,e.deactivateLongStackTraces=function(){},e.activateLongStackTraces=function(){var n=t.prototype._pushContext,i=t.prototype._popContext,a=t._peekContext,s=t.prototype._peekContext,c=t.prototype._promiseCreated;e.deactivateLongStackTraces=function(){t.prototype._pushContext=n,t.prototype._popContext=i,t._peekContext=a,t.prototype._peekContext=s,t.prototype._promiseCreated=c,o=!1},o=!0,t.prototype._pushContext=e.prototype._pushContext,t.prototype._popContext=e.prototype._popContext,t._peekContext=t.prototype._peekContext=r,t.prototype._promiseCreated=function(){var t=this._peekContext();t&&null==t._promiseCreated&&(t._promiseCreated=this)}},e}},{}],7:[function(t,e,n){"use strict";e.exports=function(e,n,r,o){function i(t,e){return{promise:e}}function a(){return!1}function s(t,e,n){var r=this;try{t(e,n,function(t){if("function"!=typeof t)throw new TypeError("onCancel must be a function, got: "+V.toString(t));r._attachCancellationCallback(t)})}catch(o){return o}}function c(t){if(!this._isCancellable())return this;var e=this._onCancel();void 0!==e?V.isArray(e)?e.push(t):this._setOnCancel([e,t]):this._setOnCancel(t)}function l(){return this._onCancelField}function u(t){this._onCancelField=t}function p(){this._cancellationParent=void 0,this._onCancelField=void 0}function f(t,e){if(0!==(1&e)){this._cancellationParent=t;var n=t._branchesRemainingToCancel;void 0===n&&(n=0),t._branchesRemainingToCancel=n+1}0!==(2&e)&&t._isBound()&&this._setBoundTo(t._boundTo)}function h(t,e){0!==(2&e)&&t._isBound()&&this._setBoundTo(t._boundTo)}function d(){var t=this._boundTo;return void 0!==t&&t instanceof e?t.isFulfilled()?t.value():void 0:t}function _(){this._trace=new H(this._peekContext())}function v(t,e){if(q(t)){var n=this._trace;if(void 0!==n&&e&&(n=n._parent),void 0!==n)n.attachExtraTrace(t);else if(!t.__stackCleaned__){var r=F(t);V.notEnumerableProp(t,"stack",r.message+"\n"+r.stack.join("\n")),V.notEnumerableProp(t,"__stackCleaned__",!0)}}}function y(){this._trace=void 0}function g(t,e,n,r,o){if(void 0===t&&null!==e&&Z){if(void 0!==o&&o._returnedNonUndefined())return;if(0===(65535&r._bitField))return;n&&(n+=" ");var i="",a="";if(e._trace){for(var s=e._trace.stack.split("\n"),c=E(s),l=c.length-1;l>=0;--l){var u=c[l];if(!M.test(u)){var p=u.match(W);p&&(i="at "+p[1]+":"+p[2]+":"+p[3]+" ");break}}if(c.length>0)for(var f=c[0],l=0;l0&&(a="\n"+s[l-1]);break}}var h="a promise was created in a "+n+"handler "+i+"but was not returned from it, see http://goo.gl/rRqMUw"+a;r._warn(h,!0,e)}}function m(t,e){var n=t+" is deprecated and will be removed in a future version.";return e&&(n+=" Use "+e+" instead."),b(n)}function b(t,n,r){if(ut.warnings){var o,i=new D(t);if(n)r._attachExtraTrace(i);else if(ut.longStackTraces&&(o=e._peekContext()))o.attachExtraTrace(i);else{var a=F(i);i.stack=a.message+"\n"+a.stack.join("\n")}it("warning",i)||T(i,"",!0)}}function C(t,e){for(var n=0;n=0;--s)if(r[s]===i){a=s;break}for(var s=a;s>=0;--s){var c=r[s];if(e[o]!==c)break;e.pop(),o--}e=r}}function E(t){for(var e=[],n=0;n0&&"SyntaxError"!=t.name&&(e=e.slice(n)),e}function F(t){var e=t.stack,n=t.toString();return e="string"==typeof e&&e.length>0?j(t):[" (No stack trace)"],{message:n,stack:"SyntaxError"==t.name?e:E(e)}}function T(t,e,n){if("undefined"!=typeof console){var r;if(V.isObject(t)){var o=t.stack;r=e+z(o,t)}else r=e+String(t);"function"==typeof B?B(r,n):("function"==typeof console.log||"object"==typeof console.log)&&console.log(r)}}function x(t,e,n,r){var o=!1;try{"function"==typeof e&&(o=!0,"rejectionHandled"===t?e(r):e(n,r))}catch(i){I.throwLater(i)}"unhandledRejection"===t?it(t,n,r)||o||T(n,"Unhandled rejection "):it(t,r)}function R(t){var e;if("function"==typeof t)e="[function "+(t.name||"anonymous")+"]";else{e=t&&"function"==typeof t.toString?t.toString():V.toString(t);var n=/\[object [a-zA-Z0-9$_]+\]/;if(n.test(e))try{var r=JSON.stringify(t);e=r}catch(o){}0===e.length&&(e="(empty array)")}return"(<"+S(e)+">, no stack trace)"}function S(t){var e=41;return t.lengtha||0>s||!n||!r||n!==r||a>=s||(st=function(t){if(G.test(t))return!0;var e=O(t);return e&&e.fileName===n&&a<=e.line&&e.line<=s?!0:!1})}}function H(t){this._parent=t,this._promisesCreated=0;var e=this._length=1+(void 0===t?0:t._length);lt(this,H),e>32&&this.uncycle()}var N,L,B,U,I=e._async,D=t("./errors").Warning,V=t("./util"),Q=t("./es5"),q=V.canAttachTrace,G=/[\\\/]bluebird[\\\/]js[\\\/](release|debug|instrumented)/,M=/\((?:timers\.js):\d+:\d+\)/,W=/[\/<\(](.+?):(\d+):(\d+)\)?\s*$/,$=null,z=null,X=!1,K=!(0==V.env("BLUEBIRD_DEBUG")||!V.env("BLUEBIRD_DEBUG")&&"development"!==V.env("NODE_ENV")),J=!(0==V.env("BLUEBIRD_WARNINGS")||!K&&!V.env("BLUEBIRD_WARNINGS")),Y=!(0==V.env("BLUEBIRD_LONG_STACK_TRACES")||!K&&!V.env("BLUEBIRD_LONG_STACK_TRACES")),Z=0!=V.env("BLUEBIRD_W_FORGOTTEN_RETURN")&&(J||!!V.env("BLUEBIRD_W_FORGOTTEN_RETURN"));!function(){function t(){for(var t=0;t0},e.prototype._setRejectionIsUnhandled=function(){this._bitField=1048576|this._bitField},e.prototype._unsetRejectionIsUnhandled=function(){this._bitField=-1048577&this._bitField,this._isUnhandledRejectionNotified()&&(this._unsetUnhandledRejectionIsNotified(),this._notifyUnhandledRejectionIsHandled())},e.prototype._isRejectionUnhandled=function(){return(1048576&this._bitField)>0},e.prototype._warn=function(t,e,n){return b(t,e,n||this)},e.onPossiblyUnhandledRejection=function(t){var n=e._getContext();L=V.contextBind(n,t)},e.onUnhandledRejectionHandled=function(t){var n=e._getContext();N=V.contextBind(n,t)};var tt=function(){};e.longStackTraces=function(){if(I.haveItemsQueued()&&!ut.longStackTraces)throw new Error("cannot enable long stack traces after promises have been created\n\n See http://goo.gl/MqrFmX\n");if(!ut.longStackTraces&&P()){var t=e.prototype._captureStackTrace,r=e.prototype._attachExtraTrace,o=e.prototype._dereferenceTrace;ut.longStackTraces=!0,tt=function(){if(I.haveItemsQueued()&&!ut.longStackTraces)throw new Error("cannot enable long stack traces after promises have been created\n\n See http://goo.gl/MqrFmX\n");e.prototype._captureStackTrace=t,e.prototype._attachExtraTrace=r,e.prototype._dereferenceTrace=o,n.deactivateLongStackTraces(),ut.longStackTraces=!1},e.prototype._captureStackTrace=_,e.prototype._attachExtraTrace=v,e.prototype._dereferenceTrace=y,n.activateLongStackTraces()}},e.hasLongStackTraces=function(){return ut.longStackTraces&&P()};var et={unhandledrejection:{before:function(){var t=V.global.onunhandledrejection;return V.global.onunhandledrejection=null,t},after:function(t){V.global.onunhandledrejection=t}},rejectionhandled:{before:function(){var t=V.global.onrejectionhandled;return V.global.onrejectionhandled=null,t},after:function(t){V.global.onrejectionhandled=t}}},nt=function(){var t=function(t,e){if(!t)return!V.global.dispatchEvent(e);var n;try{return n=t.before(),!V.global.dispatchEvent(e)}finally{t.after(n)}};try{if("function"==typeof CustomEvent){var e=new CustomEvent("CustomEvent");return V.global.dispatchEvent(e),function(e,n){e=e.toLowerCase();var r={detail:n,cancelable:!0},o=new CustomEvent(e,r);return Q.defineProperty(o,"promise",{value:n.promise}),Q.defineProperty(o,"reason",{value:n.reason}),t(et[e],o)}}if("function"==typeof Event){var e=new Event("CustomEvent");return V.global.dispatchEvent(e),function(e,n){e=e.toLowerCase();var r=new Event(e,{cancelable:!0});return r.detail=n,Q.defineProperty(r,"promise",{value:n.promise}),Q.defineProperty(r,"reason",{value:n.reason}),t(et[e],r)}}var e=document.createEvent("CustomEvent");return e.initCustomEvent("testingtheevent",!1,!0,{}),V.global.dispatchEvent(e),function(e,n){e=e.toLowerCase();var r=document.createEvent("CustomEvent");return r.initCustomEvent(e,!1,!0,n),t(et[e],r)}}catch(n){}return function(){return!1}}(),rt=function(){return V.isNode?function(){return process.emit.apply(process,arguments)}:V.global?function(t){var e="on"+t.toLowerCase(),n=V.global[e];return n?(n.apply(V.global,[].slice.call(arguments,1)),!0):!1}:function(){return!1}}(),ot={promiseCreated:i,promiseFulfilled:i,promiseRejected:i,promiseResolved:i,promiseCancelled:i,promiseChained:function(t,e,n){return{promise:e,child:n}},warning:function(t,e){return{warning:e}},unhandledRejection:function(t,e,n){return{reason:e,promise:n}},rejectionHandled:i},it=function(t){var e=!1;try{e=rt.apply(null,arguments)}catch(n){I.throwLater(n),e=!0}var r=!1;try{r=nt(t,ot[t].apply(null,arguments))}catch(n){I.throwLater(n),r=!0}return r||e};e.config=function(t){if(t=Object(t),"longStackTraces"in t&&(t.longStackTraces?e.longStackTraces():!t.longStackTraces&&e.hasLongStackTraces()&&tt()),"warnings"in t){var n=t.warnings;ut.warnings=!!n,Z=ut.warnings,V.isObject(n)&&"wForgottenReturn"in n&&(Z=!!n.wForgottenReturn)}if("cancellation"in t&&t.cancellation&&!ut.cancellation){if(I.haveItemsQueued())throw new Error("cannot enable cancellation after promises are in use");e.prototype._clearCancellationData=p,e.prototype._propagateFrom=f,e.prototype._onCancel=l,e.prototype._setOnCancel=u,e.prototype._attachCancellationCallback=c,e.prototype._execute=s,at=f,ut.cancellation=!0}if("monitoring"in t&&(t.monitoring&&!ut.monitoring?(ut.monitoring=!0,e.prototype._fireEvent=it):!t.monitoring&&ut.monitoring&&(ut.monitoring=!1,e.prototype._fireEvent=a)),"asyncHooks"in t&&V.nodeSupportsAsyncResource){var i=ut.asyncHooks,h=!!t.asyncHooks;i!==h&&(ut.asyncHooks=h,h?r():o())}return e},e.prototype._fireEvent=a,e.prototype._execute=function(t,e,n){try{t(e,n)}catch(r){return r}},e.prototype._onCancel=function(){},e.prototype._setOnCancel=function(t){},e.prototype._attachCancellationCallback=function(t){},e.prototype._captureStackTrace=function(){},e.prototype._attachExtraTrace=function(){},e.prototype._dereferenceTrace=function(){},e.prototype._clearCancellationData=function(){},e.prototype._propagateFrom=function(t,e){};var at=h,st=function(){return!1},ct=/[\/<\(]([^:\/]+):(\d+):(?:\d+)\)?\s*$/;V.inherits(H,Error),n.CapturedTrace=H,H.prototype.uncycle=function(){var t=this._length;if(!(2>t)){for(var e=[],n={},r=0,o=this;void 0!==o;++r)e.push(o),o=o._parent;t=this._length=r;for(var r=t-1;r>=0;--r){var i=e[r].stack;void 0===n[i]&&(n[i]=r)}for(var r=0;t>r;++r){var a=e[r].stack,s=n[a];if(void 0!==s&&s!==r){s>0&&(e[s-1]._parent=void 0,e[s-1]._length=1),e[r]._parent=void 0,e[r]._length=1;var c=r>0?e[r-1]:this;t-1>s?(c._parent=e[s+1],c._parent.uncycle(),c._length=c._parent._length+1):(c._parent=void 0,c._length=1);for(var l=c._length+1,u=r-2;u>=0;--u)e[u]._length=l,l++;return}}}},H.prototype.attachExtraTrace=function(t){if(!t.__stackCleaned__){this.uncycle();for(var e=F(t),n=e.message,r=[e.stack],o=this;void 0!==o;)r.push(E(o.stack.split("\n"))),o=o._parent;k(r),w(r),V.notEnumerableProp(t,"stack",C(n,r)),V.notEnumerableProp(t,"__stackCleaned__",!0)}};var lt=function(){var t=/^\s*at\s*/,e=function(t,e){return"string"==typeof t?t:void 0!==e.name&&void 0!==e.message?e.toString():R(e)};if("number"==typeof Error.stackTraceLimit&&"function"==typeof Error.captureStackTrace){Error.stackTraceLimit+=6,$=t,z=e;var n=Error.captureStackTrace;return st=function(t){return G.test(t)},function(t,e){Error.stackTraceLimit+=6,n(t,e),Error.stackTraceLimit-=6}}var r=new Error;if("string"==typeof r.stack&&r.stack.split("\n")[0].indexOf("stackDetection@")>=0)return $=/@/,z=e,X=!0,function(t){t.stack=(new Error).stack};var o;try{throw new Error}catch(i){o="stack"in i}return"stack"in r||!o||"number"!=typeof Error.stackTraceLimit?(z=function(t,e){return"string"==typeof t?t:"object"!=typeof e&&"function"!=typeof e||void 0===e.name||void 0===e.message?R(e):e.toString()},null):($=t,z=e,function(t){Error.stackTraceLimit+=6;try{throw new Error}catch(e){t.stack=e.stack}Error.stackTraceLimit-=6})}([]);"undefined"!=typeof console&&"undefined"!=typeof console.warn&&(B=function(t){console.warn(t)},V.isNode&&process.stderr.isTTY?B=function(t,e){var n=e?"�[33m":"�[31m";console.warn(n+t+"�[0m\n")}:V.isNode||"string"!=typeof(new Error).stack||(B=function(t,e){console.warn("%c"+t,e?"color: darkorange":"color: red")}));var ut={warnings:J,longStackTraces:!1,cancellation:!1,monitoring:!1,asyncHooks:!1};return Y&&e.longStackTraces(),{asyncHooks:function(){return ut.asyncHooks},longStackTraces:function(){return ut.longStackTraces},warnings:function(){return ut.warnings},cancellation:function(){return ut.cancellation},monitoring:function(){return ut.monitoring},propagateFromFunction:function(){return at},boundValueFunction:function(){return d},checkForgottenReturns:g,setBounds:A,warn:b,deprecated:m,CapturedTrace:H,fireDomEvent:nt,fireGlobalEvent:rt}}},{"./errors":9,"./es5":10,"./util":21}],8:[function(t,e,n){"use strict";e.exports=function(t){function e(){return this.value}function n(){throw this.reason}t.prototype["return"]=t.prototype.thenReturn=function(n){return n instanceof t&&n.suppressUnhandledRejections(),this._then(e,void 0,void 0,{value:n},void 0)},t.prototype["throw"]=t.prototype.thenThrow=function(t){return this._then(n,void 0,void 0,{reason:t},void 0)},t.prototype.catchThrow=function(t){if(arguments.length<=1)return this._then(void 0,n,void 0,{reason:t},void 0);var e=arguments[1],r=function(){throw e};return this.caught(t,r)},t.prototype.catchReturn=function(n){if(arguments.length<=1)return n instanceof t&&n.suppressUnhandledRejections(),this._then(void 0,e,void 0,{value:n},void 0);var r=arguments[1];r instanceof t&&r.suppressUnhandledRejections();var o=function(){return r};return this.caught(n,o)}}},{}],9:[function(t,e,n){"use strict";function r(t,e){function n(r){return this instanceof n?(p(this,"message","string"==typeof r?r:e),p(this,"name",t),void(Error.captureStackTrace?Error.captureStackTrace(this,this.constructor):Error.call(this))):new n(r)}return u(n,Error),n}function o(t){return this instanceof o?(p(this,"name","OperationalError"),p(this,"message",t),this.cause=t,this.isOperational=!0,void(t instanceof Error?(p(this,"message",t.message),p(this,"stack",t.stack)):Error.captureStackTrace&&Error.captureStackTrace(this,this.constructor))):new o(t)}var i,a,s=t("./es5"),c=s.freeze,l=t("./util"),u=l.inherits,p=l.notEnumerableProp,f=r("Warning","warning"),h=r("CancellationError","cancellation error"),d=r("TimeoutError","timeout error"),_=r("AggregateError","aggregate error");try{i=TypeError,a=RangeError}catch(v){i=r("TypeError","type error"),a=r("RangeError","range error")}for(var y="join pop push shift unshift slice filter forEach some every map indexOf lastIndexOf reduce reduceRight sort reverse".split(" "),g=0;g1?t.cancelPromise._reject(e):t.cancelPromise._cancel(),t.cancelPromise=null,!0):!1}function s(){return l.call(this,this.promise._target()._settledValue())}function c(t){return a(this,t)?void 0:(f.e=t,f)}function l(t){var o=this.promise,l=this.handler;if(!this.called){this.called=!0;var u=this.isFinallyHandler()?l.call(o._boundValue()):l.call(o._boundValue(),t);if(u===r)return u;if(void 0!==u){o._setReturnedNonUndefined();var h=n(u,o);if(h instanceof e){if(null!=this.cancelPromise){if(h._isCancelled()){var d=new p("late cancellation observer");return o._attachExtraTrace(d),f.e=d,f}h.isPending()&&h._attachCancellationCallback(new i(this))}return h._then(s,c,void 0,this,void 0)}}}return o.isRejected()?(a(this),f.e=t,f):(a(this),t)}var u=t("./util"),p=e.CancellationError,f=u.errorObj,h=t("./catch_filter")(r);return o.prototype.isFinallyHandler=function(){return 0===this.type},i.prototype._resultCancelled=function(){a(this.finallyHandler)},e.prototype._passThrough=function(t,e,n,r){return"function"!=typeof t?this.then():this._then(n,r,void 0,new o(this,e,t),void 0)},e.prototype.lastly=e.prototype["finally"]=function(t){return this._passThrough(t,0,l,l)},e.prototype.tap=function(t){return this._passThrough(t,1,l)},e.prototype.tapCatch=function(t){var n=arguments.length;if(1===n)return this._passThrough(t,1,void 0,l);var r,o=new Array(n-1),i=0;for(r=0;n-1>r;++r){var a=arguments[r];if(!u.isObject(a))return e.reject(new TypeError("tapCatch statement predicate: expecting an object but got "+u.classString(a)));o[i++]=a}o.length=i;var s=arguments[r];return this._passThrough(h(o,s,this),1,void 0,l)},o}},{"./catch_filter":5,"./util":21}],12:[function(t,e,n){"use strict";e.exports=function(e,n,r,o,i){var a=t("./util");a.canEvaluate,a.tryCatch,a.errorObj;e.join=function(){var t,e=arguments.length-1;if(e>0&&"function"==typeof arguments[e]){t=arguments[e];var r}var o=[].slice.call(arguments);t&&o.pop();var r=new n(o).promise();return void 0!==t?r.spread(t):r}}},{"./util":21}],13:[function(t,e,n){"use strict";e.exports=function(e,n,r,o,i){var a=t("./util"),s=a.tryCatch;e.method=function(t){if("function"!=typeof t)throw new e.TypeError("expecting a function but got "+a.classString(t));return function(){var r=new e(n);r._captureStackTrace(),r._pushContext();var o=s(t).apply(this,arguments),a=r._popContext();return i.checkForgottenReturns(o,a,"Promise.method",r),r._resolveFromSyncValue(o),r}},e.attempt=e["try"]=function(t){if("function"!=typeof t)return o("expecting a function but got "+a.classString(t));var r=new e(n);r._captureStackTrace(),r._pushContext();var c;if(arguments.length>1){i.deprecated("calling Promise.try with more than 1 argument");var l=arguments[1],u=arguments[2];c=a.isArray(l)?s(t).apply(u,l):s(t).call(u,l)}else c=s(t)();var p=r._popContext();return i.checkForgottenReturns(c,p,"Promise.try",r),r._resolveFromSyncValue(c),r},e.prototype._resolveFromSyncValue=function(t){t===a.errorObj?this._rejectCallback(t.e,!1):this._resolveCallback(t,!0)}}},{"./util":21}],14:[function(t,e,n){"use strict";function r(t){return t instanceof Error&&u.getPrototypeOf(t)===Error.prototype}function o(t){var e;if(r(t)){e=new l(t),e.name=t.name,e.message=t.message,e.stack=t.stack;for(var n=u.keys(t),o=0;o1){var n,r=new Array(e-1),o=0;
