There are a few different times in history when computing has had a significant effect on the world. This isn't always large scale political events, sometimes it's the capturing of an audience and setting up a culture that will reverberate for decades. The Golden Age of Arcade Games is thought to have lasted from the 1970s through to about 1983. In 1982, arcade games made more money in the USA than both their music industry and film industry combined.
Welcome to Freefall, your first major project in COMP1511. This is a game that's based on some of the classics of the Golden Age, the vertical shooters like Space Invaders and Galaga. In this assignment you will create Freefall, an array based command line game where the player must defend against falling stones!
If you'd like to try the game, it's available as command on any CSE computer. The aim of the game is to destroy all of the map's falling stones with your laser before they reach the ground. If the map is cleared of all stones, the game is won. If any stones make it past the bottom row, the game is lost.
1511 arcade solution
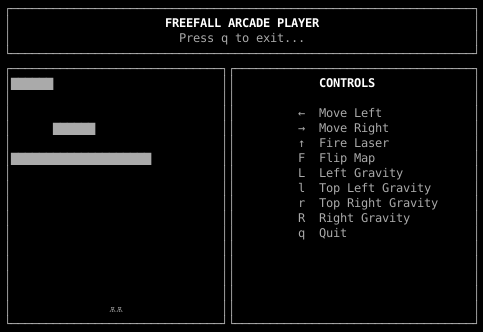
This assignment doesn't involve creating the full interactive terminal interface provided byarcade
. The final product is a more stripped down, simplified version where the game will be controlled by a series of commands of integers entered by the user.
The starter code for Freefall (see below) is already capable of printing the map, but it will be up to you to write code so that it can first add stones to the map, then read commands and make the correct changes to the map.
At time of release of this assignment (end of Week 3), COMP1511 has not yet covered all of the techniques and topics necessary to complete this assignment. At the end of Week 3, the course has covered enough content to be able to read in a single command and process its integers, but not enough to work with two dimensional arrays like the map or be able to handle multiple commands ending in End-of-Input (Ctrl-D). We will be covering these topics in the lectures, tutorials, and labs in Week 4.
The Map
The map is a two dimensional array (an array of arrays) of integers that represents the space that the game is played in. In stage 1 and 2, the map will only store either a0
or a1
. A0
represents an empty square, and a1
represents a stone. In the starter code there are#define
s for both of these values, which we will reference for the remainder of this page.
The map is a fixed-size square-shaped grid and hasSIZE
rows, andSIZE
columns.SIZE
is another#define
'd constant with the value15
.
Remember, both the rows and columns start at0
, not at1
.
|
0 |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
9 |
10 |
11 |
12 |
13 |
14 |
---|
0 |
[0][0] |
[0][1] |
[0][2] |
[0][3] |
[0][4] |
[0][5] |
[0][6] |
[0][7] |
[0][8] |
[0][9] |
[0][10] |
[0][11] |
[0][12] |
[0][13] |
[0][14] |
---|
1 |
[1][0] |
[1][1] |
[1][2] |
[1][3] |
[1][4] |
[1][5] |
[1][6] |
[1][7] |
[1][8] |
[1][9] |
[1][10] |
[1][11] |
[1][12] |
[1][13] |
[1][14] |
---|
2 |
[2][0] |
[2][1] |
[2][2] |
[2][3] |
[2][4] |
[2][5] |
[2][6] |
[2][7] |
[2][8] |
[2][9] |
[2][10] |
[2][11] |
[2][12] |
[2][13] |
[2][14] |
---|
3 |
[3][0] |
[3][1] |
[3][2] |
[3][3] |
[3][4] |
[3][5] |
[3][6] |
[3][7] |
[3][8] |
[3][9] |
[3][10] |
[3][11] |
[3][12] |
[3][13] |
[3][14] |
---|
4 |
[4][0] |
[4][1] |
[4][2] |
[4][3] |
[4][4] |
[4][5] |
[4][6] |
[4][7] |
[4][8] |
[4][9] |
[4][10] |
[4][11] |
[4][12] |
[4][13] |
[4][14] |
---|
5 |
[5][0] |
[5][1] |
[5][2] |
[5][3] |
[5][4] |
[5][5] |
[5][6] |
[5][7] |
[5][8] |
[5][9] |