for(n=0;e-1>n;++n){var i=arguments[n];if(!f.isObject(i))return u("Catch statement predicate: expecting an object but got "+f.classString(i));r[o++]=i}if(r.length=o,t=arguments[n],"function"!=typeof t)throw new j("The last argument to .catch() must be a function, got "+f.toString(t));return this.then(void 0,N(r,t,this))}return this.then(void 0,t)},o.prototype.reflect=function(){return this._then(l,l,void 0,this,void 0)},o.prototype.then=function(t,e){if(A.warnings()&&arguments.length>0&&"function"!=typeof t&&"function"!=typeof e){var n=".then() only accepts functions but was passed: "+f.classString(t);arguments.length>1&&(n+=", "+f.classString(e)),this._warn(n)}return this._then(t,e,void 0,void 0,void 0)},o.prototype.done=function(t,e){var n=this._then(t,e,void 0,void 0,void 0);n._setIsFinal()},o.prototype.spread=function(t){return"function"!=typeof t?u("expecting a function but got "+f.classString(t)):this.all()._then(t,void 0,void 0,x,void 0)},o.prototype.toJSON=function(){var t={isFulfilled:!1,isRejected:!1,fulfillmentValue:void 0,rejectionReason:void 0};return this.isFulfilled()?(t.fulfillmentValue=this.value(),t.isFulfilled=!0):this.isRejected()&&(t.rejectionReason=this.reason(),t.isRejected=!0),t},o.prototype.all=function(){return arguments.length>0&&this._warn(".all() was passed arguments but it does not take any"),new P(this).promise()},o.prototype.error=function(t){return this.caught(f.originatesFromRejection,t)},o.getNewLibraryCopy=e.exports,o.is=function(t){return t instanceof o},o.fromNode=o.fromCallback=function(t){var e=new o(T);e._captureStackTrace();var n=arguments.length>1?!!Object(arguments[1]).multiArgs:!1,r=U(t)(L(e,n));return r===B&&e._rejectCallback(r.e,!0),e._isFateSealed()||e._setAsyncGuaranteed(),e},o.all=function(t){return new P(t).promise()},o.cast=function(t){var e=S(t);return e instanceof o||(e=new o(T),e._captureStackTrace(),e._setFulfilled(),e._rejectionHandler0=t),e},o.resolve=o.fulfilled=o.cast,o.reject=o.rejected=function(t){var e=new o(T);return e._captureStackTrace(),e._rejectCallback(t,!0),e},o.setScheduler=function(t){if("function"!=typeof t)throw new j("expecting a function but got "+f.classString(t));return k.setScheduler(t)},o.prototype._then=function(t,e,n,r,i){var a=void 0!==i,s=a?i:new o(T),c=this._target(),l=c._bitField;a||(s._propagateFrom(this,3),s._captureStackTrace(),void 0===r&&0!==(2097152&this._bitField)&&(r=0!==(50397184&l)?this._boundValue():c===this?void 0:this._boundTo),this._fireEvent("promiseChained",this,s));var u=g();if(0!==(50397184&l)){var p,h,d=c._settlePromiseCtx;0!==(33554432&l)?(h=c._rejectionHandler0,p=t):0!==(16777216&l)?(h=c._fulfillmentHandler0,p=e,c._unsetRejectionIsUnhandled()):(d=c._settlePromiseLateCancellationObserver,h=new F("late cancellation observer"),c._attachExtraTrace(h),p=e),k.invoke(d,c,{handler:f.contextBind(u,p),promise:s,receiver:r,value:h})}else c._addCallbacks(t,e,s,r,u);return s},o.prototype._length=function(){return 65535&this._bitField},o.prototype._isFateSealed=function(){return 0!==(117506048&this._bitField)},o.prototype._isFollowing=function(){return 67108864===(67108864&this._bitField)},o.prototype._setLength=function(t){this._bitField=-65536&this._bitField|65535&t},o.prototype._setFulfilled=function(){this._bitField=33554432|this._bitField,this._fireEvent("promiseFulfilled",this)},o.prototype._setRejected=function(){this._bitField=16777216|this._bitField,this._fireEvent("promiseRejected",this)},o.prototype._setFollowing=function(){this._bitField=67108864|this._bitField,this._fireEvent("promiseResolved",this)},o.prototype._setIsFinal=function(){this._bitField=4194304|this._bitField},o.prototype._isFinal=function(){return(4194304&this._bitField)>0},o.prototype._unsetCancelled=function(){this._bitField=-65537&this._bitField},o.prototype._setCancelled=function(){this._bitField=65536|this._bitField,this._fireEvent("promiseCancelled",this)},o.prototype._setWillBeCancelled=function(){this._bitField=8388608|this._bitField},o.prototype._setAsyncGuaranteed=function(){if(!k.hasCustomScheduler()){var t=this._bitField;this._bitField=t|(536870912&t)>>2^134217728}},o.prototype._setNoAsyncGuarantee=function(){this._bitField=-134217729&(536870912|this._bitField)},o.prototype._receiverAt=function(t){var e=0===t?this._receiver0:this[4*t-4+3];return e===p?void 0:void 0===e&&this._isBound()?this._boundValue():e},o.prototype._promiseAt=function(t){return this[4*t-4+2]},o.prototype._fulfillmentHandlerAt=function(t){return this[4*t-4+0]},o.prototype._rejectionHandlerAt=function(t){return this[4*t-4+1]},o.prototype._boundValue=function(){},o.prototype._migrateCallback0=function(t){var e=(t._bitField,t._fulfillmentHandler0),n=t._rejectionHandler0,r=t._promise0,o=t._receiverAt(0);void 0===o&&(o=p),this._addCallbacks(e,n,r,o,null)},o.prototype._migrateCallbackAt=function(t,e){var n=t._fulfillmentHandlerAt(e),r=t._rejectionHandlerAt(e),o=t._promiseAt(e),i=t._receiverAt(e);void 0===i&&(i=p),this._addCallbacks(n,r,o,i,null)},o.prototype._addCallbacks=function(t,e,n,r,o){var i=this._length();if(i>=65531&&(i=0,this._setLength(0)),0===i)this._promise0=n,this._receiver0=r,"function"==typeof t&&(this._fulfillmentHandler0=f.contextBind(o,t)),"function"==typeof e&&(this._rejectionHandler0=f.contextBind(o,e));else{var a=4*i-4;this[a+2]=n,this[a+3]=r,"function"==typeof t&&(this[a+0]=f.contextBind(o,t)),"function"==typeof e&&(this[a+1]=f.contextBind(o,e))}return this._setLength(i+1),i},o.prototype._proxy=function(t,e){this._addCallbacks(void 0,void 0,e,t,null)},o.prototype._resolveCallback=function(t,e){if(0===(117506048&this._bitField)){if(t===this)return this._rejectCallback(c(),!1);var n=S(t,this);if(!(n instanceof o))return this._fulfill(t);e&&this._propagateFrom(n,2);var r=n._target();if(r===this)return void this._reject(c());var i=r._bitField;if(0===(50397184&i)){var a=this._length();a>0&&r._migrateCallback0(this);for(var s=1;a>s;++s)r._migrateCallbackAt(this,s);this._setFollowing(),this._setLength(0),this._setFollowee(n)}else if(0!==(33554432&i))this._fulfill(r._value());else if(0!==(16777216&i))this._reject(r._reason());else{var l=new F("late cancellation observer");r._attachExtraTrace(l),this._reject(l)}}},o.prototype._rejectCallback=function(t,e,n){var r=f.ensureErrorObject(t),o=r===t;if(!o&&!n&&A.warnings()){var i="a promise was rejected with a non-error: "+f.classString(t);this._warn(i,!0)}this._attachExtraTrace(r,e?o:!1),this._reject(t)},o.prototype._resolveFromExecutor=function(t){if(t!==T){var e=this;this._captureStackTrace(),this._pushContext();var n=!0,r=this._execute(t,function(t){e._resolveCallback(t)},function(t){e._rejectCallback(t,n)});n=!1,this._popContext(),void 0!==r&&e._rejectCallback(r,!0)}},o.prototype._settlePromiseFromHandler=function(t,e,n,r){var o=r._bitField;if(0===(65536&o)){r._pushContext();var i;e===x?n&&"number"==typeof n.length?i=U(t).apply(this._boundValue(),n):(i=B,i.e=new j("cannot .spread() a non-array: "+f.classString(n))):i=U(t).call(e,n);var a=r._popContext();o=r._bitField,0===(65536&o)&&(i===R?r._reject(n):i===B?r._rejectCallback(i.e,!1):(A.checkForgottenReturns(i,a,"",r,this),r._resolveCallback(i)))}},o.prototype._target=function(){for(var t=this;t._isFollowing();)t=t._followee();return t},o.prototype._followee=function(){return this._rejectionHandler0},o.prototype._setFollowee=function(t){this._rejectionHandler0=t},o.prototype._settlePromise=function(t,e,r,i){var a=t instanceof o,s=this._bitField,c=0!==(134217728&s);0!==(65536&s)?(a&&t._invokeInternalOnCancel(),r instanceof H&&r.isFinallyHandler()?(r.cancelPromise=t,U(e).call(r,i)===B&&t._reject(B.e)):e===l?t._fulfill(l.call(r)):r instanceof n?r._promiseCancelled(t):a||t instanceof P?t._cancel():r.cancel()):"function"==typeof e?a?(c&&t._setAsyncGuaranteed(),this._settlePromiseFromHandler(e,r,i,t)):e.call(r,i,t):r instanceof n?r._isResolved()||(0!==(33554432&s)?r._promiseFulfilled(i,t):r._promiseRejected(i,t)):a&&(c&&t._setAsyncGuaranteed(),0!==(33554432&s)?t._fulfill(i):t._reject(i))},o.prototype._settlePromiseLateCancellationObserver=function(t){var e=t.handler,n=t.promise,r=t.receiver,i=t.value;"function"==typeof e?n instanceof o?this._settlePromiseFromHandler(e,r,i,n):e.call(r,i,n):n instanceof o&&n._reject(i)},o.prototype._settlePromiseCtx=function(t){this._settlePromise(t.promise,t.handler,t.receiver,t.value)},o.prototype._settlePromise0=function(t,e,n){var r=this._promise0,o=this._receiverAt(0);this._promise0=void 0,this._receiver0=void 0,this._settlePromise(r,t,o,e)},o.prototype._clearCallbackDataAtIndex=function(t){var e=4*t-4;this[e+2]=this[e+3]=this[e+0]=this[e+1]=void 0},o.prototype._fulfill=function(t){var e=this._bitField;if(!((117506048&e)>>>16)){if(t===this){var n=c();return this._attachExtraTrace(n),this._reject(n)}this._setFulfilled(),this._rejectionHandler0=t,(65535&e)>0&&(0!==(134217728&e)?this._settlePromises():k.settlePromises(this),this._dereferenceTrace())}},o.prototype._reject=function(t){var e=this._bitField;if(!((117506048&e)>>>16))return this._setRejected(),this._fulfillmentHandler0=t,this._isFinal()?k.fatalError(t,f.isNode):void((65535&e)>0?k.settlePromises(this):this._ensurePossibleRejectionHandled())},o.prototype._fulfillPromises=function(t,e){for(var n=1;t>n;n++){var r=this._fulfillmentHandlerAt(n),o=this._promiseAt(n),i=this._receiverAt(n);this._clearCallbackDataAtIndex(n),this._settlePromise(o,r,i,e)}},o.prototype._rejectPromises=function(t,e){for(var n=1;t>n;n++){var r=this._rejectionHandlerAt(n),o=this._promiseAt(n),i=this._receiverAt(n);this._clearCallbackDataAtIndex(n),this._settlePromise(o,r,i,e)}},o.prototype._settlePromises=function(){var t=this._bitField,e=65535&t;if(e>0){if(0!==(16842752&t)){var n=this._fulfillmentHandler0;this._settlePromise0(this._rejectionHandler0,n,t),this._rejectPromises(e,n)}else{var r=this._rejectionHandler0;this._settlePromise0(this._fulfillmentHandler0,r,t),this._fulfillPromises(e,r)}this._setLength(0)}this._clearCancellationData()},o.prototype._settledValue=function(){var t=this._bitField;return 0!==(33554432&t)?this._rejectionHandler0:0!==(16777216&t)?this._fulfillmentHandler0:void 0},"undefined"!=typeof Symbol&&Symbol.toStringTag&&C.defineProperty(o.prototype,Symbol.toStringTag,{get:function(){return"Object"}}),o.defer=o.pending=function(){A.deprecated("Promise.defer","new Promise");var t=new o(T);return{promise:t,resolve:i,reject:a}},f.notEnumerableProp(o,"_makeSelfResolutionError",c),t("./method")(o,T,S,u,A),t("./bind")(o,T,S,A),t("./cancel")(o,P,u,A),t("./direct_resolve")(o),t("./synchronous_inspection")(o),t("./join")(o,P,S,T,k),o.Promise=o,o.version="3.7.2",f.toFastProperties(o),f.toFastProperties(o.prototype),s({a:1}),s({b:2}),s({c:3}),s(1),s(function(){}),s(void 0),s(!1),s(new o(T)),A.setBounds(w.firstLineError,f.lastLineError),o}},{"./async":1,"./bind":2,"./cancel":4,"./catch_filter":5,"./context":6,"./debuggability":7,"./direct_resolve":8,"./errors":9,"./es5":10,"./finally":11,"./join":12,"./method":13,"./nodeback":14,"./promise_array":16,"./synchronous_inspection":19,"./thenables":20,"./util":21,async_hooks:void 0}],16:[function(t,e,n){"use strict";e.exports=function(e,n,r,o,i){function a(t){switch(t){case-2:return[];case-3:return{};case-6:return new Map}}function s(t){var r=this._promise=new e(n);t instanceof e&&(r._propagateFrom(t,3),t.suppressUnhandledRejections()),r._setOnCancel(this),this._values=t,this._length=0,this._totalResolved=0,this._init(void 0,-2)}var c=t("./util");c.isArray;return c.inherits(s,i),s.prototype.length=function(){return this._length},s.prototype.promise=function(){return this._promise},s.prototype._init=function l(t,n){var i=r(this._values,this._promise);if(i instanceof e){i=i._target();var s=i._bitField;if(this._values=i,0===(50397184&s))return this._promise._setAsyncGuaranteed(),i._then(l,this._reject,void 0,this,n);if(0===(33554432&s))return 0!==(16777216&s)?this._reject(i._reason()):this._cancel();i=i._value()}if(i=c.asArray(i),null===i){var u=o("expecting an array or an iterable object but got "+c.classString(i)).reason();return void this._promise._rejectCallback(u,!1)}return 0===i.length?void(-5===n?this._resolveEmptyArray():this._resolve(a(n))):void this._iterate(i)},s.prototype._iterate=function(t){var n=this.getActualLength(t.length);this._length=n,this._values=this.shouldCopyValues()?new Array(n):this._values;for(var o=this._promise,i=!1,a=null,s=0;n>s;++s){var c=r(t[s],o);c instanceof e?(c=c._target(),a=c._bitField):a=null,i?null!==a&&c.suppressUnhandledRejections():null!==a?0===(50397184&a)?(c._proxy(this,s),this._values[s]=c):i=0!==(33554432&a)?this._promiseFulfilled(c._value(),s):0!==(16777216&a)?this._promiseRejected(c._reason(),s):this._promiseCancelled(s):i=this._promiseFulfilled(c,s)}i||o._setAsyncGuaranteed()},s.prototype._isResolved=function(){return null===this._values},s.prototype._resolve=function(t){this._values=null,this._promise._fulfill(t)},s.prototype._cancel=function(){!this._isResolved()&&this._promise._isCancellable()&&(this._values=null,this._promise._cancel())},s.prototype._reject=function(t){this._values=null,this._promise._rejectCallback(t,!1)},s.prototype._promiseFulfilled=function(t,e){this._values[e]=t;var n=++this._totalResolved;return n>=this._length?(this._resolve(this._values),!0):!1},s.prototype._promiseCancelled=function(){return this._cancel(),!0},s.prototype._promiseRejected=function(t){return this._totalResolved++,this._reject(t),!0},s.prototype._resultCancelled=function(){if(!this._isResolved()){var t=this._values;if(this._cancel(),t instanceof e)t.cancel();else for(var n=0;ni;++i)n[i+r]=t[i+e],t[i+e]=void 0}function o(t){this._capacity=t,this._length=0,this._front=0}o.prototype._willBeOverCapacity=function(t){return this._capacityn;++n)o[n]=t[n];return o[n]=e,o}function l(t,e,n){if(!F.isES5)return{}.hasOwnProperty.call(t,e)?t[e]:void 0;var r=Object.getOwnPropertyDescriptor(t,e);return null!=r?null==r.get&&null==r.set?r.value:n:void 0}function u(t,e,n){if(i(t))return t;var r={value:n,configurable:!0,enumerable:!1,writable:!0};return F.defineProperty(t,e,r),t}function p(t){throw t}function f(t){try{if("function"==typeof t){var e=F.names(t.prototype),n=F.isES5&&e.length>1,r=e.length>0&&!(1===e.length&&"constructor"===e[0]),o=A.test(t+"")&&F.names(t).length>0;if(n||r||o)return!0}return!1}catch(i){return!1}}function h(t){function e(){}function n(){return typeof r.foo}e.prototype=t;var r=new e;return n(),n(),t}function d(t){return H.test(t)}function _(t,e,n){for(var r=new Array(t),o=0;t>o;++o)r[o]=e+o+n;return r}function v(t){try{return t+""}catch(e){return"[no string representation]"}}function y(t){return t instanceof Error||null!==t&&"object"==typeof t&&"string"==typeof t.message&&"string"==typeof t.name}function g(t){try{u(t,"isOperational",!0)}catch(e){}}function m(t){return null==t?!1:t instanceof Error.__BluebirdErrorTypes__.OperationalError||t.isOperational===!0}function b(t){return y(t)&&F.propertyIsWritable(t,"stack")}function C(t){return{}.toString.call(t)}function w(t,e,n){for(var r=F.names(t),o=0;o10||t[0]>0}(),V.nodeSupportsAsyncResource=V.isNode&&function(){var e=!1;try{var n=t("async_hooks").AsyncResource;e="function"==typeof n.prototype.runInAsyncScope}catch(r){e=!1}return e}(),V.isNode&&V.toFastProperties(process);try{throw new Error}catch(Q){V.lastLineError=Q}e.exports=V},{"./es5":10,async_hooks:void 0}]},{},[3])(3)}),"undefined"!=typeof window&&null!==window?window.P=window.Promise:"undefined"!=typeof self&&null!==self&&(self.P=self.Promise);
neverwrote-master@df4dcf72582/api/node_modules/bluebird/js/browser/bluebird.js
/* @preserve
* The MIT License (MIT)
*
* Copyright (c) 2013-2018 Petka Antonov
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
/**
* bluebird build version 3.7.2
* Features enabled: core, race, call_get, generators, map, nodeify, promisify, props, reduce, settle, some, using, timers, filter, any, each
*/
!function(e){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=e();else if("function"==typeof define&&define.amd)define([],e);else{var f;"undefined"!=typeof window?f=window:"undefined"!=typeof global?f=global:"undefined"!=typeof self&&(f=self),f.Promise=e()}}(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof _dereq_=="function"&&_dereq_;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof _dereq_=="function"&&_dereq_;for(var o=0;o"use strict";
module.exports = function(Promise) {
var SomePromiseArray = Promise._SomePromiseArray;
function any(promises) {
var ret = new SomePromiseArray(promises);
var promise = ret.promise();
ret.setHowMany(1);
ret.setUnwrap();
ret.init();
return promise;
}
Promise.any = function (promises) {
return any(promises);
};
Promise.prototype.any = function () {
return any(this);
};
};
},{}],2:[function(_dereq_,module,exports){
"use strict";
var firstLineError;
try {throw new Error(); } catch (e) {firstLineError = e;}
var schedule = _dereq_("./schedule");
var Queue = _dereq_("./queue");
function Async() {
this._customScheduler = false;
this._isTickUsed = false;
this._lateQueue = new Queue(16);
this._normalQueue = new Queue(16);
this._haveDrainedQueues = false;
var self = this;
this.drainQueues = function () {
self._drainQueues();
};
this._schedule = schedule;
}
Async.prototype.setScheduler = function(fn) {
var prev = this._schedule;
this._schedule = fn;
this._customScheduler = true;
return prev;
};
Async.prototype.hasCustomScheduler = function() {
return this._customScheduler;
};
Async.prototype.haveItemsQueued = function () {
return this._isTickUsed || this._haveDrainedQueues;
};
Async.prototype.fatalError = function(e, isNode) {
if (isNode) {
process.stderr.write("Fatal " + (e instanceof Error ? e.stack : e) +
"\n");
process.exit(2);
} else {
this.throwLater(e);
}
};
Async.prototype.throwLater = function(fn, arg) {
if (arguments.length === 1) {
arg = fn;
fn = function () { throw arg; };
}
if (typeof setTimeout !== "undefined") {
setTimeout(function() {
fn(arg);
}, 0);
} else try {
this._schedule(function() {
fn(arg);
});
} catch (e) {
throw new Error("No async scheduler available\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
};
function AsyncInvokeLater(fn, receiver, arg) {
this._lateQueue.push(fn, receiver, arg);
this._queueTick();
}
function AsyncInvoke(fn, receiver, arg) {
this._normalQueue.push(fn, receiver, arg);
this._queueTick();
}
function AsyncSettlePromises(promise) {
this._normalQueue._pushOne(promise);
this._queueTick();
}
Async.prototype.invokeLater = AsyncInvokeLater;
Async.prototype.invoke = AsyncInvoke;
Async.prototype.settlePromises = AsyncSettlePromises;
function _drainQueue(queue) {
while (queue.length() > 0) {
_drainQueueStep(queue);
}
}
function _drainQueueStep(queue) {
var fn = queue.shift();
if (typeof fn !== "function") {
fn._settlePromises();
} else {
var receiver = queue.shift();
var arg = queue.shift();
fn.call(receiver, arg);
}
}
Async.prototype._drainQueues = function () {
_drainQueue(this._normalQueue);
this._reset();
this._haveDrainedQueues = true;
_drainQueue(this._lateQueue);
};
Async.prototype._queueTick = function () {
if (!this._isTickUsed) {
this._isTickUsed = true;
this._schedule(this.drainQueues);
}
};
Async.prototype._reset = function () {
this._isTickUsed = false;
};
module.exports = Async;
module.exports.firstLineError = firstLineError;
},{"./queue":26,"./schedule":29}],3:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL, tryConvertToPromise, debug) {
var calledBind = false;
var rejectThis = function(_, e) {
this._reject(e);
};
var targetRejected = function(e, context) {
context.promiseRejectionQueued = true;
context.bindingPromise._then(rejectThis, rejectThis, null, this, e);
};
var bindingResolved = function(thisArg, context) {
if (((this._bitField & 50397184) === 0)) {
this._resolveCallback(context.target);
}
};
var bindingRejected = function(e, context) {
if (!context.promiseRejectionQueued) this._reject(e);
};
Promise.prototype.bind = function (thisArg) {
if (!calledBind) {
calledBind = true;
Promise.prototype._propagateFrom = debug.propagateFromFunction();
Promise.prototype._boundValue = debug.boundValueFunction();
}
var maybePromise = tryConvertToPromise(thisArg);
var ret = new Promise(INTERNAL);
ret._propagateFrom(this, 1);
var target = this._target();
ret._setBoundTo(maybePromise);
if (maybePromise instanceof Promise) {
var context = {
promiseRejectionQueued: false,
promise: ret,
target: target,
bindingPromise: maybePromise
};
target._then(INTERNAL, targetRejected, undefined, ret, context);
maybePromise._then(
bindingResolved, bindingRejected, undefined, ret, context);
ret._setOnCancel(maybePromise);
} else {
ret._resolveCallback(target);
}
return ret;
};
Promise.prototype._setBoundTo = function (obj) {
if (obj !== undefined) {
this._bitField = this._bitField | 2097152;
this._boundTo = obj;
} else {
this._bitField = this._bitField & (~2097152);
}
};
Promise.prototype._isBound = function () {
return (this._bitField & 2097152) === 2097152;
};
Promise.bind = function (thisArg, value) {
return Promise.resolve(value).bind(thisArg);
};
};
},{}],4:[function(_dereq_,module,exports){
"use strict";
var old;
if (typeof Promise !== "undefined") old = Promise;
function noConflict() {
try { if (Promise === bluebird) Promise = old; }
catch (e) {}
return bluebird;
}
var bluebird = _dereq_("./promise")();
bluebird.noConflict = noConflict;
module.exports = bluebird;
},{"./promise":22}],5:[function(_dereq_,module,exports){
"use strict";
var cr = Object.create;
if (cr) {
var callerCache = cr(null);
var getterCache = cr(null);
callerCache[" size"] = getterCache[" size"] = 0;
}
module.exports = function(Promise) {
var util = _dereq_("./util");
var canEvaluate = util.canEvaluate;
var isIdentifier = util.isIdentifier;
var getMethodCaller;
var getGetter;
if (!true) {
var makeMethodCaller = function (methodName) {
return new Function("ensureMethod", " \n\
return function(obj) { \n\
'use strict' \n\
var len = this.length; \n\
ensureMethod(obj, 'methodName'); \n\
switch(len) { \n\
case 1: return obj.methodName(this[0]); \n\
case 2: return obj.methodName(this[0], this[1]); \n\
case 3: return obj.methodName(this[0], this[1], this[2]); \n\
case 0: return obj.methodName(); \n\
default: \n\
return obj.methodName.apply(obj, this); \n\
} \n\
}; \n\
".replace(/methodName/g, methodName))(ensureMethod);
};
var makeGetter = function (propertyName) {
return new Function("obj", " \n\
'use strict'; \n\
return obj.propertyName; \n\
".replace("propertyName", propertyName));
};
var getCompiled = function(name, compiler, cache) {
var ret = cache[name];
if (typeof ret !== "function") {
if (!isIdentifier(name)) {
return null;
}
ret = compiler(name);
cache[name] = ret;
cache[" size"]++;
if (cache[" size"] > 512) {
var keys = Object.keys(cache);
for (var i = 0; i < 256; ++i) delete cache[keys[i]];
cache[" size"] = keys.length - 256;
}
}
return ret;
};
getMethodCaller = function(name) {
return getCompiled(name, makeMethodCaller, callerCache);
};
getGetter = function(name) {
return getCompiled(name, makeGetter, getterCache);
};
}
function ensureMethod(obj, methodName) {
var fn;
if (obj != null) fn = obj[methodName];
if (typeof fn !== "function") {
var message = "Object " + util.classString(obj) + " has no method '" +
util.toString(methodName) + "'";
throw new Promise.TypeError(message);
}
return fn;
}
function caller(obj) {
var methodName = this.pop();
var fn = ensureMethod(obj, methodName);
return fn.apply(obj, this);
}
Promise.prototype.call = function (methodName) {
var args = [].slice.call(arguments, 1);;
if (!true) {
if (canEvaluate) {
var maybeCaller = getMethodCaller(methodName);
if (maybeCaller !== null) {
return this._then(
maybeCaller, undefined, undefined, args, undefined);
}
}
}
args.push(methodName);
return this._then(caller, undefined, undefined, args, undefined);
};
function namedGetter(obj) {
return obj[this];
}
function indexedGetter(obj) {
var index = +this;
if (index < 0) index = Math.max(0, index + obj.length);
return obj[index];
}
Promise.prototype.get = function (propertyName) {
var isIndex = (typeof propertyName === "number");
var getter;
if (!isIndex) {
if (canEvaluate) {
var maybeGetter = getGetter(propertyName);
getter = maybeGetter !== null ? maybeGetter : namedGetter;
} else {
getter = namedGetter;
}
} else {
getter = indexedGetter;
}
return this._then(getter, undefined, undefined, propertyName, undefined);
};
};
},{"./util":36}],6:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, PromiseArray, apiRejection, debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
var async = Promise._async;
Promise.prototype["break"] = Promise.prototype.cancel = function() {
if (!debug.cancellation()) return this._warn("cancellation is disabled");
var promise = this;
var child = promise;
while (promise._isCancellable()) {
if (!promise._cancelBy(child)) {
if (child._isFollowing()) {
child._followee().cancel();
} else {
child._cancelBranched();
}
break;
}
var parent = promise._cancellationParent;
if (parent == null || !parent._isCancellable()) {
if (promise._isFollowing()) {
promise._followee().cancel();
} else {
promise._cancelBranched();
}
break;
} else {
if (promise._isFollowing()) promise._followee().cancel();
promise._setWillBeCancelled();
child = promise;
promise = parent;
}
}
};
Promise.prototype._branchHasCancelled = function() {
this._branchesRemainingToCancel--;
};
Promise.prototype._enoughBranchesHaveCancelled = function() {
return this._branchesRemainingToCancel === undefined ||
this._branchesRemainingToCancel <= 0;
};
Promise.prototype._cancelBy = function(canceller) {
if (canceller === this) {
this._branchesRemainingToCancel = 0;
this._invokeOnCancel();
return true;
} else {
this._branchHasCancelled();
if (this._enoughBranchesHaveCancelled()) {
this._invokeOnCancel();
return true;
}
}
return false;
};
Promise.prototype._cancelBranched = function() {
if (this._enoughBranchesHaveCancelled()) {
this._cancel();
}
};
Promise.prototype._cancel = function() {
if (!this._isCancellable()) return;
this._setCancelled();
async.invoke(this._cancelPromises, this, undefined);
};
Promise.prototype._cancelPromises = function() {
if (this._length() > 0) this._settlePromises();
};
Promise.prototype._unsetOnCancel = function() {
this._onCancelField = undefined;
};
Promise.prototype._isCancellable = function() {
return this.isPending() && !this._isCancelled();
};
Promise.prototype.isCancellable = function() {
return this.isPending() && !this.isCancelled();
};
Promise.prototype._doInvokeOnCancel = function(onCancelCallback, internalOnly) {
if (util.isArray(onCancelCallback)) {
for (var i = 0; i < onCancelCallback.length; ++i) {
this._doInvokeOnCancel(onCancelCallback[i], internalOnly);
}
} else if (onCancelCallback !== undefined) {
if (typeof onCancelCallback === "function") {
if (!internalOnly) {
var e = tryCatch(onCancelCallback).call(this._boundValue());
if (e === errorObj) {
this._attachExtraTrace(e.e);
async.throwLater(e.e);
}
}
} else {
onCancelCallback._resultCancelled(this);
}
}
};
Promise.prototype._invokeOnCancel = function() {
var onCancelCallback = this._onCancel();
this._unsetOnCancel();
async.invoke(this._doInvokeOnCancel, this, onCancelCallback);
};
Promise.prototype._invokeInternalOnCancel = function() {
if (this._isCancellable()) {
this._doInvokeOnCancel(this._onCancel(), true);
this._unsetOnCancel();
}
};
Promise.prototype._resultCancelled = function() {
this.cancel();
};
};
},{"./util":36}],7:[function(_dereq_,module,exports){
"use strict";
module.exports = function(NEXT_FILTER) {
var util = _dereq_("./util");
var getKeys = _dereq_("./es5").keys;
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
function catchFilter(instances, cb, promise) {
return function(e) {
var boundTo = promise._boundValue();
predicateLoop: for (var i = 0; i < instances.length; ++i) {
var item = instances[i];
if (item === Error ||
(item != null && item.prototype instanceof Error)) {
if (e instanceof item) {
return tryCatch(cb).call(boundTo, e);
}
} else if (typeof item === "function") {
var matchesPredicate = tryCatch(item).call(boundTo, e);
if (matchesPredicate === errorObj) {
return matchesPredicate;
} else if (matchesPredicate) {
return tryCatch(cb).call(boundTo, e);
}
} else if (util.isObject(e)) {
var keys = getKeys(item);
for (var j = 0; j < keys.length; ++j) {
var key = keys[j];
if (item[key] != e[key]) {
continue predicateLoop;
}
}
return tryCatch(cb).call(boundTo, e);
}
}
return NEXT_FILTER;
};
}
return catchFilter;
};
},{"./es5":13,"./util":36}],8:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
var longStackTraces = false;
var contextStack = [];
Promise.prototype._promiseCreated = function() {};
Promise.prototype._pushContext = function() {};
Promise.prototype._popContext = function() {return null;};
Promise._peekContext = Promise.prototype._peekContext = function() {};
function Context() {
this._trace = new Context.CapturedTrace(peekContext());
}
Context.prototype._pushContext = function () {
if (this._trace !== undefined) {
this._trace._promiseCreated = null;
contextStack.push(this._trace);
}
};
Context.prototype._popContext = function () {
if (this._trace !== undefined) {
var trace = contextStack.pop();
var ret = trace._promiseCreated;
trace._promiseCreated = null;
return ret;
}
return null;
};
function createContext() {
if (longStackTraces) return new Context();
}
function peekContext() {
var lastIndex = contextStack.length - 1;
if (lastIndex >= 0) {
return contextStack[lastIndex];
}
return undefined;
}
Context.CapturedTrace = null;
Context.create = createContext;
Context.deactivateLongStackTraces = function() {};
Context.activateLongStackTraces = function() {
var Promise_pushContext = Promise.prototype._pushContext;
var Promise_popContext = Promise.prototype._popContext;
var Promise_PeekContext = Promise._peekContext;
var Promise_peekContext = Promise.prototype._peekContext;
var Promise_promiseCreated = Promise.prototype._promiseCreated;
Context.deactivateLongStackTraces = function() {
Promise.prototype._pushContext = Promise_pushContext;
Promise.prototype._popContext = Promise_popContext;
Promise._peekContext = Promise_PeekContext;
Promise.prototype._peekContext = Promise_peekContext;
Promise.prototype._promiseCreated = Promise_promiseCreated;
longStackTraces = false;
};
longStackTraces = true;
Promise.prototype._pushContext = Context.prototype._pushContext;
Promise.prototype._popContext = Context.prototype._popContext;
Promise._peekContext = Promise.prototype._peekContext = peekContext;
Promise.prototype._promiseCreated = function() {
var ctx = this._peekContext();
if (ctx && ctx._promiseCreated == null) ctx._promiseCreated = this;
};
};
return Context;
};
},{}],9:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, Context,
enableAsyncHooks, disableAsyncHooks) {
var async = Promise._async;
var Warning = _dereq_("./errors").Warning;
var util = _dereq_("./util");
var es5 = _dereq_("./es5");
var canAttachTrace = util.canAttachTrace;
var unhandledRejectionHandled;
var possiblyUnhandledRejection;
var bluebirdFramePattern =
/[\\\/]bluebird[\\\/]js[\\\/](release|debug|instrumented)/;
var nodeFramePattern = /\((?:timers\.js):\d+:\d+\)/;
var parseLinePattern = /[\/<\(](.+?):(\d+):(\d+)\)?\s*$/;
var stackFramePattern = null;
var formatStack = null;
var indentStackFrames = false;
var printWarning;
var debugging = !!(util.env("BLUEBIRD_DEBUG") != 0 &&
(true ||
util.env("BLUEBIRD_DEBUG") ||
util.env("NODE_ENV") === "development"));
var warnings = !!(util.env("BLUEBIRD_WARNINGS") != 0 &&
(debugging || util.env("BLUEBIRD_WARNINGS")));
var longStackTraces = !!(util.env("BLUEBIRD_LONG_STACK_TRACES") != 0 &&
(debugging || util.env("BLUEBIRD_LONG_STACK_TRACES")));
var wForgottenReturn = util.env("BLUEBIRD_W_FORGOTTEN_RETURN") != 0 &&
(warnings || !!util.env("BLUEBIRD_W_FORGOTTEN_RETURN"));
var deferUnhandledRejectionCheck;
(function() {
var promises = [];
function unhandledRejectionCheck() {
for (var i = 0; i < promises.length; ++i) {
promises[i]._notifyUnhandledRejection();
}
unhandledRejectionClear();
}
function unhandledRejectionClear() {
promises.length = 0;
}
deferUnhandledRejectionCheck = function(promise) {
promises.push(promise);
setTimeout(unhandledRejectionCheck, 1);
};
es5.defineProperty(Promise, "_unhandledRejectionCheck", {
value: unhandledRejectionCheck
});
es5.defineProperty(Promise, "_unhandledRejectionClear", {
value: unhandledRejectionClear
});
})();
Promise.prototype.suppressUnhandledRejections = function() {
var target = this._target();
target._bitField = ((target._bitField & (~1048576)) |
524288);
};
Promise.prototype._ensurePossibleRejectionHandled = function () {
if ((this._bitField & 524288) !== 0) return;
this._setRejectionIsUnhandled();
deferUnhandledRejectionCheck(this);
};
Promise.prototype._notifyUnhandledRejectionIsHandled = function () {
fireRejectionEvent("rejectionHandled",
unhandledRejectionHandled, undefined, this);
};
Promise.prototype._setReturnedNonUndefined = function() {
this._bitField = this._bitField | 268435456;
};
Promise.prototype._returnedNonUndefined = function() {
return (this._bitField & 268435456) !== 0;
};
Promise.prototype._notifyUnhandledRejection = function () {
if (this._isRejectionUnhandled()) {
var reason = this._settledValue();
this._setUnhandledRejectionIsNotified();
fireRejectionEvent("unhandledRejection",
possiblyUnhandledRejection, reason, this);
}
};
Promise.prototype._setUnhandledRejectionIsNotified = function () {
this._bitField = this._bitField | 262144;
};
Promise.prototype._unsetUnhandledRejectionIsNotified = function () {
this._bitField = this._bitField & (~262144);
};
Promise.prototype._isUnhandledRejectionNotified = function () {
return (this._bitField & 262144) > 0;
};
Promise.prototype._setRejectionIsUnhandled = function () {
this._bitField = this._bitField | 1048576;
};
Promise.prototype._unsetRejectionIsUnhandled = function () {
this._bitField = this._bitField & (~1048576);
if (this._isUnhandledRejectionNotified()) {
this._unsetUnhandledRejectionIsNotified();
this._notifyUnhandledRejectionIsHandled();
}
};
Promise.prototype._isRejectionUnhandled = function () {
return (this._bitField & 1048576) > 0;
};
Promise.prototype._warn = function(message, shouldUseOwnTrace, promise) {
return warn(message, shouldUseOwnTrace, promise || this);
};
Promise.onPossiblyUnhandledRejection = function (fn) {
var context = Promise._getContext();
possiblyUnhandledRejection = util.contextBind(context, fn);
};
Promise.onUnhandledRejectionHandled = function (fn) {
var context = Promise._getContext();
unhandledRejectionHandled = util.contextBind(context, fn);
};
var disableLongStackTraces = function() {};
Promise.longStackTraces = function () {
if (async.haveItemsQueued() && !config.longStackTraces) {
throw new Error("cannot enable long stack traces after promises have been created\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
if (!config.longStackTraces && longStackTracesIsSupported()) {
var Promise_captureStackTrace = Promise.prototype._captureStackTrace;
var Promise_attachExtraTrace = Promise.prototype._attachExtraTrace;
var Promise_dereferenceTrace = Promise.prototype._dereferenceTrace;
config.longStackTraces = true;
disableLongStackTraces = function() {
if (async.haveItemsQueued() && !config.longStackTraces) {
throw new Error("cannot enable long stack traces after promises have been created\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
Promise.prototype._captureStackTrace = Promise_captureStackTrace;
Promise.prototype._attachExtraTrace = Promise_attachExtraTrace;
Promise.prototype._dereferenceTrace = Promise_dereferenceTrace;
Context.deactivateLongStackTraces();
config.longStackTraces = false;
};
Promise.prototype._captureStackTrace = longStackTracesCaptureStackTrace;
Promise.prototype._attachExtraTrace = longStackTracesAttachExtraTrace;
Promise.prototype._dereferenceTrace = longStackTracesDereferenceTrace;
Context.activateLongStackTraces();
}
};
Promise.hasLongStackTraces = function () {
return config.longStackTraces && longStackTracesIsSupported();
};
var legacyHandlers = {
unhandledrejection: {
before: function() {
var ret = util.global.onunhandledrejection;
util.global.onunhandledrejection = null;
return ret;
},
after: function(fn) {
util.global.onunhandledrejection = fn;
}
},
rejectionhandled: {
before: function() {
var ret = util.global.onrejectionhandled;
util.global.onrejectionhandled = null;
return ret;
},
after: function(fn) {
util.global.onrejectionhandled = fn;
}
}
};
var fireDomEvent = (function() {
var dispatch = function(legacy, e) {
if (legacy) {
var fn;
try {
fn = legacy.before();
return !util.global.dispatchEvent(e);
} finally {
legacy.after(fn);
}
} else {
return !util.global.dispatchEvent(e);
}
};
try {
if (typeof CustomEvent === "function") {
var event = new CustomEvent("CustomEvent");
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var eventData = {
detail: event,
cancelable: true
};
var domEvent = new CustomEvent(name, eventData);
es5.defineProperty(
domEvent, "promise", {value: event.promise});
es5.defineProperty(
domEvent, "reason", {value: event.reason});
return dispatch(legacyHandlers[name], domEvent);
};
} else if (typeof Event === "function") {
var event = new Event("CustomEvent");
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var domEvent = new Event(name, {
cancelable: true
});
domEvent.detail = event;
es5.defineProperty(domEvent, "promise", {value: event.promise});
es5.defineProperty(domEvent, "reason", {value: event.reason});
return dispatch(legacyHandlers[name], domEvent);
};
} else {
var event = document.createEvent("CustomEvent");
event.initCustomEvent("testingtheevent", false, true, {});
util.global.dispatchEvent(event);
return function(name, event) {
name = name.toLowerCase();
var domEvent = document.createEvent("CustomEvent");
domEvent.initCustomEvent(name, false, true,
event);
return dispatch(legacyHandlers[name], domEvent);
};
}
} catch (e) {}
return function() {
return false;
};
})();
var fireGlobalEvent = (function() {
if (util.isNode) {
return function() {
return process.emit.apply(process, arguments);
};
} else {
if (!util.global) {
return function() {
return false;
};
}
return function(name) {
var methodName = "on" + name.toLowerCase();
var method = util.global[methodName];
if (!method) return false;
method.apply(util.global, [].slice.call(arguments, 1));
return true;
};
}
})();
function generatePromiseLifecycleEventObject(name, promise) {
return {promise: promise};
}
var eventToObjectGenerator = {
promiseCreated: generatePromiseLifecycleEventObject,
promiseFulfilled: generatePromiseLifecycleEventObject,
promiseRejected: generatePromiseLifecycleEventObject,
promiseResolved: generatePromiseLifecycleEventObject,
promiseCancelled: generatePromiseLifecycleEventObject,
promiseChained: function(name, promise, child) {
return {promise: promise, child: child};
},
warning: function(name, warning) {
return {warning: warning};
},
unhandledRejection: function (name, reason, promise) {
return {reason: reason, promise: promise};
},
rejectionHandled: generatePromiseLifecycleEventObject
};
var activeFireEvent = function (name) {
var globalEventFired = false;
try {
globalEventFired = fireGlobalEvent.apply(null, arguments);
} catch (e) {
async.throwLater(e);
globalEventFired = true;
}
var domEventFired = false;
try {
domEventFired = fireDomEvent(name,
eventToObjectGenerator[name].apply(null, arguments));
} catch (e) {
async.throwLater(e);
domEventFired = true;
}
return domEventFired || globalEventFired;
};
Promise.config = function(opts) {
opts = Object(opts);
if ("longStackTraces" in opts) {
if (opts.longStackTraces) {
Promise.longStackTraces();
} else if (!opts.longStackTraces && Promise.hasLongStackTraces()) {
disableLongStackTraces();
}
}
if ("warnings" in opts) {
var warningsOption = opts.warnings;
config.warnings = !!warningsOption;
wForgottenReturn = config.warnings;
if (util.isObject(warningsOption)) {
if ("wForgottenReturn" in warningsOption) {
wForgottenReturn = !!warningsOption.wForgottenReturn;
}
}
}
if ("cancellation" in opts && opts.cancellation && !config.cancellation) {
if (async.haveItemsQueued()) {
throw new Error(
"cannot enable cancellation after promises are in use");
}
Promise.prototype._clearCancellationData =
cancellationClearCancellationData;
Promise.prototype._propagateFrom = cancellationPropagateFrom;
Promise.prototype._onCancel = cancellationOnCancel;
Promise.prototype._setOnCancel = cancellationSetOnCancel;
Promise.prototype._attachCancellationCallback =
cancellationAttachCancellationCallback;
Promise.prototype._execute = cancellationExecute;
propagateFromFunction = cancellationPropagateFrom;
config.cancellation = true;
}
if ("monitoring" in opts) {
if (opts.monitoring && !config.monitoring) {
config.monitoring = true;
Promise.prototype._fireEvent = activeFireEvent;
} else if (!opts.monitoring && config.monitoring) {
config.monitoring = false;
Promise.prototype._fireEvent = defaultFireEvent;
}
}
if ("asyncHooks" in opts && util.nodeSupportsAsyncResource) {
var prev = config.asyncHooks;
var cur = !!opts.asyncHooks;
if (prev !== cur) {
config.asyncHooks = cur;
if (cur) {
enableAsyncHooks();
} else {
disableAsyncHooks();
}
}
}
return Promise;
};
function defaultFireEvent() { return false; }
Promise.prototype._fireEvent = defaultFireEvent;
Promise.prototype._execute = function(executor, resolve, reject) {
try {
executor(resolve, reject);
} catch (e) {
return e;
}
};
Promise.prototype._onCancel = function () {};
Promise.prototype._setOnCancel = function (handler) { ; };
Promise.prototype._attachCancellationCallback = function(onCancel) {
;
};
Promise.prototype._captureStackTrace = function () {};
Promise.prototype._attachExtraTrace = function () {};
Promise.prototype._dereferenceTrace = function () {};
Promise.prototype._clearCancellationData = function() {};
Promise.prototype._propagateFrom = function (parent, flags) {
;
;
};
function cancellationExecute(executor, resolve, reject) {
var promise = this;
try {
executor(resolve, reject, function(onCancel) {
if (typeof onCancel !== "function") {
throw new TypeError("onCancel must be a function, got: " +
util.toString(onCancel));
}
promise._attachCancellationCallback(onCancel);
});
} catch (e) {
return e;
}
}
function cancellationAttachCancellationCallback(onCancel) {
if (!this._isCancellable()) return this;
var previousOnCancel = this._onCancel();
if (previousOnCancel !== undefined) {
if (util.isArray(previousOnCancel)) {
previousOnCancel.push(onCancel);
} else {
this._setOnCancel([previousOnCancel, onCancel]);
}
} else {
this._setOnCancel(onCancel);
}
}
function cancellationOnCancel() {
return this._onCancelField;
}
function cancellationSetOnCancel(onCancel) {
this._onCancelField = onCancel;
}
function cancellationClearCancellationData() {
this._cancellationParent = undefined;
this._onCancelField = undefined;
}
function cancellationPropagateFrom(parent, flags) {
if ((flags & 1) !== 0) {
this._cancellationParent = parent;
var branchesRemainingToCancel = parent._branchesRemainingToCancel;
if (branchesRemainingToCancel === undefined) {
branchesRemainingToCancel = 0;
}
parent._branchesRemainingToCancel = branchesRemainingToCancel + 1;
}
if ((flags & 2) !== 0 && parent._isBound()) {
this._setBoundTo(parent._boundTo);
}
}
function bindingPropagateFrom(parent, flags) {
if ((flags & 2) !== 0 && parent._isBound()) {
this._setBoundTo(parent._boundTo);
}
}
var propagateFromFunction = bindingPropagateFrom;
function boundValueFunction() {
var ret = this._boundTo;
if (ret !== undefined) {
if (ret instanceof Promise) {
if (ret.isFulfilled()) {
return ret.value();
} else {
return undefined;
}
}
}
return ret;
}
function longStackTracesCaptureStackTrace() {
this._trace = new CapturedTrace(this._peekContext());
}
function longStackTracesAttachExtraTrace(error, ignoreSelf) {
if (canAttachTrace(error)) {
var trace = this._trace;
if (trace !== undefined) {
if (ignoreSelf) trace = trace._parent;
}
if (trace !== undefined) {
trace.attachExtraTrace(error);
} else if (!error.__stackCleaned__) {
var parsed = parseStackAndMessage(error);
util.notEnumerableProp(error, "stack",
parsed.message + "\n" + parsed.stack.join("\n"));
util.notEnumerableProp(error, "__stackCleaned__", true);
}
}
}
function longStackTracesDereferenceTrace() {
this._trace = undefined;
}
function checkForgottenReturns(returnValue, promiseCreated, name, promise,
parent) {
if (returnValue === undefined && promiseCreated !== null &&
wForgottenReturn) {
if (parent !== undefined && parent._returnedNonUndefined()) return;
if ((promise._bitField & 65535) === 0) return;
if (name) name = name + " ";
var handlerLine = "";
var creatorLine = "";
if (promiseCreated._trace) {