[5][10] |
[5][11] |
[5][12] |
[5][13] |
[5][14] |
---|
6 |
[6][0] |
[6][1] |
[6][2] |
[6][3] |
[6][4] |
[6][5] |
[6][6] |
[6][7] |
[6][8] |
[6][9] |
[6][10] |
[6][11] |
[6][12] |
[6][13] |
[6][14] |
---|
7 |
[7][0] |
[7][1] |
[7][2] |
[7][3] |
[7][4] |
[7][5] |
[7][6] |
[7][7] |
[7][8] |
[7][9] |
[7][10] |
[7][11] |
[7][12] |
[7][13] |
[7][14] |
---|
8 |
[8][0] |
[8][1] |
[8][2] |
[8][3] |
[8][4] |
[8][5] |
[8][6] |
[8][7] |
[8][8] |
[8][9] |
[8][10] |
[8][11] |
[8][12] |
[8][13] |
[8][14] |
---|
9 |
[9][0] |
[9][1] |
[9][2] |
[9][3] |
[9][4] |
[9][5] |
[9][6] |
[9][7] |
[9][8] |
[9][9] |
[9][10] |
[9][11] |
[9][12] |
[9][13] |
[9][14] |
---|
10 |
[10][0] |
[10][1] |
[10][2] |
[10][3] |
[10][4] |
[10][5] |
[10][6] |
[10][7] |
[10][8] |
[10][9] |
[10][10] |
[10][11] |
[10][12] |
[10][13] |
[10][14] |
---|
11 |
[11][0] |
[11][1] |
[11][2] |
[11][3] |
[11][4] |
[11][5] |
[11][6] |
[11][7] |
[11][8] |
[11][9] |
[11][10] |
[11][11] |
[11][12] |
[11][13] |
[11][14] |
---|
12 |
[12][0] |
[12][1] |
[12][2] |
[12][3] |
[12][4] |
[12][5] |
[12][6] |
[12][7] |
[12][8] |
[12][9] |
[12][10] |
[12][11] |
[12][12] |
[12][13] |
[12][14] |
---|
13 |
[13][0] |
[13][1] |
[13][2] |
[13][3] |
[13][4] |
[13][5] |
[13][6] |
[13][7] |
[13][8] |
[13][9] |
[13][10] |
[13][11] |
[13][12] |
[13][13] |
[13][14] |
---|
14 |
[14][0] |
[14][1] |
[14][2] |
[14][3] |
[14][4] |
[14][5] |
[14][6] |
[14][7] |
[14][8] |
[14][9] |
[14][10] |
[14][11] |
[14][12] |
[14][13] |
[14][14] |
---|
The top left corner of the grid is[0][0]
and the bottom right corner of the grid is[SIZE - 1][SIZE - 1]
. Note that, like the array itself, we are using rows as the first coordinate in pairs of coordinates. This is the opposite order you might be used to in maths.
For example, if in a command we are given an input pair of coordinates5 6
, we will use that to find a particular square in our map by accessing the individual element in the array:map[5][6]
. This represents the square in the 6th row and the 7th column (remember arrays start counting at0
).
When the program begins, all of the squares in the map should have the valueEMPTY
. The starter code does this part for you. You must then populate the map with stones (i.e.,STONE
) by scanning the locations of horizontal lines of stones (more detail on this later). After this, you must scan in commands untilEOF
, making the appropriate changes to the map. The format of the inputted commands and changes to the map required are specified in this page.
If you ever have a question in the form of "What should my program do if it is given these inputs?" you can run the Freefall reference solution and copy its behaviour. This is a complete solution to the assignment which does not use the interactive terminal interface of1511 arcade
.
1511 freefall_reference
Allowed C Features
In this assignment, there are no restrictions on C features, except for those in theStyle Guide.
Westronglyencourage you to complete the assessment using only features taught in lectures up to and including Week 4. The only C features you will need to get full marks in the assignment are:
int
(and possiblydouble
) variables;
if
statements, including all relational and logical operators;
while
loops;
int
arrays, including two dimensional arrays;
printf
andscanf
; and
- functions.
Using any other features will not increase your marks (and may make it more likely to make style mistakes that cost you marks).
If you choose to disregard this advice, youmuststill follow theStyle Guide. You also may be unable to get help from course staff if you use features not taught in COMP1511.