var traceLines = promiseCreated._trace.stack.split("\n");
var stack = cleanStack(traceLines);
for (var i = stack.length - 1; i >= 0; --i) {
var line = stack[i];
if (!nodeFramePattern.test(line)) {
var lineMatches = line.match(parseLinePattern);
if (lineMatches) {
handlerLine = "at " + lineMatches[1] +
":" + lineMatches[2] + ":" + lineMatches[3] + " ";
}
break;
}
}
if (stack.length > 0) {
var firstUserLine = stack[0];
for (var i = 0; i < traceLines.length; ++i) {
if (traceLines[i] === firstUserLine) {
if (i > 0) {
creatorLine = "\n" + traceLines[i - 1];
}
break;
}
}
}
}
var msg = "a promise was created in a " + name +
"handler " + handlerLine + "but was not returned from it, " +
"see http://goo.gl/rRqMUw" +
creatorLine;
promise._warn(msg, true, promiseCreated);
}
}
function deprecated(name, replacement) {
var message = name +
" is deprecated and will be removed in a future version.";
if (replacement) message += " Use " + replacement + " instead.";
return warn(message);
}
function warn(message, shouldUseOwnTrace, promise) {
if (!config.warnings) return;
var warning = new Warning(message);
var ctx;
if (shouldUseOwnTrace) {
promise._attachExtraTrace(warning);
} else if (config.longStackTraces && (ctx = Promise._peekContext())) {
ctx.attachExtraTrace(warning);
} else {
var parsed = parseStackAndMessage(warning);
warning.stack = parsed.message + "\n" + parsed.stack.join("\n");
}
if (!activeFireEvent("warning", warning)) {
formatAndLogError(warning, "", true);
}
}
function reconstructStack(message, stacks) {
for (var i = 0; i < stacks.length - 1; ++i) {
stacks[i].push("From previous event:");
stacks[i] = stacks[i].join("\n");
}
if (i < stacks.length) {
stacks[i] = stacks[i].join("\n");
}
return message + "\n" + stacks.join("\n");
}
function removeDuplicateOrEmptyJumps(stacks) {
for (var i = 0; i < stacks.length; ++i) {
if (stacks[i].length === 0 ||
((i + 1 < stacks.length) && stacks[i][0] === stacks[i+1][0])) {
stacks.splice(i, 1);
i--;
}
}
}
function removeCommonRoots(stacks) {
var current = stacks[0];
for (var i = 1; i < stacks.length; ++i) {
var prev = stacks[i];
var currentLastIndex = current.length - 1;
var currentLastLine = current[currentLastIndex];
var commonRootMeetPoint = -1;
for (var j = prev.length - 1; j >= 0; --j) {
if (prev[j] === currentLastLine) {
commonRootMeetPoint = j;
break;
}
}
for (var j = commonRootMeetPoint; j >= 0; --j) {
var line = prev[j];
if (current[currentLastIndex] === line) {
current.pop();
currentLastIndex--;
} else {
break;
}
}
current = prev;
}
}
function cleanStack(stack) {
var ret = [];
for (var i = 0; i < stack.length; ++i) {
var line = stack[i];
var isTraceLine = " (No stack trace)" === line ||
stackFramePattern.test(line);
var isInternalFrame = isTraceLine && shouldIgnore(line);
if (isTraceLine && !isInternalFrame) {
if (indentStackFrames && line.charAt(0) !== " ") {
line = " " + line;
}
ret.push(line);
}
}
return ret;
}
function stackFramesAsArray(error) {
var stack = error.stack.replace(/\s+$/g, "").split("\n");
for (var i = 0; i < stack.length; ++i) {
var line = stack[i];
if (" (No stack trace)" === line || stackFramePattern.test(line)) {
break;
}
}
if (i > 0 && error.name != "SyntaxError") {
stack = stack.slice(i);
}
return stack;
}
function parseStackAndMessage(error) {
var stack = error.stack;
var message = error.toString();
stack = typeof stack === "string" && stack.length > 0
? stackFramesAsArray(error) : [" (No stack trace)"];
return {
message: message,
stack: error.name == "SyntaxError" ? stack : cleanStack(stack)
};
}
function formatAndLogError(error, title, isSoft) {
if (typeof console !== "undefined") {
var message;
if (util.isObject(error)) {
var stack = error.stack;
message = title + formatStack(stack, error);
} else {
message = title + String(error);
}
if (typeof printWarning === "function") {
printWarning(message, isSoft);
} else if (typeof console.log === "function" ||
typeof console.log === "object") {
console.log(message);
}
}
}
function fireRejectionEvent(name, localHandler, reason, promise) {
var localEventFired = false;
try {
if (typeof localHandler === "function") {
localEventFired = true;
if (name === "rejectionHandled") {
localHandler(promise);
} else {
localHandler(reason, promise);
}
}
} catch (e) {
async.throwLater(e);
}
if (name === "unhandledRejection") {
if (!activeFireEvent(name, reason, promise) && !localEventFired) {
formatAndLogError(reason, "Unhandled rejection ");
}
} else {
activeFireEvent(name, promise);
}
}
function formatNonError(obj) {
var str;
if (typeof obj === "function") {
str = "[function " +
(obj.name || "anonymous") +
"]";
} else {
str = obj && typeof obj.toString === "function"
? obj.toString() : util.toString(obj);
var ruselessToString = /\[object [a-zA-Z0-9$_]+\]/;
if (ruselessToString.test(str)) {
try {
var newStr = JSON.stringify(obj);
str = newStr;
}
catch(e) {
}
}
if (str.length === 0) {
str = "(empty array)";
}
}
return ("(<" + snip(str) + ">, no stack trace)");
}
function snip(str) {
var maxChars = 41;
if (str.length < maxChars) {
return str;
}
return str.substr(0, maxChars - 3) + "...";
}
function longStackTracesIsSupported() {
return typeof captureStackTrace === "function";
}
var shouldIgnore = function() { return false; };
var parseLineInfoRegex = /[\/<\(]([^:\/]+):(\d+):(?:\d+)\)?\s*$/;
function parseLineInfo(line) {
var matches = line.match(parseLineInfoRegex);
if (matches) {
return {
fileName: matches[1],
line: parseInt(matches[2], 10)
};
}
}
function setBounds(firstLineError, lastLineError) {
if (!longStackTracesIsSupported()) return;
var firstStackLines = (firstLineError.stack || "").split("\n");
var lastStackLines = (lastLineError.stack || "").split("\n");
var firstIndex = -1;
var lastIndex = -1;
var firstFileName;
var lastFileName;
for (var i = 0; i < firstStackLines.length; ++i) {
var result = parseLineInfo(firstStackLines[i]);
if (result) {
firstFileName = result.fileName;
firstIndex = result.line;
break;
}
}
for (var i = 0; i < lastStackLines.length; ++i) {
var result = parseLineInfo(lastStackLines[i]);
if (result) {
lastFileName = result.fileName;
lastIndex = result.line;
break;
}
}
if (firstIndex < 0 || lastIndex < 0 || !firstFileName || !lastFileName ||
firstFileName !== lastFileName || firstIndex >= lastIndex) {
return;
}
shouldIgnore = function(line) {
if (bluebirdFramePattern.test(line)) return true;
var info = parseLineInfo(line);
if (info) {
if (info.fileName === firstFileName &&
(firstIndex <= info.line && info.line <= lastIndex)) {
return true;
}
}
return false;
};
}
function CapturedTrace(parent) {
this._parent = parent;
this._promisesCreated = 0;
var length = this._length = 1 + (parent === undefined ? 0 : parent._length);
captureStackTrace(this, CapturedTrace);
if (length > 32) this.uncycle();
}
util.inherits(CapturedTrace, Error);
Context.CapturedTrace = CapturedTrace;
CapturedTrace.prototype.uncycle = function() {
var length = this._length;
if (length < 2) return;
var nodes = [];
var stackToIndex = {};
for (var i = 0, node = this; node !== undefined; ++i) {
nodes.push(node);
node = node._parent;
}
length = this._length = i;
for (var i = length - 1; i >= 0; --i) {
var stack = nodes[i].stack;
if (stackToIndex[stack] === undefined) {
stackToIndex[stack] = i;
}
}
for (var i = 0; i < length; ++i) {
var currentStack = nodes[i].stack;
var index = stackToIndex[currentStack];
if (index !== undefined && index !== i) {
if (index > 0) {
nodes[index - 1]._parent = undefined;
nodes[index - 1]._length = 1;
}
nodes[i]._parent = undefined;
nodes[i]._length = 1;
var cycleEdgeNode = i > 0 ? nodes[i - 1] : this;
if (index < length - 1) {
cycleEdgeNode._parent = nodes[index + 1];
cycleEdgeNode._parent.uncycle();
cycleEdgeNode._length =
cycleEdgeNode._parent._length + 1;
} else {
cycleEdgeNode._parent = undefined;
cycleEdgeNode._length = 1;
}
var currentChildLength = cycleEdgeNode._length + 1;
for (var j = i - 2; j >= 0; --j) {
nodes[j]._length = currentChildLength;
currentChildLength++;
}
return;
}
}
};
CapturedTrace.prototype.attachExtraTrace = function(error) {
if (error.__stackCleaned__) return;
this.uncycle();
var parsed = parseStackAndMessage(error);
var message = parsed.message;
var stacks = [parsed.stack];
var trace = this;
while (trace !== undefined) {
stacks.push(cleanStack(trace.stack.split("\n")));
trace = trace._parent;
}
removeCommonRoots(stacks);
removeDuplicateOrEmptyJumps(stacks);
util.notEnumerableProp(error, "stack", reconstructStack(message, stacks));
util.notEnumerableProp(error, "__stackCleaned__", true);
};
var captureStackTrace = (function stackDetection() {
var v8stackFramePattern = /^\s*at\s*/;
var v8stackFormatter = function(stack, error) {
if (typeof stack === "string") return stack;
if (error.name !== undefined &&
error.message !== undefined) {
return error.toString();
}
return formatNonError(error);
};
if (typeof Error.stackTraceLimit === "number" &&
typeof Error.captureStackTrace === "function") {
Error.stackTraceLimit += 6;
stackFramePattern = v8stackFramePattern;
formatStack = v8stackFormatter;
var captureStackTrace = Error.captureStackTrace;
shouldIgnore = function(line) {
return bluebirdFramePattern.test(line);
};
return function(receiver, ignoreUntil) {
Error.stackTraceLimit += 6;
captureStackTrace(receiver, ignoreUntil);
Error.stackTraceLimit -= 6;
};
}
var err = new Error();
if (typeof err.stack === "string" &&
err.stack.split("\n")[0].indexOf("stackDetection@") >= 0) {
stackFramePattern = /@/;
formatStack = v8stackFormatter;
indentStackFrames = true;
return function captureStackTrace(o) {
o.stack = new Error().stack;
};
}
var hasStackAfterThrow;
try { throw new Error(); }
catch(e) {
hasStackAfterThrow = ("stack" in e);
}
if (!("stack" in err) && hasStackAfterThrow &&
typeof Error.stackTraceLimit === "number") {
stackFramePattern = v8stackFramePattern;
formatStack = v8stackFormatter;
return function captureStackTrace(o) {
Error.stackTraceLimit += 6;
try { throw new Error(); }
catch(e) { o.stack = e.stack; }
Error.stackTraceLimit -= 6;
};
}
formatStack = function(stack, error) {
if (typeof stack === "string") return stack;
if ((typeof error === "object" ||
typeof error === "function") &&
error.name !== undefined &&
error.message !== undefined) {
return error.toString();
}
return formatNonError(error);
};
return null;
})([]);
if (typeof console !== "undefined" && typeof console.warn !== "undefined") {
printWarning = function (message) {
console.warn(message);
};
if (util.isNode && process.stderr.isTTY) {
printWarning = function(message, isSoft) {
var color = isSoft ? "\u001b[33m" : "\u001b[31m";
console.warn(color + message + "\u001b[0m\n");
};
} else if (!util.isNode && typeof (new Error().stack) === "string") {
printWarning = function(message, isSoft) {
console.warn("%c" + message,
isSoft ? "color: darkorange" : "color: red");
};
}
}
var config = {
warnings: warnings,
longStackTraces: false,
cancellation: false,
monitoring: false,
asyncHooks: false
};
if (longStackTraces) Promise.longStackTraces();
return {
asyncHooks: function() {
return config.asyncHooks;
},
longStackTraces: function() {
return config.longStackTraces;
},
warnings: function() {
return config.warnings;
},
cancellation: function() {
return config.cancellation;
},
monitoring: function() {
return config.monitoring;
},
propagateFromFunction: function() {
return propagateFromFunction;
},
boundValueFunction: function() {
return boundValueFunction;
},
checkForgottenReturns: checkForgottenReturns,
setBounds: setBounds,
warn: warn,
deprecated: deprecated,
CapturedTrace: CapturedTrace,
fireDomEvent: fireDomEvent,
fireGlobalEvent: fireGlobalEvent
};
};
},{"./errors":12,"./es5":13,"./util":36}],10:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
function returner() {
return this.value;
}
function thrower() {
throw this.reason;
}
Promise.prototype["return"] =
Promise.prototype.thenReturn = function (value) {
if (value instanceof Promise) value.suppressUnhandledRejections();
return this._then(
returner, undefined, undefined, {value: value}, undefined);
};
Promise.prototype["throw"] =
Promise.prototype.thenThrow = function (reason) {
return this._then(
thrower, undefined, undefined, {reason: reason}, undefined);
};
Promise.prototype.catchThrow = function (reason) {
if (arguments.length <= 1) {
return this._then(
undefined, thrower, undefined, {reason: reason}, undefined);
} else {
var _reason = arguments[1];
var handler = function() {throw _reason;};
return this.caught(reason, handler);
}
};
Promise.prototype.catchReturn = function (value) {
if (arguments.length <= 1) {
if (value instanceof Promise) value.suppressUnhandledRejections();
return this._then(
undefined, returner, undefined, {value: value}, undefined);
} else {
var _value = arguments[1];
if (_value instanceof Promise) _value.suppressUnhandledRejections();
var handler = function() {return _value;};
return this.caught(value, handler);
}
};
};
},{}],11:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL) {
var PromiseReduce = Promise.reduce;
var PromiseAll = Promise.all;
function promiseAllThis() {
return PromiseAll(this);
}
function PromiseMapSeries(promises, fn) {
return PromiseReduce(promises, fn, INTERNAL, INTERNAL);
}
Promise.prototype.each = function (fn) {
return PromiseReduce(this, fn, INTERNAL, 0)
._then(promiseAllThis, undefined, undefined, this, undefined);
};
Promise.prototype.mapSeries = function (fn) {
return PromiseReduce(this, fn, INTERNAL, INTERNAL);
};
Promise.each = function (promises, fn) {
return PromiseReduce(promises, fn, INTERNAL, 0)
._then(promiseAllThis, undefined, undefined, promises, undefined);
};
Promise.mapSeries = PromiseMapSeries;
};
},{}],12:[function(_dereq_,module,exports){
"use strict";
var es5 = _dereq_("./es5");
var Objectfreeze = es5.freeze;
var util = _dereq_("./util");
var inherits = util.inherits;
var notEnumerableProp = util.notEnumerableProp;
function subError(nameProperty, defaultMessage) {
function SubError(message) {
if (!(this instanceof SubError)) return new SubError(message);
notEnumerableProp(this, "message",
typeof message === "string" ? message : defaultMessage);
notEnumerableProp(this, "name", nameProperty);
if (Error.captureStackTrace) {
Error.captureStackTrace(this, this.constructor);
} else {
Error.call(this);
}
}
inherits(SubError, Error);
return SubError;
}
var _TypeError, _RangeError;
var Warning = subError("Warning", "warning");
var CancellationError = subError("CancellationError", "cancellation error");
var TimeoutError = subError("TimeoutError", "timeout error");
var AggregateError = subError("AggregateError", "aggregate error");
try {
_TypeError = TypeError;
_RangeError = RangeError;
} catch(e) {
_TypeError = subError("TypeError", "type error");
_RangeError = subError("RangeError", "range error");
}
var methods = ("join pop push shift unshift slice filter forEach some " +
"every map indexOf lastIndexOf reduce reduceRight sort reverse").split(" ");
for (var i = 0; i < methods.length; ++i) {
if (typeof Array.prototype[methods[i]] === "function") {
AggregateError.prototype[methods[i]] = Array.prototype[methods[i]];
}
}
es5.defineProperty(AggregateError.prototype, "length", {
value: 0,
configurable: false,
writable: true,
enumerable: true
});
AggregateError.prototype["isOperational"] = true;
var level = 0;
AggregateError.prototype.toString = function() {
var indent = Array(level * 4 + 1).join(" ");
var ret = "\n" + indent + "AggregateError of:" + "\n";
level++;
indent = Array(level * 4 + 1).join(" ");
for (var i = 0; i < this.length; ++i) {
var str = this[i] === this ? "[Circular AggregateError]" : this[i] + "";
var lines = str.split("\n");
for (var j = 0; j < lines.length; ++j) {
lines[j] = indent + lines[j];
}
str = lines.join("\n");
ret += str + "\n";
}
level--;
return ret;
};
function OperationalError(message) {
if (!(this instanceof OperationalError))
return new OperationalError(message);
notEnumerableProp(this, "name", "OperationalError");
notEnumerableProp(this, "message", message);
this.cause = message;
this["isOperational"] = true;
if (message instanceof Error) {
notEnumerableProp(this, "message", message.message);
notEnumerableProp(this, "stack", message.stack);
} else if (Error.captureStackTrace) {
Error.captureStackTrace(this, this.constructor);
}
}
inherits(OperationalError, Error);
var errorTypes = Error["__BluebirdErrorTypes__"];
if (!errorTypes) {
errorTypes = Objectfreeze({
CancellationError: CancellationError,
TimeoutError: TimeoutError,
OperationalError: OperationalError,
RejectionError: OperationalError,
AggregateError: AggregateError
});
es5.defineProperty(Error, "__BluebirdErrorTypes__", {
value: errorTypes,
writable: false,
enumerable: false,
configurable: false
});
}
module.exports = {
Error: Error,
TypeError: _TypeError,
RangeError: _RangeError,
CancellationError: errorTypes.CancellationError,
OperationalError: errorTypes.OperationalError,
TimeoutError: errorTypes.TimeoutError,
AggregateError: errorTypes.AggregateError,
Warning: Warning
};
},{"./es5":13,"./util":36}],13:[function(_dereq_,module,exports){
var isES5 = (function(){
"use strict";
return this === undefined;
})();
if (isES5) {
module.exports = {
freeze: Object.freeze,
defineProperty: Object.defineProperty,
getDescriptor: Object.getOwnPropertyDescriptor,
keys: Object.keys,
names: Object.getOwnPropertyNames,
getPrototypeOf: Object.getPrototypeOf,
isArray: Array.isArray,
isES5: isES5,
propertyIsWritable: function(obj, prop) {
var descriptor = Object.getOwnPropertyDescriptor(obj, prop);
return !!(!descriptor || descriptor.writable || descriptor.set);
}
};
} else {
var has = {}.hasOwnProperty;
var str = {}.toString;
var proto = {}.constructor.prototype;
var ObjectKeys = function (o) {
var ret = [];
for (var key in o) {
if (has.call(o, key)) {
ret.push(key);
}
}
return ret;
};
var ObjectGetDescriptor = function(o, key) {
return {value: o[key]};
};
var ObjectDefineProperty = function (o, key, desc) {
o[key] = desc.value;
return o;
};
var ObjectFreeze = function (obj) {
return obj;
};
var ObjectGetPrototypeOf = function (obj) {
try {
return Object(obj).constructor.prototype;
}
catch (e) {
return proto;
}
};
var ArrayIsArray = function (obj) {
try {
return str.call(obj) === "[object Array]";
}
catch(e) {
return false;
}
};
module.exports = {
isArray: ArrayIsArray,
keys: ObjectKeys,
names: ObjectKeys,
defineProperty: ObjectDefineProperty,
getDescriptor: ObjectGetDescriptor,
freeze: ObjectFreeze,
getPrototypeOf: ObjectGetPrototypeOf,
isES5: isES5,
propertyIsWritable: function() {
return true;
}
};
}
},{}],14:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL) {
var PromiseMap = Promise.map;
Promise.prototype.filter = function (fn, options) {
return PromiseMap(this, fn, options, INTERNAL);
};
Promise.filter = function (promises, fn, options) {
return PromiseMap(promises, fn, options, INTERNAL);
};
};
},{}],15:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, tryConvertToPromise, NEXT_FILTER) {
var util = _dereq_("./util");
var CancellationError = Promise.CancellationError;
var errorObj = util.errorObj;
var catchFilter = _dereq_("./catch_filter")(NEXT_FILTER);
function PassThroughHandlerContext(promise, type, handler) {
this.promise = promise;
this.type = type;
this.handler = handler;
this.called = false;
this.cancelPromise = null;
}
PassThroughHandlerContext.prototype.isFinallyHandler = function() {
return this.type === 0;
};
function FinallyHandlerCancelReaction(finallyHandler) {
this.finallyHandler = finallyHandler;
}
FinallyHandlerCancelReaction.prototype._resultCancelled = function() {
checkCancel(this.finallyHandler);
};
function checkCancel(ctx, reason) {
if (ctx.cancelPromise != null) {
if (arguments.length > 1) {
ctx.cancelPromise._reject(reason);
} else {
ctx.cancelPromise._cancel();
}
ctx.cancelPromise = null;
return true;
}
return false;
}
function succeed() {
return finallyHandler.call(this, this.promise._target()._settledValue());
}
function fail(reason) {
if (checkCancel(this, reason)) return;
errorObj.e = reason;
return errorObj;
}
function finallyHandler(reasonOrValue) {
var promise = this.promise;
var handler = this.handler;
if (!this.called) {
this.called = true;
var ret = this.isFinallyHandler()
? handler.call(promise._boundValue())
: handler.call(promise._boundValue(), reasonOrValue);
if (ret === NEXT_FILTER) {
return ret;
} else if (ret !== undefined) {
promise._setReturnedNonUndefined();
var maybePromise = tryConvertToPromise(ret, promise);
if (maybePromise instanceof Promise) {
if (this.cancelPromise != null) {
if (maybePromise._isCancelled()) {
var reason =
new CancellationError("late cancellation observer");
promise._attachExtraTrace(reason);
errorObj.e = reason;
return errorObj;
} else if (maybePromise.isPending()) {
maybePromise._attachCancellationCallback(
new FinallyHandlerCancelReaction(this));
}
}
return maybePromise._then(
succeed, fail, undefined, this, undefined);
}
}
}
if (promise.isRejected()) {
checkCancel(this);
errorObj.e = reasonOrValue;
return errorObj;
} else {
checkCancel(this);
return reasonOrValue;
}
}
Promise.prototype._passThrough = function(handler, type, success, fail) {
if (typeof handler !== "function") return this.then();
return this._then(success,
fail,
undefined,
new PassThroughHandlerContext(this, type, handler),
undefined);
};
Promise.prototype.lastly =
Promise.prototype["finally"] = function (handler) {
return this._passThrough(handler,
0,
finallyHandler,
finallyHandler);
};
Promise.prototype.tap = function (handler) {
return this._passThrough(handler, 1, finallyHandler);
};
Promise.prototype.tapCatch = function (handlerOrPredicate) {
var len = arguments.length;
if(len === 1) {
return this._passThrough(handlerOrPredicate,
1,
undefined,
finallyHandler);
} else {
var catchInstances = new Array(len - 1),
j = 0, i;
for (i = 0; i < len - 1; ++i) {
var item = arguments[i];
if (util.isObject(item)) {
catchInstances[j++] = item;
} else {
return Promise.reject(new TypeError(
"tapCatch statement predicate: "
+ "expecting an object but got " + util.classString(item)
));
}
}
catchInstances.length = j;
var handler = arguments[i];
return this._passThrough(catchFilter(catchInstances, handler, this),
1,
undefined,
finallyHandler);
}
};
return PassThroughHandlerContext;
};
},{"./catch_filter":7,"./util":36}],16:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise,
apiRejection,
INTERNAL,
tryConvertToPromise,
Proxyable,
debug) {
var errors = _dereq_("./errors");
var TypeError = errors.TypeError;
var util = _dereq_("./util");
var errorObj = util.errorObj;
var tryCatch = util.tryCatch;
var yieldHandlers = [];
function promiseFromYieldHandler(value, yieldHandlers, traceParent) {
for (var i = 0; i < yieldHandlers.length; ++i) {
traceParent._pushContext();
var result = tryCatch(yieldHandlers[i])(value);
traceParent._popContext();
if (result === errorObj) {
traceParent._pushContext();
var ret = Promise.reject(errorObj.e);
traceParent._popContext();
return ret;
}
var maybePromise = tryConvertToPromise(result, traceParent);
if (maybePromise instanceof Promise) return maybePromise;
}
return null;
}
function PromiseSpawn(generatorFunction, receiver, yieldHandler, stack) {
if (debug.cancellation()) {
var internal = new Promise(INTERNAL);
var _finallyPromise = this._finallyPromise = new Promise(INTERNAL);
this._promise = internal.lastly(function() {
return _finallyPromise;
});
internal._captureStackTrace();
internal._setOnCancel(this);
} else {
var promise = this._promise = new Promise(INTERNAL);
promise._captureStackTrace();
}
this._stack = stack;
this._generatorFunction = generatorFunction;
this._receiver = receiver;
this._generator = undefined;
this._yieldHandlers = typeof yieldHandler === "function"
? [yieldHandler].concat(yieldHandlers)
: yieldHandlers;
this._yieldedPromise = null;
this._cancellationPhase = false;
}
util.inherits(PromiseSpawn, Proxyable);
PromiseSpawn.prototype._isResolved = function() {
return this._promise === null;
};
PromiseSpawn.prototype._cleanup = function() {
this._promise = this._generator = null;
if (debug.cancellation() && this._finallyPromise !== null) {
this._finallyPromise._fulfill();
this._finallyPromise = null;
}
};
PromiseSpawn.prototype._promiseCancelled = function() {
if (this._isResolved()) return;
var implementsReturn = typeof this._generator["return"] !== "undefined";
var result;
if (!implementsReturn) {
var reason = new Promise.CancellationError(
"generator .return() sentinel");
Promise.coroutine.returnSentinel = reason;
this._promise._attachExtraTrace(reason);
this._promise._pushContext();
result = tryCatch(this._generator["throw"]).call(this._generator,
reason);
this._promise._popContext();
} else {
this._promise._pushContext();
result = tryCatch(this._generator["return"]).call(this._generator,
undefined);
this._promise._popContext();
}
this._cancellationPhase = true;
this._yieldedPromise = null;
this._continue(result);
};
PromiseSpawn.prototype._promiseFulfilled = function(value) {
this._yieldedPromise = null;
this._promise._pushContext();
var result = tryCatch(this._generator.next).call(this._generator, value);
this._promise._popContext();
this._continue(result);
};
PromiseSpawn.prototype._promiseRejected = function(reason) {
this._yieldedPromise = null;
this._promise._attachExtraTrace(reason);
this._promise._pushContext();
var result = tryCatch(this._generator["throw"])
.call(this._generator, reason);
this._promise._popContext();
this._continue(result);
};
PromiseSpawn.prototype._resultCancelled = function() {
if (this._yieldedPromise instanceof Promise) {
var promise = this._yieldedPromise;
this._yieldedPromise = null;
promise.cancel();
}
};
PromiseSpawn.prototype.promise = function () {
return this._promise;
};
PromiseSpawn.prototype._run = function () {
this._generator = this._generatorFunction.call(this._receiver);
this._receiver =
this._generatorFunction = undefined;
this._promiseFulfilled(undefined);
};
PromiseSpawn.prototype._continue = function (result) {
var promise = this._promise;
if (result === errorObj) {
this._cleanup();
if (this._cancellationPhase) {
return promise.cancel();
} else {
return promise._rejectCallback(result.e, false);
}
}
var value = result.value;
if (result.done === true) {
this._cleanup();
if (this._cancellationPhase) {
return promise.cancel();
} else {
return promise._resolveCallback(value);
}
} else {
var maybePromise = tryConvertToPromise(value, this._promise);
if (!(maybePromise instanceof Promise)) {
maybePromise =
promiseFromYieldHandler(maybePromise,
this._yieldHandlers,
this._promise);
if (maybePromise === null) {
this._promiseRejected(
new TypeError(
"A value %s was yielded that could not be treated as a promise\u000a\u000a See http://goo.gl/MqrFmX\u000a\u000a".replace("%s", String(value)) +
"From coroutine:\u000a" +
this._stack.split("\n").slice(1, -7).join("\n")
)
);
return;
}
}
maybePromise = maybePromise._target();
var bitField = maybePromise._bitField;
;
if (((bitField & 50397184) === 0)) {
this._yieldedPromise = maybePromise;
maybePromise._proxy(this, null);
} else if (((bitField & 33554432) !== 0)) {
Promise._async.invoke(
this._promiseFulfilled, this, maybePromise._value()
);
} else if (((bitField & 16777216) !== 0)) {
Promise._async.invoke(
this._promiseRejected, this, maybePromise._reason()
);
} else {
this._promiseCancelled();
}
}
};
Promise.coroutine = function (generatorFunction, options) {
if (typeof generatorFunction !== "function") {
throw new TypeError("generatorFunction must be a function\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
var yieldHandler = Object(options).yieldHandler;
var PromiseSpawn$ = PromiseSpawn;
var stack = new Error().stack;
return function () {
var generator = generatorFunction.apply(this, arguments);
var spawn = new PromiseSpawn$(undefined, undefined, yieldHandler,
stack);
var ret = spawn.promise();
spawn._generator = generator;
spawn._promiseFulfilled(undefined);
return ret;
};
};
Promise.coroutine.addYieldHandler = function(fn) {
if (typeof fn !== "function") {
throw new TypeError("expecting a function but got " + util.classString(fn));
}
yieldHandlers.push(fn);
};
Promise.spawn = function (generatorFunction) {
debug.deprecated("Promise.spawn()", "Promise.coroutine()");
if (typeof generatorFunction !== "function") {
return apiRejection("generatorFunction must be a function\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
var spawn = new PromiseSpawn(generatorFunction, this);
var ret = spawn.promise();
spawn._run(Promise.spawn);
return ret;
};
};
},{"./errors":12,"./util":36}],17:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, PromiseArray, tryConvertToPromise, INTERNAL, async) {
var util = _dereq_("./util");
var canEvaluate = util.canEvaluate;
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
var reject;
if (!true) {
if (canEvaluate) {
var thenCallback = function(i) {
return new Function("value", "holder", " \n\
'use strict'; \n\
holder.pIndex = value; \n\
holder.checkFulfillment(this); \n\
".replace(/Index/g, i));
};
var promiseSetter = function(i) {
return new Function("promise", "holder", " \n\
'use strict'; \n\
holder.pIndex = promise; \n\
".replace(/Index/g, i));
};
var generateHolderClass = function(total) {
var props = new Array(total);
for (var i = 0; i < props.length; ++i) {
props[i] = "this.p" + (i+1);
}
var assignment = props.join(" = ") + " = null;";
var cancellationCode= "var promise;\n" + props.map(function(prop) {
return " \n\
promise = " + prop + "; \n\
if (promise instanceof Promise) { \n\
promise.cancel(); \n\
} \n\
";
}).join("\n");
var passedArguments = props.join(", ");
var name = "Holder$" + total;
var code = "return function(tryCatch, errorObj, Promise, async) { \n\
'use strict'; \n\
function [TheName](fn) { \n\
[TheProperties] \n\
this.fn = fn; \n\
this.asyncNeeded = true; \n\
this.now = 0; \n\
} \n\
\n\
[TheName].prototype._callFunction = function(promise) { \n\
promise._pushContext(); \n\
var ret = tryCatch(this.fn)([ThePassedArguments]); \n\
promise._popContext(); \n\
if (ret === errorObj) { \n\
promise._rejectCallback(ret.e, false); \n\
} else { \n\
promise._resolveCallback(ret); \n\
} \n\
}; \n\
\n\
[TheName].prototype.checkFulfillment = function(promise) { \n\
var now = ++this.now; \n\
if (now === [TheTotal]) { \n\
if (this.asyncNeeded) { \n\
async.invoke(this._callFunction, this, promise); \n\
} else { \n\
this._callFunction(promise); \n\
} \n\
\n\
} \n\
}; \n\
\n\
[TheName].prototype._resultCancelled = function() { \n\
[CancellationCode] \n\
}; \n\
\n\
return [TheName]; \n\
}(tryCatch, errorObj, Promise, async); \n\
";
code = code.replace(/\[TheName\]/g, name)
.replace(/\[TheTotal\]/g, total)
.replace(/\[ThePassedArguments\]/g, passedArguments)
.replace(/\[TheProperties\]/g, assignment)
.replace(/\[CancellationCode\]/g, cancellationCode);
return new Function("tryCatch", "errorObj", "Promise", "async", code)
(tryCatch, errorObj, Promise, async);
};
var holderClasses = [];
var thenCallbacks = [];
var promiseSetters = [];
for (var i = 0; i < 8; ++i) {
holderClasses.push(generateHolderClass(i + 1));
thenCallbacks.push(thenCallback(i + 1));
promiseSetters.push(promiseSetter(i + 1));
}
reject = function (reason) {
this._reject(reason);
};
}}
Promise.join = function () {
var last = arguments.length - 1;
var fn;
if (last > 0 && typeof arguments[last] === "function") {
fn = arguments[last];
if (!true) {
if (last <= 8 && canEvaluate) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
var HolderClass = holderClasses[last - 1];
var holder = new HolderClass(fn);
var callbacks = thenCallbacks;
for (var i = 0; i < last; ++i) {
var maybePromise = tryConvertToPromise(arguments[i], ret);
if (maybePromise instanceof Promise) {
maybePromise = maybePromise._target();
var bitField = maybePromise._bitField;
;
if (((bitField & 50397184) === 0)) {
maybePromise._then(callbacks[i], reject,
undefined, ret, holder);
promiseSetters[i](maybePromise, holder);
holder.asyncNeeded = false;
} else if (((bitField & 33554432) !== 0)) {
callbacks[i].call(ret,
maybePromise._value(), holder);
} else if (((bitField & 16777216) !== 0)) {
ret._reject(maybePromise._reason());
} else {
ret._cancel();
}
} else {
callbacks[i].call(ret, maybePromise, holder);
}
}
if (!ret._isFateSealed()) {
if (holder.asyncNeeded) {
var context = Promise._getContext();
holder.fn = util.contextBind(context, holder.fn);
}
ret._setAsyncGuaranteed();
ret._setOnCancel(holder);
}
return ret;
}
}
}
var args = [].slice.call(arguments);;
if (fn) args.pop();
var ret = new PromiseArray(args).promise();
return fn !== undefined ? ret.spread(fn) : ret;
};
};
},{"./util":36}],18:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise,
PromiseArray,
apiRejection,
tryConvertToPromise,
INTERNAL,
debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
var async = Promise._async;
function MappingPromiseArray(promises, fn, limit, _filter) {
this.constructor$(promises);
this._promise._captureStackTrace();
var context = Promise._getContext();
this._callback = util.contextBind(context, fn);
this._preservedValues = _filter === INTERNAL
? new Array(this.length())
: null;
this._limit = limit;
this._inFlight = 0;
this._queue = [];
async.invoke(this._asyncInit, this, undefined);
if (util.isArray(promises)) {
for (var i = 0; i < promises.length; ++i) {
var maybePromise = promises[i];
if (maybePromise instanceof Promise) {
maybePromise.suppressUnhandledRejections();
}
}
}
}
util.inherits(MappingPromiseArray, PromiseArray);
MappingPromiseArray.prototype._asyncInit = function() {
this._init$(undefined, -2);
};
MappingPromiseArray.prototype._init = function () {};
MappingPromiseArray.prototype._promiseFulfilled = function (value, index) {
var values = this._values;
var length = this.length();
var preservedValues = this._preservedValues;
var limit = this._limit;
if (index < 0) {
index = (index * -1) - 1;
values[index] = value;
if (limit >= 1) {
this._inFlight--;
this._drainQueue();
if (this._isResolved()) return true;
}
} else {
if (limit >= 1 && this._inFlight >= limit) {
values[index] = value;
this._queue.push(index);
return false;
}
if (preservedValues !== null) preservedValues[index] = value;
var promise = this._promise;
var callback = this._callback;
var receiver = promise._boundValue();
promise._pushContext();
var ret = tryCatch(callback).call(receiver, value, index, length);
var promiseCreated = promise._popContext();
debug.checkForgottenReturns(
ret,
promiseCreated,
preservedValues !== null ? "Promise.filter" : "Promise.map",
promise
);
if (ret === errorObj) {
this._reject(ret.e);
return true;
}
var maybePromise = tryConvertToPromise(ret, this._promise);
if (maybePromise instanceof Promise) {
maybePromise = maybePromise._target();
var bitField = maybePromise._bitField;
;
if (((bitField & 50397184) === 0)) {
if (limit >= 1) this._inFlight++;
values[index] = maybePromise;
maybePromise._proxy(this, (index + 1) * -1);
return false;
} else if (((bitField & 33554432) !== 0)) {
ret = maybePromise._value();
} else if (((bitField & 16777216) !== 0)) {
this._reject(maybePromise._reason());
return true;
} else {
this._cancel();
return true;
}
}
values[index] = ret;
}
var totalResolved = ++this._totalResolved;
if (totalResolved >= length) {
if (preservedValues !== null) {
this._filter(values, preservedValues);
} else {
this._resolve(values);
}
return true;
}
return false;
};
MappingPromiseArray.prototype._drainQueue = function () {
var queue = this._queue;
var limit = this._limit;
var values = this._values;
while (queue.length > 0 && this._inFlight < limit) {
if (this._isResolved()) return;
var index = queue.pop();
this._promiseFulfilled(values[index], index);
}
};
MappingPromiseArray.prototype._filter = function (booleans, values) {
var len = values.length;
var ret = new Array(len);
var j = 0;
for (var i = 0; i < len; ++i) {
if (booleans[i]) ret[j++] = values[i];
}
ret.length = j;
this._resolve(ret);
};
MappingPromiseArray.prototype.preservedValues = function () {
return this._preservedValues;
};
function map(promises, fn, options, _filter) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
var limit = 0;
if (options !== undefined) {
if (typeof options === "object" && options !== null) {
if (typeof options.concurrency !== "number") {
return Promise.reject(
new TypeError("'concurrency' must be a number but it is " +
util.classString(options.concurrency)));
}
limit = options.concurrency;
} else {
return Promise.reject(new TypeError(
"options argument must be an object but it is " +
util.classString(options)));
}
}
limit = typeof limit === "number" &&
isFinite(limit) && limit >= 1 ? limit : 0;
return new MappingPromiseArray(promises, fn, limit, _filter).promise();
}
Promise.prototype.map = function (fn, options) {
return map(this, fn, options, null);
};
Promise.map = function (promises, fn, options, _filter) {
return map(promises, fn, options, _filter);
};
};
},{"./util":36}],19:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, INTERNAL, tryConvertToPromise, apiRejection, debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
Promise.method = function (fn) {
if (typeof fn !== "function") {
throw new Promise.TypeError("expecting a function but got " + util.classString(fn));
}
return function () {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._pushContext();
var value = tryCatch(fn).apply(this, arguments);
var promiseCreated = ret._popContext();
debug.checkForgottenReturns(
value, promiseCreated, "Promise.method", ret);
ret._resolveFromSyncValue(value);
return ret;
};
};
Promise.attempt = Promise["try"] = function (fn) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._pushContext();
var value;
if (arguments.length > 1) {
debug.deprecated("calling Promise.try with more than 1 argument");
var arg = arguments[1];
var ctx = arguments[2];
value = util.isArray(arg) ? tryCatch(fn).apply(ctx, arg)
: tryCatch(fn).call(ctx, arg);
} else {
value = tryCatch(fn)();
}
var promiseCreated = ret._popContext();
debug.checkForgottenReturns(
value, promiseCreated, "Promise.try", ret);
ret._resolveFromSyncValue(value);
return ret;
};
Promise.prototype._resolveFromSyncValue = function (value) {
if (value === util.errorObj) {
this._rejectCallback(value.e, false);
} else {
this._resolveCallback(value, true);
}
};
};
},{"./util":36}],20:[function(_dereq_,module,exports){
"use strict";
var util = _dereq_("./util");
var maybeWrapAsError = util.maybeWrapAsError;
var errors = _dereq_("./errors");
var OperationalError = errors.OperationalError;
var es5 = _dereq_("./es5");
function isUntypedError(obj) {
return obj instanceof Error &&
es5.getPrototypeOf(obj) === Error.prototype;
}
var rErrorKey = /^(?:name|message|stack|cause)$/;
function wrapAsOperationalError(obj) {
var ret;
if (isUntypedError(obj)) {
ret = new OperationalError(obj);
ret.name = obj.name;
ret.message = obj.message;
ret.stack = obj.stack;
var keys = es5.keys(obj);
for (var i = 0; i < keys.length; ++i) {
var key = keys[i];
if (!rErrorKey.test(key)) {
ret[key] = obj[key];
}
}
return ret;
}
util.markAsOriginatingFromRejection(obj);
return obj;
}
function nodebackForPromise(promise, multiArgs) {
return function(err, value) {
if (promise === null) return;
if (err) {
var wrapped = wrapAsOperationalError(maybeWrapAsError(err));
promise._attachExtraTrace(wrapped);
promise._reject(wrapped);
} else if (!multiArgs) {
promise._fulfill(value);
} else {
var args = [].slice.call(arguments, 1);;
promise._fulfill(args);
}
promise = null;
};
}
module.exports = nodebackForPromise;
},{"./errors":12,"./es5":13,"./util":36}],21:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
var util = _dereq_("./util");
var async = Promise._async;
var tryCatch = util.tryCatch;
var errorObj = util.errorObj;
function spreadAdapter(val, nodeback) {
var promise = this;
if (!util.isArray(val)) return successAdapter.call(promise, val, nodeback);
var ret =
tryCatch(nodeback).apply(promise._boundValue(), [null].concat(val));
if (ret === errorObj) {
async.throwLater(ret.e);
}
}
function successAdapter(val, nodeback) {
var promise = this;
var receiver = promise._boundValue();
var ret = val === undefined
? tryCatch(nodeback).call(receiver, null)
: tryCatch(nodeback).call(receiver, null, val);
if (ret === errorObj) {
async.throwLater(ret.e);
}
}
function errorAdapter(reason, nodeback) {
var promise = this;
if (!reason) {
var newReason = new Error(reason + "");
newReason.cause = reason;
reason = newReason;
}
var ret = tryCatch(nodeback).call(promise._boundValue(), reason);
if (ret === errorObj) {
async.throwLater(ret.e);
}
}
Promise.prototype.asCallback = Promise.prototype.nodeify = function (nodeback,
options) {
if (typeof nodeback == "function") {
var adapter = successAdapter;
if (options !== undefined && Object(options).spread) {
adapter = spreadAdapter;
}
this._then(
adapter,
errorAdapter,
undefined,
this,
nodeback
);
}
return this;
};
};
},{"./util":36}],22:[function(_dereq_,module,exports){
"use strict";
module.exports = function() {
var makeSelfResolutionError = function () {
return new TypeError("circular promise resolution chain\u000a\u000a See http://goo.gl/MqrFmX\u000a");
};
var reflectHandler = function() {
return new Promise.PromiseInspection(this._target());
};
var apiRejection = function(msg) {
return Promise.reject(new TypeError(msg));
};
function Proxyable() {}
var UNDEFINED_BINDING = {};
var util = _dereq_("./util");
util.setReflectHandler(reflectHandler);
var getDomain = function() {
var domain = process.domain;
if (domain === undefined) {
return null;
}
return domain;
};
var getContextDefault = function() {
return null;
};
var getContextDomain = function() {
return {
domain: getDomain(),
async: null
};
};
var AsyncResource = util.isNode && util.nodeSupportsAsyncResource ?