Starter Code
Download the starter code (freefall.c) hereor use this command on your CSE account to copy the file into your current directory:
cp -n /web/cs1511/20T2/activities/freefall/freefall.c .
freefall.c
is the starting point for your Freefall program. We've provided you with some constants and some starter code to display the map as basic integers on the screen; you'll be completing the rest of the program.
Inputs and Validity
All of the inputs to your program will be in the form of a sequence of integers on standard input.
After scanning in all of the inputs relating to the rows of stone to place on the map, you will need to scan commands untilEOF
. In these commands, the first number specifies the type of command, for instance, if the first number is1
then this represents a command to move the player. Commands may optionally have additional so-called "argument" integers after the initial one.
You can be guaranteed that the first integer of any command will be valid. You can assume that the correct number of integers will be given for any particular command. In many other places, you may be given invalid input, such as negative numbers where you don't expect them. This includes any of the argument integers after the first integer of each command, and also includes the first part of scanning lines of stone in to the map. The ways in which commands might be invalid will be specified in each relevant section for you.
Throughout this assignment we encourage you to think like ahacker. What inputs would break your code? If you find any inputs which break the reference solution, please let us know!
Stage 1
Stage 1 implements the ability to read in lines of stone and place them onto the map, move the player to the left and right (and print this on the screen), and fire the laser to destroy fallingSTONE
.
Placing Stone
The program should first ask for the number of lines ofSTONE
s as an integer. Then, the program will scan the locations of the lines as a group of four integers in the following format:
row column length value
Therow
andcolumn
represent the left-most block of a horizontal line of blocks to the placed on the map. Thelength
tells you how many stones should be in this horizontal line. For stage 1 and 2, the fourth integer will always be1
representing a stone, however in other stages there will be other possibilities such as exploding TNT and marching blocks. This number is the value which should be placed in the array itself to make up the specified line. For example:
Command |
Meaning |
---|
0 0 5 1
|
Place a line of stone starting at[0][0] and ending at[0][4] . All5 squares in the line will be set to1 (STONE ).
|
6 7 1 1
|
Place a line of stone starting at[6][7] and ending at[6][7] . The1 square in the line will be set to1 (STONE ).
|
5 0 15 1
|
Place a line of stone starting at[5][0] and ending at[5][14] . All15 squares in the line will be set to1 (STONE ).
|
./freefall
How many lines of stone? 3 Enter lines of stone: 0 0 5 1 6 7 1 1 5 0 15 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P
After scanning in the inputs relating to placing lines of stone on the map, the program should then callprintMap
to display the map on the screen. The line of code to do this is already in the starter code. After printing the map, the program should start to scan in commands untilEOF
. After processing each command, your program should callprintMap
once again to show the changes made to the map. Details of each command that your program must implement are shown below.
In the starter code, the program currently initialises every square in the map toEMPTY
then prints the map as a grid of integers.
Invalid Input and Assumptions
- The first number scanned in (i.e. the number of coordinate pairs specified) will always be valid. It will always be a number greater than zero.
- It is possible for the first three integers (
row column length
) to result in a line which is partially or wholly outside of the map. If this is the case, you should ignore this line completely and not make any changes to the map.
- You can assume the third integer (length) will always be positive.
- The fourth integer can be assumed to be valid. For this stage it will always be
1
(you don't have to check this).
Moving the Player
Summary: Move Player Command
Command Name |
"Move Player" |
---|
Command Number |
1 |
---|
Inputs |
direction
|
---|
Examples |
Command |
Meaning |
---|
1 -1
|
Move the player once to the left.
|
1 1
|
Move the player once to the right.
|
1 2
|
Invalid. Don't make any changes.
|
|
---|
For the next part of stage 1, you will implement a command to move the player to the left or right on the screen.
The first integer of any move player command will always be a1
. The second integer represents the direction.-1
means to move the player to the left and1
means to move the player to the right.
Information about the player's current position is given to theprintMap
function, so it is recommended to update theplayerX
variable in the main function when this command is received. This will have the effect of changing the position of the P printed byprintMap
the next time it is called.
Note that after each command has been scanned in and processed the map is printed once again.
./freefall
How many lines of stone? 1 Enter lines of stone: 0 3 10 1 0 0 0 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 1 -1 0 0 0 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 1 1 0 0 0 1 1 1 1 1 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P
./freefall
How many lines of stone? 1 Enter lines of stone: 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 1 5 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P
Invalid Input and Assumptions
- The second integer of a move player command may be something else other than
-1
or1
. In this case, your program should print out the map unchanged.
- Your program may be given a sequence of move player commands which would theoretically move the player off the map. Ensure the player is always below a column of the map. If the player is on an edge column and a move player command is received which would push it over the edge, your program should print out the map unchanged.
Firing the Laser
Summary: Fire Laser Command
Command Name |
"Fire Laser" |
---|
Command Number |
2 |
---|
Inputs |
(none)
|
---|
Examples |
Command |
Meaning |
---|
2
|
Fire the laser upwards.
|
|
---|
For the final part of stage 1, you will implement a command to fire the laser and destroy some blocks directly above the player.
This time the first integer of this command will always be a2
. There will not be any additional integers after this.
This command means to fire the laser at the player's current column. The laser will destroy at most 3 stones above the player. Destroying a stone can be done by changing the value in the array fromSTONE
toEMPTY
.
If there are more than three stones in the player's current column, the closest three stones should be destroyed only.
Hint:Even though you are looping through a 2D array, only onewhile
loop is required to complete this command as you are looping through a straight line in the array.
./freefall
How many lines of stone? 4 Enter lines of stone: 0 6 3 1 1 6 3 1 2 6 3 1 3 6 3 1 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 2 0 0 0 0 0 0 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 2 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 1 -1 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 2 0 0 0 0 0 0 1 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P
Invalid Input and Assumptions
- Since there is only one number in this command, there are no invalid input possibilities.
- Note that there may be no stones in the player's current column, in which case no changes should be made.
It is possible to score a passing mark in this assignment with the completion of stage 1 only.
You can run the autotests for stage 1 by running the following command:
1511 autotest-stage 01 freefall
Stage 2
In stage 2, you will be implementing a command to shift everything in the map down by one position, and you will be implementing functionality to determine whether the game has been won or lost.
Shift Everything Down
Summary: Shift Everything Down Command
Command Name |
"Shift Everything Down" |
---|
Command Number |
3 |
---|
Inputs |
(none)
|
---|
Examples |
Command |
Meaning |
---|
3
|
Move everything on the map down one position.
|
|
---|
For the first part of stage 2, you will need to shift all items in the array down by one position. This should occur whenever the program reads in a command of:3
.
There are no arguments after this command, it is another single integer command.
When your program shifts the contents of the array down, the integers which were in the bottom row will disappear, and the integers in the top row will all becomeEMPTY
.
Invalid Input and Assumptions
- Since this command only has one number, there is no possibility for invalid input.
./freefall
How many lines of stone? 2 Enter lines of stone: 1 1 2 1 5 5 5 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P
End Conditions
There are two ways to complete a game of Freefall:
- The game is won if the entire map is EMPTY.
- The game is lost if there is any
STONE
on the last row as a3
(shift everything down) command is given. The only case in which the game is not lost when the shift everything down command is given, is when the entire bottom row of the map isEMPTY
.
In stage 2 you must add code to check for these conditions. The win conditions should be checked after every fire laser command. If the game is won, the program should print the map once and then print "Game Won!" and end.
The lose condition should be checkedbeforethe contents of the array are shifted down. If the game is lost, the program should not shift the grid down, but should still print the map once and then print "Game Lost!" and end.
Invalid Input and Assumptions
- You can assume that there will always be at least one non-
EMPTY
block on the map when the game starts.
./freefall
How many lines of stone? 1 Enter lines of stone: 0 7 1 1 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 2 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P Game Won!
./freefall
How many lines of stone? 1 Enter lines of stone: 13 3 5 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 P 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 0 0 0 0 0 P 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 0 0 0 0 0 0 0 P Game Lost!
You can run the autotests for stage 2 by running the following command:
1511 autotest-stage 02 freefall
If you've passed all of the autotests for stage 2, you should be able to "plug" your freefall implementation into the arcade player like this:
1511 arcade freefall.c
Please note that if your program is not passing tests, or does not behave in the way1511 arcade
expects, this may not work.1511 arcade
should not be used as a debugging tool. It isnotdesigned to provide helpful information about your code.