_dereq_("async_hooks").AsyncResource : null;
var getContextAsyncHooks = function() {
return {
domain: getDomain(),
async: new AsyncResource("Bluebird::Promise")
};
};
var getContext = util.isNode ? getContextDomain : getContextDefault;
util.notEnumerableProp(Promise, "_getContext", getContext);
var enableAsyncHooks = function() {
getContext = getContextAsyncHooks;
util.notEnumerableProp(Promise, "_getContext", getContextAsyncHooks);
};
var disableAsyncHooks = function() {
getContext = getContextDomain;
util.notEnumerableProp(Promise, "_getContext", getContextDomain);
};
var es5 = _dereq_("./es5");
var Async = _dereq_("./async");
var async = new Async();
es5.defineProperty(Promise, "_async", {value: async});
var errors = _dereq_("./errors");
var TypeError = Promise.TypeError = errors.TypeError;
Promise.RangeError = errors.RangeError;
var CancellationError = Promise.CancellationError = errors.CancellationError;
Promise.TimeoutError = errors.TimeoutError;
Promise.OperationalError = errors.OperationalError;
Promise.RejectionError = errors.OperationalError;
Promise.AggregateError = errors.AggregateError;
var INTERNAL = function(){};
var APPLY = {};
var NEXT_FILTER = {};
var tryConvertToPromise = _dereq_("./thenables")(Promise, INTERNAL);
var PromiseArray =
_dereq_("./promise_array")(Promise, INTERNAL,
tryConvertToPromise, apiRejection, Proxyable);
var Context = _dereq_("./context")(Promise);
/*jshint unused:false*/
var createContext = Context.create;
var debug = _dereq_("./debuggability")(Promise, Context,
enableAsyncHooks, disableAsyncHooks);
var CapturedTrace = debug.CapturedTrace;
var PassThroughHandlerContext =
_dereq_("./finally")(Promise, tryConvertToPromise, NEXT_FILTER);
var catchFilter = _dereq_("./catch_filter")(NEXT_FILTER);
var nodebackForPromise = _dereq_("./nodeback");
var errorObj = util.errorObj;
var tryCatch = util.tryCatch;
function check(self, executor) {
if (self == null || self.constructor !== Promise) {
throw new TypeError("the promise constructor cannot be invoked directly\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
if (typeof executor !== "function") {
throw new TypeError("expecting a function but got " + util.classString(executor));
}
}
function Promise(executor) {
if (executor !== INTERNAL) {
check(this, executor);
}
this._bitField = 0;
this._fulfillmentHandler0 = undefined;
this._rejectionHandler0 = undefined;
this._promise0 = undefined;
this._receiver0 = undefined;
this._resolveFromExecutor(executor);
this._promiseCreated();
this._fireEvent("promiseCreated", this);
}
Promise.prototype.toString = function () {
return "[object Promise]";
};
Promise.prototype.caught = Promise.prototype["catch"] = function (fn) {
var len = arguments.length;
if (len > 1) {
var catchInstances = new Array(len - 1),
j = 0, i;
for (i = 0; i < len - 1; ++i) {
var item = arguments[i];
if (util.isObject(item)) {
catchInstances[j++] = item;
} else {
return apiRejection("Catch statement predicate: " +
"expecting an object but got " + util.classString(item));
}
}
catchInstances.length = j;
fn = arguments[i];
if (typeof fn !== "function") {
throw new TypeError("The last argument to .catch() " +
"must be a function, got " + util.toString(fn));
}
return this.then(undefined, catchFilter(catchInstances, fn, this));
}
return this.then(undefined, fn);
};
Promise.prototype.reflect = function () {
return this._then(reflectHandler,
reflectHandler, undefined, this, undefined);
};
Promise.prototype.then = function (didFulfill, didReject) {
if (debug.warnings() && arguments.length > 0 &&
typeof didFulfill !== "function" &&
typeof didReject !== "function") {
var msg = ".then() only accepts functions but was passed: " +
util.classString(didFulfill);
if (arguments.length > 1) {
msg += ", " + util.classString(didReject);
}
this._warn(msg);
}
return this._then(didFulfill, didReject, undefined, undefined, undefined);
};
Promise.prototype.done = function (didFulfill, didReject) {
var promise =
this._then(didFulfill, didReject, undefined, undefined, undefined);
promise._setIsFinal();
};
Promise.prototype.spread = function (fn) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
return this.all()._then(fn, undefined, undefined, APPLY, undefined);
};
Promise.prototype.toJSON = function () {
var ret = {
isFulfilled: false,
isRejected: false,
fulfillmentValue: undefined,
rejectionReason: undefined
};
if (this.isFulfilled()) {
ret.fulfillmentValue = this.value();
ret.isFulfilled = true;
} else if (this.isRejected()) {
ret.rejectionReason = this.reason();
ret.isRejected = true;
}
return ret;
};
Promise.prototype.all = function () {
if (arguments.length > 0) {
this._warn(".all() was passed arguments but it does not take any");
}
return new PromiseArray(this).promise();
};
Promise.prototype.error = function (fn) {
return this.caught(util.originatesFromRejection, fn);
};
Promise.getNewLibraryCopy = module.exports;
Promise.is = function (val) {
return val instanceof Promise;
};
Promise.fromNode = Promise.fromCallback = function(fn) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
var multiArgs = arguments.length > 1 ? !!Object(arguments[1]).multiArgs
: false;
var result = tryCatch(fn)(nodebackForPromise(ret, multiArgs));
if (result === errorObj) {
ret._rejectCallback(result.e, true);
}
if (!ret._isFateSealed()) ret._setAsyncGuaranteed();
return ret;
};
Promise.all = function (promises) {
return new PromiseArray(promises).promise();
};
Promise.cast = function (obj) {
var ret = tryConvertToPromise(obj);
if (!(ret instanceof Promise)) {
ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._setFulfilled();
ret._rejectionHandler0 = obj;
}
return ret;
};
Promise.resolve = Promise.fulfilled = Promise.cast;
Promise.reject = Promise.rejected = function (reason) {
var ret = new Promise(INTERNAL);
ret._captureStackTrace();
ret._rejectCallback(reason, true);
return ret;
};
Promise.setScheduler = function(fn) {
if (typeof fn !== "function") {
throw new TypeError("expecting a function but got " + util.classString(fn));
}
return async.setScheduler(fn);
};
Promise.prototype._then = function (
didFulfill,
didReject,
_, receiver,
internalData
) {
var haveInternalData = internalData !== undefined;
var promise = haveInternalData ? internalData : new Promise(INTERNAL);
var target = this._target();
var bitField = target._bitField;
if (!haveInternalData) {
promise._propagateFrom(this, 3);
promise._captureStackTrace();
if (receiver === undefined &&
((this._bitField & 2097152) !== 0)) {
if (!((bitField & 50397184) === 0)) {
receiver = this._boundValue();
} else {
receiver = target === this ? undefined : this._boundTo;
}
}
this._fireEvent("promiseChained", this, promise);
}
var context = getContext();
if (!((bitField & 50397184) === 0)) {
var handler, value, settler = target._settlePromiseCtx;
if (((bitField & 33554432) !== 0)) {
value = target._rejectionHandler0;
handler = didFulfill;
} else if (((bitField & 16777216) !== 0)) {
value = target._fulfillmentHandler0;
handler = didReject;
target._unsetRejectionIsUnhandled();
} else {
settler = target._settlePromiseLateCancellationObserver;
value = new CancellationError("late cancellation observer");
target._attachExtraTrace(value);
handler = didReject;
}
async.invoke(settler, target, {
handler: util.contextBind(context, handler),
promise: promise,
receiver: receiver,
value: value
});
} else {
target._addCallbacks(didFulfill, didReject, promise,
receiver, context);
}
return promise;
};
Promise.prototype._length = function () {
return this._bitField & 65535;
};
Promise.prototype._isFateSealed = function () {
return (this._bitField & 117506048) !== 0;
};
Promise.prototype._isFollowing = function () {
return (this._bitField & 67108864) === 67108864;
};
Promise.prototype._setLength = function (len) {
this._bitField = (this._bitField & -65536) |
(len & 65535);
};
Promise.prototype._setFulfilled = function () {
this._bitField = this._bitField | 33554432;
this._fireEvent("promiseFulfilled", this);
};
Promise.prototype._setRejected = function () {
this._bitField = this._bitField | 16777216;
this._fireEvent("promiseRejected", this);
};
Promise.prototype._setFollowing = function () {
this._bitField = this._bitField | 67108864;
this._fireEvent("promiseResolved", this);
};
Promise.prototype._setIsFinal = function () {
this._bitField = this._bitField | 4194304;
};
Promise.prototype._isFinal = function () {
return (this._bitField & 4194304) > 0;
};
Promise.prototype._unsetCancelled = function() {
this._bitField = this._bitField & (~65536);
};
Promise.prototype._setCancelled = function() {
this._bitField = this._bitField | 65536;
this._fireEvent("promiseCancelled", this);
};
Promise.prototype._setWillBeCancelled = function() {
this._bitField = this._bitField | 8388608;
};
Promise.prototype._setAsyncGuaranteed = function() {
if (async.hasCustomScheduler()) return;
var bitField = this._bitField;
this._bitField = bitField |
(((bitField & 536870912) >> 2) ^
134217728);
};
Promise.prototype._setNoAsyncGuarantee = function() {
this._bitField = (this._bitField | 536870912) &
(~134217728);
};
Promise.prototype._receiverAt = function (index) {
var ret = index === 0 ? this._receiver0 : this[
index * 4 - 4 + 3];
if (ret === UNDEFINED_BINDING) {
return undefined;
} else if (ret === undefined && this._isBound()) {
return this._boundValue();
}
return ret;
};
Promise.prototype._promiseAt = function (index) {
return this[
index * 4 - 4 + 2];
};
Promise.prototype._fulfillmentHandlerAt = function (index) {
return this[
index * 4 - 4 + 0];
};
Promise.prototype._rejectionHandlerAt = function (index) {
return this[
index * 4 - 4 + 1];
};
Promise.prototype._boundValue = function() {};
Promise.prototype._migrateCallback0 = function (follower) {
var bitField = follower._bitField;
var fulfill = follower._fulfillmentHandler0;
var reject = follower._rejectionHandler0;
var promise = follower._promise0;
var receiver = follower._receiverAt(0);
if (receiver === undefined) receiver = UNDEFINED_BINDING;
this._addCallbacks(fulfill, reject, promise, receiver, null);
};
Promise.prototype._migrateCallbackAt = function (follower, index) {
var fulfill = follower._fulfillmentHandlerAt(index);
var reject = follower._rejectionHandlerAt(index);
var promise = follower._promiseAt(index);
var receiver = follower._receiverAt(index);
if (receiver === undefined) receiver = UNDEFINED_BINDING;
this._addCallbacks(fulfill, reject, promise, receiver, null);
};
Promise.prototype._addCallbacks = function (
fulfill,
reject,
promise,
receiver,
context
) {
var index = this._length();
if (index >= 65535 - 4) {
index = 0;
this._setLength(0);
}
if (index === 0) {
this._promise0 = promise;
this._receiver0 = receiver;
if (typeof fulfill === "function") {
this._fulfillmentHandler0 = util.contextBind(context, fulfill);
}
if (typeof reject === "function") {
this._rejectionHandler0 = util.contextBind(context, reject);
}
} else {
var base = index * 4 - 4;
this[base + 2] = promise;
this[base + 3] = receiver;
if (typeof fulfill === "function") {
this[base + 0] =
util.contextBind(context, fulfill);
}
if (typeof reject === "function") {
this[base + 1] =
util.contextBind(context, reject);
}
}
this._setLength(index + 1);
return index;
};
Promise.prototype._proxy = function (proxyable, arg) {
this._addCallbacks(undefined, undefined, arg, proxyable, null);
};
Promise.prototype._resolveCallback = function(value, shouldBind) {
if (((this._bitField & 117506048) !== 0)) return;
if (value === this)
return this._rejectCallback(makeSelfResolutionError(), false);
var maybePromise = tryConvertToPromise(value, this);
if (!(maybePromise instanceof Promise)) return this._fulfill(value);
if (shouldBind) this._propagateFrom(maybePromise, 2);
var promise = maybePromise._target();
if (promise === this) {
this._reject(makeSelfResolutionError());
return;
}
var bitField = promise._bitField;
if (((bitField & 50397184) === 0)) {
var len = this._length();
if (len > 0) promise._migrateCallback0(this);
for (var i = 1; i < len; ++i) {
promise._migrateCallbackAt(this, i);
}
this._setFollowing();
this._setLength(0);
this._setFollowee(maybePromise);
} else if (((bitField & 33554432) !== 0)) {
this._fulfill(promise._value());
} else if (((bitField & 16777216) !== 0)) {
this._reject(promise._reason());
} else {
var reason = new CancellationError("late cancellation observer");
promise._attachExtraTrace(reason);
this._reject(reason);
}
};
Promise.prototype._rejectCallback =
function(reason, synchronous, ignoreNonErrorWarnings) {
var trace = util.ensureErrorObject(reason);
var hasStack = trace === reason;
if (!hasStack && !ignoreNonErrorWarnings && debug.warnings()) {
var message = "a promise was rejected with a non-error: " +
util.classString(reason);
this._warn(message, true);
}
this._attachExtraTrace(trace, synchronous ? hasStack : false);
this._reject(reason);
};
Promise.prototype._resolveFromExecutor = function (executor) {
if (executor === INTERNAL) return;
var promise = this;
this._captureStackTrace();
this._pushContext();
var synchronous = true;
var r = this._execute(executor, function(value) {
promise._resolveCallback(value);
}, function (reason) {
promise._rejectCallback(reason, synchronous);
});
synchronous = false;
this._popContext();
if (r !== undefined) {
promise._rejectCallback(r, true);
}
};
Promise.prototype._settlePromiseFromHandler = function (
handler, receiver, value, promise
) {
var bitField = promise._bitField;
if (((bitField & 65536) !== 0)) return;
promise._pushContext();
var x;
if (receiver === APPLY) {
if (!value || typeof value.length !== "number") {
x = errorObj;
x.e = new TypeError("cannot .spread() a non-array: " +
util.classString(value));
} else {
x = tryCatch(handler).apply(this._boundValue(), value);
}
} else {
x = tryCatch(handler).call(receiver, value);
}
var promiseCreated = promise._popContext();
bitField = promise._bitField;
if (((bitField & 65536) !== 0)) return;
if (x === NEXT_FILTER) {
promise._reject(value);
} else if (x === errorObj) {
promise._rejectCallback(x.e, false);
} else {
debug.checkForgottenReturns(x, promiseCreated, "", promise, this);
promise._resolveCallback(x);
}
};
Promise.prototype._target = function() {
var ret = this;
while (ret._isFollowing()) ret = ret._followee();
return ret;
};
Promise.prototype._followee = function() {
return this._rejectionHandler0;
};
Promise.prototype._setFollowee = function(promise) {
this._rejectionHandler0 = promise;
};
Promise.prototype._settlePromise = function(promise, handler, receiver, value) {
var isPromise = promise instanceof Promise;
var bitField = this._bitField;
var asyncGuaranteed = ((bitField & 134217728) !== 0);
if (((bitField & 65536) !== 0)) {
if (isPromise) promise._invokeInternalOnCancel();
if (receiver instanceof PassThroughHandlerContext &&
receiver.isFinallyHandler()) {
receiver.cancelPromise = promise;
if (tryCatch(handler).call(receiver, value) === errorObj) {
promise._reject(errorObj.e);
}
} else if (handler === reflectHandler) {
promise._fulfill(reflectHandler.call(receiver));
} else if (receiver instanceof Proxyable) {
receiver._promiseCancelled(promise);
} else if (isPromise || promise instanceof PromiseArray) {
promise._cancel();
} else {
receiver.cancel();
}
} else if (typeof handler === "function") {
if (!isPromise) {
handler.call(receiver, value, promise);
} else {
if (asyncGuaranteed) promise._setAsyncGuaranteed();
this._settlePromiseFromHandler(handler, receiver, value, promise);
}
} else if (receiver instanceof Proxyable) {
if (!receiver._isResolved()) {
if (((bitField & 33554432) !== 0)) {
receiver._promiseFulfilled(value, promise);
} else {
receiver._promiseRejected(value, promise);
}
}
} else if (isPromise) {
if (asyncGuaranteed) promise._setAsyncGuaranteed();
if (((bitField & 33554432) !== 0)) {
promise._fulfill(value);
} else {
promise._reject(value);
}
}
};
Promise.prototype._settlePromiseLateCancellationObserver = function(ctx) {
var handler = ctx.handler;
var promise = ctx.promise;
var receiver = ctx.receiver;
var value = ctx.value;
if (typeof handler === "function") {
if (!(promise instanceof Promise)) {
handler.call(receiver, value, promise);
} else {
this._settlePromiseFromHandler(handler, receiver, value, promise);
}
} else if (promise instanceof Promise) {
promise._reject(value);
}
};
Promise.prototype._settlePromiseCtx = function(ctx) {
this._settlePromise(ctx.promise, ctx.handler, ctx.receiver, ctx.value);
};
Promise.prototype._settlePromise0 = function(handler, value, bitField) {
var promise = this._promise0;
var receiver = this._receiverAt(0);
this._promise0 = undefined;
this._receiver0 = undefined;
this._settlePromise(promise, handler, receiver, value);
};
Promise.prototype._clearCallbackDataAtIndex = function(index) {
var base = index * 4 - 4;
this[base + 2] =
this[base + 3] =
this[base + 0] =
this[base + 1] = undefined;
};
Promise.prototype._fulfill = function (value) {
var bitField = this._bitField;
if (((bitField & 117506048) >>> 16)) return;
if (value === this) {
var err = makeSelfResolutionError();
this._attachExtraTrace(err);
return this._reject(err);
}
this._setFulfilled();
this._rejectionHandler0 = value;
if ((bitField & 65535) > 0) {
if (((bitField & 134217728) !== 0)) {
this._settlePromises();
} else {
async.settlePromises(this);
}
this._dereferenceTrace();
}
};
Promise.prototype._reject = function (reason) {
var bitField = this._bitField;
if (((bitField & 117506048) >>> 16)) return;
this._setRejected();
this._fulfillmentHandler0 = reason;
if (this._isFinal()) {
return async.fatalError(reason, util.isNode);
}
if ((bitField & 65535) > 0) {
async.settlePromises(this);
} else {
this._ensurePossibleRejectionHandled();
}
};
Promise.prototype._fulfillPromises = function (len, value) {
for (var i = 1; i < len; i++) {
var handler = this._fulfillmentHandlerAt(i);
var promise = this._promiseAt(i);
var receiver = this._receiverAt(i);
this._clearCallbackDataAtIndex(i);
this._settlePromise(promise, handler, receiver, value);
}
};
Promise.prototype._rejectPromises = function (len, reason) {
for (var i = 1; i < len; i++) {
var handler = this._rejectionHandlerAt(i);
var promise = this._promiseAt(i);
var receiver = this._receiverAt(i);
this._clearCallbackDataAtIndex(i);
this._settlePromise(promise, handler, receiver, reason);
}
};
Promise.prototype._settlePromises = function () {
var bitField = this._bitField;
var len = (bitField & 65535);
if (len > 0) {
if (((bitField & 16842752) !== 0)) {
var reason = this._fulfillmentHandler0;
this._settlePromise0(this._rejectionHandler0, reason, bitField);
this._rejectPromises(len, reason);
} else {
var value = this._rejectionHandler0;
this._settlePromise0(this._fulfillmentHandler0, value, bitField);
this._fulfillPromises(len, value);
}
this._setLength(0);
}
this._clearCancellationData();
};
Promise.prototype._settledValue = function() {
var bitField = this._bitField;
if (((bitField & 33554432) !== 0)) {
return this._rejectionHandler0;
} else if (((bitField & 16777216) !== 0)) {
return this._fulfillmentHandler0;
}
};
if (typeof Symbol !== "undefined" && Symbol.toStringTag) {
es5.defineProperty(Promise.prototype, Symbol.toStringTag, {
get: function () {
return "Object";
}
});
}
function deferResolve(v) {this.promise._resolveCallback(v);}
function deferReject(v) {this.promise._rejectCallback(v, false);}
Promise.defer = Promise.pending = function() {
debug.deprecated("Promise.defer", "new Promise");
var promise = new Promise(INTERNAL);
return {
promise: promise,
resolve: deferResolve,
reject: deferReject
};
};
util.notEnumerableProp(Promise,
"_makeSelfResolutionError",
makeSelfResolutionError);
_dereq_("./method")(Promise, INTERNAL, tryConvertToPromise, apiRejection,
debug);
_dereq_("./bind")(Promise, INTERNAL, tryConvertToPromise, debug);
_dereq_("./cancel")(Promise, PromiseArray, apiRejection, debug);
_dereq_("./direct_resolve")(Promise);
_dereq_("./synchronous_inspection")(Promise);
_dereq_("./join")(
Promise, PromiseArray, tryConvertToPromise, INTERNAL, async);
Promise.Promise = Promise;
Promise.version = "3.7.2";
_dereq_('./call_get.js')(Promise);
_dereq_('./generators.js')(Promise, apiRejection, INTERNAL, tryConvertToPromise, Proxyable, debug);
_dereq_('./map.js')(Promise, PromiseArray, apiRejection, tryConvertToPromise, INTERNAL, debug);
_dereq_('./nodeify.js')(Promise);
_dereq_('./promisify.js')(Promise, INTERNAL);
_dereq_('./props.js')(Promise, PromiseArray, tryConvertToPromise, apiRejection);
_dereq_('./race.js')(Promise, INTERNAL, tryConvertToPromise, apiRejection);
_dereq_('./reduce.js')(Promise, PromiseArray, apiRejection, tryConvertToPromise, INTERNAL, debug);
_dereq_('./settle.js')(Promise, PromiseArray, debug);
_dereq_('./some.js')(Promise, PromiseArray, apiRejection);
_dereq_('./timers.js')(Promise, INTERNAL, debug);
_dereq_('./using.js')(Promise, apiRejection, tryConvertToPromise, createContext, INTERNAL, debug);
_dereq_('./any.js')(Promise);
_dereq_('./each.js')(Promise, INTERNAL);
_dereq_('./filter.js')(Promise, INTERNAL);
util.toFastProperties(Promise);
util.toFastProperties(Promise.prototype);
function fillTypes(value) {
var p = new Promise(INTERNAL);
p._fulfillmentHandler0 = value;
p._rejectionHandler0 = value;
p._promise0 = value;
p._receiver0 = value;
}
// Complete slack tracking, opt out of field-type tracking and
// stabilize map
fillTypes({a: 1});
fillTypes({b: 2});
fillTypes({c: 3});
fillTypes(1);
fillTypes(function(){});
fillTypes(undefined);
fillTypes(false);
fillTypes(new Promise(INTERNAL));
debug.setBounds(Async.firstLineError, util.lastLineError);
return Promise;
};
},{"./any.js":1,"./async":2,"./bind":3,"./call_get.js":5,"./cancel":6,"./catch_filter":7,"./context":8,"./debuggability":9,"./direct_resolve":10,"./each.js":11,"./errors":12,"./es5":13,"./filter.js":14,"./finally":15,"./generators.js":16,"./join":17,"./map.js":18,"./method":19,"./nodeback":20,"./nodeify.js":21,"./promise_array":23,"./promisify.js":24,"./props.js":25,"./race.js":27,"./reduce.js":28,"./settle.js":30,"./some.js":31,"./synchronous_inspection":32,"./thenables":33,"./timers.js":34,"./using.js":35,"./util":36,"async_hooks":undefined}],23:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL, tryConvertToPromise,
apiRejection, Proxyable) {
var util = _dereq_("./util");
var isArray = util.isArray;
function toResolutionValue(val) {
switch(val) {
case -2: return [];
case -3: return {};
case -6: return new Map();
}
}
function PromiseArray(values) {
var promise = this._promise = new Promise(INTERNAL);
if (values instanceof Promise) {
promise._propagateFrom(values, 3);
values.suppressUnhandledRejections();
}
promise._setOnCancel(this);
this._values = values;
this._length = 0;
this._totalResolved = 0;
this._init(undefined, -2);
}
util.inherits(PromiseArray, Proxyable);
PromiseArray.prototype.length = function () {
return this._length;
};
PromiseArray.prototype.promise = function () {
return this._promise;
};
PromiseArray.prototype._init = function init(_, resolveValueIfEmpty) {
var values = tryConvertToPromise(this._values, this._promise);
if (values instanceof Promise) {
values = values._target();
var bitField = values._bitField;
;
this._values = values;
if (((bitField & 50397184) === 0)) {
this._promise._setAsyncGuaranteed();
return values._then(
init,
this._reject,
undefined,
this,
resolveValueIfEmpty
);
} else if (((bitField & 33554432) !== 0)) {
values = values._value();
} else if (((bitField & 16777216) !== 0)) {
return this._reject(values._reason());
} else {
return this._cancel();
}
}
values = util.asArray(values);
if (values === null) {
var err = apiRejection(
"expecting an array or an iterable object but got " + util.classString(values)).reason();
this._promise._rejectCallback(err, false);
return;
}
if (values.length === 0) {
if (resolveValueIfEmpty === -5) {
this._resolveEmptyArray();
}
else {
this._resolve(toResolutionValue(resolveValueIfEmpty));
}
return;
}
this._iterate(values);
};
PromiseArray.prototype._iterate = function(values) {
var len = this.getActualLength(values.length);
this._length = len;
this._values = this.shouldCopyValues() ? new Array(len) : this._values;
var result = this._promise;
var isResolved = false;
var bitField = null;
for (var i = 0; i < len; ++i) {
var maybePromise = tryConvertToPromise(values[i], result);
if (maybePromise instanceof Promise) {
maybePromise = maybePromise._target();
bitField = maybePromise._bitField;
} else {
bitField = null;
}
if (isResolved) {
if (bitField !== null) {
maybePromise.suppressUnhandledRejections();
}
} else if (bitField !== null) {
if (((bitField & 50397184) === 0)) {
maybePromise._proxy(this, i);
this._values[i] = maybePromise;
} else if (((bitField & 33554432) !== 0)) {
isResolved = this._promiseFulfilled(maybePromise._value(), i);
} else if (((bitField & 16777216) !== 0)) {
isResolved = this._promiseRejected(maybePromise._reason(), i);
} else {
isResolved = this._promiseCancelled(i);
}
} else {
isResolved = this._promiseFulfilled(maybePromise, i);
}
}
if (!isResolved) result._setAsyncGuaranteed();
};
PromiseArray.prototype._isResolved = function () {
return this._values === null;
};
PromiseArray.prototype._resolve = function (value) {
this._values = null;
this._promise._fulfill(value);
};
PromiseArray.prototype._cancel = function() {
if (this._isResolved() || !this._promise._isCancellable()) return;
this._values = null;
this._promise._cancel();
};
PromiseArray.prototype._reject = function (reason) {
this._values = null;
this._promise._rejectCallback(reason, false);
};
PromiseArray.prototype._promiseFulfilled = function (value, index) {
this._values[index] = value;
var totalResolved = ++this._totalResolved;
if (totalResolved >= this._length) {
this._resolve(this._values);
return true;
}
return false;
};
PromiseArray.prototype._promiseCancelled = function() {
this._cancel();
return true;
};
PromiseArray.prototype._promiseRejected = function (reason) {
this._totalResolved++;
this._reject(reason);
return true;
};
PromiseArray.prototype._resultCancelled = function() {
if (this._isResolved()) return;
var values = this._values;
this._cancel();
if (values instanceof Promise) {
values.cancel();
} else {
for (var i = 0; i < values.length; ++i) {
if (values[i] instanceof Promise) {
values[i].cancel();
}
}
}
};
PromiseArray.prototype.shouldCopyValues = function () {
return true;
};
PromiseArray.prototype.getActualLength = function (len) {
return len;
};
return PromiseArray;
};
},{"./util":36}],24:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL) {
var THIS = {};
var util = _dereq_("./util");
var nodebackForPromise = _dereq_("./nodeback");
var withAppended = util.withAppended;
var maybeWrapAsError = util.maybeWrapAsError;
var canEvaluate = util.canEvaluate;
var TypeError = _dereq_("./errors").TypeError;
var defaultSuffix = "Async";
var defaultPromisified = {__isPromisified__: true};
var noCopyProps = [
"arity", "length",
"name",
"arguments",
"caller",
"callee",
"prototype",
"__isPromisified__"
];
var noCopyPropsPattern = new RegExp("^(?:" + noCopyProps.join("|") + ")$");
var defaultFilter = function(name) {
return util.isIdentifier(name) &&
name.charAt(0) !== "_" &&
name !== "constructor";
};
function propsFilter(key) {
return !noCopyPropsPattern.test(key);
}
function isPromisified(fn) {
try {
return fn.__isPromisified__ === true;
}
catch (e) {
return false;
}
}
function hasPromisified(obj, key, suffix) {
var val = util.getDataPropertyOrDefault(obj, key + suffix,
defaultPromisified);
return val ? isPromisified(val) : false;
}
function checkValid(ret, suffix, suffixRegexp) {
for (var i = 0; i < ret.length; i += 2) {
var key = ret[i];
if (suffixRegexp.test(key)) {
var keyWithoutAsyncSuffix = key.replace(suffixRegexp, "");
for (var j = 0; j < ret.length; j += 2) {
if (ret[j] === keyWithoutAsyncSuffix) {
throw new TypeError("Cannot promisify an API that has normal methods with '%s'-suffix\u000a\u000a See http://goo.gl/MqrFmX\u000a"
.replace("%s", suffix));
}
}
}
}
}
function promisifiableMethods(obj, suffix, suffixRegexp, filter) {
var keys = util.inheritedDataKeys(obj);
var ret = [];
for (var i = 0; i < keys.length; ++i) {
var key = keys[i];
var value = obj[key];
var passesDefaultFilter = filter === defaultFilter
? true : defaultFilter(key, value, obj);
if (typeof value === "function" &&
!isPromisified(value) &&
!hasPromisified(obj, key, suffix) &&
filter(key, value, obj, passesDefaultFilter)) {
ret.push(key, value);
}
}
checkValid(ret, suffix, suffixRegexp);
return ret;
}
var escapeIdentRegex = function(str) {
return str.replace(/([$])/, "\\$");
};
var makeNodePromisifiedEval;
if (!true) {
var switchCaseArgumentOrder = function(likelyArgumentCount) {
var ret = [likelyArgumentCount];
var min = Math.max(0, likelyArgumentCount - 1 - 3);
for(var i = likelyArgumentCount - 1; i >= min; --i) {
ret.push(i);
}
for(var i = likelyArgumentCount + 1; i <= 3; ++i) {
ret.push(i);
}
return ret;
};
var argumentSequence = function(argumentCount) {
return util.filledRange(argumentCount, "_arg", "");
};
var parameterDeclaration = function(parameterCount) {
return util.filledRange(
Math.max(parameterCount, 3), "_arg", "");
};
var parameterCount = function(fn) {
if (typeof fn.length === "number") {
return Math.max(Math.min(fn.length, 1023 + 1), 0);
}
return 0;
};
makeNodePromisifiedEval =
function(callback, receiver, originalName, fn, _, multiArgs) {
var newParameterCount = Math.max(0, parameterCount(fn) - 1);
var argumentOrder = switchCaseArgumentOrder(newParameterCount);
var shouldProxyThis = typeof callback === "string" || receiver === THIS;
function generateCallForArgumentCount(count) {
var args = argumentSequence(count).join(", ");
var comma = count > 0 ? ", " : "";
var ret;
if (shouldProxyThis) {
ret = "ret = callback.call(this, {{args}}, nodeback); break;\n";
} else {
ret = receiver === undefined
? "ret = callback({{args}}, nodeback); break;\n"
: "ret = callback.call(receiver, {{args}}, nodeback); break;\n";
}
return ret.replace("{{args}}", args).replace(", ", comma);
}
function generateArgumentSwitchCase() {
var ret = "";
for (var i = 0; i < argumentOrder.length; ++i) {
ret += "case " + argumentOrder[i] +":" +
generateCallForArgumentCount(argumentOrder[i]);
}
ret += " \n\
default: \n\
var args = new Array(len + 1); \n\
var i = 0; \n\
for (var i = 0; i < len; ++i) { \n\
args[i] = arguments[i]; \n\
} \n\
args[i] = nodeback; \n\
[CodeForCall] \n\
break; \n\
".replace("[CodeForCall]", (shouldProxyThis
? "ret = callback.apply(this, args);\n"
: "ret = callback.apply(receiver, args);\n"));
return ret;
}
var getFunctionCode = typeof callback === "string"
? ("this != null ? this['"+callback+"'] : fn")
: "fn";
var body = "'use strict'; \n\
var ret = function (Parameters) { \n\
'use strict'; \n\
var len = arguments.length; \n\
var promise = new Promise(INTERNAL); \n\
promise._captureStackTrace(); \n\
var nodeback = nodebackForPromise(promise, " + multiArgs + "); \n\
var ret; \n\
var callback = tryCatch([GetFunctionCode]); \n\
switch(len) { \n\
[CodeForSwitchCase] \n\
} \n\
if (ret === errorObj) { \n\
promise._rejectCallback(maybeWrapAsError(ret.e), true, true);\n\
} \n\
if (!promise._isFateSealed()) promise._setAsyncGuaranteed(); \n\
return promise; \n\
}; \n\
notEnumerableProp(ret, '__isPromisified__', true); \n\
return ret; \n\
".replace("[CodeForSwitchCase]", generateArgumentSwitchCase())
.replace("[GetFunctionCode]", getFunctionCode);
body = body.replace("Parameters", parameterDeclaration(newParameterCount));
return new Function("Promise",
"fn",
"receiver",
"withAppended",
"maybeWrapAsError",
"nodebackForPromise",
"tryCatch",
"errorObj",
"notEnumerableProp",
"INTERNAL",
body)(
Promise,
fn,
receiver,
withAppended,
maybeWrapAsError,
nodebackForPromise,
util.tryCatch,
util.errorObj,
util.notEnumerableProp,
INTERNAL);
};
}
function makeNodePromisifiedClosure(callback, receiver, _, fn, __, multiArgs) {
var defaultThis = (function() {return this;})();
var method = callback;
if (typeof method === "string") {
callback = fn;
}
function promisified() {
var _receiver = receiver;
if (receiver === THIS) _receiver = this;
var promise = new Promise(INTERNAL);
promise._captureStackTrace();
var cb = typeof method === "string" && this !== defaultThis
? this[method] : callback;
var fn = nodebackForPromise(promise, multiArgs);
try {
cb.apply(_receiver, withAppended(arguments, fn));
} catch(e) {
promise._rejectCallback(maybeWrapAsError(e), true, true);
}
if (!promise._isFateSealed()) promise._setAsyncGuaranteed();
return promise;
}
util.notEnumerableProp(promisified, "__isPromisified__", true);
return promisified;
}
var makeNodePromisified = canEvaluate
? makeNodePromisifiedEval
: makeNodePromisifiedClosure;
function promisifyAll(obj, suffix, filter, promisifier, multiArgs) {
var suffixRegexp = new RegExp(escapeIdentRegex(suffix) + "$");
var methods =
promisifiableMethods(obj, suffix, suffixRegexp, filter);
for (var i = 0, len = methods.length; i < len; i+= 2) {
var key = methods[i];
var fn = methods[i+1];
var promisifiedKey = key + suffix;
if (promisifier === makeNodePromisified) {
obj[promisifiedKey] =
makeNodePromisified(key, THIS, key, fn, suffix, multiArgs);
} else {
var promisified = promisifier(fn, function() {
return makeNodePromisified(key, THIS, key,
fn, suffix, multiArgs);
});
util.notEnumerableProp(promisified, "__isPromisified__", true);
obj[promisifiedKey] = promisified;
}
}
util.toFastProperties(obj);
return obj;
}
function promisify(callback, receiver, multiArgs) {
return makeNodePromisified(callback, receiver, undefined,
callback, null, multiArgs);
}
Promise.promisify = function (fn, options) {
if (typeof fn !== "function") {
throw new TypeError("expecting a function but got " + util.classString(fn));
}
if (isPromisified(fn)) {
return fn;
}
options = Object(options);
var receiver = options.context === undefined ? THIS : options.context;
var multiArgs = !!options.multiArgs;
var ret = promisify(fn, receiver, multiArgs);
util.copyDescriptors(fn, ret, propsFilter);
return ret;
};
Promise.promisifyAll = function (target, options) {
if (typeof target !== "function" && typeof target !== "object") {
throw new TypeError("the target of promisifyAll must be an object or a function\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
options = Object(options);
var multiArgs = !!options.multiArgs;
var suffix = options.suffix;
if (typeof suffix !== "string") suffix = defaultSuffix;
var filter = options.filter;
if (typeof filter !== "function") filter = defaultFilter;
var promisifier = options.promisifier;
if (typeof promisifier !== "function") promisifier = makeNodePromisified;
if (!util.isIdentifier(suffix)) {
throw new RangeError("suffix must be a valid identifier\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
var keys = util.inheritedDataKeys(target);
for (var i = 0; i < keys.length; ++i) {
var value = target[keys[i]];
if (keys[i] !== "constructor" &&
util.isClass(value)) {
promisifyAll(value.prototype, suffix, filter, promisifier,
multiArgs);
promisifyAll(value, suffix, filter, promisifier, multiArgs);
}
}
return promisifyAll(target, suffix, filter, promisifier, multiArgs);
};
};
},{"./errors":12,"./nodeback":20,"./util":36}],25:[function(_dereq_,module,exports){
"use strict";
module.exports = function(
Promise, PromiseArray, tryConvertToPromise, apiRejection) {
var util = _dereq_("./util");
var isObject = util.isObject;
var es5 = _dereq_("./es5");
var Es6Map;
if (typeof Map === "function") Es6Map = Map;
var mapToEntries = (function() {
var index = 0;
var size = 0;
function extractEntry(value, key) {
this[index] = value;
this[index + size] = key;
index++;
}
return function mapToEntries(map) {
size = map.size;
index = 0;
var ret = new Array(map.size * 2);
map.forEach(extractEntry, ret);
return ret;
};
})();
var entriesToMap = function(entries) {
var ret = new Es6Map();
var length = entries.length / 2 | 0;
for (var i = 0; i < length; ++i) {
var key = entries[length + i];
var value = entries[i];
ret.set(key, value);
}
return ret;
};
function PropertiesPromiseArray(obj) {
var isMap = false;
var entries;
if (Es6Map !== undefined && obj instanceof Es6Map) {
entries = mapToEntries(obj);
isMap = true;
} else {
var keys = es5.keys(obj);
var len = keys.length;
entries = new Array(len * 2);
for (var i = 0; i < len; ++i) {
var key = keys[i];
entries[i] = obj[key];
entries[i + len] = key;
}
}
this.constructor$(entries);
this._isMap = isMap;
this._init$(undefined, isMap ? -6 : -3);
}
util.inherits(PropertiesPromiseArray, PromiseArray);
PropertiesPromiseArray.prototype._init = function () {};
PropertiesPromiseArray.prototype._promiseFulfilled = function (value, index) {
this._values[index] = value;
var totalResolved = ++this._totalResolved;
if (totalResolved >= this._length) {
var val;
if (this._isMap) {
val = entriesToMap(this._values);
} else {
val = {};
var keyOffset = this.length();
for (var i = 0, len = this.length(); i < len; ++i) {
val[this._values[i + keyOffset]] = this._values[i];
}
}
this._resolve(val);
return true;
}
return false;
};
PropertiesPromiseArray.prototype.shouldCopyValues = function () {
return false;
};
PropertiesPromiseArray.prototype.getActualLength = function (len) {
return len >> 1;
};
function props(promises) {
var ret;
var castValue = tryConvertToPromise(promises);
if (!isObject(castValue)) {
return apiRejection("cannot await properties of a non-object\u000a\u000a See http://goo.gl/MqrFmX\u000a");
} else if (castValue instanceof Promise) {
ret = castValue._then(
Promise.props, undefined, undefined, undefined, undefined);
} else {
ret = new PropertiesPromiseArray(castValue).promise();
}
if (castValue instanceof Promise) {
ret._propagateFrom(castValue, 2);
}
return ret;
}
Promise.prototype.props = function () {
return props(this);
};
Promise.props = function (promises) {
return props(promises);
};
};
},{"./es5":13,"./util":36}],26:[function(_dereq_,module,exports){
"use strict";
function arrayMove(src, srcIndex, dst, dstIndex, len) {
for (var j = 0; j < len; ++j) {
dst[j + dstIndex] = src[j + srcIndex];
src[j + srcIndex] = void 0;
}
}
function Queue(capacity) {
this._capacity = capacity;
this._length = 0;
this._front = 0;
}
Queue.prototype._willBeOverCapacity = function (size) {
return this._capacity < size;
};
Queue.prototype._pushOne = function (arg) {
var length = this.length();
this._checkCapacity(length + 1);
var i = (this._front + length) & (this._capacity - 1);
this[i] = arg;
this._length = length + 1;
};
Queue.prototype.push = function (fn, receiver, arg) {
var length = this.length() + 3;
if (this._willBeOverCapacity(length)) {
this._pushOne(fn);
this._pushOne(receiver);
this._pushOne(arg);
return;
}
var j = this._front + length - 3;
this._checkCapacity(length);
var wrapMask = this._capacity - 1;
this[(j + 0) & wrapMask] = fn;
this[(j + 1) & wrapMask] = receiver;
this[(j + 2) & wrapMask] = arg;
this._length = length;
};
Queue.prototype.shift = function () {
var front = this._front,
ret = this[front];
this[front] = undefined;
this._front = (front + 1) & (this._capacity - 1);
this._length--;
return ret;
};
Queue.prototype.length = function () {
return this._length;
};
Queue.prototype._checkCapacity = function (size) {
if (this._capacity < size) {
this._resizeTo(this._capacity << 1);
}
};
Queue.prototype._resizeTo = function (capacity) {
var oldCapacity = this._capacity;
this._capacity = capacity;
var front = this._front;
var length = this._length;
var moveItemsCount = (front + length) & (oldCapacity - 1);
arrayMove(this, 0, this, oldCapacity, moveItemsCount);
};
module.exports = Queue;
},{}],27:[function(_dereq_,module,exports){
"use strict";
module.exports = function(
Promise, INTERNAL, tryConvertToPromise, apiRejection) {
var util = _dereq_("./util");
var raceLater = function (promise) {
return promise.then(function(array) {
return race(array, promise);
});
};
function race(promises, parent) {
var maybePromise = tryConvertToPromise(promises);
if (maybePromise instanceof Promise) {
return raceLater(maybePromise);
} else {
promises = util.asArray(promises);
if (promises === null)
return apiRejection("expecting an array or an iterable object but got " + util.classString(promises));
}
var ret = new Promise(INTERNAL);
if (parent !== undefined) {
ret._propagateFrom(parent, 3);
}
var fulfill = ret._fulfill;
var reject = ret._reject;
for (var i = 0, len = promises.length; i < len; ++i) {
var val = promises[i];
if (val === undefined && !(i in promises)) {
continue;
}
Promise.cast(val)._then(fulfill, reject, undefined, ret, null);
}
return ret;
}
Promise.race = function (promises) {
return race(promises, undefined);
};
Promise.prototype.race = function () {
return race(this, undefined);
};
};
},{"./util":36}],28:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise,
PromiseArray,
apiRejection,
tryConvertToPromise,
INTERNAL,
debug) {
var util = _dereq_("./util");
var tryCatch = util.tryCatch;
function ReductionPromiseArray(promises, fn, initialValue, _each) {
this.constructor$(promises);
var context = Promise._getContext();
this._fn = util.contextBind(context, fn);
if (initialValue !== undefined) {
initialValue = Promise.resolve(initialValue);
initialValue._attachCancellationCallback(this);
}
this._initialValue = initialValue;
this._currentCancellable = null;
if(_each === INTERNAL) {
this._eachValues = Array(this._length);
} else if (_each === 0) {
this._eachValues = null;
} else {
this._eachValues = undefined;
}
this._promise._captureStackTrace();
this._init$(undefined, -5);
}
util.inherits(ReductionPromiseArray, PromiseArray);
ReductionPromiseArray.prototype._gotAccum = function(accum) {
if (this._eachValues !== undefined &&
this._eachValues !== null &&
accum !== INTERNAL) {
this._eachValues.push(accum);
}
};
ReductionPromiseArray.prototype._eachComplete = function(value) {
if (this._eachValues !== null) {
this._eachValues.push(value);
}
return this._eachValues;
};
ReductionPromiseArray.prototype._init = function() {};
ReductionPromiseArray.prototype._resolveEmptyArray = function() {
this._resolve(this._eachValues !== undefined ? this._eachValues
: this._initialValue);
};
ReductionPromiseArray.prototype.shouldCopyValues = function () {
return false;
};
ReductionPromiseArray.prototype._resolve = function(value) {
this._promise._resolveCallback(value);
this._values = null;
};
ReductionPromiseArray.prototype._resultCancelled = function(sender) {
if (sender === this._initialValue) return this._cancel();
if (this._isResolved()) return;
this._resultCancelled$();
if (this._currentCancellable instanceof Promise) {
this._currentCancellable.cancel();
}
if (this._initialValue instanceof Promise) {
this._initialValue.cancel();
}
};
ReductionPromiseArray.prototype._iterate = function (values) {
this._values = values;
var value;
var i;
var length = values.length;
if (this._initialValue !== undefined) {
value = this._initialValue;
i = 0;
} else {
value = Promise.resolve(values[0]);
i = 1;
}
this._currentCancellable = value;
for (var j = i; j < length; ++j) {
var maybePromise = values[j];
if (maybePromise instanceof Promise) {
maybePromise.suppressUnhandledRejections();
}
}
if (!value.isRejected()) {
for (; i < length; ++i) {
var ctx = {
accum: null,
value: values[i],
index: i,
length: length,
array: this
};
value = value._then(gotAccum, undefined, undefined, ctx, undefined);
if ((i & 127) === 0) {
value._setNoAsyncGuarantee();
}
}
}
if (this._eachValues !== undefined) {
value = value
._then(this._eachComplete, undefined, undefined, this, undefined);
}
value._then(completed, completed, undefined, value, this);
};
Promise.prototype.reduce = function (fn, initialValue) {
return reduce(this, fn, initialValue, null);
};
Promise.reduce = function (promises, fn, initialValue, _each) {
return reduce(promises, fn, initialValue, _each);
};
function completed(valueOrReason, array) {
if (this.isFulfilled()) {
array._resolve(valueOrReason);
} else {
array._reject(valueOrReason);
}
}
function reduce(promises, fn, initialValue, _each) {
if (typeof fn !== "function") {
return apiRejection("expecting a function but got " + util.classString(fn));
}
var array = new ReductionPromiseArray(promises, fn, initialValue, _each);
return array.promise();
}
function gotAccum(accum) {
this.accum = accum;
this.array._gotAccum(accum);
var value = tryConvertToPromise(this.value, this.array._promise);
if (value instanceof Promise) {
this.array._currentCancellable = value;
return value._then(gotValue, undefined, undefined, this, undefined);
} else {
return gotValue.call(this, value);
}
}
function gotValue(value) {
var array = this.array;
var promise = array._promise;
var fn = tryCatch(array._fn);
promise._pushContext();
var ret;
if (array._eachValues !== undefined) {
ret = fn.call(promise._boundValue(), value, this.index, this.length);
} else {
ret = fn.call(promise._boundValue(),
this.accum, value, this.index, this.length);
}
if (ret instanceof Promise) {
array._currentCancellable = ret;
}
var promiseCreated = promise._popContext();
debug.checkForgottenReturns(
ret,
promiseCreated,
array._eachValues !== undefined ? "Promise.each" : "Promise.reduce",
promise
);
return ret;
}
};
},{"./util":36}],29:[function(_dereq_,module,exports){
"use strict";
var util = _dereq_("./util");
var schedule;
var noAsyncScheduler = function() {
throw new Error("No async scheduler available\u000a\u000a See http://goo.gl/MqrFmX\u000a");
};
var NativePromise = util.getNativePromise();
if (util.isNode && typeof MutationObserver === "undefined") {
var GlobalSetImmediate = global.setImmediate;
var ProcessNextTick = process.nextTick;
schedule = util.isRecentNode
? function(fn) { GlobalSetImmediate.call(global, fn); }
: function(fn) { ProcessNextTick.call(process, fn); };
} else if (typeof NativePromise === "function" &&
typeof NativePromise.resolve === "function") {
var nativePromise = NativePromise.resolve();
schedule = function(fn) {
nativePromise.then(fn);
};
} else if ((typeof MutationObserver !== "undefined") &&
!(typeof window !== "undefined" &&
window.navigator &&
(window.navigator.standalone || window.cordova)) &&
("classList" in document.documentElement)) {
schedule = (function() {
var div = document.createElement("div");
var opts = {attributes: true};
var toggleScheduled = false;
var div2 = document.createElement("div");
var o2 = new MutationObserver(function() {
div.classList.toggle("foo");
toggleScheduled = false;
});
o2.observe(div2, opts);
var scheduleToggle = function() {
if (toggleScheduled) return;
toggleScheduled = true;
div2.classList.toggle("foo");
};
return function schedule(fn) {
var o = new MutationObserver(function() {
o.disconnect();
fn();
});
o.observe(div, opts);
scheduleToggle();
};
})();
} else if (typeof setImmediate !== "undefined") {
schedule = function (fn) {
setImmediate(fn);
};
} else if (typeof setTimeout !== "undefined") {
schedule = function (fn) {
setTimeout(fn, 0);
};
} else {
schedule = noAsyncScheduler;
}
module.exports = schedule;
},{"./util":36}],30:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, PromiseArray, debug) {
var PromiseInspection = Promise.PromiseInspection;
var util = _dereq_("./util");
function SettledPromiseArray(values) {
this.constructor$(values);
}
util.inherits(SettledPromiseArray, PromiseArray);
SettledPromiseArray.prototype._promiseResolved = function (index, inspection) {
this._values[index] = inspection;
var totalResolved = ++this._totalResolved;
if (totalResolved >= this._length) {
this._resolve(this._values);
return true;
}
return false;
};
SettledPromiseArray.prototype._promiseFulfilled = function (value, index) {
var ret = new PromiseInspection();
ret._bitField = 33554432;
ret._settledValueField = value;
return this._promiseResolved(index, ret);
};
SettledPromiseArray.prototype._promiseRejected = function (reason, index) {
var ret = new PromiseInspection();
ret._bitField = 16777216;
ret._settledValueField = reason;
return this._promiseResolved(index, ret);
};
Promise.settle = function (promises) {
debug.deprecated(".settle()", ".reflect()");
return new SettledPromiseArray(promises).promise();
};
Promise.allSettled = function (promises) {
return new SettledPromiseArray(promises).promise();
};
Promise.prototype.settle = function () {
return Promise.settle(this);
};
};
},{"./util":36}],31:[function(_dereq_,module,exports){
"use strict";
module.exports =
function(Promise, PromiseArray, apiRejection) {
var util = _dereq_("./util");
var RangeError = _dereq_("./errors").RangeError;
var AggregateError = _dereq_("./errors").AggregateError;
var isArray = util.isArray;
var CANCELLATION = {};
function SomePromiseArray(values) {
this.constructor$(values);
this._howMany = 0;
this._unwrap = false;
this._initialized = false;
}
util.inherits(SomePromiseArray, PromiseArray);
SomePromiseArray.prototype._init = function () {
if (!this._initialized) {
return;
}
if (this._howMany === 0) {
this._resolve([]);
return;
}
this._init$(undefined, -5);
var isArrayResolved = isArray(this._values);
if (!this._isResolved() &&
isArrayResolved &&
this._howMany > this._canPossiblyFulfill()) {
this._reject(this._getRangeError(this.length()));
}
};
SomePromiseArray.prototype.init = function () {
this._initialized = true;
this._init();
};
SomePromiseArray.prototype.setUnwrap = function () {
this._unwrap = true;
};
SomePromiseArray.prototype.howMany = function () {
return this._howMany;
};
SomePromiseArray.prototype.setHowMany = function (count) {
this._howMany = count;
};
SomePromiseArray.prototype._promiseFulfilled = function (value) {
this._addFulfilled(value);
if (this._fulfilled() === this.howMany()) {
this._values.length = this.howMany();
if (this.howMany() === 1 && this._unwrap) {
this._resolve(this._values[0]);
} else {
this._resolve(this._values);
}
return true;
}
return false;
};
SomePromiseArray.prototype._promiseRejected = function (reason) {
this._addRejected(reason);
return this._checkOutcome();
};
SomePromiseArray.prototype._promiseCancelled = function () {
if (this._values instanceof Promise || this._values == null) {
return this._cancel();
}
this._addRejected(CANCELLATION);
return this._checkOutcome();
};
SomePromiseArray.prototype._checkOutcome = function() {
if (this.howMany() > this._canPossiblyFulfill()) {
var e = new AggregateError();
for (var i = this.length(); i < this._values.length; ++i) {
if (this._values[i] !== CANCELLATION) {
e.push(this._values[i]);
}
}
if (e.length > 0) {
this._reject(e);
} else {
this._cancel();
}
return true;
}
return false;
};
SomePromiseArray.prototype._fulfilled = function () {
return this._totalResolved;
};
SomePromiseArray.prototype._rejected = function () {
return this._values.length - this.length();
};
SomePromiseArray.prototype._addRejected = function (reason) {
this._values.push(reason);
};
SomePromiseArray.prototype._addFulfilled = function (value) {
this._values[this._totalResolved++] = value;
};
SomePromiseArray.prototype._canPossiblyFulfill = function () {
return this.length() - this._rejected();
};
SomePromiseArray.prototype._getRangeError = function (count) {
var message = "Input array must contain at least " +
this._howMany + " items but contains only " + count + " items";
return new RangeError(message);
};
SomePromiseArray.prototype._resolveEmptyArray = function () {
this._reject(this._getRangeError(0));
};
function some(promises, howMany) {
if ((howMany | 0) !== howMany || howMany < 0) {
return apiRejection("expecting a positive integer\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
var ret = new SomePromiseArray(promises);
var promise = ret.promise();
ret.setHowMany(howMany);
ret.init();
return promise;
}
Promise.some = function (promises, howMany) {
return some(promises, howMany);
};
Promise.prototype.some = function (howMany) {
return some(this, howMany);
};
Promise._SomePromiseArray = SomePromiseArray;
};
},{"./errors":12,"./util":36}],32:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise) {
function PromiseInspection(promise) {
if (promise !== undefined) {
promise = promise._target();
this._bitField = promise._bitField;
this._settledValueField = promise._isFateSealed()
? promise._settledValue() : undefined;
}
else {
this._bitField = 0;
this._settledValueField = undefined;
}
}
PromiseInspection.prototype._settledValue = function() {
return this._settledValueField;
};
var value = PromiseInspection.prototype.value = function () {
if (!this.isFulfilled()) {
throw new TypeError("cannot get fulfillment value of a non-fulfilled promise\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
return this._settledValue();
};
var reason = PromiseInspection.prototype.error =
PromiseInspection.prototype.reason = function () {
if (!this.isRejected()) {
throw new TypeError("cannot get rejection reason of a non-rejected promise\u000a\u000a See http://goo.gl/MqrFmX\u000a");
}
return this._settledValue();
};
var isFulfilled = PromiseInspection.prototype.isFulfilled = function() {
return (this._bitField & 33554432) !== 0;
};
var isRejected = PromiseInspection.prototype.isRejected = function () {
return (this._bitField & 16777216) !== 0;
};
var isPending = PromiseInspection.prototype.isPending = function () {
return (this._bitField & 50397184) === 0;
};
var isResolved = PromiseInspection.prototype.isResolved = function () {
return (this._bitField & 50331648) !== 0;
};
PromiseInspection.prototype.isCancelled = function() {
return (this._bitField & 8454144) !== 0;
};
Promise.prototype.__isCancelled = function() {
return (this._bitField & 65536) === 65536;
};
Promise.prototype._isCancelled = function() {
return this._target().__isCancelled();
};
Promise.prototype.isCancelled = function() {
return (this._target()._bitField & 8454144) !== 0;
};
Promise.prototype.isPending = function() {
return isPending.call(this._target());
};
Promise.prototype.isRejected = function() {
return isRejected.call(this._target());
};
Promise.prototype.isFulfilled = function() {
return isFulfilled.call(this._target());
};
Promise.prototype.isResolved = function() {
return isResolved.call(this._target());
};
Promise.prototype.value = function() {
return value.call(this._target());
};
Promise.prototype.reason = function() {
var target = this._target();
target._unsetRejectionIsUnhandled();
return reason.call(target);
};
Promise.prototype._value = function() {
return this._settledValue();
};
Promise.prototype._reason = function() {
this._unsetRejectionIsUnhandled();
return this._settledValue();
};
Promise.PromiseInspection = PromiseInspection;
};
},{}],33:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL) {
var util = _dereq_("./util");
var errorObj = util.errorObj;
var isObject = util.isObject;
function tryConvertToPromise(obj, context) {
if (isObject(obj)) {
if (obj instanceof Promise) return obj;
var then = getThen(obj);
if (then === errorObj) {
if (context) context._pushContext();
var ret = Promise.reject(then.e);
if (context) context._popContext();
return ret;
} else if (typeof then === "function") {
if (isAnyBluebirdPromise(obj)) {
var ret = new Promise(INTERNAL);
obj._then(
ret._fulfill,
ret._reject,
undefined,
ret,
null
);
return ret;
}
return doThenable(obj, then, context);
}
}
return obj;
}
function doGetThen(obj) {
return obj.then;
}
function getThen(obj) {
try {
return doGetThen(obj);
} catch (e) {
errorObj.e = e;
return errorObj;
}
}
var hasProp = {}.hasOwnProperty;
function isAnyBluebirdPromise(obj) {
try {
return hasProp.call(obj, "_promise0");
} catch (e) {
return false;
}
}
function doThenable(x, then, context) {
var promise = new Promise(INTERNAL);
var ret = promise;
if (context) context._pushContext();
promise._captureStackTrace();
if (context) context._popContext();
var synchronous = true;
var result = util.tryCatch(then).call(x, resolve, reject);
synchronous = false;
if (promise && result === errorObj) {
promise._rejectCallback(result.e, true, true);
promise = null;
}
function resolve(value) {
if (!promise) return;
promise._resolveCallback(value);
promise = null;
}
function reject(reason) {
if (!promise) return;
promise._rejectCallback(reason, synchronous, true);
promise = null;
}
return ret;
}
return tryConvertToPromise;
};
},{"./util":36}],34:[function(_dereq_,module,exports){
"use strict";
module.exports = function(Promise, INTERNAL, debug) {
var util = _dereq_("./util");
var TimeoutError = Promise.TimeoutError;
function HandleWrapper(handle) {
this.handle = handle;
}
HandleWrapper.prototype._resultCancelled = function() {
clearTimeout(this.handle);
};
var afterValue = function(value) { return delay(+this).thenReturn(value); };
var delay = Promise.delay = function (ms, value) {
var ret;
var handle;
if (value !== undefined) {
ret = Promise.resolve(value)
._then(afterValue, null, null, ms, undefined);
if (debug.cancellation() && value instanceof Promise) {
ret._setOnCancel(value);
}
} else {
ret = new Promise(INTERNAL);
handle = setTimeout(function() { ret._fulfill(); }, +ms);
if (debug.cancellation()) {
ret._setOnCancel(new HandleWrapper(handle));
}
ret._captureStackTrace();
}
ret._setAsyncGuaranteed();
return ret;
};
Promise.prototype.delay = function (ms) {
return delay(ms, this);
};
var afterTimeout = function (promise, message, parent) {
var err;
if (typeof message !== "string") {
if (message instanceof Error) {
err = message;
} else {
err = new TimeoutError("operation timed out");
}
} else {
err = new TimeoutError(message);
}
util.markAsOriginatingFromRejection(err);
promise._attachExtraTrace(err);
promise._reject(err);
if (parent != null) {
parent.cancel();
}
};
function successClear(value) {
clearTimeout(this.handle);
return value;
}
function failureClear(reason) {
clearTimeout(this.handle);
throw reason;
}
Promise.prototype.timeout = function (ms, message) {
ms = +ms;
var ret, parent;
var handleWrapper = new HandleWrapper(setTimeout(function timeoutTimeout() {
if (ret.isPending()) {
afterTimeout(ret, message, parent);
}
}, ms));
if (debug.cancellation()) {
parent = this.then();
ret = parent._then(successClear, failureClear,
undefined, handleWrapper, undefined);
ret._setOnCancel(handleWrapper);
} else {
ret = this._then(successClear, failureClear,
undefined, handleWrapper, undefined);
}
return ret;
};
};
},{"./util":36}],35:[function(_dereq_,module,exports){
"use strict";
module.exports = function (Promise, apiRejection, tryConvertToPromise,
createContext, INTERNAL, debug) {
var util = _dereq_("./util");
var TypeError = _dereq_("./errors").TypeError;
var inherits = _dereq_("./util").inherits;
...